C++ is a powerful and flexible programming language that allows developers to create efficient software for a variety of applications.
One of the most important features in C++ that enhances its power and flexibility is the template mechanism.
This article will explore the concept of C++ templates, their syntax, usage, and the various types of templates that make C++ a powerful language for high-performance computing.
What is a C++ Template?
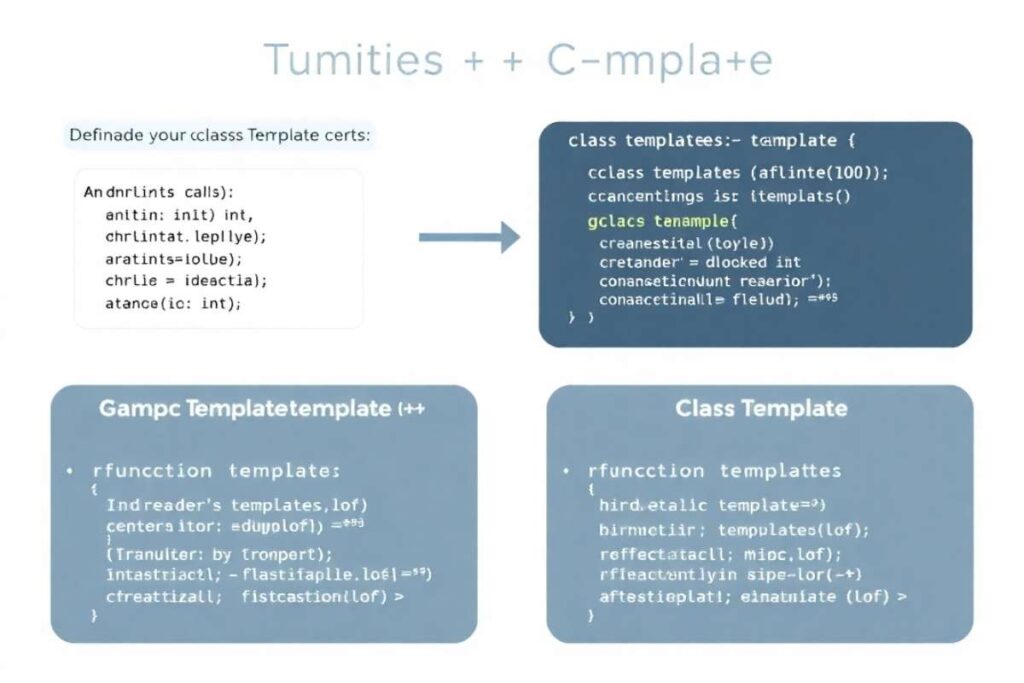
A C++ template is a feature that allows the creation of functions, classes, and other constructs that can work with any data type.
Instead of defining separate functions or classes for each data type, C++ templates enable you to create a single blueprint or generic function/class that can handle multiple data types.
In essence, C++ templates allow developers to define a function or class without specifying the exact type. The type is determined when the template is instantiated with a particular data type.
Types of C++ Templates
There are two primary types of C++ templates:
- Function Templates: These templates allow you to define a generic function that can work with any data type.
- Class Templates: These templates allow you to define a generic class that can handle any data type.
Both function and class templates improve code modularity and reuse, making it easier to work with different data types.
How Do C++ Templates Work?
When a C++ template is used in a program, the compiler generates the appropriate function or class based on the data type provided.
This process is called template instantiation. When you call a template function or create an instance of a template class, the compiler creates the specific implementation of the function or class for the provided data type.
Compile-Time Evaluation
One of the most powerful aspects of C++ templates is that they are evaluated at compile-time, not at runtime.
This means that the code generated by the template is optimized for the specific data types, and there is no runtime overhead associated with the use of templates.
The compiler essentially writes the specialized code behind the scenes, making it as efficient as if you had written separate code for each type.
Syntax of a Template
The basic syntax for defining a C++ template is as follows:
cpptemplate <typename T>
T functionName(T arg) {
// function body
}
Here, T
is a placeholder for the data type that will be determined when the template is instantiated.
For classes, the syntax is similar:
cpptemplate <typename T>
class ClassName {
T value;
public:
ClassName(T val) : value(val) {}
T getValue() { return value; }
};
In both examples, typename T
indicates that T
is a template parameter. When the function or class is used, T
will be replaced by the appropriate data type.
Function Templates in C++
A function template is a generic function that can accept any data type as its argument. This allows you to define a single function that works with multiple types. Here’s an example:
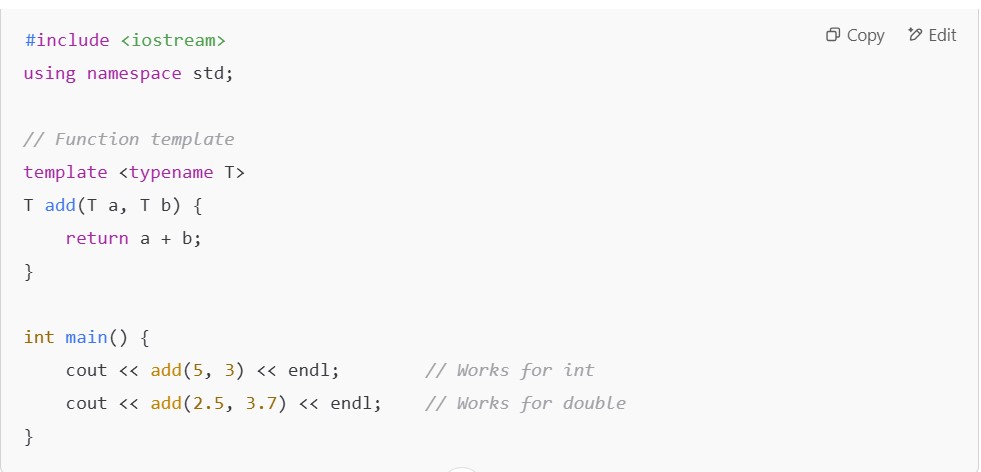
C++ Templates
In this code, the add
function is a C++ template that works with both int
and double
types. The compiler generates a separate version of the add
function for each data type when the template is instantiated.
Function Template Specialization
Sometimes, you may want to provide a different implementation for a specific type. This is where template specialization comes in. Here’s an example of how you can specialize a function template for a specific type, like char
:
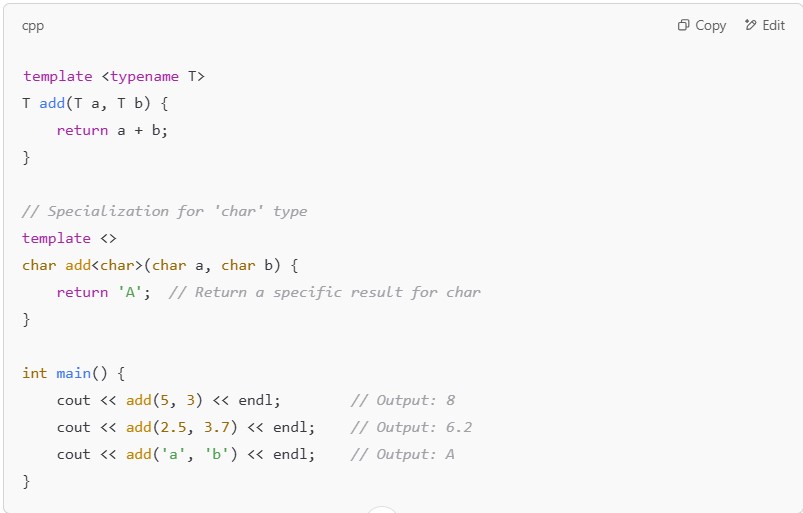
cpptemplate <typename T>
T add(T a, T b) {
return a + b;
}
// Specialization for 'char' type
template <>
char add<char>(char a, char b) {
return 'A'; // Return a specific result for char
}
int main() {
cout << add(5, 3) << endl; // Output: 8
cout << add(2.5, 3.7) << endl; // Output: 6.2
cout << add('a', 'b') << endl; // Output: A
}
In this case, the function template is specialized for char
data type, providing a custom implementation.
Class Templates in C++
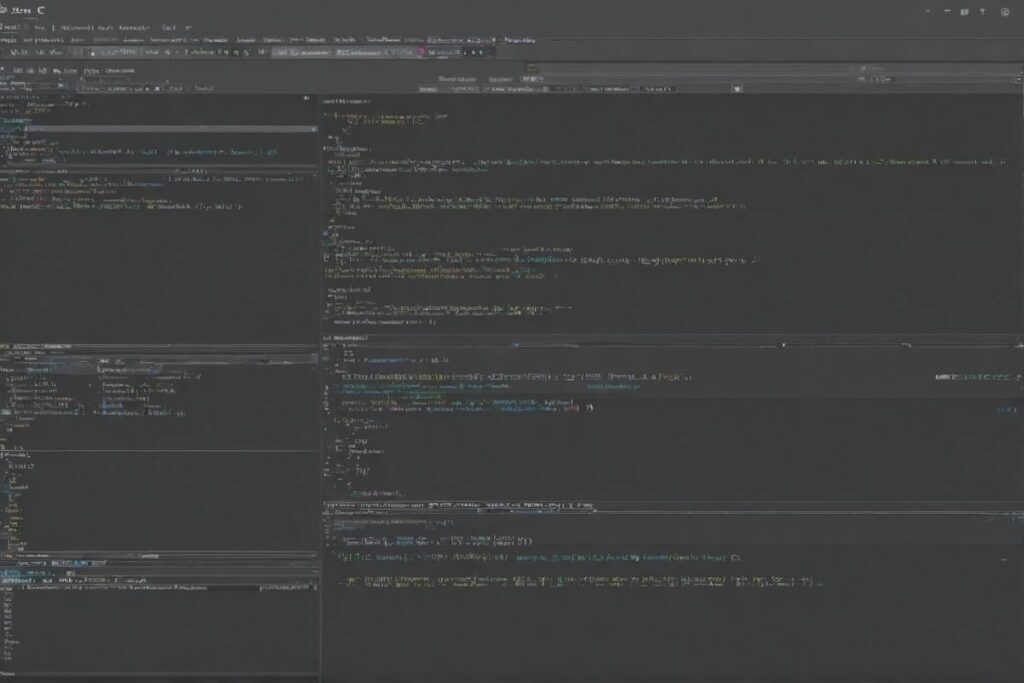
Creating Class Templates
A class template allows you to define a class that can handle any data type. Here’s an example:
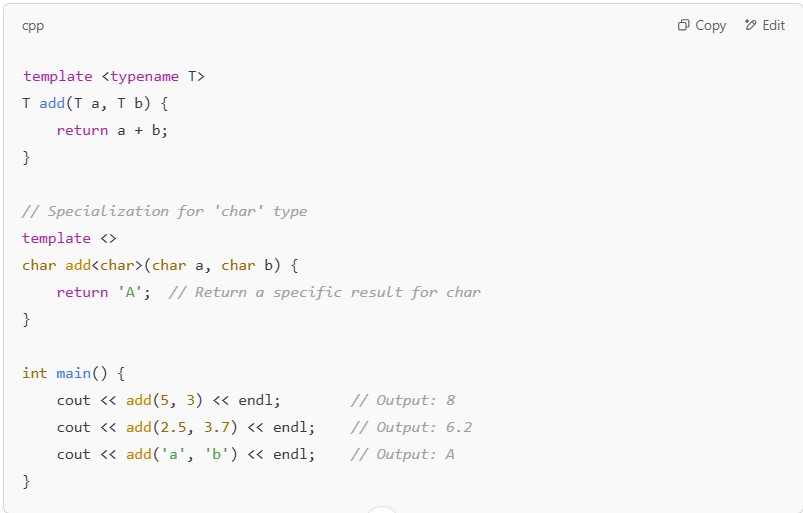
cpptemplate <typename T>
class Box {
private:
T value;
public:
Box(T val) : value(val) {}
T getValue() { return value; }
};
int main() {
Box<int> box1(10);
cout << box1.getValue() << endl; // Output: 10
Box<double> box2(5.5);
cout << box2.getValue() << endl; // Output: 5.5
}
In this code, the Box
class is a C++ template that can be instantiated with any data type, like int
, double
, or user-defined types.
Class Template Specialization
Just like function templates, you can specialize class templates for specific types. Here’s an example:
cpptemplate <typename T>
class Printer {
public:
void print(T value) {
cout << value << endl;
}
};
// Specialization for 'char'
template <>
class Printer<char> {
public:
void print(char value) {
cout << "Character: " << value << endl;
}
};
int main() {
Printer<int> intPrinter;
intPrinter.print(10); // Output: 10
Printer<char> charPrinter;
charPrinter.print('A'); // Output: Character: A
}
In this case, the Printer
class is specialized for char
, providing a custom print format.
Advanced Topics in C++ Templates
Template Template Parameters
Template template parameters allow you to pass a template as an argument to another template. This is useful when you need to work with containers or other types that are also templates. Here’s an example:
cpptemplate <typename T>
class Container {
private:
T value;
public:
Container(T val) : value(val) {}
T getValue() { return value; }
};
template <template <typename> class ContainerType, typename T>
class Wrapper {
private:
ContainerType<T> container;
public:
Wrapper(T val) : container(val) {}
T getValue() { return container.getValue(); }
};
int main() {
Wrapper<Container, int> w(10);
cout << w.getValue() << endl; // Output: 10
}
Here, Wrapper
accepts a template template parameter, allowing you to pass the Container
class template to it.
Default Template Parameters
C++ templates allow you to specify default values for template parameters. This feature can be useful when you want to provide a default type if no type is provided during instantiation:
cpptemplate <typename T = int>
class Box {
private:
T value;
public:
Box(T val) : value(val) {}
T getValue() { return value; }
};
int main() {
Box<> box1(5); // Uses default type 'int'
cout << box1.getValue() << endl; // Output: 5
}
In this example, the Box
class has a default template parameter of int
, so you don’t have to specify it explicitly when creating an instance.
The Standard Template Library (STL)
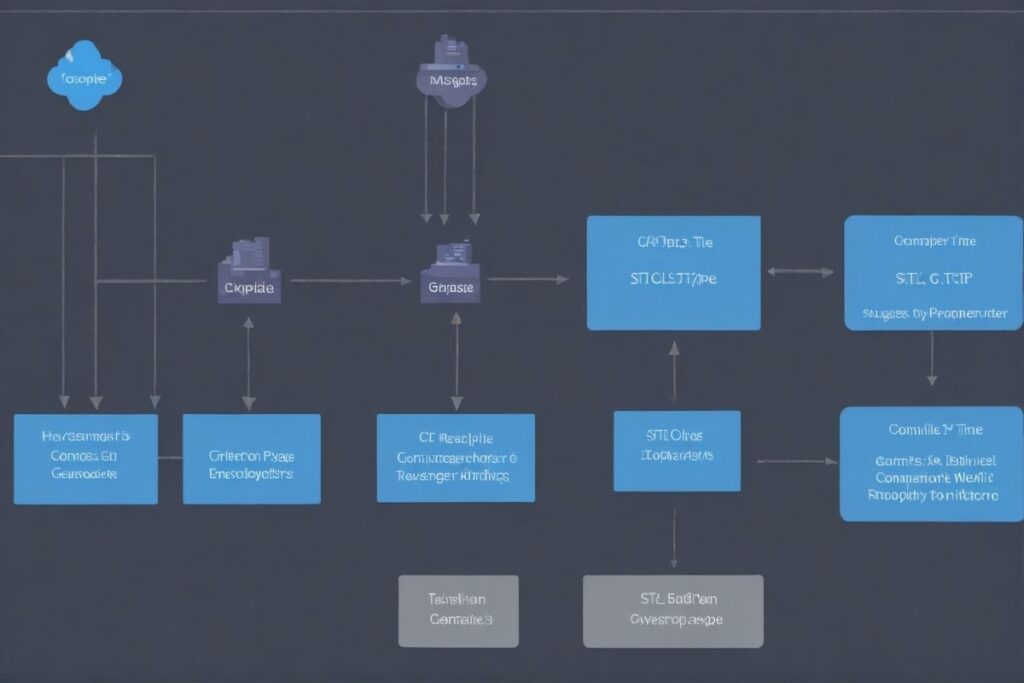
The Standard Template Library (STL) is a powerful collection of template classes and functions provided by the C++ Standard Library.
It includes several commonly used data structures and algorithms that are implemented using templates, making them generic and type-safe. Some of the key components of STL are:
- Containers: These are template classes like
vector
,list
,deque
,map
, andset
that store and manage collections of data. - Iterators: These are used to access the elements of containers.
- Algorithms: STL provides a range of algorithms like
sort()
,find()
, andreverse()
that work with any container.
The STL provides a wide range of functionality and is one of the key reasons why C++ is so popular in the development of high-performance applications.
Conclusion
C++ templates are an essential feature of the language that provides developers with the ability to write generic and reusable code.
By allowing functions and classes to operate on any data type, C++ templates promote code reusability, reduce redundancy, and improve maintainability.
With templates, you can create flexible and efficient programs without sacrificing performance.
In this article, we have covered the fundamentals of C++ templates, including function templates, class templates,
FAQs
What is a C++ Template?
A C++ template allows you to create generic functions and classes that can work with any data type, making the code reusable and type-safe.
How Do C++ Templates Work?
C++ templates generate code for a specific data type during compile-time, ensuring that the function or class works for any data type without extra runtime overhead.
What are Function Templates in C++?
A function template is a generic function that works with any data type. You define it with the template
keyword followed by a placeholder type (e.g., T
), and the compiler creates a specialized version of the function based on the data type used.
What are Class Templates in C++?
A class template allows you to define a class that can handle any data type. You can create instances of a class template for different data types, enabling code reuse and flexibility.
What is Template Specialization in C++?
Template specialization allows you to provide a custom implementation for a specific data type, different from the general template behavior. This is useful when the general template needs to behave differently for certain types.
What is the Standard Template Library (STL) in C++?
The STL is a collection of template-based classes and functions in C++ that provide useful data structures (e.g., vector
, map
, set
) and algorithms (e.g., sort
, find
, reverse
) to simplify coding.
How Do I Declare a Template Class in C++?
To declare a template class in C++, use the template
keyword followed by the type placeholder. For example:
cppCopyEdittemplate <typename T>
class Box {
T value;
public:
Box(T val) : value(val) {}
T getValue() { return value; }
};
What is Template Instantiation?
Template instantiation occurs when you use a template with a specific data type, and the compiler generates the appropriate function or class for that type at compile-time.
What are Default Template Parameters in C++?
Default template parameters allow you to specify default types for templates. If no type is provided during instantiation, the default type is used.
cppCopyEdittemplate <typename T = int>
class Box { /* ... */ };
Can C++ Templates Be Used with Multiple Types?
Yes, C++ templates can be used with multiple types. A template can be instantiated with different data types, making it highly flexible and reusable across various scenarios.