Binary to hex conversion is a straightforward process that simplifies the representation of binary numbers into hexadecimal format for easier understanding and usage.
Whether you’re working on programming projects, data encoding, or just learning computer science basics, understanding how to convert binary to hexadecimal is essential.
In this article, we’ll cover the basics of binary to hex conversion provide examples and share tools and tips for smooth calculations.
What is Binary to Hex Conversion?
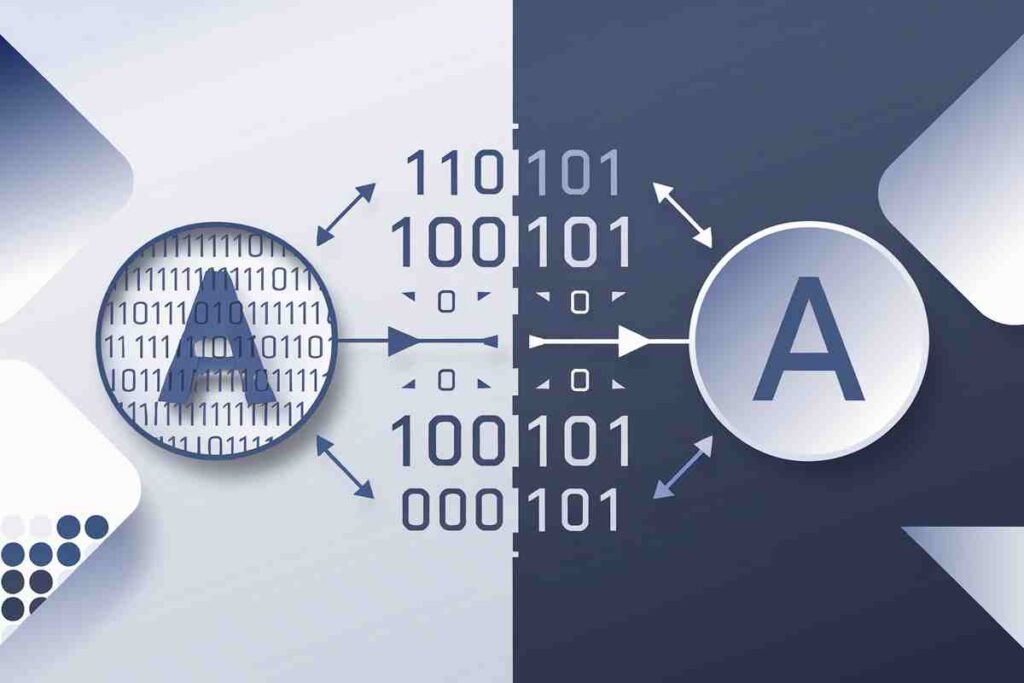
Binary to hex and converting hex to binary are fundamental concepts in computing. Binary is a base 2 number system consisting of only 0s and 1s, while hexadecimal is a base 16 system using numbers 0-9 and letters A-F. Converting binary to hex helps simplify the representation of large binary values.
For instance:
Binary: 1010 1110
Hexadecimal: AE
This process is especially useful in fields like software development and digital electronics, where compact data representation is crucial.
How to Convert Binary to Hex Manually
The conversion process is simple if you follow these steps:
The convert binary to hex process is simple if you follow these steps:
Step 1: Break Binary into Groups of Four
Hexadecimal digits correspond to four binary bits. Split the binary number into 4-bit groups starting from the right. Add leading zeros if necessary.
Example:
Binary: 110101101
Grouped: 0001 1010 1101
Step 2: Convert Each Group to Hexadecimal
Use a binary to hex table to map each 4-bit group to its corresponding hex digit:
Binary | Hex |
---|---|
0000 | 0 |
0001 | 1 |
0010 | 2 |
0011 | 3 |
0100 | 4 |
0101 | 5 |
0110 | 6 |
0111 | 7 |
1000 | 8 |
1001 | 9 |
1010 | A |
1011 | B |
1100 | C |
1101 | D |
1110 | E |
1111 | F |
Example Conversion:
- Group 0001 = 1
- Group 1010 = A
- Group 1101 = D
Result: 1AD
Step 3: Combine Hex Digits
Write the hex digits together to get the final result.
Binary to Hexadecimal Example
Example: Convert Binary 110111101111
- Split into groups: 1101 1110 1111
- Convert using the table:
- 1101 = D
- 1110 = E
- 1111 = F
Hexadecimal = DEF
This method works universally for any binary number.
Binary to Hexadecimal Example
Let’s work through another example to solidify your understanding:
Example: Convert Binary 110111101111
- Split into groups:
1101 1110 1111
- Convert using the table:
1101
=D
1110
=E
1111
=F
- Combine: Hexadecimal =
DEF
This method works universally for any binary number.
Convert Hex to Binary
Just as we convert binary to hex, we often need to convert hex to binary. Each hexadecimal digit maps to a 4-bit binary sequence:
Example: Convert Hexadecimal 2F to Binary:
- 2 = 0010
- F = 1111
Result: 0010 1111
A hex to binary converter can help speed up this process, making it effortless
Using a Binary to Hexadecimal Converter with Solution
Manual conversions are educational but time-consuming. Luckily, online binary to hexadecimal converters simplify the process.
These tools allow you to input binary numbers and instantly receive the hexadecimal equivalent, often with step-by-step solutions to aid learning.
Recommended Tools:
- RapidTables – User-friendly binary to hex converter.
- Calculator Soup – Detailed explanations alongside results.
- OnlineConversion – Supports various number system conversions.
Using these tools ensures accuracy and saves time during complex calculations.
Binary to Hex Python Implementation
Python is an excellent programming language for binary to hexadecimal conversions. Here’s a simple script to automate the process:
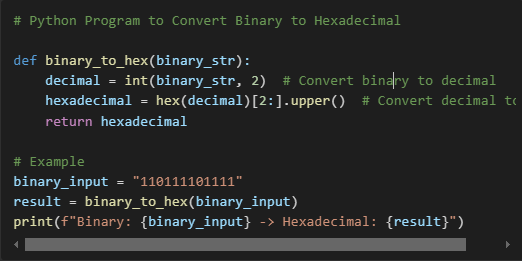
Output
When you run the above script with the input 110111101111
, it will output:

This Python implementation is efficient and easy to integrate into larger projects.
Applications of Binary to Hex Conversion
- Programming: Hexadecimal is commonly used in programming to represent colors (e.g.,
#FF5733
) or memory addresses. - Digital Electronics: Compact hexadecimal representation makes it easier to debug and design circuits.
- Data Encoding: Hexadecimal simplifies the representation of binary-encoded data like images or files.
Advanced Binary to Hexadecimal Conversion Methods
While the manual conversion of binary to hex is relatively simple, there are advanced methods that can be beneficial in certain scenarios.
Let’s explore a few of these methods that could help you handle larger numbers or automate the conversion process in different programming environments.
Method 1: Grouping Bits and Using Masks
In more complex systems, especially in embedded systems or low-level programming, you might encounter situations where you need to convert binary numbers to hexadecimal using a method known as bit masking.
Here, you extract specific bit patterns and convert them into hexadecimal values directly, optimizing performance.
This approach can be useful when working with memory locations or hardware interfaces where speed is crucial.
For instance, let’s assume you need to extract specific 4-bit values from a binary string and convert them to hexadecimal for efficient data processing:
- Example: Binary number:
1101001110110100
- Process: You can use bit-masking techniques to group every 4 bits and directly map them to their hexadecimal equivalents.
This advanced approach can be achieved using languages like C or Assembly and is highly optimized for hardware-level applications.
Method 2: Bitwise Operators in Programming Languages
Bitwise operators in languages like Python, JavaScript, C, and Java allow you to manipulate individual bits directly. These operators can help you perform binary to hex conversions without the need for additional libraries.
Here’s how you can do that using bitwise operators in Python:
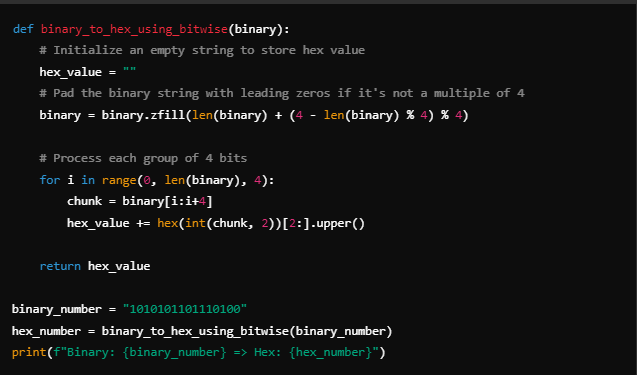
In this example, the binary string is processed using bitwise techniques, and the result is returned as a hexadecimal string. This method offers a lot of flexibility and speed for large numbers.
Practical Examples of Using Binary to Hexadecimal in Real-World Applications
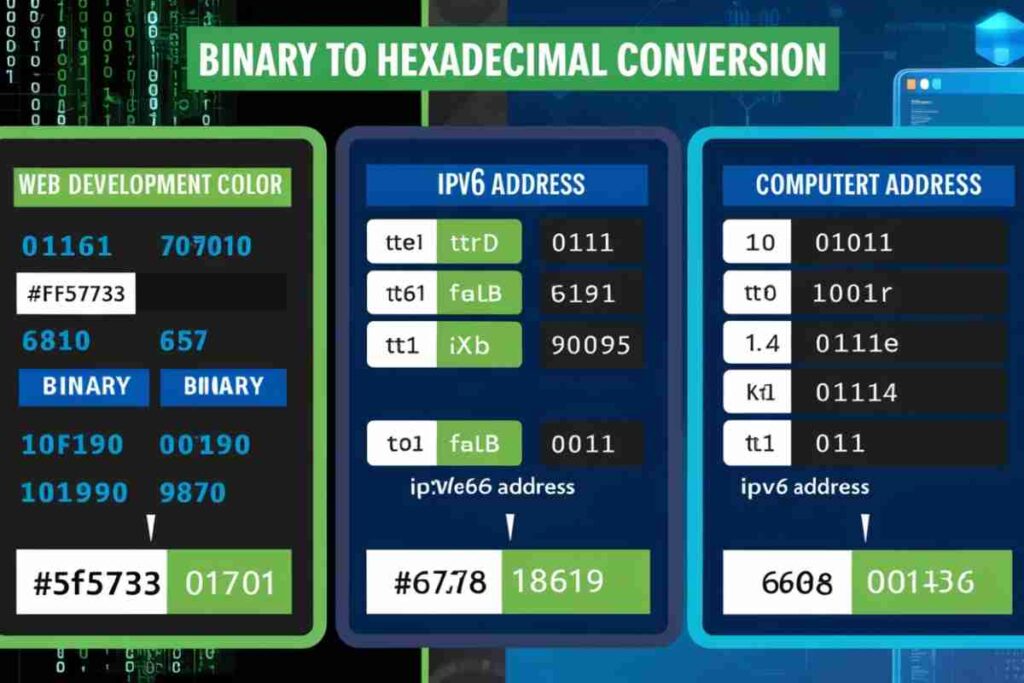
Now that you understand how to convert binary to hex, let’s look at some real-world applications of this conversion.
These applications demonstrate the power of hexadecimal notation in fields such as web development, networking, and debugging.
Web Development: Color Codes in Hexadecimal
In web development, binary to hex conversion plays a crucial role in defining color codes. For example, colors in HTML and CSS are often represented using hexadecimal values, where each color channel (red, green, blue) is expressed in two hexadecimal digits.
For example:
- A color in RGB:
RGB(255, 99, 71)
- In Hex:
#FF6347
The conversion steps are as follows:
- Convert each decimal value (255, 99, 71) into binary.
- Group each 8-bit binary value into two hexadecimal digits.
- The result gives the hex value
#FF6347
, which represents the tomato red color in web development.
Networking: IP Address Representation
In networking, especially with IPv6, hexadecimal values are used to represent addresses because the binary version of an IPv6 address can be excessively long. Hexadecimal allows IPv6 addresses to be displayed more compactly.
IPv6 Example:
- Binary:
0010000000000001000000000000000100000000000000000000000000000001
- Hexadecimal:
2001:0000:0000:0000:0000:0000:0000:0001
This conversion makes it easier to read and manage long binary strings.
Debugging: Memory Address Representation
When working with low-level code or debugging, binary to hex conversion is vital for examining memory addresses.
Computer systems often store memory addresses as binary, but these addresses are represented in hexadecimal to make them easier for programmers to interpret.
For example, in C or C++, a program might use hexadecimal to display memory addresses, which simplifies debugging and memory management.
- Example: A memory address in binary might be
100110010101111100110000111010100
. - In hexadecimal, it could be displayed as
0x9C7F30D
.
This hexadecimal format is much more compact and easier to handle than the binary equivalent.
Tips for Converting Binary to Hex Efficiently
- Use Padding: If your binary string isn’t a multiple of 4, pad it with leading zeros.
- Use Tools: Online converters can handle large binary numbers quickly.
- Memorize the Binary to Hex Table: This speeds up manual conversions.
- Understand Built-in Functions: Languages like Python offer
bin(x)
andhex(x)
functions for easy conversion. - Verify Results: Double-check to ensure accuracy.
Conclusion
Binary to hex conversion is an essential skill in computing, enabling compact and readable data representation. Whether you’re a student, developer, or electronics enthusiast, mastering this process is invaluable.
Use the manual steps, Python scripts, and online tools provided in this article to enhance your efficiency. With practice, converting binary to hexadecimal will become second nature.
FAQs
What is binary to hexadecimal?
It’s the process of converting binary numbers to their equivalent hexadecimal values.
Why is binary converted to hex?
Hex simplifies large binary numbers, making them shorter and easier to read.
How many binary digits equal one hex digit?
Four binary digits correspond to one hexadecimal digit.
Can I use Python for conversions?
Yes, Python’s built-in int()
and hex()
functions make it easy to convert binary to hex.
Are online converters accurate?
Yes, reputable tools like RapidTables or Calculator Soup are highly reliable.
What is a binary to hex table?
A reference table showing 4-bit binary numbers and their hexadecimal equivalents.
Is hex used in modern programming?
Yes, it’s used for memory addresses, colors in design, and debugging.
What’s the largest hex digit?
The largest hexadecimal digit is F
, representing binary 1111
.