Bash scripting is an essential tool for automating tasks and managing system operations. One of its core features is the ability to work with command-line arguments efficiently.
Among these, bah is an invaluable tool for handling arguments passed to a script or function.
In this guide, we’ll explore what Bash $ is, how it compares to $*, when to use it, and practical examples to incorporate it into your scripts.
What Does Bash $@ Mean?
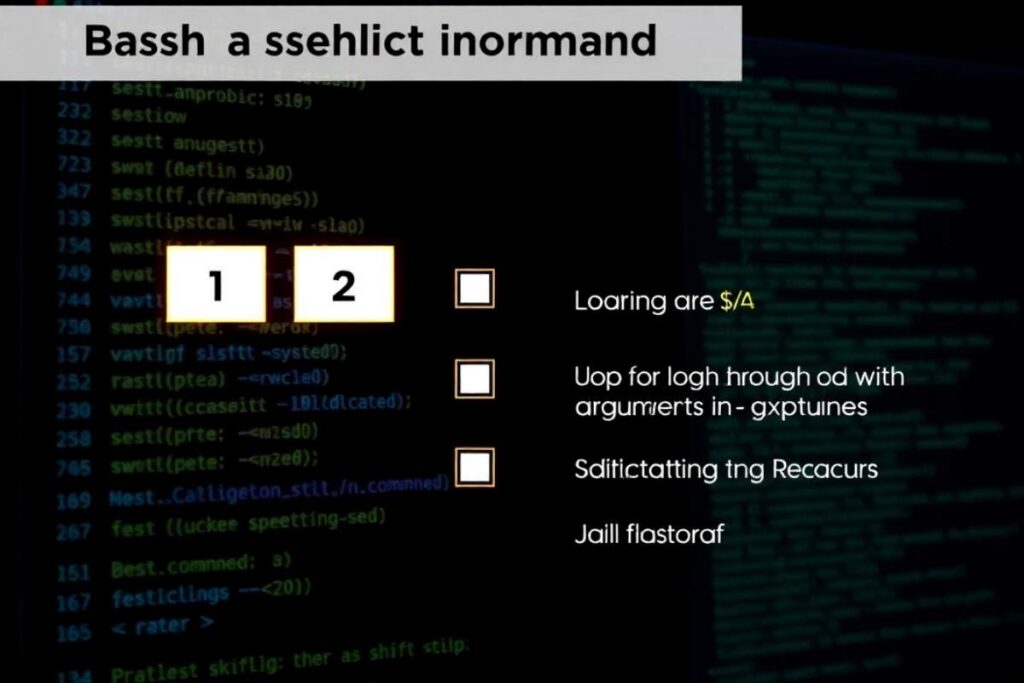
In Bash scripting, $@ is a special variable that represents all the arguments passed to a script or function.
When used, it expands each argument as a separate entity, allowing you to manage multiple arguments individually. This is especially useful for processing command-line inputs in your scripts.
Example:

When you run the script with:

The output will be:

The arguments passed are: arg1 arg2 arg3
Here, $@ expands to all arguments passed to the script (arg1, arg2, arg3). It preserves each argument as a separate entity, making it easy to handle multiple inputs efficiently.
Bash $@ ,$*
Both $@ and $* refer to all arguments passed to a script, but they behave differently, especially when quoted. It’s crucial to understand the distinction between the two for more advanced argument handling.
Bash $@:
- Without quotes: Expands each argument as a separate word.
- With quotes (“$@”): Preserves each argument, even if it contains spaces, by treating them as distinct words.
Bash $*:
- Without quotes: Expands all arguments into a single string, with each argument separated by spaces.
- With quotes (“$*”) combines all arguments into one string, separating them with the first character of the IFS (Internal Field Separator).
Example:
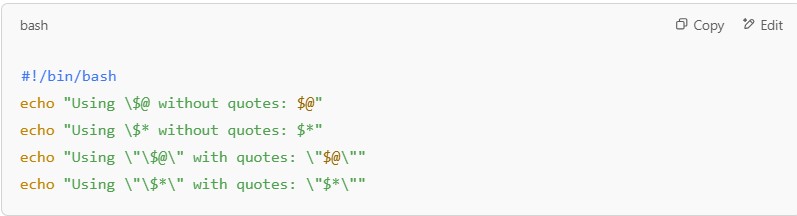
If you execute the script with:

The output will be:
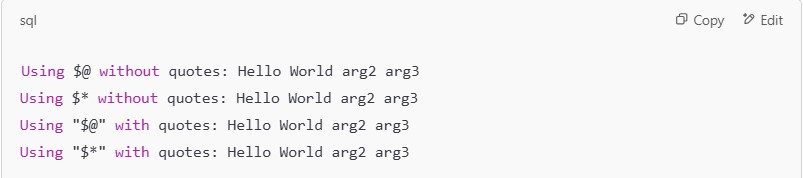
When quoted, $@ and $* preserve arguments with spaces as distinct entities, but they still have differences:

Summary:
- $@ treats each argument as a separate entity, especially useful for handling spaces within arguments.
- $* merges all arguments into one string, which can lead to undesirable results when dealing with multi-word arguments.
For scripts that require precise control over individual arguments (especially those containing spaces), $@ is the preferred choice.
When to Use Bash in Shell Scripts
Knowing when to use Bash @ is essential for writing efficient and maintainable scripts. Below are some common scenarios where $@ shines:
Handling Multiple Arguments
If your script needs to process multiple arguments, $@ allows you to loop through and manipulate each argument separately.
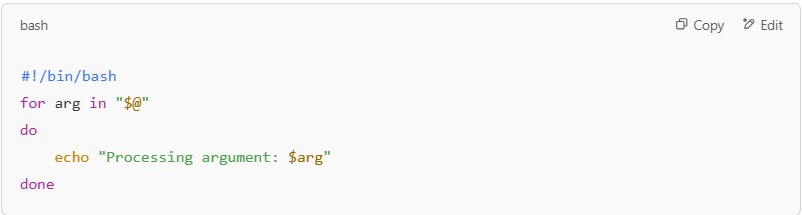
This script will print each argument on a new line.
Passing Arguments to Other Scripts
You can pass all arguments from one script to another using $@. This is especially useful for creating wrapper scripts that forward parameters.

Here, the current script forwards all received arguments to another_script.sh.
Argument Validation
Use $@ to validate if the correct number of arguments have been passed to your script.
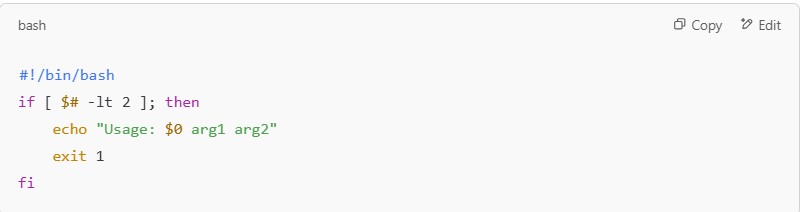
This script checks if two arguments are provided, displaying an error message if not.
Advanced Bash $@ Usage
Now that we’ve covered the basics, let’s dive into some advanced techniques for using $@ in Bash scripting.
Using $@ in Functions
In Bash functions, $@ can be used to pass multiple arguments. This improves the reusability and flexibility of your functions.
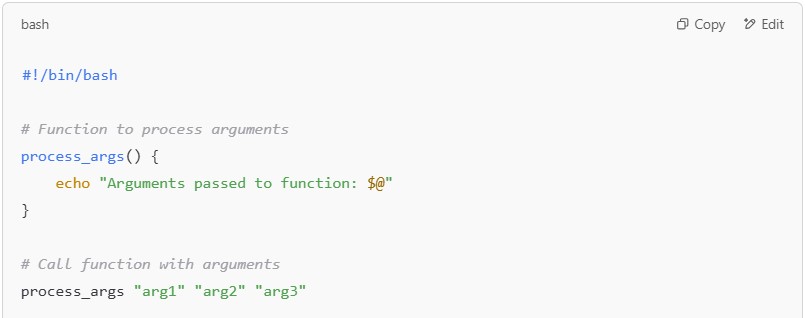
In this example, the function process_args
accepts multiple arguments and processes them using $@.
Combining Bash with Other Commands
You can combine $@ with commands like find, xargs, or grep to build more complex scripts. For example:
Example with find:

This script takes directories as arguments and lists the files within them, leveraging $@ to process each argument individually.
Bash $@ and shift
In some scenarios, you may need to move through arguments dynamically. The shift command in Bash shifts positional parameters to the left, allowing you to process arguments one by one.
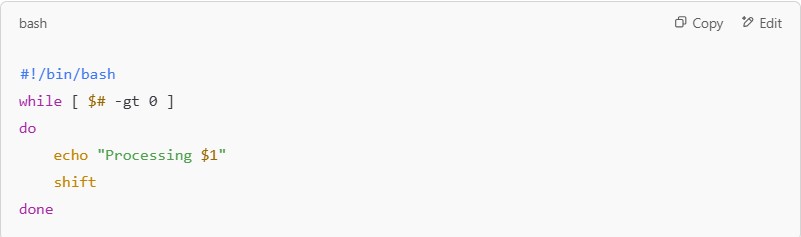
This script processes each argument individually, shifting them left as it goes until there are no more arguments.
Bash and Positional Parameters
Positional parameters like $1, $2, etc., directly refer to specific arguments passed to a script. However, when you need to handle a variable number of arguments, $@ becomes more flexible than using positional parameters directly.
#!/bin/bash
echo "The first argument is: $1"
echo "The second argument is: $2"
In contrast, $@ allows you to handle an undefined number of arguments, making it a more scalable solution.
Best Practices for Using Bash $@
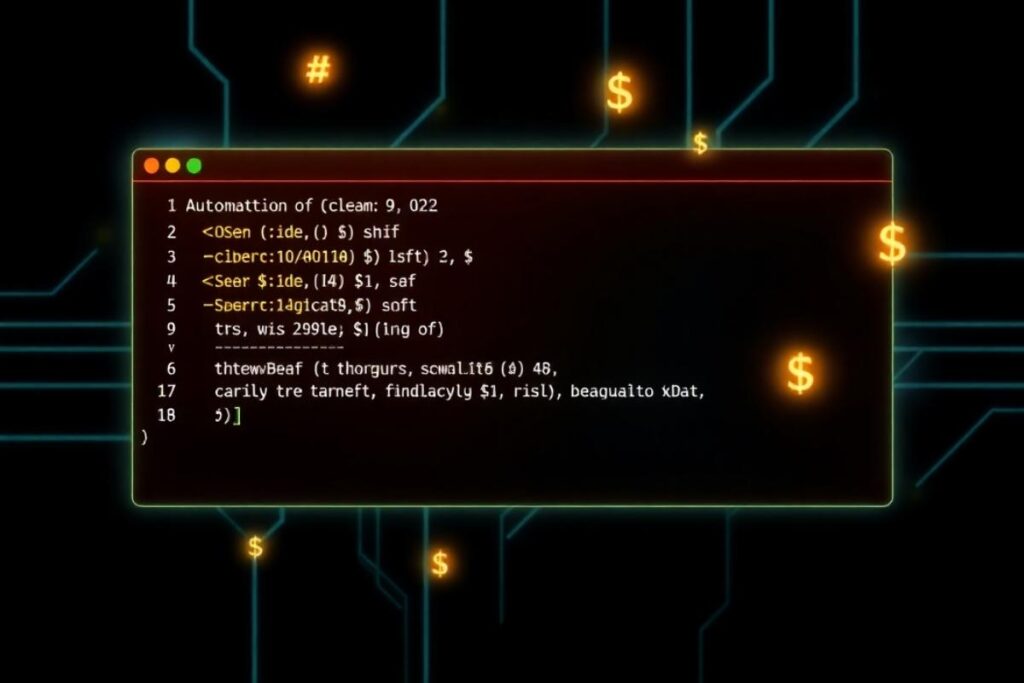
Here are a few best practices when working with Bash $@:
- Always quote $@ to preserve arguments that may contain spaces.
- Use loops to iterate over arguments for fine control.
- Validate arguments before processing them to ensure your script handles inputs correctly.
Conclusion
Mastering Bash $@ is key to writing dynamic, flexible, and efficient shell scripts. Whether you’re looping through arguments, passing them between scripts, or validating inputs, $@ is an indispensable tool in any scripter’s arsenal.
Understanding the differences between Bash $@ and $*, and knowing when to use each, will allow you to tackle more complex scripting scenarios. By combining $@ with functions, command-line tools, and positional parameters, you can unlock even more power in your scripts.
FAQs
What is Bash $@ in scripting?
Bash $@ is a special variable that represents all arguments passed to a script or function. It treats each argument separately, making it ideal for processing multiple arguments.
How is Bash $@ different from $*?
$@ expands to each argument as a separate word when quoted, preserving spaces in arguments. $* combines all arguments into a single string, with a space separating them.
When should I use “$@” over “$*”?
Use “$@” when you need to preserve each argument individually, especially for multi-word arguments. Use “$*” if you want to merge arguments into one string.
What does ${} do in Bash?
The ${} syntax is used for parameter expansion, allowing manipulation or substitution of variables in Bash.
Can I pass arguments to a function using Bash $@?
Yes, you can pass all arguments to a function using $@, allowing flexible and reusable function design.
What is the difference between Bash $@ and positional parameters?
Bash $@ refers to all arguments as a list, while positional parameters like $1
, $2
refer to specific arguments passed to the script.
How do I process each argument passed to my script using Bash $@?
You can use a loop to process each argument passed to your script, ensuring fine-grained control over each one.
Can I pass Bash $@ to another script?
Yes, you can forward all arguments to another script by using $@.
What is the role of shift in Bash scripting?
The shift
command shifts positional parameters to the left, allowing you to process arguments one by one.
Does Bash work in Python scripts?
No, Bash $@ is specific to Bash. In Python, command-line arguments are accessed using sys.argv
.