Working with substrings is a core skill for anyone working with text data in Python. A substring refers to a specific part of a larger string, and Python provides several powerful tools and methods for extracting and manipulating these substrings.
In this guide, you’ll discover how to leverage Python’s slicing syntax, built-in string methods like split()
and find()
, and advanced techniques like regular expressions.
By mastering substring operations, you’ll enhance your ability to process and analyze text data efficiently, whether you’re dealing with simple or complex scenarios.
Understanding Substring Python
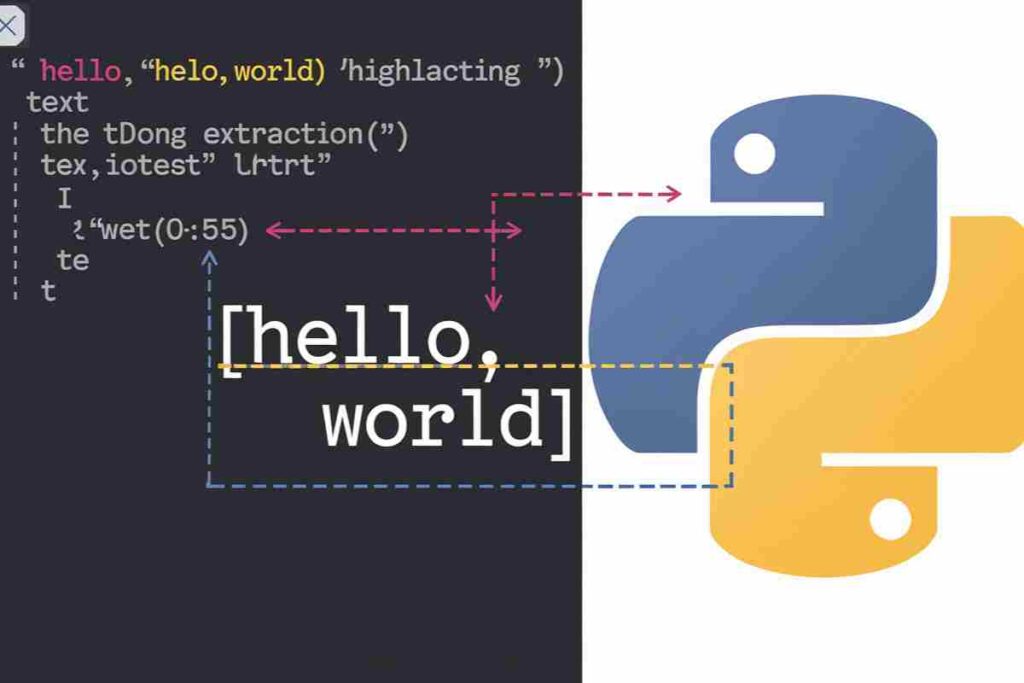
A substring in Python is simply a part of a string. Python provides a variety of ways to extract these substrings in Python, whether you’re looking for a specific section of text or need to manipulate strings for further processing.
The most common method for extracting substrings in Substring Python is by using slicing, a powerful technique built into Python.
How to Extract a Substring in Python
To get a substring in Python, you can use slicing. The slicing syntax is simple and works as follows:

- start: The starting index of the substring (inclusive).
- end: The ending index of the substring (exclusive).
For example:

Here, the substring is extracted from index 0 to index 4 (the character at index 5 is not included). The above example demonstrates how to extract the first 5 characters from the string “Hello, world!”.
Python Substring Before Character
“One common use case for substring Python is extracting the part of a string before a specific character. For instance, you might want to extract everything before a comma or a space. Here’s how you can do it:

In the example above, we used the split()
method, which splits the string at each comma, and then accessed the first element (everything before the comma). This is a quick and easy way to extract substrings before a specific character.
If you’re looking for a more general solution without knowing the character ahead of time, you can use the find()
method to locate the character and then use slicing:

Python Substring After Character
Similarly, you can also extract the substring after a specific character. For example, if you want to grab everything after the comma in the string “Hello, world!”, here’s how to do it:

In this case, we used the find()
method to locate the position of the comma, then sliced the string starting from the character after the comma.
Substring Python Methods and Techniques
Python has several built-in methods to help with string manipulation, making it easier to extract Substring Python based on specific conditions. Some of the most useful methods include:
Find()
The find() method in Substring Python returns the index of the first occurrence of a specified substring. If the substring is not found, it returns -1. It can be useful when extracting substrings before or after a specific character.

You can combine the find()
method with slicing to easily extract substrings.
Split()
The split()
method divides a string into a list based on a delimiter (e.g., a space, comma, or any other character). It’s especially helpful when you need to extract parts of a string that are separated by a particular character.

By splitting the string, you can get a list of substrings and easily access the part of the string you need.
Replace()
If you’re looking to extract or manipulate a substring in place, you can use the replace()
method to substitute one part of a string with another. This method is useful for modifying the string’s content before extracting a substring.

Using Regular Expressions for Complex Substrings
In some cases, you may need to work with more complex patterns or multiple conditions when extracting substrings. This is where regular expressions (regex) come into play. The re
module in Python allows you to define patterns to search for substrings that match specific rules.
For example, to extract everything before a comma using regex:
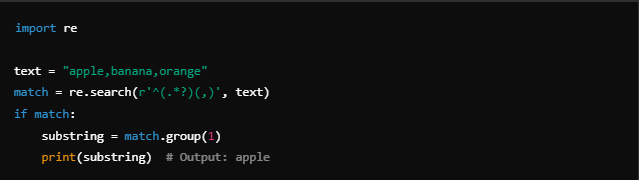
This regular expression searches for the first occurrence of a comma and extracts everything before it. The re.search()
method returns a match object, and match.group(1)
retrieves the first captured group, which is the substring before the comma.
Substring Python W3Schools Example
W3Schools is a popular resource for learning Python and other programming languages. They offer simple tutorials and examples, including one on how to handle substrings in Python.
You can access their article on Python substrings for more examples and detailed explanations on string slicing and other techniques.
Conclusion
In Python, mastering substrings is key to effective text manipulation. Using slicing, split()
, find()
, and regular expressions, you can easily extract parts of a string.
Whether you’re grabbing substrings before or after a character, Substring Python provides powerful tools for these tasks, making string manipulation easy and efficient.
If you’re new to Python or want to improve your skills, practicing substring techniques will help. Also, don’t forget to explore Python’s documentation and resources like W3Schools for more tips.
FAQs
What is a substring Python?
A substring is a part of a string. It can be extracted using slicing or string methods in Python.
How do I extract a substring in Python?
You can extract a substring using slicing: string[start:end]
.
How can I get a substring before a character in Python?
Use split()
or find()
to get a substring before a specific character.
How do I extract a substring after a character in Python?
Use find()
to locate the character, then slice the string from the next index.
What is string slicing in Python?
String slicing allows you to extract a part of a string using the syntax string[start:end]
.
How can I check if a substring exists in Python?
Use the in
keyword: substring in string
.
What is the difference between find()
and index()
?
find()
returns -1 if not found, while index()
raises an error.
Can I use regular expressions for substring extraction?
Yes, the re
module allows extracting substrings using regular expressions for complex patterns.