Python developers often encounter the error “local variable referenced before assignment” while working with variables in functions, loops, conditionals, and nested scopes.
This common issue can be confusing for beginners, but it’s entirely solvable with a clear understanding of Python’s scoping rules and how variables are managed.
Let’s dive into what causes this error, explore solutions with step-by-step examples, and share best practices to prevent it effectively in your Python projects.
What Is the “Local Variable Referenced Before Assignment” Error?
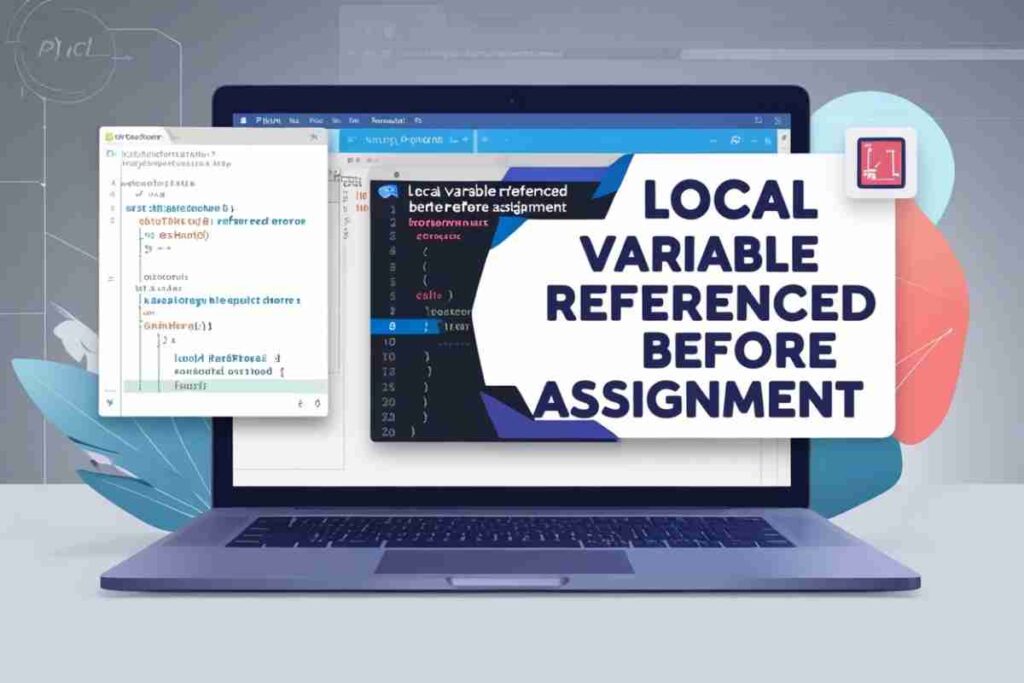
The error “local variable referenced before assignment” occurs when a variable is used inside a function before it is assigned any value.
In Python, variables inside a function are treated as local unless explicitly declared otherwise, leading to this error when an attempt is made to access them before they are defined.
For example:

Here, Python interprets count
as a local variable but cannot find any prior assignment to it in the function scope, triggering the error.
Causes of the Error
No Assignment Before Use
The most common cause is attempting to use a variable without assigning it a value within the function.
Example:

Overwriting Global Variables
When you attempt to modify a global variable inside a function without declaring it as global
, Python treats it as a local variable, causing the error.
Example:
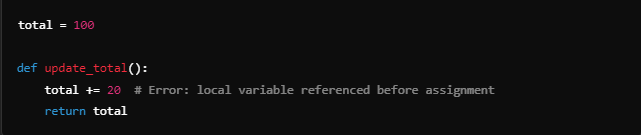
Using Variables in Conditional Statements
If a variable is conditionally assigned within an if
statement and referenced before the condition is met, the error arises.
Example:

How to Fix the “Local Variable Referenced Before Assignment” Error
Assign a Value Before Using
Ensure that the variable is assigned a value before it is used in the function.
Corrected Example:

Use the global
Keyword
If the variable is declared outside the function, explicitly declare it as global
inside the function to modify it.
Corrected Example:
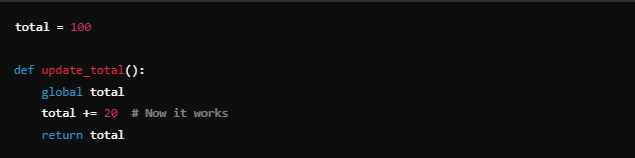
Handle Conditional Assignments Properly
Ensure variables in conditional statements are assigned a default value before the condition is evaluated.
Corrected Example:
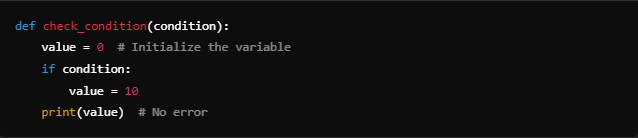
Use the nonlocal
Keyword
When working with nested functions, use nonlocal
to modify variables from an enclosing scope.
Example:
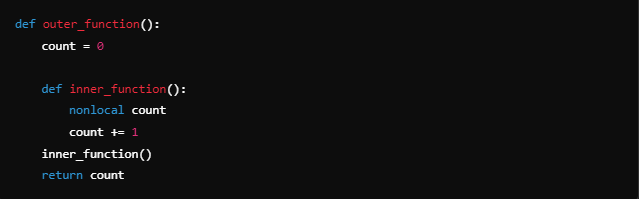
Understanding Python’s Scoping Rules
To effectively address the “local variable referenced before assignment” error, it’s crucial to understand Python’s scoping rules.
In Python, variables can either be local, global, or nonlocal, depending on where they are defined and used in the program. These scopes determine where a variable is accessible and whether or not it can be modified within a function.
Local Scope
A variable that is assigned within a function is considered local to that function. It can only be used inside that function unless explicitly passed around.
If you try to use this local variable before assigning it a value, you will encounter the “local variable referenced before assignment” error.
Global Scope
Global variables are defined outside of functions and are accessible throughout the entire program. However, if you try to modify a global variable inside a function without using the global
keyword, Python will treat it as a local variable, which can lead to the error.
Nonlocal Scope
In nested functions, you can use the nonlocal
keyword to refer to a variable in the nearest enclosing scope (excluding the global scope).
If this keyword is omitted, Python will treat the variable as local to the innermost function, causing the error if it’s used before assignment.
When Does Python Treat a Variable as Local?
Assigning a Value Inside a Function
When you assign a value to a variable inside a function, Python automatically treats it as a local variable for that function. For example:

However, if you reference a variable before it’s assigned, Python will throw the “local variable referenced before assignment” error. To fix this, you must initialize the variable before use.
Troubleshooting Common Scenarios
Modifying Global Variables Inside Functions
If you want to modify a global variable inside a function, you need to declare it as global
to avoid the error. Without this declaration, Python will treat the global variable as local and expect it to be assigned a value within the function.
Example of Error:

Fixed Code:
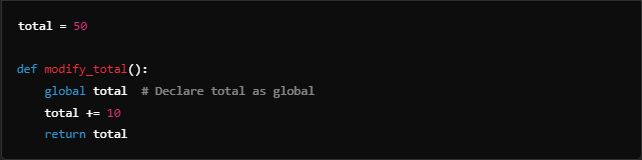
This makes the total
variable refer to the global one, allowing you to modify its value within the function.
Unassigned Variables in Conditional Blocks
Another common cause of this error is unassigned variables in conditional blocks. If a variable is only assigned in one branch of a conditional statement (e.g., an if
block), the variable may not be assigned in all situations. This can result in the error if you attempt to use it later.
Example of Error:

Fixed Code:
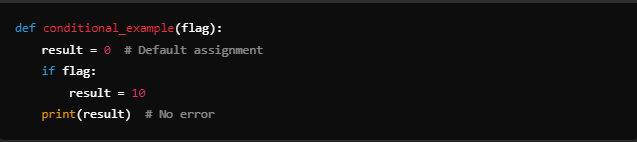
By assigning a default value to result
before the conditional block, you avoid the “local variable referenced before assignment” error, ensuring that the variable is always defined.
Best Practices to Avoid This Error
- Always Initialize Variables: Assign a value to variables before using them. This is especially important in loops and conditionals.
- Understand Python Scope Rules: Familiarize yourself with local, global, and nonlocal scopes to prevent accidental misuse.
- Avoid Overwriting Built-ins: Don’t use names of Python built-in functions or keywords as variable names.
- Use Descriptive Variable Names: Clear and descriptive names reduce the chances of accidental reassignments or misunderstandings.
Common Scenarios and Solutions
Local Variable Referenced Before Assignment in Python Loops
Loops often lead to this error when a variable is used but not initialized outside the loop.
Example:

Solution:
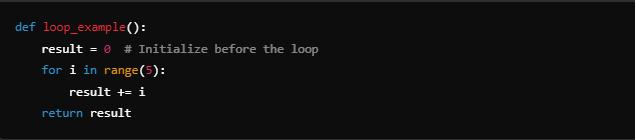
Local Variable Referenced Before Assignment in If Statements
Conditional statements can cause this error when a variable is used outside the condition without proper initialization.
Example:

Solution:
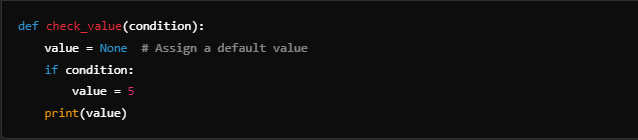
Unbound Local Variable Referenced Before Assignment
This variation of the error occurs when the nonlocal
or global
keywords are improperly omitted in nested or global scope.
Example:
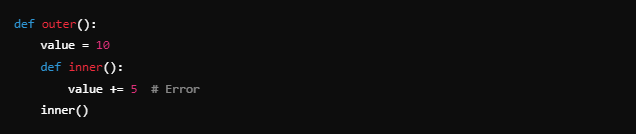
Solution:
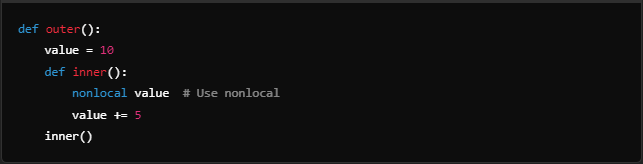
Learning from Official Python Documentation
For an in-depth understanding of Python’s scope rules, refer to the official Python documentation. It provides detailed insights into how Python manages local and global scopes, as well as variable assignments.
Related Errors and Troubleshooting
Errors similar to “local variable referenced before assignment” include:
- NameError: Raised when a variable is not defined at all.
- AttributeError: Occurs when an invalid attribute is accessed on an object.
- TypeError: Triggered when an operation is performed on an inappropriate type.
By understanding how these errors relate to variable handling in Python, you can debug code more effectively.
Conclusion
The “local variable referenced before assignment” error in Python is common but manageable with a solid understanding of variable scopes and initialization.
By initializing variables, using global
or nonlocal
appropriately, and adopting best practices, you can avoid this error and write cleaner, error-free Python code.
FAQs
What does “local variable referenced before assignment” mean in Python?
It means you tried to use a variable in a function before giving it a value.
How do I fix the “local variable referenced before assignment” error?
Ensure the variable is assigned a value before it’s used in your function or code block.
Can this error happen with global variables?
Yes, if you try to modify a global variable inside a function without using the global
keyword.
What is the role of the global
keyword?
The global
keyword allows you to modify a global variable inside a function, avoiding the error.
What is the difference between local
and global
variables?
Local variables are defined within a function, while global variables are defined outside and can be accessed anywhere.
How do conditionals cause this error?
If a variable is only assigned inside a conditional block and used outside, it may not be defined for all paths.
What does “unbound local variable” mean?
It means you referenced a variable that’s local to a function but hasn’t been assigned a value yet.
Can nested functions cause this error?
Yes, if you try to modify a variable from an outer scope without using nonlocal
, this error may occur.