Memory management plays a crucial role in optimizing deep learning workloads, especially when using PyTorch on GPUs. One of the key parameters that impact memory fragmentation and performance is max_split_size_mb
.
Understanding how to configure this setting can significantly improve memory utilization and prevent out-of-memory (OOM) errors.
In this article, we’ll explore max split size, its default value, implementation in various frameworks like Stable Diffusion, Hugging Face, and Automatic1111, and how it can be set in different environments like Google Colab.
max split size mb is a PyTorch environment variable that controls the memory allocator’s behavior. It specifies the maximum size (in megabytes) for which a memory block can be split. Setting this value correctly helps reduce fragmentation and optimize memory usage during model training and inference.
What is max split size mb?
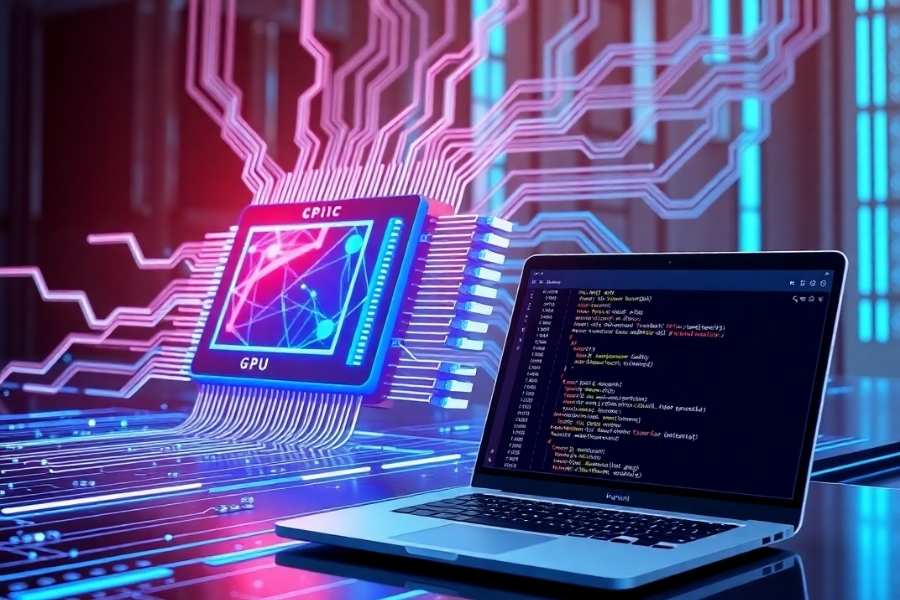
By default, max split size mb is not explicitly set in PyTorch. However, the CUDA memory allocator dynamically determines the best split size based on memory demands. If you experience excessive fragmentation or OOM errors, manually configuring max_split_siz
e can be beneficial.
How to Set max_split_size
You can set max split size mb using the PYTORCH_CUDA_ALLOC_CONF
environment variable:
export PYTORCH_CUDA_ALLOC_CONF=max_split_mb:50
Or in a Python script:
import os
os.environ['PYTORCH_CUDA_ALLOC_CONF'] = 'max_split_size:50'
This ensures that memory blocks larger than 50 MB will not be split, helping maintain more contiguous memory space.
max split size mb in Stable Diffusion
Stable Diffusion, an AI-based image generation model, can be memory-intensive. Users running Stable Diffusion with Automatic1111 or Hugging Face’s implementations may need to fine-tune max split size mb to prevent OOM errors. Increasing this value can enhance model stability when generating high-resolution images.
For Automatic1111’s Web UI, set the configuration in your startup script:
set PYTORCH_CUDA_ALLOC_CONF=max_split_size_mb:512
This setting helps in optimizing memory allocation for large models.
max_split_size_mb
in Hugging Face Transformers
Hugging Face’s transformer models often demand high GPU memory. Setting max_split_size_mb
can prevent excessive memory fragmentation when fine-tuning large models like BERT, GPT-3, or T5.
Example:
os.environ['PYTORCH_CUDA_ALLOC_CONF'] = 'max_split_size_mb:256'
This ensures efficient memory utilization during training or inference.
Configuring max_split_size_mb
in Google Colab
Google Colab users often encounter OOM errors when training deep learning models. To mitigate this, set max_split_size_mb
before running your model:
import os
os.environ['PYTORCH_CUDA_ALLOC_CONF'] = 'max_split_size:128'
This adjustment can improve memory efficiency, especially for large models.
max_split_size_mb
and vllm
vLLM
is an optimized inference framework designed for serving large language models efficiently. Configuring max_split_size_mb
can help allocate memory more effectively and avoid fragmentation during inference. Users experimenting with vLLM
should test different values to optimize performance.
Advanced Memory Configuration
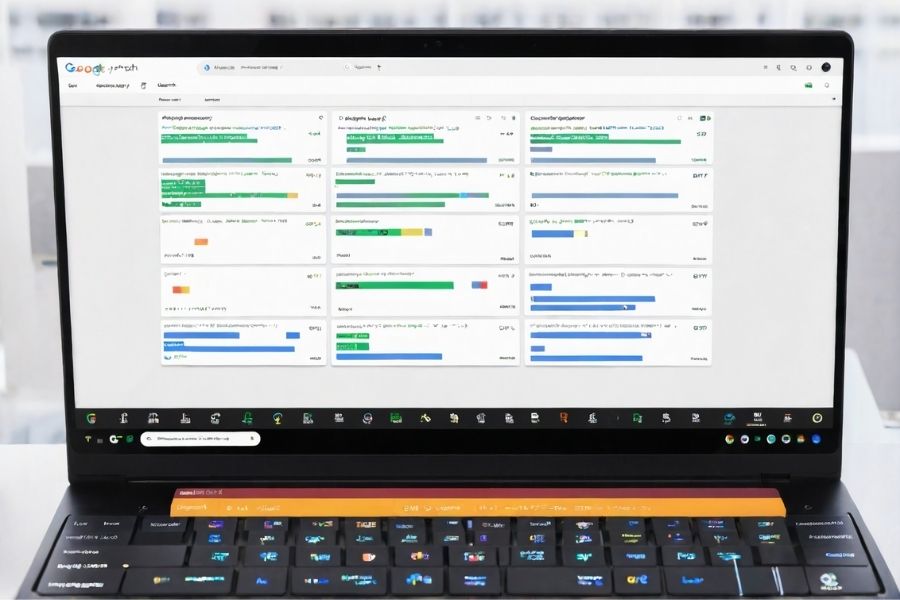
For advanced memory management, you can combine max_split_size_mb
with garbage collection threshold settings:
set PYTORCH_CUDA_ALLOC_CONF=garbage_collection_threshold:0.9,max_split_size:512
This setting ensures that memory is efficiently allocated while reducing unnecessary memory fragmentation.
Community Insights: max_split_size_mb
on Reddit
Reddit discussions highlight various user experiences with max_split_size_mb
. Common recommendations include:
- Setting max split size mb to 128 MB for general use.
- Increasing it to 512 MB for large-scale models like Stable Diffusion and GPT.
- Experimenting with different values based on available GPU memory.
max split size mb Tutorial and Example
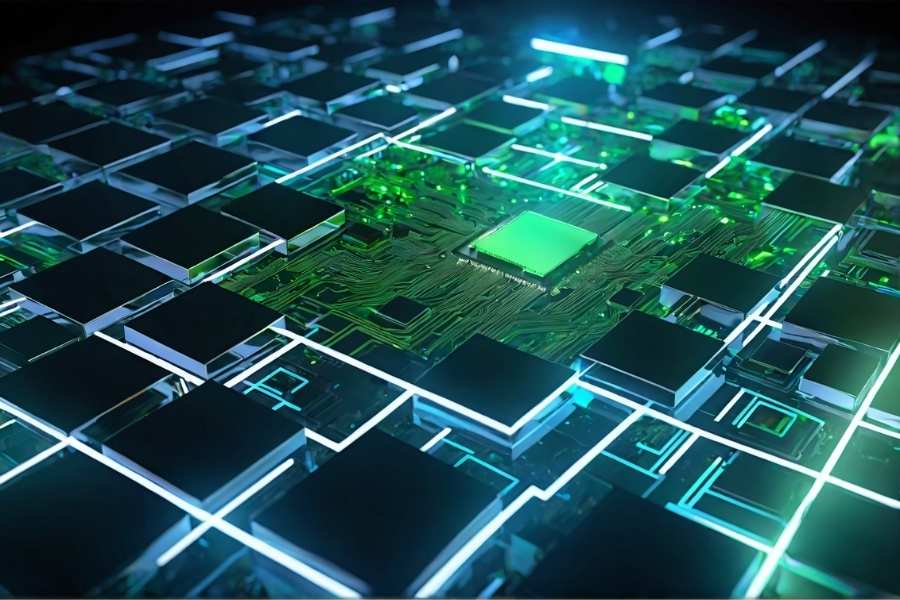
Example Usage in PyTorch:
import os
os.environ['PYTORCH_CUDA_ALLOC_CONF'] = 'max_split_size_mb:256'
import torch
# Load a model
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
model = torch.nn.Linear(100, 200).to(device)
This example demonstrates how to set max_split_size_mb
before loading a model in PyTorch.
Conclusion
Optimizing max_split_size_mb in PyTorch is essential for managing GPU memory efficiently, especially when working with large models in Stable Diffusion, Hugging Face Transformers, and vLLM.
While PyTorch’s memory allocator dynamically handles memory allocation, manually configuring max split size mb can help reduce fragmentation and prevent out-of-memory (OOM) errors.
The ideal value for max split size mb depends on your GPU memory availability and model size. Experimenting with different values—such as 128 MB for general use and 512 MB for large models—can significantly improve performance. Additionally, combining this setting with garbage collection thresholds can further optimize memory utilization.
For Google Colab users, setting an appropriate max split size mbvalue before running a model can prevent crashes and enhance efficiency.
Ultimately, fine-tuning max split size mb based on your specific deep learning workload can lead to better memory management and improved model performance.
FAQs
What is max split size mb in PyTorch?
It is an environment variable that controls PyTorch’s memory allocator, determining the maximum size of memory blocks before splitting.
Why should I set max_split_size_mb manually?
Manually setting it helps reduce memory fragmentation, optimize GPU usage, and prevent out-of-memory (OOM) errors.
What is the default value of max_split_size_mb?
By default, PyTorch dynamically determines the best split size, but it is not explicitly set unless configured by the user.
How do I set max split size mb in a Python script?
Use the following command before loading your model:
pythonCopyEditimport os
os.environ['PYTORCH_CUDA_ALLOC_CONF'] = 'max split size mb:128'
How does max split size mb affect Stable Diffusion?
For Stable Diffusion (Automatic1111, Hugging Face), setting 512 MB or higher can reduce OOM errors and improve stability when generating high-resolution images.
What value should I use for Hugging Face Transformers?
For large models like BERT, GPT, or T5, setting 256 MB or more helps manage memory efficiently during fine-tuning and inference.
Can I set max split size mb in Google Colab?
Yes, use os.environ[‘PYTORCH_CUDA_ALLOC_CONF’] = ‘max split size mb:128’ before running your model to optimize memory allocation.
Does max_split_mb help with vLLM?
Yes, adjusting it can improve memory efficiency and reduce fragmentation when serving large language models with vLLM.
Can I combine max_split_size with other settings?
Yes, you can combine it with garbage_collection_threshold to further enhance memory efficiency and reduce fragmentation: