If you’ve worked with C or C++, you’ve likely encountered size_t
. But what exactly is it, and why is it essential?
size_t
is a special unsigned integer type that plays a crucial role in memory allocation, array indexing, and standard library functions.
In this comprehensive guide, we’ll explore the definition, usage, advantages, and key differences between siz
e and other integer types like int
.
What is size_t in C and C++?
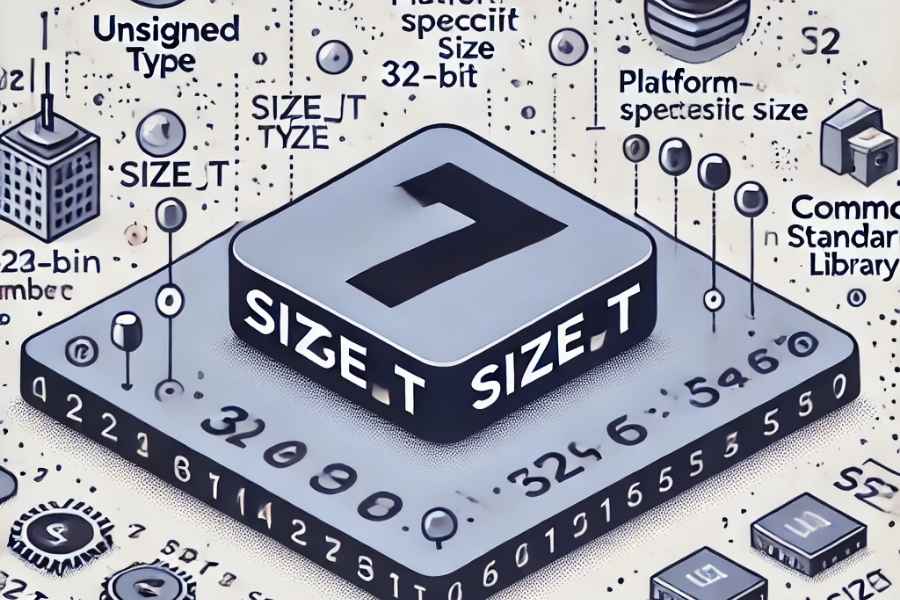
Size_t
is a type alias defined in the <cstddef>
and <stddef.h>
headers in C++. It is primarily used to represent sizes of objects in memory.
The type is platform-dependent and is typically chosen to be large enough to accommodate the maximum possible object size.
Key Characteristics:
- Unsigned Type: It cannot hold negative values.
- Platform-Dependent: Its size varies based on the architecture (32-bit or 64-bit systems).
- Standardized: Defined in the
<stddef.h>
and<cstddef>
headers. - Memory-Specific: Used primarily for measuring memory sizes and safe indexing.
Why Use size_t Instead of int?
Using size t
instead of int
offers several advantages:
- Prevents Negative Values: Since it is unsigned, it eliminates errors related to negative indices.
- Handles Large Data Sizes: On 64-bit systems, it can store larger values than
int
. - Improves Portability: It ensures compatibility across different platforms and compilers.
- Optimized for Memory Operations: Many standard functions use it for safe memory handling.
Size and Range of size-t
The size of size-t
depends on the system architecture:
System Architecture | Size | Maximum Value |
---|---|---|
32-bit | 4 bytes | 4,294,967,295 (2^32 – 1) |
64-bit | 8 bytes | 18,446,744,073,709,551,615 (2^64 – 1) |
size t vs int: Key Differences
Feature | size_t | int |
Signed/Unsigned | Unsigned | Signed/Unsigned |
Size | Platform-dependent | Platform-dependent |
Usage | Memory allocation, array indexing | General-purpose operations |
Risk of Negative Values | No | Yes |
Header File | <stddef.h> or <cstddef> | None required |
Common Uses in C and C++
The size-t
type is widely used in both C and C++ for handling memory sizes, loop iterations, and data structure operations. In C, it is often used with functions like malloc()
, strlen()
, and memcpy()
, while in C++, it is commonly seen in STL containers like std::vector
and std::string
.
Memory Management
#include <stdlib.h>
#include <stdio.h>
int main() {
size_t num = 10;
int* arr = (int*) malloc(num * sizeof(int));
printf("Memory allocated for %zu elements.\n", num);
free(arr);
return 0;
}
String Handling with strlen()
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, World!";
size_t length = strlen(str);
printf("String length: %zu\n", length);
return 0;
}
Iterating Through Arrays
#include <stdio.h>
int main() {
int arr[] = {1, 2, 3, 4, 5};
size-t length = sizeof(arr) / sizeof(arr[0]);
for (size t i = 0; i < length; i++) {
printf("%d ", arr[i]);
}
return 0;
}
Working with memcpy
#include <stdio.h>
#include <string.h>
int main() {
char src[] = "Hello";
char dest[10];
memcpy(dest, src, strlen(src) + 1);
printf("Copied String: %s\n", dest);
return 0;
}
Advanced Topics: Real-World Applications
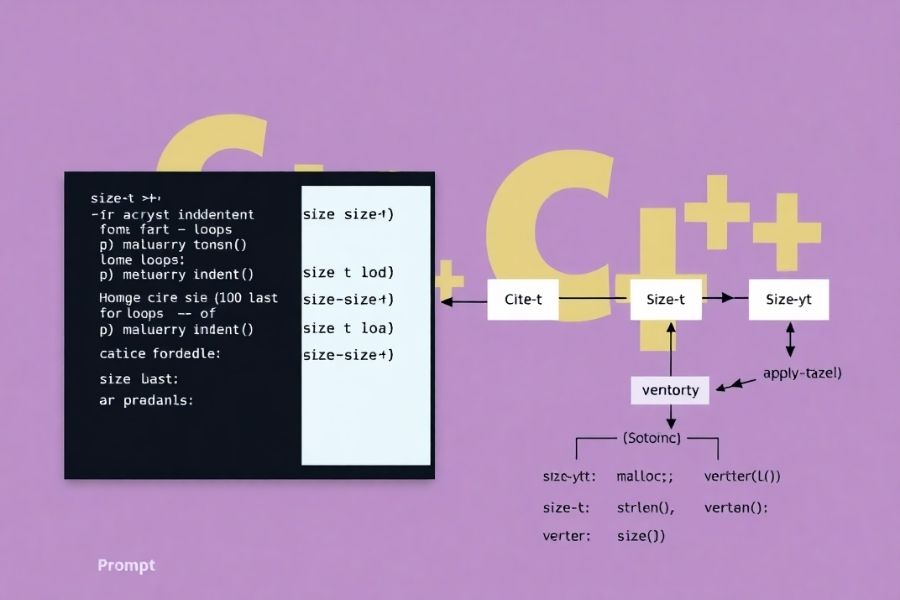
handling large datasets, and optimizing performance in system-level programming. It is often used in high-performance computing, embedded systems, and large-scale data processing tasks where precise memory control is necessary.
Usage in STL Containers
size-t
is commonly used in the Standard Template Library (STL) to define container sizes:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {10, 20, 30};
for (size-t i = 0; i < numbers.size(); i++) {
std::cout << numbers[i] << " ";
}
return 0;
}
Best Practices
- Always use
size-t
for sizes, array indexing, and loop counters when dealing with standard library functions. - Avoid mixing
size -t
with signed integers to prevent unintended bugs. - Use explicit type conversions when necessary to avoid warnings or error
How It Helps in Performance Optimization
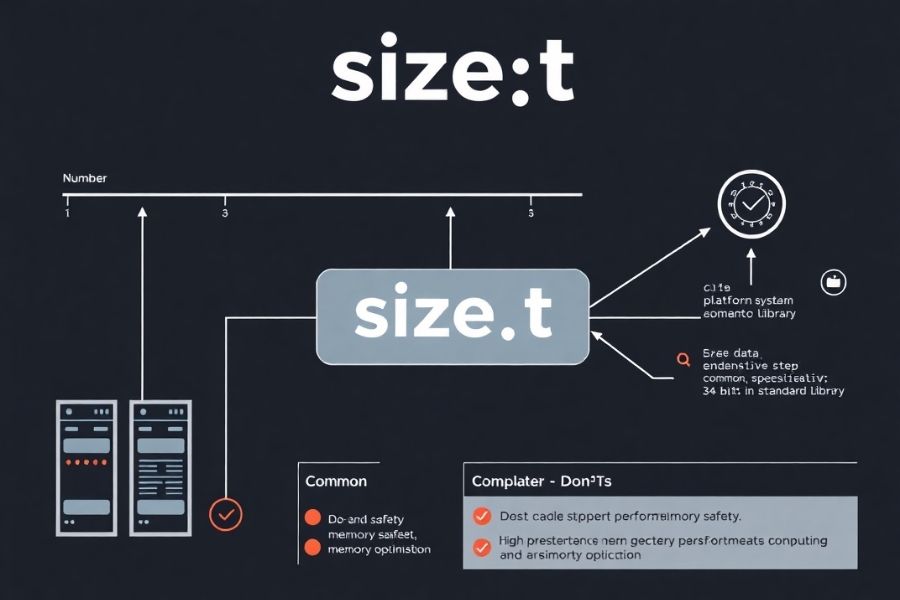
Since size_t
is optimized for memory operations, using it reduces overhead and enhances performance in large-scale applications, particularly in:
- File processing
- Data-intensive computations
- Dynamic memory allocation in high-performance computing
Conclusion
sizet is a fundamental type in both C and C++, designed for safe memory handling, array indexing, and efficient performance. In C, size_t
is widely used for functions like malloc()
, strlen()
, and memcpy()
, ensuring safe memory allocation and manipulation. In C++, it is commonly used in STL containers like std::vector
and std::string
to handle sizes and indexing without overflow risks.
The primary advantage of using size t in C is its ability to prevent negative values in array indexing and loop counters, making code more reliable and portable across different architectures. Similarly, size t in C++ improves the safety and performance of container-based operations, reducing signed-unsigned conversion issues.
By choosing size t over standard integer types, developers can optimize memory usage and ensure compatibility across 32-bit and 64-bit systems. Its use enhances portability, efficiency, and program stability, making it an essential part of modern C and C++ programming.
FAQs
What is size_t
?
size t is an unsigned integer type used to represent sizes of objects in memory and is the return type of sizeof
and alignof
.
What is size_t
in C?
In C, size_t
is defined in <stddef.h>
and other standard headers. It is primarily used for memory-related functions like malloc()
, strlen()
, and memcpy()
.
What is size_t
in C++?
In C++, size_t
is defined in <cstddef>
and is commonly used in STL containers like std::vector
and std::string
for indexing and size representation.
Why is size_t
used in C?
size_t
ensures safe memory allocation, indexing, and loop iterations by preventing negative values and supporting large object sizes.
Why is size_t
used in C++?
It improves portability and efficiency when handling container sizes and memory operations, ensuring compatibility across different architectures.
How does size_t
improve C programming?
It eliminates integer overflow risks in array indexing and memory allocation, making code safer and more reliable.
How does size_t
improve C++ programming?
In C++, size_t
ensures STL containers operate safely across different systems without unexpected signed/unsigned conversion issues.
What are the key differences between size_t
in C and C++?
Both languages use size_t
for memory management, but in C++, it is more frequently used in STL containers and is available in the std
namespace.