Master Python += is one of the most versatile and commonly used compound assignment operators in Python. It combines the addition operation and assignment into one step, enabling cleaner and more efficient code.
This article explores Python + = in detail, provides practical examples, and compares it with other operators to offer a comprehensive understanding of its utility.
What Is Python +=?
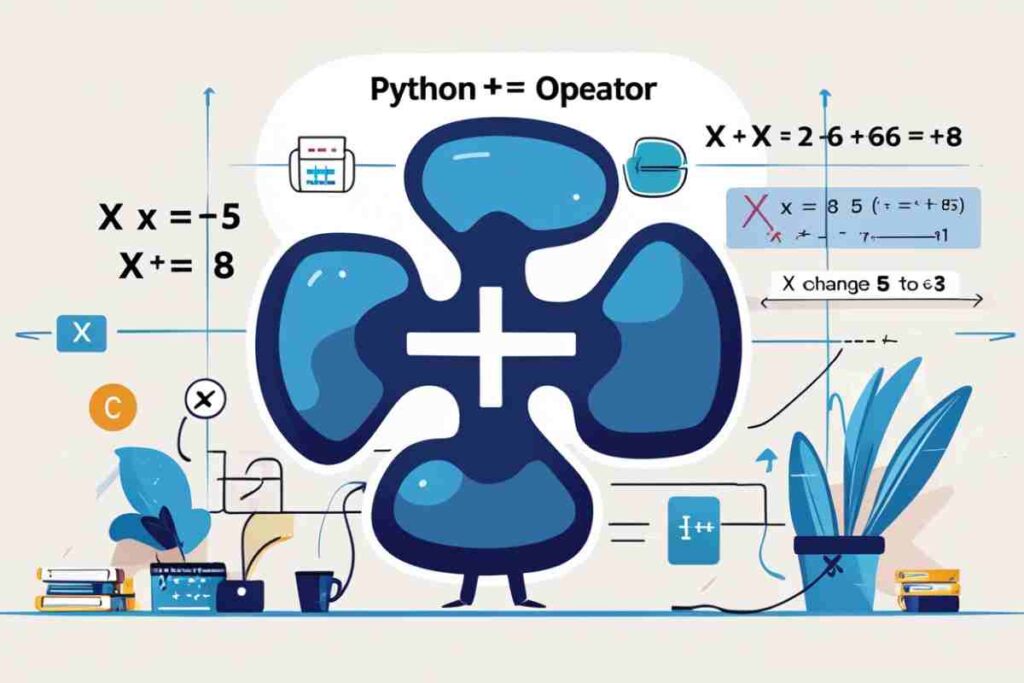
Python + = is a shorthand operator that combines addition and assignment into a single, concise statement. For example, instead of writing:

you can simply use:

The += operator updates the value of a variable by adding the right-hand operand to its current value. This operator supports multiple data types, such as numbers, strings, and lists, provided they have addition semantics.
How Python += Works
When you use the += operator, Python evaluates the right-hand operand, adds it to the left-hand operand, and assigns the result back to the variable. Here is a simple breakdown of the process:
- Evaluate the Operand: The value on the right-hand side of the operator is computed.
- Perform Addition: This value is added to the current value of the variable.
- Reassign the Variable: The result replaces the original value of the variable.
Syntax

variable
: The variable to update.value
: The value to add to the variable.
Python += Examples Across Data Types
Adding Numbers
Using += with integers or floats is straightforward:

Concatenating Strings
Strings can be combined efficiently with +=:

Extending Lists
With lists, += adds elements from one list to another:

Combining += with Mutable and Immutable Types
When working with immutable types like numbers or strings, += creates a new object. However, for mutable types like lists, it modifies the original object.
For additional examples, explore Python + = Stack Overflow discussions.
Why Use Python +=? Key Advantages
Conciseness
By reducing redundancy, += saves time and effort. Writing x += 5
instead of x = x + 5
is not only faster but also less error-prone.
Enhanced Readability
The += operator clearly communicates intent, making your code easier to understand and maintain.
Optimized Performance
Although the performance improvement is marginal in many cases, eliminating redundant variable lookups can make a difference in larger programs.
Comparing Python += with Other Operators
Python += vs. Python = +
The += operator modifies the existing object (if mutable), while =
creates a new object.
Python += vs. Multiplicative Operators (*=)
Similar to +=, operators like *= or -= combine their respective arithmetic operation with assignment:

Python + = with Immutable vs. Mutable Types
- Immutable Types: Using += with integers or strings creates a new object. For example:

- Mutable Types: For lists, += extends the same object:

For deeper insights, refer to Real Python’s Operator Guide.
Avoiding Common Mistakes with Python + =
TypeError: Unsupported Operand Types
The += operator requires compatible data types. Attempting to add incompatible types raises an error:

Solution: Convert operands to compatible types:

Misunderstanding Behavior with Mutable Types
When working with mutable types like lists, be cautious. Using += modifies the original object rather than creating a copy:

Advanced Use Cases of Python +=
Using Python + = in List Comprehensions
You can use += in conjunction with list comprehensions to build or modify lists more concisely:

Combining += with Defaultdict
The += operator works seamlessly with collections like defaultdict
to simplify accumulation tasks:
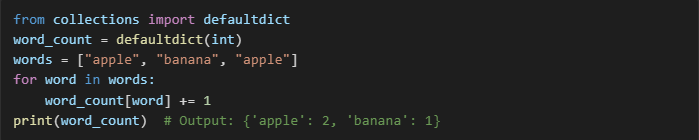
Extending Numpy Arrays
In numerical computing, += is often used to update arrays element-wise:

Combining += with Pandas DataFrames
In data analysis, you can use += to update columns in Pandas DataFrames:
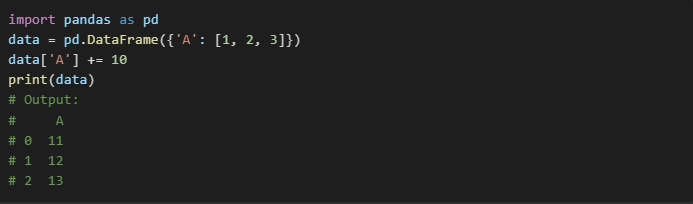
Avoiding Common Mistakes with Python + =
TypeError: Unsupported Operand Types
The += operator requires compatible data types. Attempting to add incompatible types raises an error:

Solution: Convert operands to compatible types:

Misunderstanding Behavior with Mutable Types
When working with mutable types like lists, be cautious. Using += modifies the original object rather than creating a copy:

Conclusion
Python += is a versatile and powerful tool for streamlining your code. Whether you’re working with numbers, strings, or lists, mastering this operator enhances your ability to write clean and efficient Python programs.
By understanding its behavior across different data types and avoiding common pitfalls, you can leverage += to its fullest potential for writing efficient and effective Python programs. in creating concise, efficient, and highly readable Python code.
FAQs
What does the +=
operator do in Python?
The +=
operator adds a value to a variable and updates it in place, simplifying assignments.
Can +=
be used with strings?
Yes, +=
concatenates strings efficiently without needing explicit reassignment.
How does +=
work with lists?
For lists, +=
extends them by appending elements from another iterable.
Does +=
create a new object in memory?
It depends on the data type—immutable types like integers create new objects, while mutable types like lists modify in place.
Is +=
the same as x = x + y
?
Functionally similar, but +=
can modify objects in place when used with mutable types.
Can +=
be used with tuples?
No, tuples are immutable, so +=
creates a new tuple instead of modifying the original.
Is +=
more efficient than x = x + y
?
For mutable types like lists, +=
is more efficient as it avoids creating a new object.
Can +=
be overridden in custom classes?
Yes, you can define __iadd__
in a class to customize +=
behavior.