When working with Python, you might wonder how to use a Python do while loop effectively. While Python doesn’t have a built-in do while construct, it provides robust alternatives that can mimic this functionality.
IIn this article, we’ll explore how Python do while loops work, why Python lacks them, and how to replicate this behavior with practical examples and useful alternatives.
What is a Do While Loop?
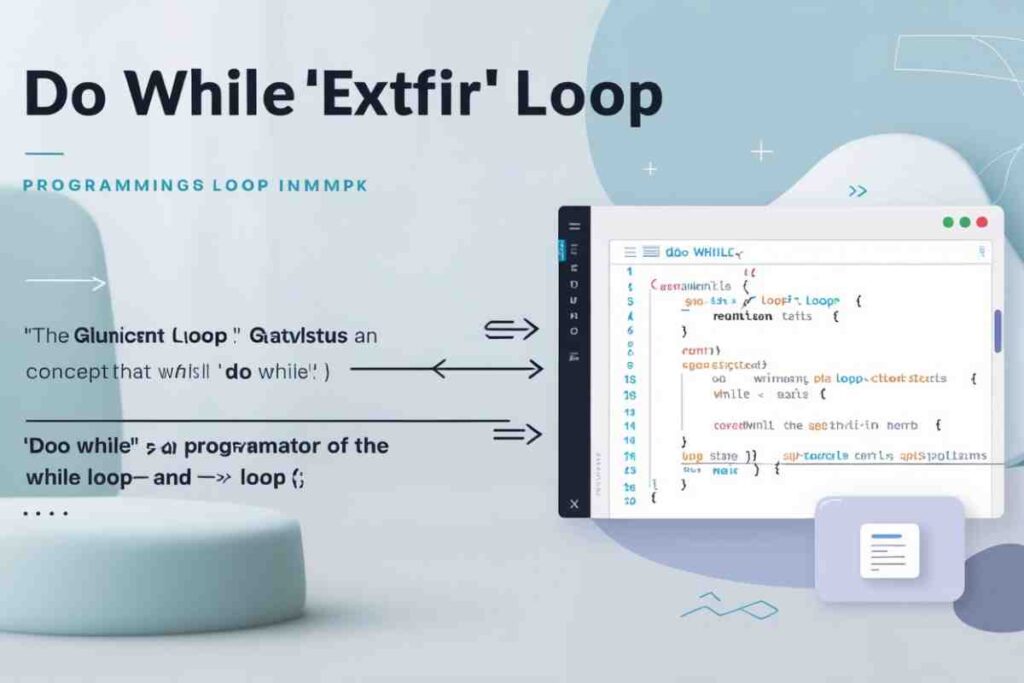
A do while loop is a control flow statement commonly used in many programming languages, such as C, C++, and Java.
The main characteristic of a do while loop is that it executes the block of code at least once before checking the condition. Here’s a general syntax::

In essence, the loop guarantees one execution regardless of the condition, making it unique compared to while or for loops.
Why Doesn’t Python Have a Do While Loop?
Python doesn’t have a built-in do while loop, and the reason lies in the language’s core philosophy of simplicity and readability.
Python prioritizes having clear, easy-to-understand syntax. By omitting a do while loop, Python reduces complexity in its control flow structures.
A Python do while loop would execute the loop’s body before evaluating the condition, ensuring at least one iteration. However, Python’s design philosophy encourages the use of while loops combined with logical patterns to achieve the same effect.
Python encourages developers to think more clearly about their loops and logic. Instead of do while loops, Python provides flexible constructs such as while loops, for loops, functions, and even try-except blocks.
Although some programmers transitioning from other languages might miss do while loops, Python’s alternatives align with its design principles of clarity and simplicity.
How to Mimic a Do While Loop in Python
To create a similar effect to a Python do while loop, you can use a combination of a while loop and specific logic to ensure the code block executes at least once. Here’s an example of a Python do while loop workaround:
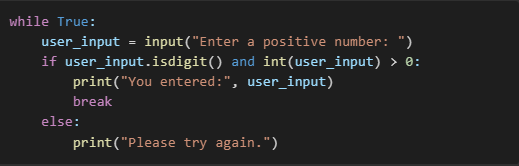
Key Takeaways:
while True
ensures the loop runs indefinitely until explicitly broken.- The break statement allows us to exit the loop after one or more iterations.
- This ensures the loop executes at least once before checking a condition.
Key Differences Between Python While Loop and Do While
Feature | Do While Loop | Python While Loop |
---|---|---|
Execution Guarantee | Runs at least once | May not run at all if the condition is initially false |
Condition Checking | Checked after the first iteration | Checked before the first iteration |
Explicit Logic | Not needed, runs by default once | Requires a while True loop and break statement to mimic behavior |
Example: Simulating Do While for User Input
Here’s another practical example of mimicking a Python do while loop:
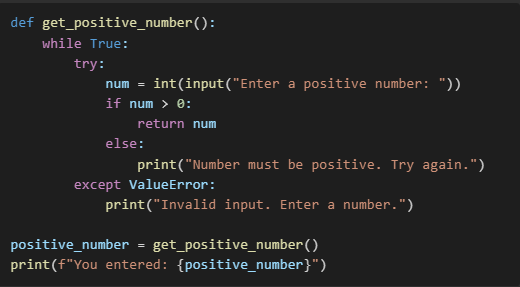
This code demonstrates a reusable function to get validated user input, showcasing a clean implementation of a Python do while loop alternative.
Common Pitfalls in Mimicking Do While Loops
While mimicking do while loops in Python, there are some common mistakes to avoid:
- Forgetting the Break Statement:
- If you forget to include a
break
statement, the loop will run indefinitely.
- If you forget to include a
- Logical Errors in Condition:
- Ensure the condition correctly mimics a do while loop, so it executes at least once and then evaluates the condition properly.
- Performance Issues with Large Iterations:
- Using
while True
can lead to inefficient execution if the termination condition is not well-optimized.
- Using
Best Practices for Using While Loops Instead of Do While Loops in Python
- Use Functions for Better Readability:
- Encapsulating logic in a function improves code readability and maintainability.
def get_valid_number(): while True: num = int(input("Enter a positive number: ")) if num > 0: return num print("Please enter a positive number.")
- Avoid Infinite Loops:
- Always ensure there’s a termination condition inside the loop to prevent infinite looping.
- Use Sentinel Values:
- Utilize a sentinel value to break out of the loop cleanly.
user_input = None while user_input != "exit": user_input = input("Enter a command (or type 'exit' to stop): ")
- Consider Exception Handling:
- For input validation, use
try-except
to catch errors gracefully.
while True: try: num = int(input("Enter a number: ")) break except ValueError: print("Invalid input, please enter a number.")
- For input validation, use
Alternatives to Do While in Python
Using Functions
Functions can encapsulate repetitive logic, making code cleaner and easier to read. Here’s an example:
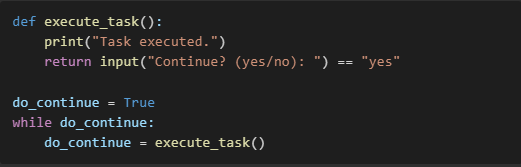
This structure ensures the code block runs at least once and allows for flexible continuation.
Combining For Loop and While Logic
Although less common, for loops in Python can also incorporate conditions to replicate do while behavior. For example:
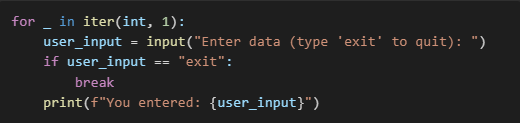
This approach uses Python’s infinite iter generator for a creative workaround.
Using Flags or Sentinel Values
Another approach is to use a flag or sentinel value to control the loop:
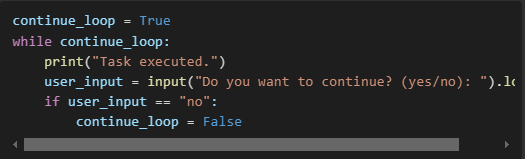
This technique provides a clear, concise way to mimic do while logic without additional complexity.
Use Cases of Do While in Python
- Menu-driven Programs: Applications with options that need to display menus repeatedly.
- Data Validation: Ensuring user inputs are valid before proceeding.
- Retry Mechanisms: Repeating tasks, such as API calls, until a successful response is received.
- Interactive Simulations: Running a simulation where at least one iteration is mandatory before reevaluating conditions.
- Debugging and Testing: Reproducing a sequence of tasks while altering conditions dynamically.
Python Do While: A Real-World Example
Let’s look at a practical, real-world example of mimicking a do while loop in Python:
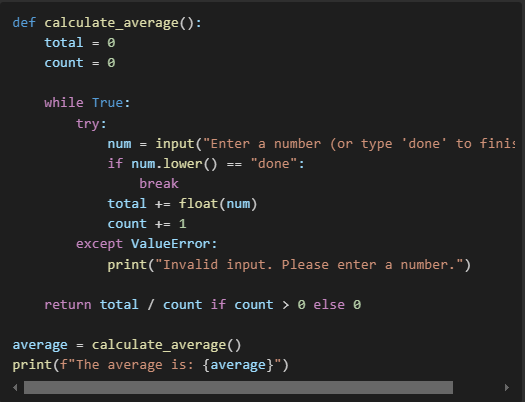
This program ensures users input data at least once, calculates the average, and gracefully handles invalid inputs.
Conclusion
Although Python doesn’t natively support the do while loop, the language’s flexibility ensures that alternatives like while loops, functions, and creative combinations can effectively achieve the same outcome. By understanding these patterns, you can write cleaner and more Pythonic code.
For more details, check out this article on Python loops or explore the differences between various loop types in Python.
The absence of a native do while loop in Python is not a limitation but an opportunity to embrace Pythonic problem-solving techniques.
FAQs
Can I use a Python while loop as a do while loop?
Yes, by ensuring the loop condition allows the block to execute at least once, you can mimic do while behavior.
Why doesn’t Python include do while loops?
Python prioritizes simplicity and readability, relying on while loops and other constructs instead.
Where can I learn more about Python loops?
You can explore resources like W3Schools’ Python loop tutorials or Real Python.
What is the key difference between do while and while in Python?
Do while ensures one execution before condition checking, unlike Python’s while loop.
How can I simulate a do while loop in Python?
Use a while True loop with break conditions to mimic do while functionality.
Is using a function better for do while simulation?
Yes, functions can make the simulation more modular and reusable in Python.
Can I use a for loop to replace do while?
Yes, with some creative use of iterators or range, you can simulate similar behavior.
What are the common use cases for mimicking do while in Python?
Data validation, menu-driven programs, and retry mechanisms are common scenarios.