In Cypress testing, objects play a significant role in managing test results, DOM elements, and configurations. To simplify debugging, logging, API interactions, or comparisons, converting these objects into strings is often necessary.
This guide focuses on how to efficiently Convert object to string Cypress using native JavaScript methods like JSON.stringify()
, handling nested structures, and overcoming challenges like circular references.
Whether you’re a beginner or an experienced tester, this resource will streamline your workflow and help you make the most of Cypress testing.
Why Convert object to string cypress?
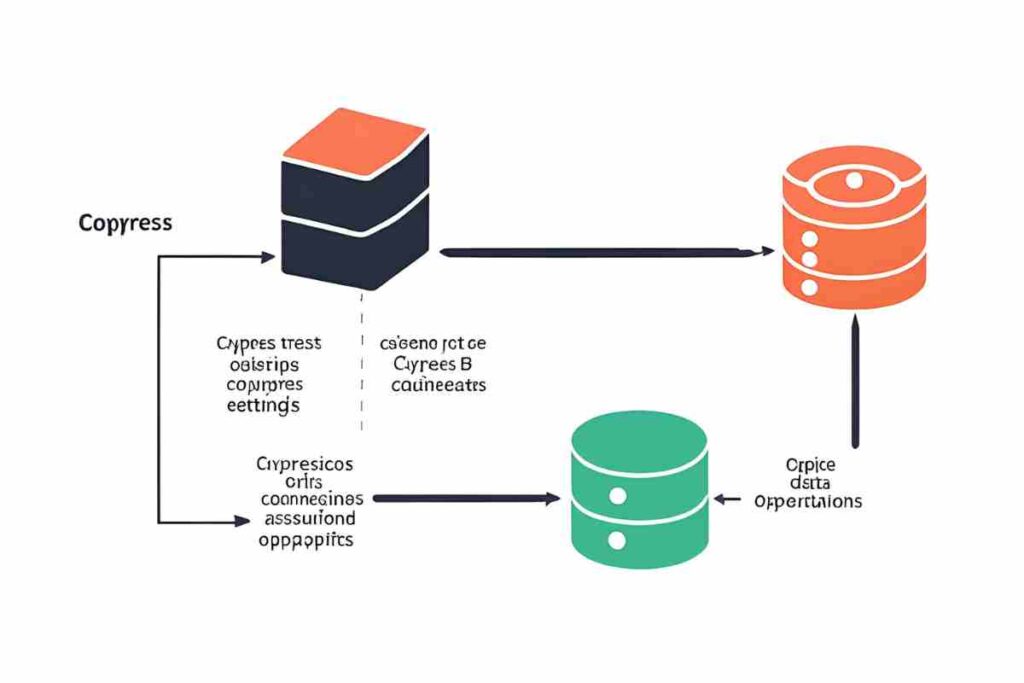
In Cypress, objects are frequently used to store various types of data such as test results, configuration settings, and elements in the DOM.
However, when dealing with objects, especially in assertions or when sending data to APIs, the need to Convert object to string Cypress often arises.
Whether you need to display a readable version of the object or compare it with expected values, converting objects to strings is essential for clarity and functionality.
You might also encounter scenarios where you need to log the object or store it for later use in the form of a string, by using methods to Convert object to string Cypress.
In these cases, understanding how to convert an object to string in Cypress can be incredibly beneficial. Let’s dive deeper into the various techniques available in Cypress for performing this conversion.
Basic JavaScript Methods to Convert object to string cypress
Before we dive into Cypress-specific solutions, it’s important to understand how to convert objects to strings in JavaScript.
These methods are applicable in Cypress as it is built on top of JavaScript. Here are a few commonly used techniques to Convert object to string Cypress:
Using JSON.stringify()
The JSON.stringify()
method is one of the most commonly used approaches to convert an object to string in Cypress. This method takes a JavaScript object and returns its stringified version in JSON format. Here’s an example:

This approach is especially useful if you need to preserve the structure of the object and convert it into a string that can be easily logged or sent to an API.
However, note that functions and undefined values are not serialized using this method.
Converting Simple Objects
For simpler objects without nested structures, JSON.stringify()
works perfectly fine. Here’s a Cypress test example of how to Convert object to string Cypress within a Cypress test:
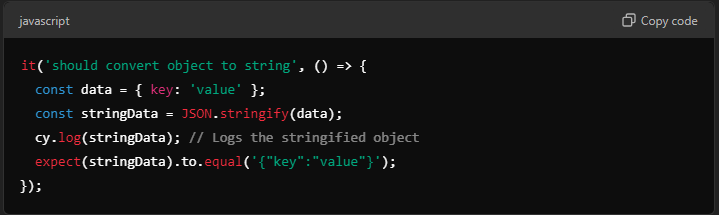
In the above example, cy.log()
is used to log the stringified object, and Cypress assertions ensure that the conversion worked as expected.
Handling Complex Objects in Cypress
When working with more complex JavaScript objects, the process to Convert object to string Cypress might require more handling, especially when dealing with nested objects or circular references. In such cases, there are a few best practices to follow:
Handling Nested Objects
Nested objects can easily be stringified using JSON.stringify()
, and the method will preserve their structure. Here’s an example:
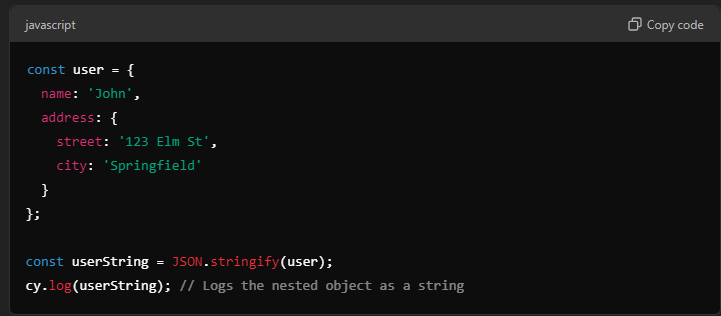
This ensures that the entire object structure, including nested keys, is maintained when converted to a string.
Dealing with Circular References
Circular references can cause JSON.stringify()
to fail. A circular reference occurs when an object references itself directly or indirectly, causing an infinite loop when you try to Convert object to string Cypress.
To handle such situations, you can use custom serialization logic or a library like flatted that supports circular references.

After installing flatted, you can use it to safely convert objects with circular references to strings:
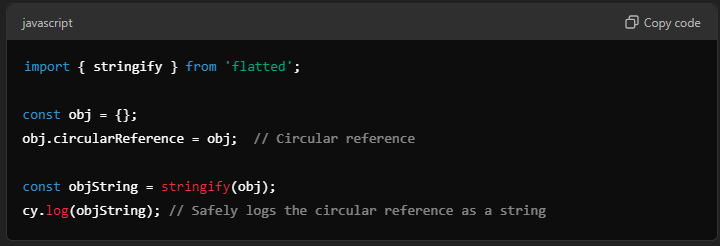
By using flatted
, you can convert even circular objects to a string without running into errors.
Convert object to string cypress Using GitHub Examples
If you prefer learning through real-life examples, the Cypress GitHub repository is a great resource. Here, you can explore various test scripts and see how Cypress development handle object-to-string conversion in real-world scenarios.
Many Cypress tests involve objects that need to be serialized, and GitHub provides a rich collection of code snippets for you to reference.
You can visit the official Cypress GitHub repository and search for examples related to object serialization. The Cypress community is constantly contributing to solving complex scenarios, and you might find ready-made solutions to your problem.
Convert object to string cypress with JSON Methods
In certain scenarios, converting an object to string in Cypress JSON format is especially useful. JSON serialization allows for a clean representation of the object, which is highly readable and can be used in API calls or other external systems. Here’s how you can use JSON methods effectively in Cypress:
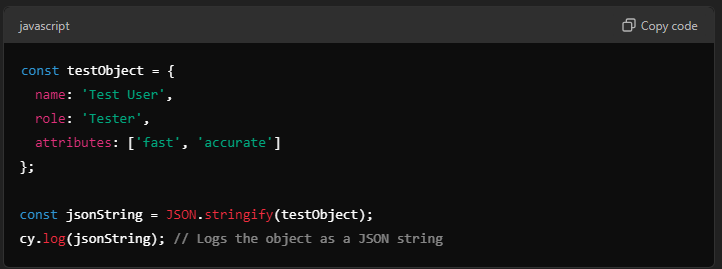
This stringified version is ideal for cases where you need to send the object as part of a network request or need a compact, readable representation of the data.
Use Cases for Converting Object to String in Cypress
Let’s take a look at some common use cases where you might need to convert an object to string in Cypress:
- Logging: Converting objects to strings allows you to log structured data in a readable format, making it easier to debug tests.
- API Requests: Many APIs require data to be sent as JSON strings. By converting objects to JSON format, you can easily send them in requests.
- Assertions: Sometimes, you might want to assert on a stringified version of an object to ensure data integrity across your tests.
- Data Storage: Storing objects as strings in local storage or session storage can help in saving data between different test runs or for cross-test comparisons.
Conclusion
Converting objects to strings in Cypress is essential for debugging, logging, API calls, data storage, assertions, error handling, reporting, and seamless test execution.
By using methods like JSON.stringify()
and handling complex cases such as circular references, you can simplify your testing workflow. Master these techniques to make your Cypress tests more efficient and effective!
FAQs
Can you convert an object to a string?
Yes, you can use methods like JSON.stringify()
in JavaScript to convert an object into a string format.
How to convert object data type into string?
Use JSON.stringify(object)
to convert an object data type into a string while maintaining its structure.
How to convert file object into string?
For a file object, you can use FileReader
with readAsText()
to read and convert it into a string.
How do you convert a set object to a string?
Convert a Set
object to an array using Array.from()
or the spread operator, then use JSON.stringify()
.
Which method is used to convert objects to string?
The most common method is JSON.stringify()
, which serializes objects into JSON string format.
How do I convert a character set to a string?
Use the Array.from()
method on the set, then join the characters using .join('')
.
How to parse an object to string?
Parsing typically involves converting objects to strings using JSON.stringify()
, maintaining the JSON format.
How to convert an object to a string in JavaScript?
In JavaScript, use JSON.stringify()
to convert an object into a string for logging, API calls, or storage.