Python’s zip()
function is a versatile and powerful tool for combining two lists into pairs. Whether you’re iterating over two lists simultaneously or creating tuples, zip()
simplifies your coding process.
This guide delves deep into how to use Python zip two lists effectively, providing examples, practical applications, and tips for handling complex scenarios.
What is Python’s zip()
Function?
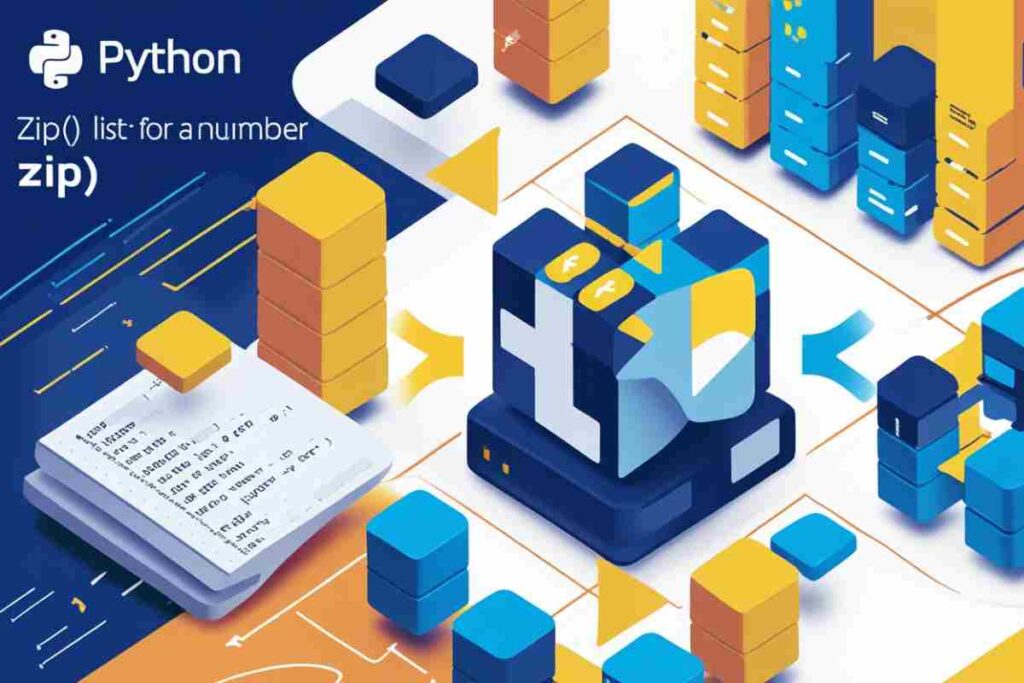
The zip()
function in Python combines elements from two or more iterables (like lists or tuples) into pairs. Each resulting pair contains one element from each iterable at the same index.
Here’s a basic syntax:

For instance:

By zipping these two lists, you create pairs of elements aligned by their indices.
Key Use Cases of Python Zip Two Lists
Iterating Over Two Lists Simultaneously
One of the most common applications is iterating over two lists together using a for-loop.
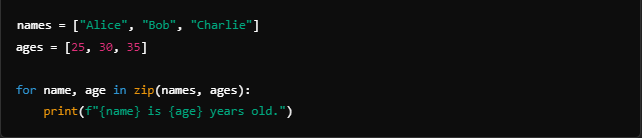
Output:

By combining lists, you avoid manual indexing and nested loops, resulting in cleaner code.
Creating Tuples from Two Lists
zip()
naturally creates tuples when combining lists.
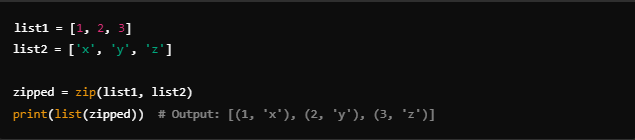
Each tuple contains paired elements from the input lists.
Handling Lists of Different Lengths
When lists of different lengths are zipped, the output is truncated to the shortest list.
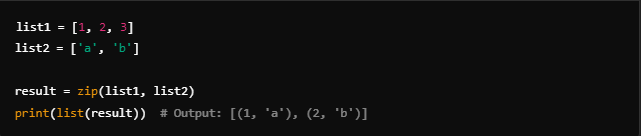
To retain all elements, use itertools.zip_longest
:
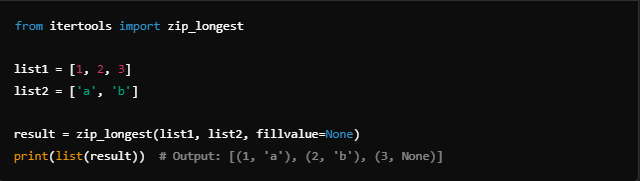
Advanced Applications
Transposing a Matrix
Matrix transposition rearranges rows into columns, and vice versa.

Creating Dictionaries
Pair lists to form a dictionary:
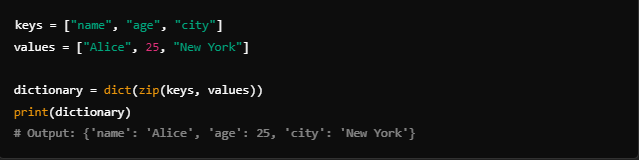
Parall3el Sorting
Sort two lists based on one of them:
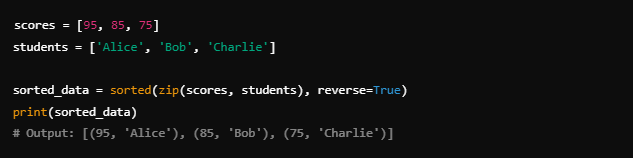
Why Use Python Zip Two Lists?
Using zip()
is not just about convenience; it’s also about making your code clean, readable, and efficient. Scenarios where you might need Python zip two lists include:
- Iterating over two lists together: Simplifies nested loops.
- Creating pairs of data: Useful in data processing or matrix operations.
- Handling varying list lengths: Learn how Python deals with uneven lists.
Let’s explore these use cases in-depth.
How to Use Python Zip Two Lists in Loops
One of the most common applications of zip()
is in for-loops. Here’s an example:
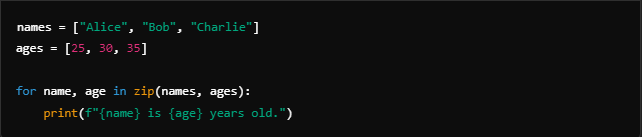
Output:

By using zip()
here, you iterate over both lists simultaneously without manually indexing them.
Converting Python Zip Two Lists into Tuples
The output of zip()
is an iterator, which can be converted into a list of tuples for better usability:
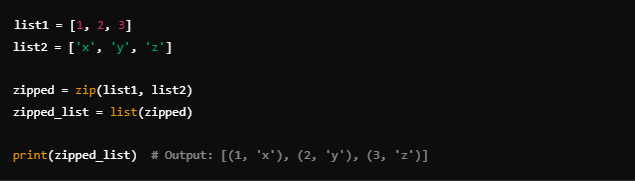
Each tuple represents a paired element from the two lists.
Handling Different Lengths: Python Zip Two Lists
When two lists have different lengths, the zip()
function stops at the shortest list.
Example:
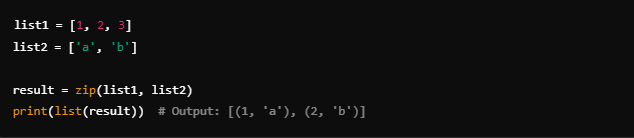
To avoid truncation, consider using itertools.zip_longest
:
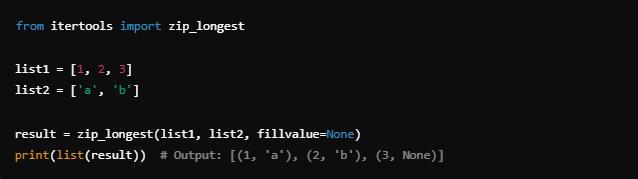
This method ensures no data loss when zipping lists of unequal lengths.
Practical Examples of Python Zip Two Lists
- Creating a Dictionary from Two Lists
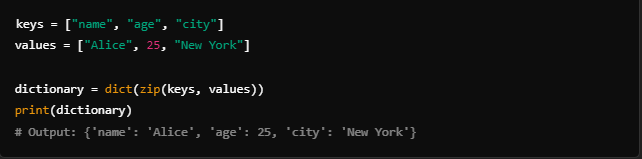
- Unzipping Lists
You can also reverse the zip()
operation using the *
operator:
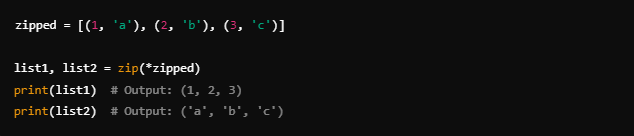
- Matrix Transposition
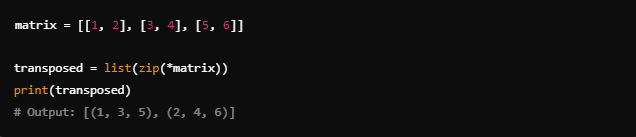
Performance Considerations
The zip()
function is highly efficient, as it uses lazy evaluation. This means it doesn’t create a full list in memory unless explicitly converted (e.g., with list(zip(...))
). This is ideal for large datasets.
Common Use Cases in Real-World Applications
- Data Analysis: Pairing columns of data for processing.
- Game Development: Mapping player names to scores.
- Web Development: Combining user inputs into structured formats.
Conclusion
The zip()
function in Python simplifies working with multiple lists by combining them into pairs, tuples, or even dictionaries, making code more efficient and readable.
From iterating through lists to handling varying lengths, its versatility is invaluable for developers, enabling cleaner, faster, and more efficient code execution in a wide range of applications.
Whether you’re working on data analysis, game development, or web applications, understanding and using Python zip two lists effectively can significantly enhance your coding skills.
FAQs
What does Python’s zip()
function do?
It combines elements from two or more lists into pairs based on their indices.
Can I zip more than two lists?
Yes, you can zip multiple lists by passing them as arguments to zip()
.
What happens if lists have different lengths?
zip()
stops at the shortest list. Use itertools.zip_longest
to handle unequal lengths.
How to unzip a zipped object?
Use the *
operator with zip()
, like zip(*zipped_object)
.
Can I create a dictionary using zip()
?
Yes, use dict(zip(keys, values))
to create a dictionary.
Is zip()
memory efficient?
Yes, it uses lazy evaluation, making it efficient for large datasets.
What is returned by zip()
?
It returns a zip object, which can be converted to a list or tuple.
How to handle missing values in zipped lists?
Use itertools.zip_longest
and specify a fillvalue
for missing elements.