When it comes to text manipulation in JavaScript, one of the most common tasks developers face is capitalizing the first letter of a word. Whether you’re working on user input, formatting titles, or preparing data for display, learning how to convert the first letter of a word to uppercase is an essential skill.
In this comprehensive guide, we’ll dive into multiple methods for achieving the desired result in JavaScript, along with tips and best practices for improving performance and readability.
By the end of this article, you’ll be equipped with various approaches to JavaScript uppercase first letter and ready to handle even more complex text formatting tasks
Why JavaScript Uppercase First Letter is Important
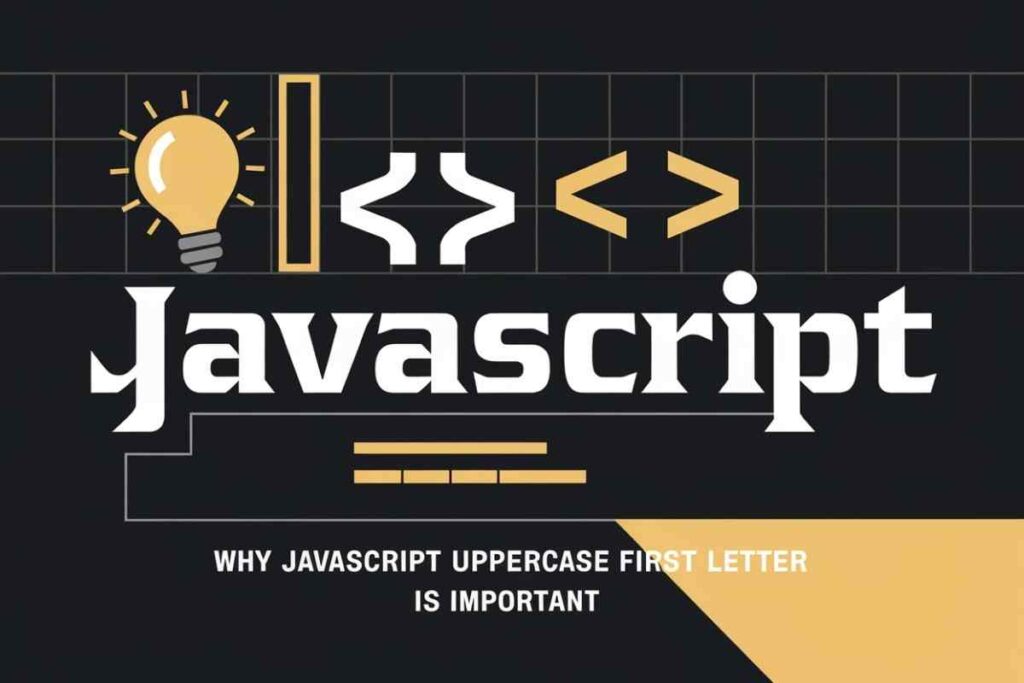
Capitalizing the first letter of a string is a widely used technique in both web development and programming. This is especially useful for:
- Formatting User Input: Ensuring that names, titles, or any other text input begins with a capital letter.
- Displaying Content Neatly: Creating well-structured, readable content in user interfaces.
- Data Formatting: Correcting strings automatically in case of unformatted or inconsistent data entries.
Learning how to manipulate strings efficiently in JavaScript is crucial for developers who aim to create polished and user-friendly applications.
Basic Methods to Uppercase the First Letter in JavaScript
There are a few popular ways to capitalize the first-letter of a word in JavaScript, each suited for different situations. Below, we’ll explore the most common methods, including examples.
Using charAt()
and toUpperCase()
One of the simplest and most straightforward ways to capitalize the first-letter of a string is by combining the charAt()
method with the toUpperCase()
method. Here’s how it works:
- Retrieve the first character of the string using the
charAt()
method. - Convert the first character to uppercase using the
toUpperCase()
method. - Concatenate the uppercase character with the rest of the string.
Example:
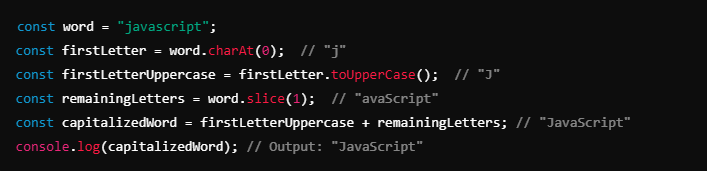
This method gives you full control over the string manipulation, making it easy to capitalize the first letter and leave the rest of the string intact.
Using slice()
and toUpperCase()
Another common approach for capitalizing the first letter is using the slice()
method. This method allows you to extract a part of the string (from the second character onward) while leaving the first character intact.
Example:

This method is slightly shorter and combines the capitalized first letter with the remaining part of the string in one line.
Edge Cases and Additional Considerations
When working with strings, it’s important to consider various edge cases to ensure that your code behaves as expected under all conditions.
Handling Empty Strings
If the string is empty, neither of the methods will work as expected. Always check if the string is not empty before applying string manipulation

Handling Non-Alpha Characters
If the string begins with a non-alphabetic character, you may want to skip the capitalization or handle it differently. For example, handling strings that start with numbers or punctuation marks:

In such cases, consider sanitizing or validating input before proceeding with text manipulation.
Improving Performance and Readability
While the methods described above are suitable for most use cases, it’s always a good idea to consider performance, especially when dealing with large datasets or frequent string manipulations.
Use a Function for Reusability
If you need to perform this task multiple times in your code, consider wrapping it in a reusable function:
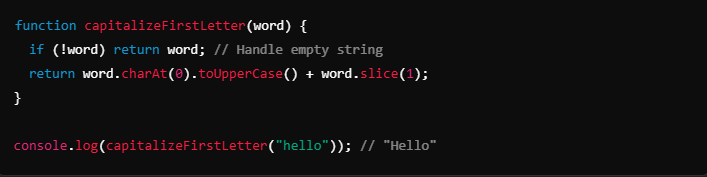
Avoiding Repetition
To improve readability, avoid repeating the same logic throughout your code. Instead, call a function or use a more declarative approach whenever possible.
Alternatives for Capitalizing the First Letter
While charAt()
, slice()
, and toUpperCase()
are the most commonly used methods, you might also consider other alternatives for specific use cases.
Using Regular Expressions
You can use a regular expression (regex) to match the first letter of a string and replace it with the uppercase version:

This method is particularly useful if you’re working with more complex strings or need to handle multiple occurrences of uppercase transformations.
Using String Interpolation (Template Literals)
In some situations, you may prefer using template literals for better readability and concise code:

Template literals offer a cleaner and more readable way to manipulate strings.
Conclusion
Capitalizing the first letter of a string in JavaScript is a common and essential task for developers. Whether you use charAt()
and toUpperCase()
, slice()
and toUpperCase()
, or regular expressions, JavaScript offers several ways to achieve this functionality.
We covered not only basic methods but also edge cases, performance optimization tips, and alternative approaches, ensuring you can handle any situation.
By implementing these strategies effectively, you can format text, improve data presentation, and create a more user-friendly experience in your applications. With these techniques at your disposal, you’re now ready to tackle text manipulation with confidence!
FAQS
How do I capitalize the first letter of a string in JavaScript?
You can use charAt(0).toUpperCase()
to get the first letter in uppercase and concatenate it with the rest of the string using slice()
or substring()
.
What is the best method to uppercase the first letter in JavaScript?
The most common and efficient way is using charAt(0).toUpperCase()
combined with slice(1)
to capitalize the first letter while keeping the rest of the string unchanged.
Can I use JavaScript to capitalize the first letter of each word in a sentence?
Yes, you can loop through each word in a sentence, capitalize the first letter using the same method, and join the words back together.
How do I handle empty strings when capitalizing the first letter?
You should check if the string is empty before applying the transformation. If it’s empty, return the string as is without trying to manipulate it.
Does JavaScript’s toUpperCase()
affect the rest of the string?
No, toUpperCase()
only affects the specific character it is applied to. For example, word.charAt(0).toUpperCase()
only capitalizes the first letter, not the entire string.
Can I use regular expressions to capitalize the first letter in JavaScript?
Yes, you can use replace()
with a regular expression to target and capitalize the first letter of a string, like word.replace(/^./, word[0].toUpperCase())
.
Is there a one-liner solution to capitalize the first letter in JavaScript?
Yes, you can use this concise solution: const capitalized = word.charAt(0).toUpperCase() + word.slice(1);
How do I capitalize the first letter of a string without affecting other characters?
You can safely use word.charAt(0).toUpperCase() + word.slice(1)
to only capitalize the first letter and keep the rest of the string as it is.