In pandas, when you work with slices from a DataFrame, you may encounter an error message: A value is trying to be set on a copy of a slice from a DataFrame. This is a common issue that confuses both beginners and seasoned programmers alike.
The warning, also known as SettingWithCopyWarning
, appears when pandas detects that you are trying to modify data that might not be part of the original DataFrame.
This blog post will explain the issue in detail, how to fix it, and why it’s important to understand this warning to avoid unexpected behaviors in your data analysis.
What Does “A Value is Trying to Be Set on a Copy of a Slice from a DataFrame” Mean?
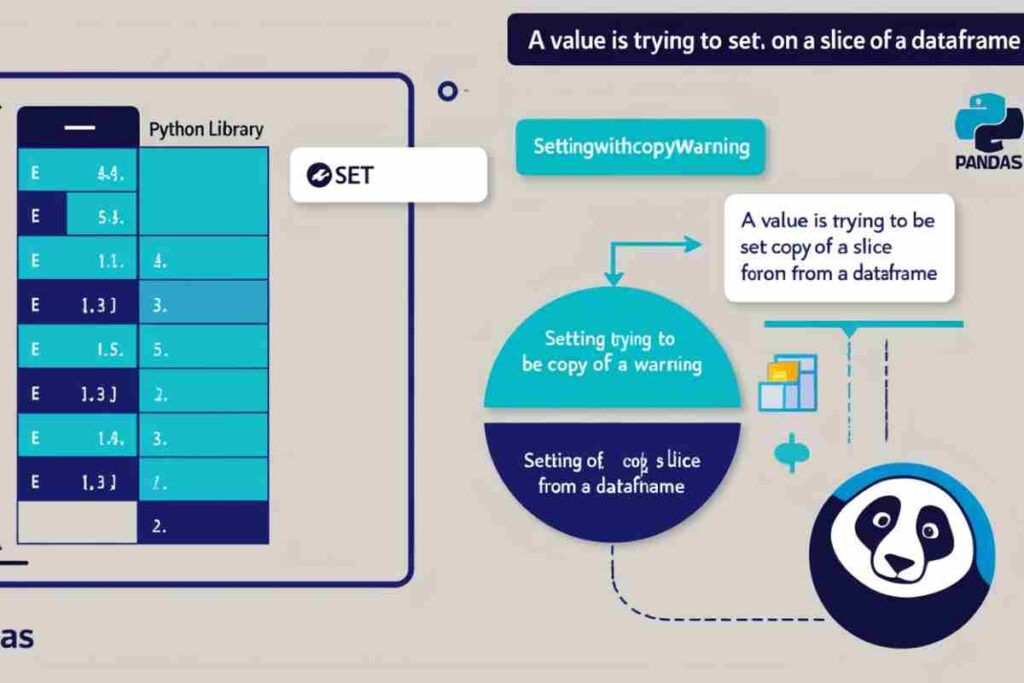
The warning “A value is trying to be set on a copy of a slice from a Data-Frame” is raised when pandas detects an operation where it’s unclear whether you are working with a copy or a view of a Data-Frame.
A view is a reference to the original data, and changes to a view will directly modify the original data. A copy, on the other hand, is a separate object, and modifications to a copy will not affect the original data.
This distinction is crucial when you are working with pandas DataFrames, as modifying a copy unintentionally could lead to confusion and errors in your data.
What is the SettingWithCopyWarning?
The SettingWith
CopyWarning
is pandas‘ way of alerting you that you are performing an operation that could cause ambiguity. This is important because, when you try to set a value on a copy of a slice, there’s no guarantee that the change will be reflected in the original Data-Frame.
In other words, if you modify a copy, you might end up with inconsistent data. While it may not always result in an error, it can cause problems when working with large datasets or when you expect changes to be reflected in your original Data-Frame.
Why Does the Warning Appear?
This warning typically appears under these scenarios:
- Slicing a DataFrame: When you slice a Data-Frame using standard indexing or conditions, pandas may create a view or a copy. When you attempt to modify the values in the resulting slice, pandas cannot determine if you are modifying the original data or just a copy.
- Chained Assignment: This occurs when you try to set a value on a DataFrame slice using a chain of indexing operations. For example, performing something like
df['col'][i] = value
can result in this warning because it’s unclear whether you’re modifying the DataFrame directly or working on a temporary copy. - Ambiguous Operations: Operations that pandas cannot clearly categorize as modifying a copy or a view will trigger the
SettingWithCopyWarning
. This ambiguity arises from pandas’ internal optimization for performance, where sometimes slices are returned as views and sometimes as copies.
How to Fix “A Value is Trying to Be Set on a Copy of a Slice from a DataFrame”?
Fixing this warning involves ensuring that pandas knows whether you’re working with a copy or a view. Here are some effective solutions:
Use .loc[]
for Assignment
One of the most effective ways to avoid this warning is to use the .loc[]
indexer when setting values in a DataFrame. .loc[]
is designed to work with DataFrames and ensures that you’re modifying the original DataFrame, not a copy. For example:

In this case, .loc[]
helps ensure that you’re working with the original DataFrame rather than a slice.
Avoid Chained Assignment
Chained assignment refers to performing multiple indexing operations in a single line. This is a common cause of the SettingWithCopyWarning
. For example:

This operation first slices the DataFrame based on a condition, then attempts to modify the values. To avoid this, you should use .loc[]
instead, which ensures the operation is done directly on the original DataFrame.

This way, pandas will not be confused about whether you’re modifying the original data or a copy.
Check if You Are Working with a Copy or a View
To avoid the SettingWithCopyWarning
, you can also explicitly check if you’re working with a view or a copy.
For example, using the .copy()
method ensures that you’re working with a separate copy of the data, which can then be modified without issues:

Use SettingWithCopyWarning Ignore
(Not Recommended)
If you’re aware of the warning and want to ignore it temporarily, you can use the pd.set_option()
function to suppress the warning:

However, it’s not recommended to completely ignore the warning because it can lead to unexpected behavior in your analysis. Instead, focus on fixing the underlying cause of the issue.
Best Practices to Avoid the SettingWithCopyWarning
To prevent encountering the SettingWithCopyWarning
in the first place, it’s a good practice to follow these strategies:
- Use
.loc[]
or.iloc[]
for all assignments: These are more reliable and avoid ambiguity. - Avoid chained indexing: Always access DataFrame elements in a clear and explicit manner.
- Ensure you understand when pandas creates a copy or a view: This helps you avoid mistakenly modifying data that isn’t part of the original DataFrame.
Common Pitfalls and How to Avoid Them
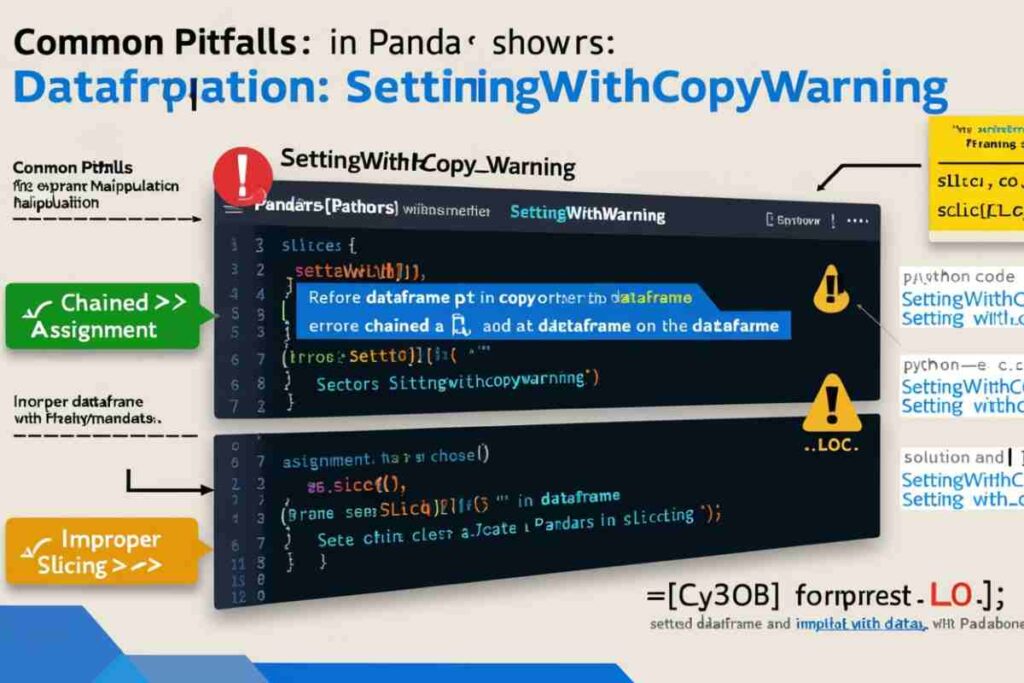
Let’s take a look at some common mistakes that lead to this warning:
Slicing without .loc[]
When slicing a DataFrame and then trying to modify the slice, pandas cannot guarantee whether it’s a view or a copy, which triggers the warning. For example:

This should be rewritten as:

Assigning to a Non-existent Column
Attempting to assign a value to a non-existent column in a slice will also raise issues:

Instead, use .loc[]
for both filtering and assignment:

Conclusion
In conclusion, the warning “A value is trying to be set on a copy of a slice from a DataFrame” is a helpful signal from pandas that warns you about potential issues with modifying data in an unclear way.
By understanding what triggers the SettingWithCopyWarning
and using the appropriate solutions like .loc[]
and avoiding chained assignments, you can ensure that your data manipulations are done safely and as expected.
While it’s possible to suppress the warning with SettingWithCopyWarning ignore
, it’s always better to address the underlying cause to maintain the integrity of your data analysis.
FAQs
What is the SettingWithCopyWarning in pandas?
The SettingWithCopyWarning
is a warning triggered when pandas detects ambiguity in modifying a DataFrame slice, potentially affecting the original data.
How do I fix “A value is trying to be set on a copy of a slice from a DataFrame”?
Use .loc[]
for assignment to avoid ambiguity and ensure you’re modifying the original DataFrame.
Why does pandas raise the SettingWithCopyWarning?
This warning appears when pandas is unsure whether you’re modifying a view or a copy of a DataFrame, which could lead to unintended changes.
Can I ignore the SettingWithCopyWarning?
You can ignore it temporarily with pd.set_option('mode.chained_assignment', None)
, but it’s better to fix the root cause to avoid errors.
What is chained assignment in pandas?
Chained assignment occurs when you perform multiple indexing operations in one line, leading to ambiguity about whether you’re modifying a view or a copy.
How can I safely modify a DataFrame without triggering the warning?
Use .loc[]
to modify DataFrame values explicitly, ensuring you’re working with the original DataFrame.
What does the .copy()
method do in pandas?
The .copy()
method creates a duplicate of a DataFrame, so modifications on the copy don’t affect the original data.
Is it always necessary to use .loc[]
to avoid the warning?
Yes, using .loc[]
is the recommended approach to avoid the warning and ensure you’re safely modifying your DataFrame.