JavaScript’s replace()
method is one of the most commonly used functions when manipulating strings. Whether you’re working with a basic text replacement or need advanced functionality with regular expressions, the replace()
method offers flexibility and power.
In this article, we’ll dive deep into how the JavaScript replace()
method works, its syntax, use cases, and best practices, including examples to help you master this essential feature.
What is JavaScript Replace()?
The replace()
method in JavaScript is a built-in function of the String
prototype that is used to replace parts of a string based on a given pattern (either a string or a regular expression).
It returns a new string with the specified replacements. The original string remains unchanged as strings in JavaScript are immutable.
Key Features:
- Pattern Matching: The method can accept both strings and regular expressions as the pattern.
- One-time Replacement: By default, it replaces only the first occurrence of the pattern unless a global flag (
g
) is used in a regular expression. - String or Function Replacement: You can provide either a string or a function as the replacement value.
Syntax of JavaScript replace()
The basic syntax of the replace()
method is as follows:
pattern
: The substring or regular expression that you want to match.replacement
: The string or function that will replace the matched pattern.
Parameters Explained:
pattern
:- This can be either a string or a
RegExp
(regular expression). If a string is used, only the first occurrence is replaced. - If a regular expression is used, more advanced search and replace capabilities are available, such as global (
g
) and case-insensitive (i
) flags.
- This can be either a string or a
replacement
:- A string that will replace the matched pattern.
- You can also pass a function which will be called for each match.
Return Value:
- A new string with the replaced values. The original string remains unchanged.
How JavaScript replace()
Works with Strings
If the pattern
is a string, the method will replace only the first occurrence of the string with the specified replacement.
Example:

Limitations with String Patterns:
- When using a string as a pattern, only the first match is replaced.
- If you want to replace all occurrences of a string, you will need to use a
RegExp
with the global (g
) flag.
How to Replace Multiple Occurrences in JavaScript
If you need to replace all occurrences of a pattern in the string, you must use a regular expression with the g
(global) flag.
Example with Regular Expression:

Special Replacement Patterns in JavaScript replace()
When using a string as the replacement, you can include special replacement patterns. These patterns allow you to use parts of the matched string in the replacement.
Replacement Patterns:
Pattern | Description |
---|---|
$& | Inserts the matched substring. |
$1 , $2 , …, $n | Inserts the nth capturing group. |
$` | Inserts the part of the string before the match. |
$' | Inserts the part of the string after the match. |
Example:
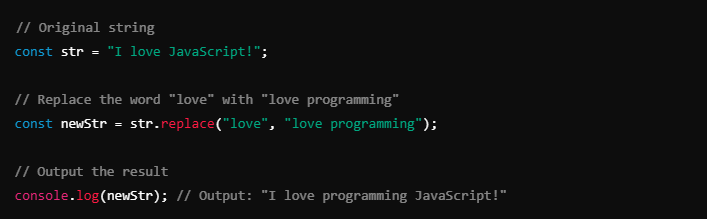
Using Functions as Replacement in JavaScript replace()
In some cases, you might want the replacement string to be dynamically generated based on the match. This is where using a function as the second argument comes in handy.
Function Signature:

match
: The matched substring.p1
,p2
, …: The values from capture groups.offset
: The position of the match in the string.string
: The entire original string.
Example:
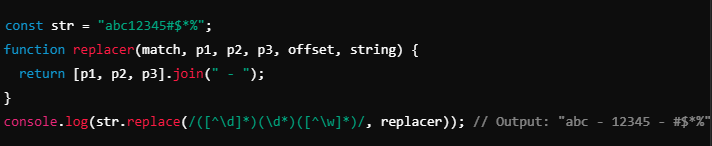
How to Use JavaScript replace()
with Regular Expressions
Case-Insensitive Replacement:
To make your search case-insensitive, use the i
flag with the regular expression.

Replacing Specific Parts Using Capture Groups:
Using capturing groups in regular expressions allows you to target specific parts of the matched string.

Best Practices for Using JavaScript replace()
- Use Regular Expressions for Global Replacement: If you want to replace all instances of a pattern, don’t forget to use the
g
flag. - Handle Edge Cases: If the pattern can potentially match an empty string, handle it properly to avoid unexpected behavior.
For exampleconsole.log("".replace("", "_")); // "_"
- Consider Performance: Using regular expressions with complex patterns or using a function to replace each match can impact performance. Avoid unnecessary complexity in performance-critical code.
JavaScript replace()
vs replaceAll()
In recent versions of JavaScript, the replaceAll()
method was introduced as a simpler alternative for replacing all occurrences of a pattern without needing to use the global flag.

Differences:
replace()
: Replaces only the first occurrence unless used with a regular expression with theg
flag.replaceAll()
: Replaces all occurrences without needing to use regular expressions.
Conclusion
The JavaScript replace()
method is a versatile and powerful tool for string manipulation. By understanding how to use it with both simple strings and regular expressions, you can efficiently perform complex replacements.
Whether you need to replace a single occurrence or multiple matches, JavaScript’s replace()
method provides the flexibility to meet your needs.
For deeper usage, always remember to consider regular expressions for more complex patterns and replace dynamic content with functions for advanced replacements.
FAQS
How does the replace()
method work in JavaScript?
It returns a new string with some matches of a pattern replaced by a replacement. The pattern can be a string or regex, and the replacement can be a string or function.
Can replace()
replace all occurrences?
No, it replaces only the first match. Use the g
flag in a regex or replaceAll()
to replace all occurrences.
What is $&
in replace()
?
$&
inserts the entire matched substring in the replacement string.
Can I use a function as the replacement?
Yes, you can pass a function that receives match details and returns the replacement string.
What’s the difference between replace()
and replaceAll()
?
replace()
replaces only the first match, while replaceAll()
replaces all matches in a string.
What if the pattern is an empty string?
The replacement is prepended to the start of the string.
How do capturing groups work in replace()
?
Capturing groups are used with $1
, $2
, etc., in the replacement string to refer to matched parts.
Can replace()
handle special characters?
Yes, but special characters like $
, \
, need to be escaped.