R, a widely-used programming language in the data science and analytics community, offers a variety of operators to help manipulate and filter data.
One of the most powerful and frequently used operators is the “OR” operator (|), which allows users to apply conditional logic across multiple criteria.
In this article, we’ll take an in-depth look at the OR operator in R, how it functions, and some real-world examples.
We’ll also cover related concepts such as using AND and OR together, and provide insights into specific cases like “George R. Oliver fees earned or paid in cash” and RTX operations.
What is the “OR” Operator in R?
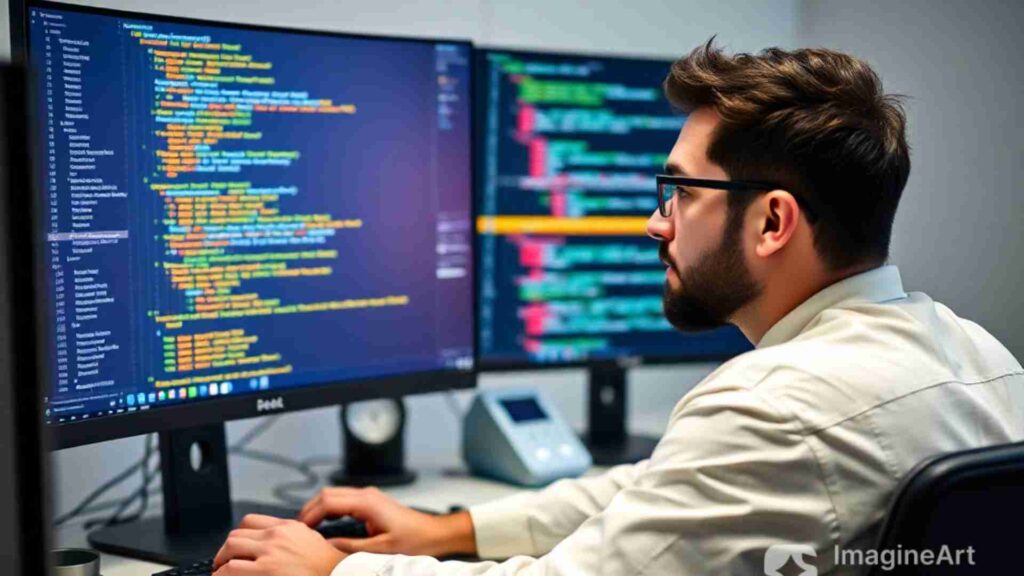
In R, the OR operator is represented by the vertical bar |. It is used to combine two or more logical conditions, returning TRUE when at least one of those conditions is satisfied.
This makes it particularly useful when you want to filter or subset data based on multiple criteria.
Basic Syntax of OR in R
The general syntax of the OR operator in R looks like this:
df[condition1 | condition2, ]
In this case, condition1 and condition2 are logical expressions, and the operator | checks whether either of the conditions evaluates to TRUE. If either condition is TRUE, the row is included in the output.
Example of Using the OR Operator in R
Let’s illustrate the usage of the OR operator with a simple example. Suppose you have a data frame containing basketball team statistics, and you want to filter for rows where the points are
#create data frame
df <- data.frame(team=c(‘A’, ‘A’, ‘B’, ‘B’, ‘B’, ‘B’, ‘C’, ‘C’),
points=c(25, 12, 15, 14, 19, 23, 25, 29),
assists=c(5, 7, 7, 9, 12, 9, 9, 4))
#filter rows where points > 20 or assists = 9
df[(df$points > 20) | (df$assists == 9), ]
Output:
css
team points assists
1 A 25 5
4 B 14 9
6 B 23 9
7 C 25 9
8 C 29 4
In this example, the filtered rows include those where either the points are greater than 20 or assists equal 9.
If you’ve ever encountered data frame warnings while filtering like “a value is trying to be set on a copy of a slice from a data frame,” check out this guide on handling data frame slicing issues
OR and AND in R
In R, the AND operator (&) and OR operator (|) are often used together to apply more complex filtering logic. The AND operator returns TRUE only when both conditions are satisfied, while the OR operator returns TRUE if at least one of the conditions is TRUE.
Example of Combining AND and OR Operators
Let’s assume you have a similar dataset and you want to filter for rows where the points are greater than 20 OR the assists are equal to 9, AND the team is ‘B’:
df[(df$points > 20) | ((df$assists == 9) & (df$team == ‘B’)), ]
This will filter rows where either the points are greater than 20, or both the assists equal 9 and the team is ‘B’.
Related Concepts: “George R. Oliver Fees Earned or Paid in Cash”
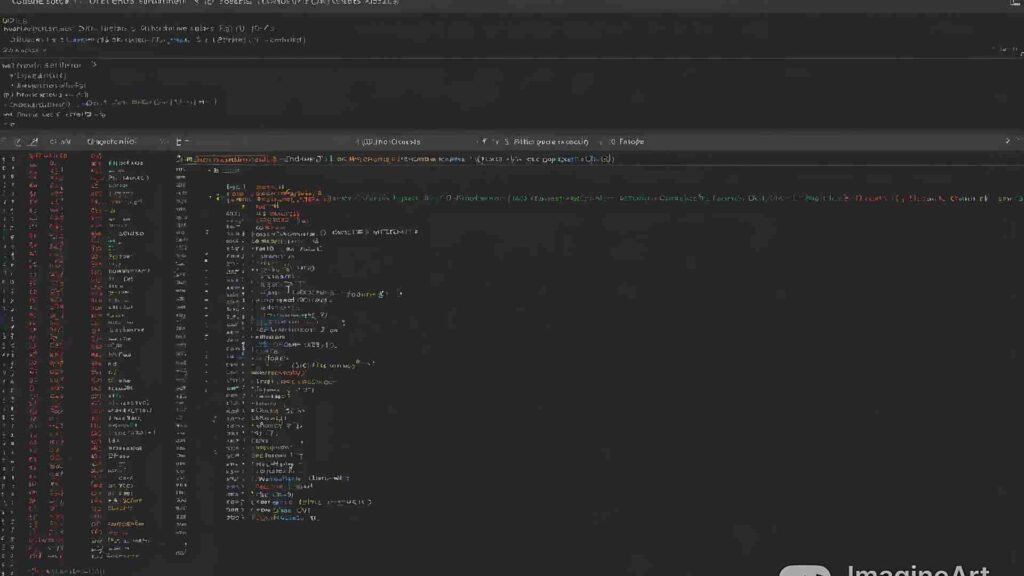
In the real world, the OR operator can also be used for business and financial operations, such as filtering rows related to transactions or payments.
For example if you’re analyzing data about George R. Oliver’s fees, specifically those that were earned or paid in cash, you might use the OR operator to filter records that match either of these criteria:
R
df[(df$fees_type == ‘earned’) | (df$payment_method == ‘cash’), ]
This would filter rows where either the fee type is “earned” or the payment method is “cash”. In finance and accounting, this is a useful way to narrow down records based on specific criteria.
Case Study: George R. Oliver RTX Fees Earned or Paid in Cash
Another related example could involve the analysis of data for RTX fees earned or paid in cash by George R. Oliver.
This scenario could involve a dataset that contains various payment methods and transaction types, and you might want to analyze the rows where either the fees were earned or paid in cash. Here’s how you would approach it:
R
df[(df$transaction_type == ‘earned’) | (df$payment_method == ‘cash’), ]
This command would help filter out records where the transaction type is “earned” or where the payment method is “cash,” allowing you to focus specifically on cash transactions or earned fees.
Advanced Use of the OR Operator in R
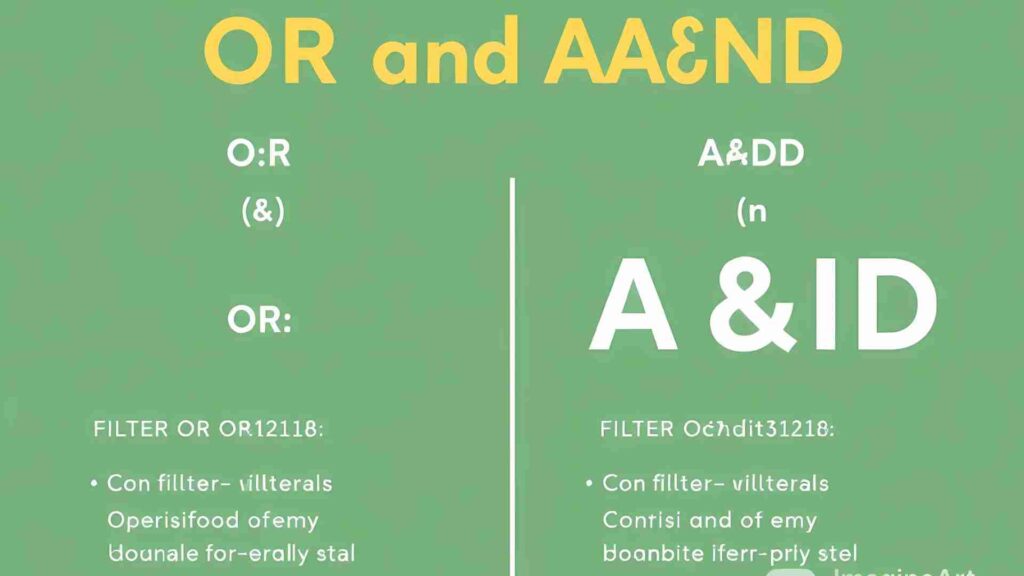
The OR operator becomes even more powerful when used in combination with other operators and functions in R. For instance, you can use it in the context of subsetting, data wrangling, or logical comparisons to analyze large datasets.
Using the OR Operator with dplyr Package
If you’re working with the dplyr package, which is widely used for data manipulation, you can combine the OR operator with functions like filter() to make your code more readable and efficient.
library(dplyr)
df <- data.frame(team=c(‘A’, ‘A’, ‘B’, ‘B’, ‘B’, ‘B’, ‘C’, ‘C’),
points=c(25, 12, 15, 14, 19, 23, 25, 29),
assists=c(5, 7, 7, 9, 12, 9, 9, 4))
# Using filter() with OR operator
df %>%
filter(points > 20 | assists == 9)
This syntax is more concise and leverages the power of dplyr for efficient data manipulation.
Conclusion
The OR operator in R (|) is a versatile tool that allows you to filter, analyze and manipulate data based on multiple conditions.
Whether you’re working with numeric values, strings or performing financial analyses like filtering “George R. Oliver fees earned or paid in cash” the OR operator helps you capture a broader range of data that meets at least one of your conditions.
Combining the OR operator with the AND operator (&) allows for even more complex filtering, making it an essential tool in any data scientist’s toolkit.
When working with large datasets this ability to apply logical comparisons is crucial for efficient and meaningful data analysis.
FAQs
What is the “OR” Operator in R?
The “OR” operator in R, represented by “|”, returns TRUE if at least one of the given conditions is met.
How is the OR Operator Used in R Syntax?
The OR operator in R combines logical conditions, like df[condition1 | condition2, ], to filter data based on multiple criteria.
Can You Give an Example of the OR Operator in R?
Yes, filtering rows where points > 20 or assists == 9 in a dataset can be done using df[(df$points > 20) | (df$assists == 9), ].
How Does the OR Operator Work with AND in R?
You can combine AND (&) and OR (|) to apply complex filtering logic, like df[(df$points > 20) | ((df$assists == 9) & (df$team == ‘B’))].
How Do You Filter Data Using OR with Fees Earned or Paid in Cash?
You can filter records where fees are earned or paid in cash with df[(df$fees_type == ‘earned’) | (df$payment_method == ‘cash’), ].
What Does RTX Fees Earned or Paid in Cash Mean?
Filtering rows where RTX fees were earned or paid in cash can be done with df[(df$transaction_type == ‘earned’) | (df$payment_method == ‘cash’), ].
How Can You Use the OR Operator with dplyr in R?
With dplyr, you can filter data more efficiently using df %>% filter(points > 20 | assists == 9).
How Can the OR Operator Enhance Data Analysis in R?
The OR operator helps in capturing broader data ranges by combining multiple conditions, crucial for efficient data analysis.