When working with C++, you’ll often need to convert data types, especially when dealing with user inputs or file data. One of the most common conversions is from a C++ string to int.
This conversion is essential for performing arithmetic or comparison operations on data initially stored as strings, enabling efficient data processing and manipulation in C++ programs.
In this guide, we’ll explore the various ways to convert a string to an integer in C++ using built-in functions like stoi()
as well as alternative methods like stringstream
and direct parsing.
What is a String in C++?
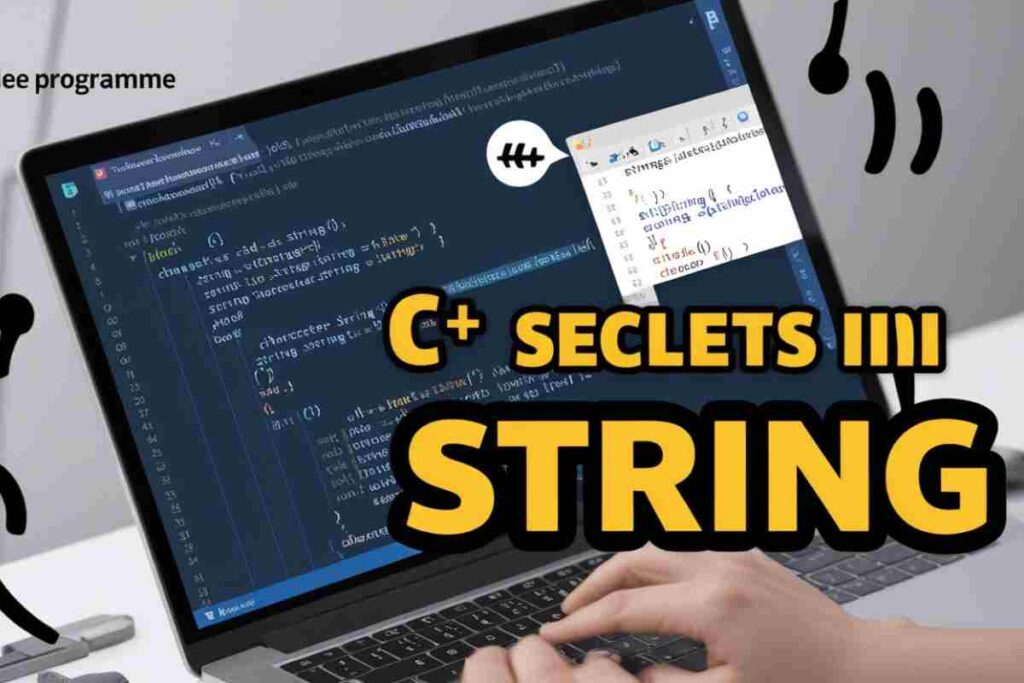
In C++, a string is a sequence of characters enclosed within double quotes. It can be manipulated in various ways, such as concatenation, comparison, and extraction of individual characters.
The C++ string type is part of the C++ Standard Library, specifically in the <string>
header.
Converting String to Int in C++ Using stoi()
The most straightforward method for converting a string to int in C++ is by using the stoi()
function, which stands for string to integer.
This function is part of the C++ Standard Library and allows you to easily convert a string representation of a number into its corresponding integer value.
Example of Using stoi()
in C++:
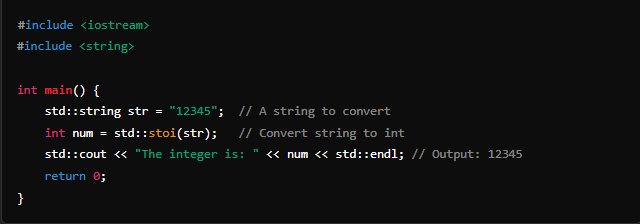
In the example above, we simply pass a string to the stoi()
function, which returns the integer representation of the string.
Key Features of stoi()
:
- It throws exceptions if the conversion fails, such as
std::invalid_argument
when the string does not contain a valid number orstd::out_of_range
when the number is too large to fit in anint
.
How to Handle Exceptions with stoi()
Since stoi()
can throw exceptions, it’s essential to handle them using try-catch
blocks.
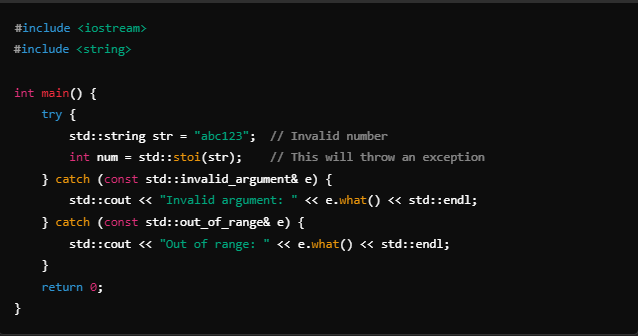
In this case, the program will catch and handle the invalid_argument
exception when attempting to convert a non-numeric string.
Convert String to Int C++ Without stoi()
While stoi()
is commonly used, it’s not the only option available. If you prefer not to use stoi()
, there are other ways to convert a string to an integer.
For example, using a stringstream
from the C++ Standard Library provides an alternative solution.
Using stringstream
to Convert String to Int in C++:
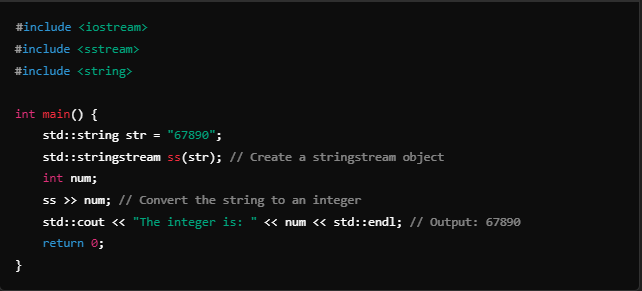
In this method, we use stringstream
to stream the string data and extract the integer. It works similarly to stoi()
, but it may offer better flexibility when dealing with complex parsing tasks.
Char to Int in C++
Another related conversion you might need is from char to int. This is particularly useful when working with individual characters representing digits.
In C++, you can convert a character representing a number to its integer value by subtracting the character ‘0’. This method works because characters corresponding to digits are stored in consecutive memory locations.
Example of Converting Char to Int in C++:
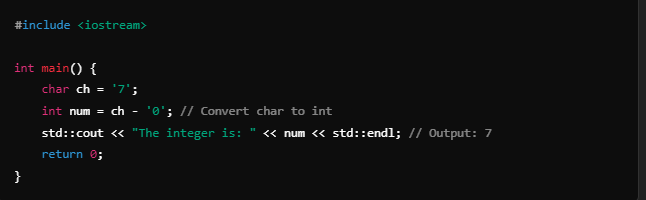
This method is especially efficient when you only need to convert individual digits from a string or character array.
Handling Edge Cases When Converting String to Int in C++
When converting a string to an integer, you must be mindful of potential edge cases. Some common scenarios include:
- Empty Strings: Converting an empty string can cause errors, and handling this case with proper checks is important.
- Leading and Trailing Whitespace: A string with spaces around the number might cause errors if not trimmed first.
- Large Numbers: Ensure that the number represented by the string is within the range of the
int
type. Larger values may require the use oflong
orlong long
.
Example: Handling Edge Cases in Conversion
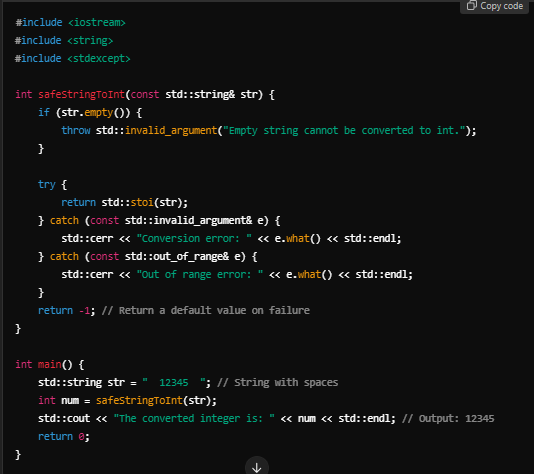
Concatenate Int to String in C++
Another common task when working with strings and integers is concatenating an integer to a string. In C++, this is typically done by converting the integer to a string and then using the +
operator or stringstream
to concatenate.
Example: Concatenate Int to String C++:
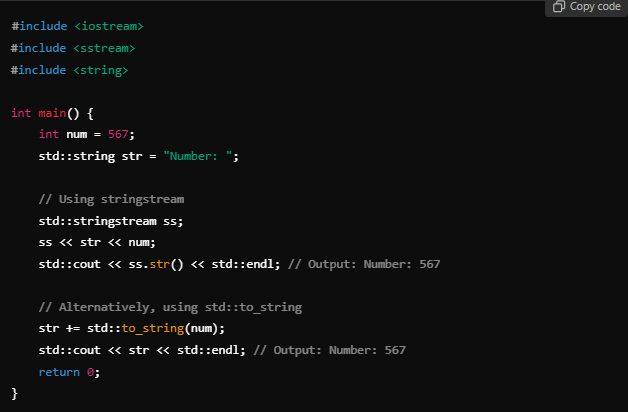
In this example, we use two methods to concatenate the integer to the string: stringstream
and to_string()
.
Conclusion
Converting strings to integers in C++ is a common task that can be done using various methods like stoi()
, stringstream
, and more, offering flexibility and efficiency.
Always handle edge cases and exceptions to prevent errors. Understanding related conversions, such as char
to int
or concatenating integers to strings, will improve your code’s reliability.
By following these techniques, you can efficiently handle numeric data in C++, making your programs more robust and error-free.
FAQs
What is the easiest way to convert a string to an int in C++?
The easiest way is to use the stoi()
function, which directly converts a string to an integer.
What happens if the string is not a valid number in C++?
If the string is invalid, stoi()
throws an std::invalid_argument
exception.
Can I convert a string with extra spaces to an int in C++?
No, leading or trailing spaces cause stoi()
to throw an exception. Trim the string first.
Is there a way to convert a string to an int without using stoi()
?
Yes, you can use stringstream
or manual parsing to convert a string to an integer.
How do I handle large numbers when converting strings to integers?
For large numbers, use stoll()
for long long
integers or stringstream
for greater control.
Can I convert a single character to an int in C++?
Yes, subtract '0'
from the character to get its integer value.
What should I do if the string contains non-numeric characters?
Handle errors using a try-catch
block to catch exceptions like std::invalid_argument
.
How can I concatenate an int to a string in C++?
You can use std::to_string()
or a stringstream
to concatenate an integer to a string.
How do I convert a C++ string to int?
You can convert a C++ string to int using the stoi()
function, which safely converts a string containing numeric characters to an integer. Alternatively, you can use stringstream
for more flexibility.