When working with files and filenames in programming, one of the most common tasks is extracting specific information embedded within the filename.
One of the most frequent needs is extracting an ID (like a user ID, order ID, or product ID) from the filename, and this can be efficiently done using regular expressions (regex).
In this comprehensive guide, we’ll explore how to use regex to extract IDs from filenames, common use cases, and tips to improve the accuracy of your regex patterns.
Whether you’re processing large datasets, automating file management tasks, or organizing files by their IDs, this guide has you covered.
What is Regex and How Does It Help in Extracting IDs from Filenames?
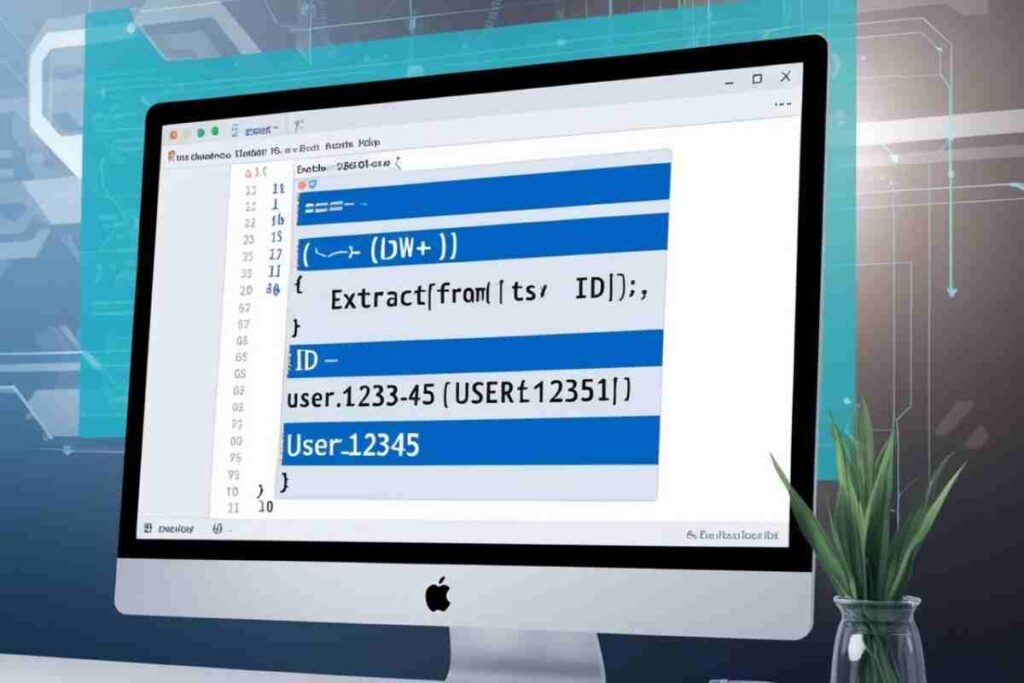
Regex (short for regular expressions) is a powerful tool used for pattern matching and text manipulation. It allows you to search, match, and manipulate text in a flexible manner, making it ideal for tasks like extracting specific parts of filenames, such as IDs.
When filenames contain patterns, regex enables you to extract meaningful information, such as IDs, timestamps, or other data, based on defined patterns. This is especially useful in environments where filenames are structured but the data within them needs to be parsed automatically.
How to Extract ID from Filename Using Regex: Basic Example
Consider the following filename: user12345_report.txt
.
Here’s how you can use regex to extract the ID (user12345
):
- Regex Pattern:
^(\w+)_
^
: Asserts the start of the string.(\w+)
: Matches and captures one or more word characters (letters, digits, underscores)._
: Matches the underscore separating the ID from the rest of the filename.
This regex pattern will successfully capture the ID user12345
from filenames that follow the user12345_report.txt
format.
Common Use Cases for Regex to Extract IDs from Filenames
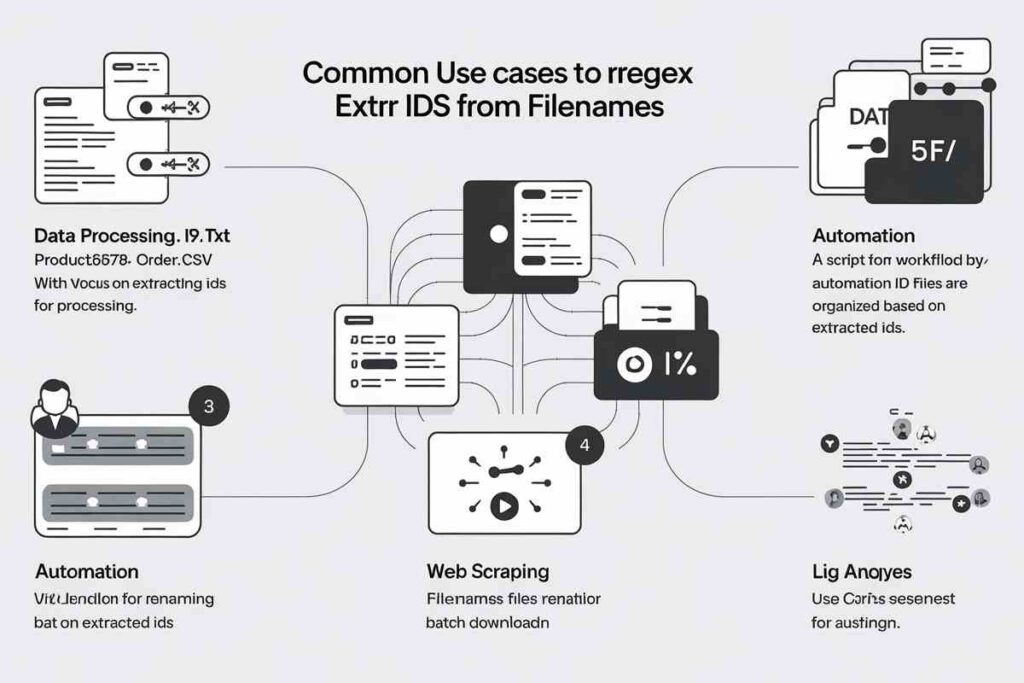
Regex for extracting IDs from filenames is widely used in various fields. Here are some common use cases:
- Data Processing: When dealing with large datasets, extracting user IDs, order IDs, or product IDs from filenames helps automate processing and file organization.
- Example: Organizing customer order files where each filename contains a unique order ID.
- File Management: Regex can help automate file organization based on extracted IDs. For example, sorting files into folders named after user or product IDs.
- Example: Automatically sorting invoices by customer ID.
- Automation: Regex is commonly used in scripts for automatically processing files, such as renaming, moving, or deleting files based on their extracted IDs.
- Example: A batch script that renames files by removing timestamps or other non-essential parts of the filename.
- Web Scraping: In web scraping, filenames or URLs often contain IDs that need to be extracted for data analysis.
- Example: Extracting video IDs from URLs for batch downloads.
- Log Analysis: Filenames that include session or user IDs are often used in log files. Regex helps in extracting these IDs for analysis.
- Example: Analyzing session data for a specific user ID.
Key Regex Components for Extracting IDs from Filenames
To effectively use regex for extracting IDs, it’s important to understand the key components of a regex pattern:
- Literal Characters: These directly match the characters in the filename, such as letters or numbers.
- Special Characters: These are used to match specific patterns:
\d
for digits (e.g.,123
)\w
for word characters (letters, digits, or underscores).
for any character except a newline
- Anchors:
^
and$
are used to match the beginning and end of the string, respectively. - Quantifiers: These specify how many times a pattern should appear:
+
means one or more occurrences (e.g.,\d+
for one or more digits)*
means zero or more occurrences
- Escape Sequences: These are used for special characters in filenames (e.g.,
\s
for space,\?
for a question mark).
Advanced Regex Examples for Extracting IDs
- Extracting Alphanumeric IDs:
- Pattern:
^(\w+)_.*\.[a-z]+$
- This will capture an alphanumeric ID followed by any other characters before the file extension. It works for filenames like
user123_report.csv
oradmin_4567_data.txt
.
- This will capture an alphanumeric ID followed by any other characters before the file extension. It works for filenames like
- Pattern:
- Handling Multiple IDs in Filenames:
- Pattern:
(\d+)_.*_(\d+)
- This pattern extracts two numeric IDs separated by underscores. It’s useful for filenames like
user1234_order5678.txt
.
- This pattern extracts two numeric IDs separated by underscores. It’s useful for filenames like
- Pattern:
- Extracting IDs with Different Extensions:
- Pattern:
^(\w+)_.*\.[a-zA-Z]+$
- This pattern captures the ID before any file extension, regardless of whether it’s
.txt
,.csv
,.jpg
, etc.
- This pattern captures the ID before any file extension, regardless of whether it’s
- Pattern:
Common Issues and How to Fix Them
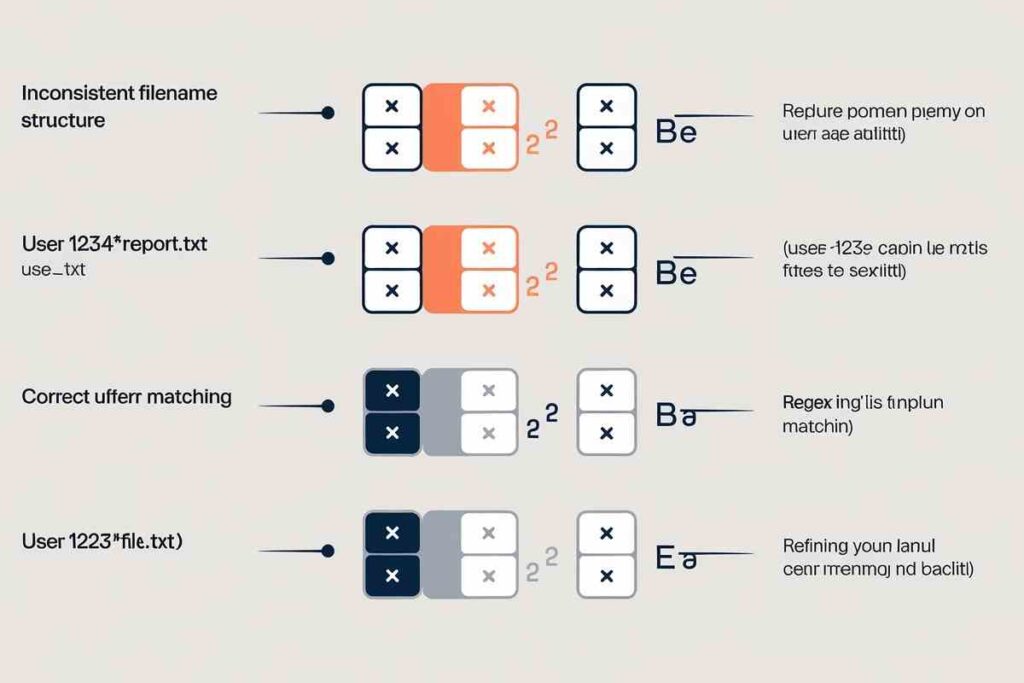
When using regex to extract IDs from filenames, some common issues may arise. Here are the issues and their solutions:
- Inconsistent Filename Structure:
- Issue: Filenames that don’t follow a consistent pattern can break your regex.
- Fix: Establish a naming convention to standardize filenames. If filenames vary significantly, use multiple regex patterns to handle different cases.
- Incorrect Pattern Matching:
- Issue: Your regex may not capture the right portion of the filename.
- Fix: Refine your pattern by using more specific capture groups or anchors. Use tools like Regex101 to test and debug your regex.
- Handling File Extensions:
- Issue: Variations in file extensions can cause regex mismatches.
- Fix: Use a general pattern like
\.[a-zA-Z]+$
to match any file extension, not just one specific type.
- Special Characters in Filenames:
- Issue: Filenames with spaces, special characters, or punctuation may cause issues.
- Fix: Use escape sequences (e.g.,
\?
for a question mark) to handle special characters.
Best Practices for Writing Regex to Extract ID from Filenames
- Ensure Consistent Naming: Having a consistent file naming convention reduces complexity and minimizes the chance of mismatches.
- Test Your Patterns: Use online tools like Regex101 to test your regex with different sample filenames before deploying it.
- Refine Your Patterns: Start with simple patterns and refine them as you identify edge cases or variations in your filenames.
- Handle Edge Cases: Consider scenarios where filenames might have multiple IDs, special characters, or various extensions. Adjust your regex accordingly.
Conclusion
Using regex to extract IDs from filenames is an incredibly useful technique for organizing and automating tasks related to file management and data processing. By understanding the fundamentals of regular expressions and refining your patterns, you can ensure that your ID extraction is reliable and accurate.
If you are dealing with large datasets, automating file management, or processing files in a batch, mastering regex can save time and reduce errors. With the examples and tips provided, you’ll be able to handle filenames in various formats and structures with ease.
FAQS
What is regex, and how does it help in extracting IDs from filenames?
Regex (regular expression) is a tool used to match patterns in text. It helps extract specific parts, like IDs, from filenames based on patterns you define.
How do I write a regex to extract an ID from a filename?
To extract an ID, use a pattern like ^(\w+)_
where \w+
captures the ID before the underscore. Adjust the pattern depending on your filename structure.
What if filenames have different structures?
If filenames vary, you may need multiple regex patterns or conditional statements to handle the differences and ensure accurate extraction.
Can regex handle different file extensions?
Yes, regex can be customized to match any file extension by using patterns like \.[a-zA-Z]+$
to match various types of extensions.
How do I test my regex pattern?
You can test your regex pattern using online tools like Regex101 to input sample filenames and verify if it extracts the ID correctly.
How can I handle filenames with special characters or spaces?
Use escape sequences in your regex pattern to handle special characters like spaces (\s
), question marks (\?
), and others to ensure proper extraction.
Is regex case-sensitive when extracting IDs from filenames?
By default, regex is case-sensitive. To match both uppercase and lowercase letters, you can use the i
flag (e.g., /^(\w+)_/i
).
What should I do if my regex isn’t working as expected?
Refine your regex pattern by adjusting capture groups, anchors, or special characters. Make sure it fits the exact structure of your filenames.