When working with Python, managing package versions can become a tricky task, especially when different projects require different versions of the same library.
This process will help you manage dependencies efficiently, avoid conflicts, and ensure compatibility with your project requirements.
Whether you’re upgrading a package or need to install an older version, learning how to install a specific version using pip
is crucial for maintaining a stable development environment.
In this comprehensive guide, we will walk you through how to use the pip install
command to install specific versions of Python packages.
What Is Pip and Why Is It Important for Managing Python Packages?
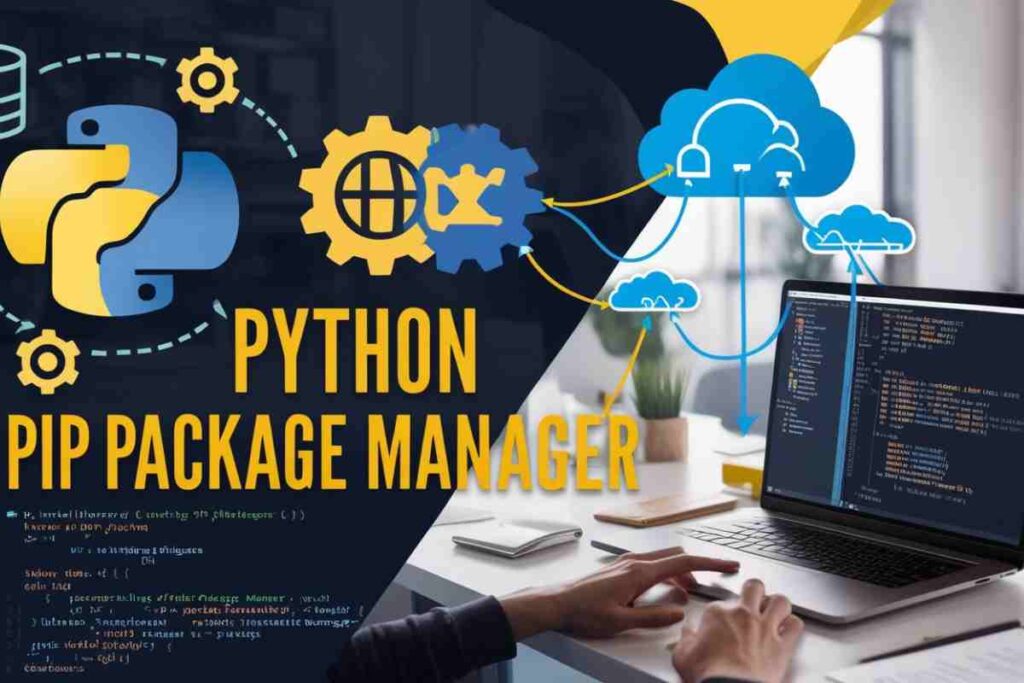
pip
is the most widely used package management tool in Python. It allows developers to install, upgrade, and manage libraries and dependencies for their Python projects.
Using pip
, you can easily install the latest versions of packages, specify versions, or even upgrade/downgrade packages to match specific project requirements.
Using the pip install
command for installing specific versions of packages is essential in avoiding compatibility issues, particularly when projects require certain features or bug fixes that are only available in certain versions of a package.
How to Pip Install Specific Version of a Package
The standard pip install
command installs the latest version of a package available in the Python Package Index (PyPI). To install a specific version of a package, you can specify the version number after the package name using the ==
operator. For example:

Here, 1.0.0
is the version of the package you want to install. This will ensure that the exact version specified is installed. For example, if you want to install requests
version 2.18.4
, you would run:

Installing a Specific Version with Other Operators
pip
also supports other version specifiers, which allow you to be more flexible with the versions you install. Some useful version specifiers include:
>=
: Install a version greater than or equal to a specified version.

<=
: Install a version less than or equal to a specified version.

>
: Install a version greater than a specified version.

<
: Install a version less than a specified version.

These version specifiers allow you to be more specific in selecting the range of versions that are compatible with your project.
How to Upgrade or Downgrade Packages Using Pip
In some cases, you may need to upgrade or downgrade a package to a specific version. You can do this by specifying the desired version number after the package name using ==
.
Pip Upgrade to Specific Version
If you already have a package installed and want to upgrade to a particular version, simply run the following command:

The --upgrade
flag ensures that pip
will uninstall the current version and install the new one. For instance, to upgrade the requests
library to version 2.25.0
, you can run:

Pip Install Latest Version
If you want to install the latest version of a package, you can use the following command:

This will automatically fetch the latest version available on PyPI. For example:

This will install the latest stable version of requests
. However, if you’re working in a project where a specific version is required, using the ==
operator will prevent potential compatibility issues.
Install Specific Version of Python Package in Virtual Environments
When managing different Python projects, it’s often a good idea to use virtual environments. These isolated environments allow you to install specific versions of libraries without affecting your global Python environment.
How to Install Specific Version in Virtual Environment
To create a virtual environment and install a specific version of a package, follow these steps:
1.Create a virtual environment:

2. Activate the virtual environment:
- For Windows:

- For Linux/Mac:

3. Install the specific version of the package:

For example, to install requests
version 2.18.4
within your virtual environment:

This ensures that your project uses the required version without interfering with other projects.
Pip Install for Specific Python Version on Windows
In some cases, you may want to install a package for a specific version of Python, especially if you’re running multiple Python versions on your system.
How to Install Packages for Specific Python Version
On Windows, you can specify which Python version you want to use with pip
by calling the pythonX.Y
version directly. For example, if you have Python 3.7 and 3.9 installed, you can install packages for Python 3.7 by running:

This ensures that the package is installed for Python 3.7, even if you have other versions installed.
Install Specific Version of Python on Your System
In some situations, you may need to install a specific version of Python itself, especially if you’re working with older projects that depend on an earlier Python version. Here’s how you can do that:
- Using
pyenv
: For Linux and MacOS, you can usepyenv
to install and manage multiple Python versions on your system. - Windows: You can download specific Python versions from the official Python website.
Once the desired Python version is installed, you can create a virtual environment for that specific version and proceed with installing the necessary packages.
Handling Dependencies with pip freeze
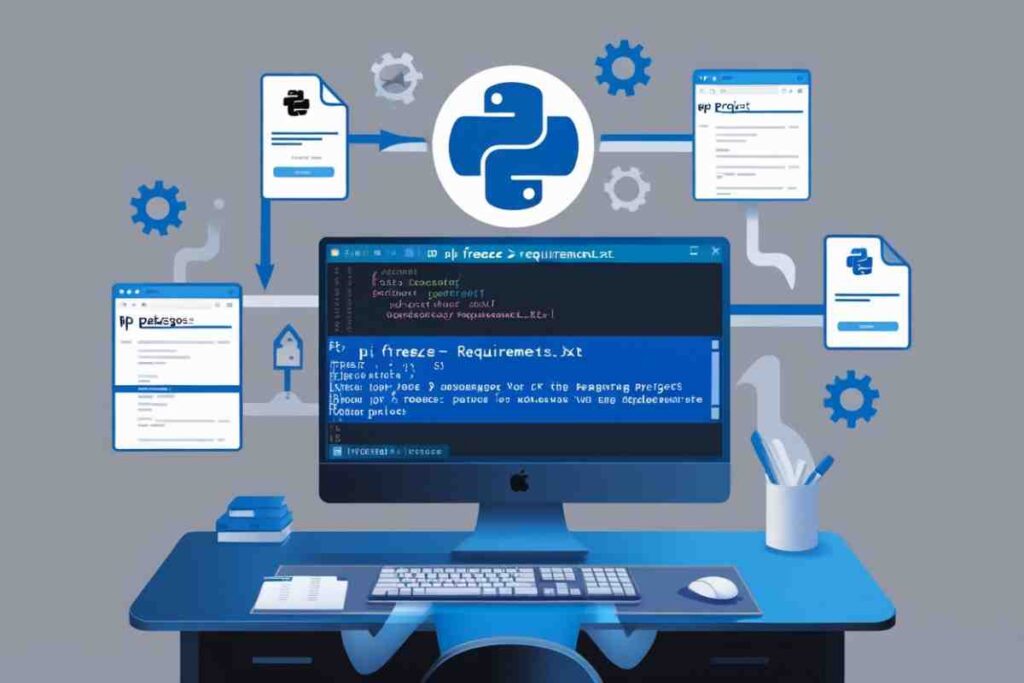
If you’re working with multiple versions of packages, it’s helpful to generate a requirements.txt
file using pip freeze
. This file will list all the dependencies with their versions.
You can generate a requirements.txt
by running:

This file can be used to recreate your environment with the exact versions of packages required for your project.
Installing Dependencies from requirements.txt
To install dependencies from a requirements.txt
file, run:

This ensures all the specified versions of the libraries are installed for your project.
Conclusion
Learning how to pip install specific version of a Python package is an essential skill for any Python developer, ensuring smooth project workflows and stability.
Whether you’re upgrading, downgrading, or just managing dependencies within a virtual environment, pip
gives you the flexibility you need to keep your projects running smoothly.
By using specific version numbers or version specifiers, you can ensure that your dependencies are compatible with your project requirements.
If you need to install for a particular version of Python, pip
can help with that as well, ensuring compatibility across different environments.
FAQs
How do I install a specific version of a package?
Use the command: pip install package-name==version-number
, for example, pip install requests==2.18.4
.
Can I upgrade a package to a specific version?
Yes, use: pip install --upgrade package-name==version-number
.
How do I downgrade a package using pip?
To downgrade, run: pip install package-name==older-version
.
How can I check the installed version of a package?
Use: pip show package-name
to display the installed version.
Can I install multiple specific versions at once?
Yes, list them in a requirements file, then run: pip install -r requirements.txt
.
How do I install a specific version in a virtual environment?
First activate the virtual environment, then run: pip install package-name==version-number
.
Can I install packages for a specific Python version?
Yes, use: pythonX.Y -m pip install package-name
, replacing X.Y
with your desired Python version.
What if a version of a package is not available?
Check PyPI or try using a different version specifier with pip.