“Segmentation fault (core dumped)” is a common error message that often perplexes developers. This issue occurs when a program attempts to access restricted memory, leading to a crash.
In this blog post, we’ll explore what a segmentation fault is, the common causes behind it, and how to fix it in different programming languages and environments, including Python, PHP, and Ubuntu.
What is a Segmentation Fault (Core Dumped)?
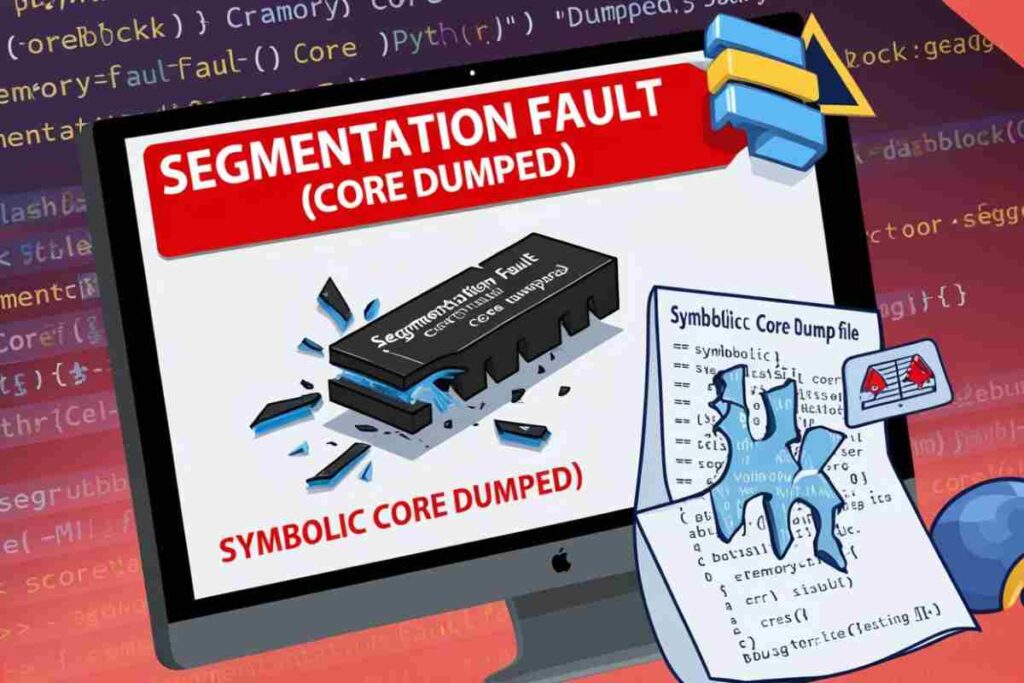
A Segmentation Fault is an error that occurs when a program tries to access a portion of memory that it’s not allowed to, often due to invalid memory access, such as reading or writing to restricted areas.
The system automatically generates a “core dump,” which is a file that contains a snapshot of the program’s memory at the time of the crash. This is often useful for debugging and understanding why the program failed.
When you see the message “Segmentation fault (core dumped)”, it usually indicates that your program has tried to perform one of the following illegal memory operations:
- Accessing memory that has not been allocated.
- Writing to a read-only memory region.
- Trying to access memory that is no longer available.
- Dereferencing a null pointer.
The core dump contains valuable data that can help you identify the specific location in your program where the fault occurred.
Understanding how to handle and prevent these errors is essential for developers working in any environment.
Causes of Segmentation Fault (Core Dumped)
Dereferencing Null or Uninitialized Pointers
In languages like C and C++, pointers are used to refer to specific memory locations. A segmentation fault commonly occurs when a pointer is dereferenced before being properly initialized or after being set to NULL.
In these cases, the program tries to access memory that it doesn’t have permission to use.
For example, in C:

This error can be avoided by always ensuring that pointers are initialized before use.
Buffer Overflows
A buffer overflow happens when a program writes more data to a buffer than it can hold. This typically leads to a segmentation fault as the program ends up overwriting memory locations that it shouldn’t be accessing.
Buffer overflows are common in C, C++, and other low-level languages, and can be very dangerous because they can lead to security vulnerabilities.
Example of a buffer overflow in C:

In this case, writing beyond the buffer’s limit would overwrite adjacent memory, leading to a segmentation fault.
Accessing Freed Memory (Dangling Pointers)
Once a pointer has been freed, it no longer points to valid memory. If the program tries to use this pointer afterward, it leads to undefined behavior and usually a segmentation fault. This situation is referred to as accessing “dangling pointers.”
Example of a dangling pointer in C:

Stack Overflow
A stack overflow occurs when the program’s stack exceeds its allocated memory limit. This often happens due to infinite recursion or excessively deep recursion. When the stack overflows, it causes the program to crash, often resulting in a segmentation fault.
Example of infinite recursion causing stack overflow:

Memory Leaks
While memory leaks themselves don’t directly cause segmentation faults, they contribute to overall system instability, potentially leading to crashes and performance degradation.
If the program consumes too much memory over time without releasing it, the system may eventually run out of memory, causing the program to crash with a segmentation fault.
How to Fix Segmentation Fault (Core Dumped) Errors
Use Debugging Tools
When a segmentation fault occurs, debugging tools like gdb (GNU Debugger) in C/C++ or pdb in Python can help you pinpoint the problem’s location. For C/C++, you can load the core dump into gdb to inspect the state of the program and the call stack.
Example for C/C++:

For Python, you can use pdb to step through the code and identify where the crash happens:

Check for Null Pointers and Proper Initialization
Always check that your pointers are not null before dereferencing them. You can also use tools like valgrind to check for memory leaks and invalid memory accesses.
Example:

Avoid Buffer Overflows
To prevent buffer overflows, ensure that you never write more data than your buffer can hold. Use safer functions like strncpy instead of strcpy, or better yet, use higher-level languages like Python that handle memory management automatically.
Manage Memory Properly
In languages like C and C++, it’s crucial to free memory that is no longer needed. However, make sure that you never access memory after it’s been freed. Use tools like valgrind to check for memory leaks and improper memory usage.
Prevent Stack Overflow with Tail Recursion
If you’re using recursion in your program, make sure to implement it in a way that avoids infinite recursion. Some compilers also support tail-call optimization, which can prevent stack overflows by reusing the current stack frame.
Segmentation Fault in Python
In Python, segmentation faults typically occur in extension modules or when using C/C++ libraries. The Python interpreter itself does not throw segmentation faults in typical Python code.
However, if you’re working with Python bindings or interfacing with low-level code, you might encounter this issue.
For example, Segmentation fault (core dumped) python can occur if you use an extension module that improperly manages memory.
To debug Python segmentation faults, use tools like gdb or Python’s faulthandler module to get a detailed stack trace.
Segmentation Fault on Ubuntu
On Ubuntu and other Linux-based systems, segmentation faults can happen for various reasons, from incorrect memory management in the code to problems with the system’s memory.
When running into a Segmentation fault (core dumped) ubuntu error, the core dump file is stored by default in the current directory or /var/crash
.
To inspect the core dump in Ubuntu, you can use the gdb tool to analyze it:

This will allow you to inspect the crash and understand what went wrong.
Segmentation Fault in PHP
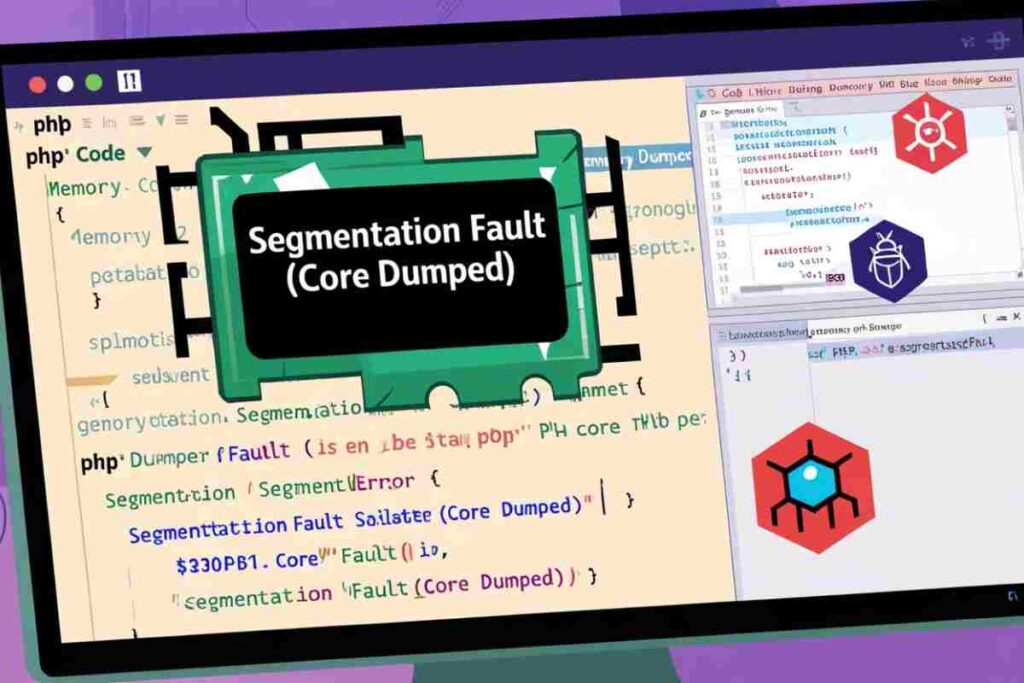
A Segmentation fault (core dumped PHP) can occur when PHP is interacting with a low-level C extension or when using a faulty PHP extension.
This typically happens if PHP code interacts with unmanaged memory (like in PHP extensions written in C or C++) that mismanages memory. To debug PHP segmentation faults, you should use gdb with PHP to locate the exact problem.
Conclusion
A Segmentation Fault (core dumped) can be frustrating, but it’s key to understanding how your program uses memory. By following best practices like proper pointer management, avoiding buffer overflows, and using debugging tools, you can reduce these errors.
Take time to debug your code using tools like gdb, valgrind, or pdb. This will not only solve the issue but also improve your knowledge of memory management and help you write more efficient, error-free code.
FAQs
What causes a segmentation fault?
A segmentation fault occurs when a program tries to access memory it’s not allowed to, like dereferencing null pointers or writing beyond buffer limits.
How can I fix a segmentation fault in C?
Ensure proper pointer initialization, check for buffer overflows, and use debugging tools like gdb to pinpoint the issue.
What is a core dump?
A core dump is a file that captures the memory of a program at the time it crashes, helping in debugging.
Does Python cause segmentation faults?
Segmentation faults in Python usually happen when using C extensions or third-party modules that mishandle memory.
How can I debug a segmentation fault in Python?
Use Python’s pdb or external debugging tools like gdb to analyze the crash and locate the issue.
Can memory leaks cause a segmentation fault?
While memory leaks don’t directly cause segmentation faults, they can lead to system instability, which may result in crashes.
What is the role of valgrind in debugging segmentation faults?
Valgrind helps detect memory issues, such as leaks and invalid accesses, which can prevent segmentation faults.
How do I prevent segmentation faults in my code?
Proper memory management, such as avoiding null pointer dereferencing, checking bounds, and using debugging tools, can prevent segmentation faults.