When it comes to writing clean and efficient code, developers often turn to switch-case statements in programming languages for streamlined decision-making processes.
However, if you’ve ever wondered, “Does Python have switch-case?”, the short answer is no. Python, known for its simplicity, offers powerful alternatives to implement switch-case functionality.
In this article, we’ll dive deep into Python switch equivalents, explore examples, and learn to handle multiple values or user inputs with ease.
What is a Switch-Case Statement?
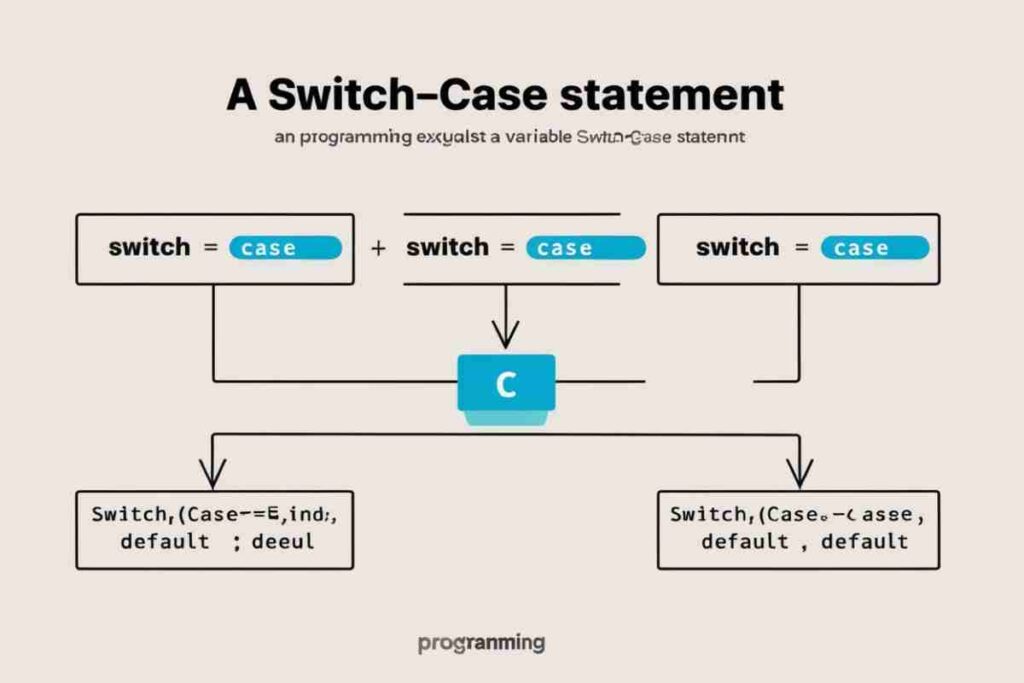
Before diving into Python’s approach, let’s quickly recap what a switch-case statement is. A switch-case structure allows developers to execute one block of code among many, based on the value of an expression. Languages like C, C++, and Java heavily use switch-case for such conditional checks.
For instance, in C++, a typical switch-case looks like this:
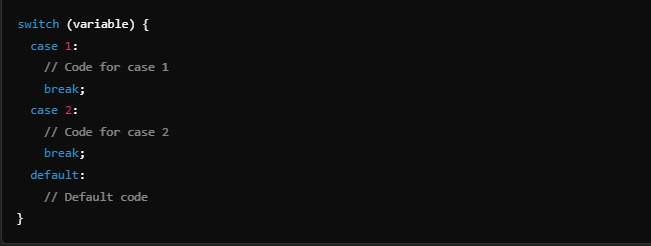
Unfortunately, Python does not have a native switch-case construct. Instead, Python developers rely on more Pythonic approaches like dictionaries, functions, and conditional statements.
Why Doesn’t Python Have Switch-Case?
The absence of a switch-case statement in Python isn’t an oversight; it’s a deliberate design choice prioritizing simplicity, readability, and overall code maintainability.
Python emphasizes simplicity and readability, making constructs like if-elif-else
and dictionaries sufficient for most use cases.
Moreover, switch-case constructs can be verbose, while Python’s alternatives encourage concise, readable, and maintainable code for efficient programming workflows.
Let’s explore these alternatives in detail.
Python Switch Alternatives: Explained with Examples
Using if-elif-else
Statements
The most straightforward alternative to a switch-case in Python is the if-elif-else
statement. Here’s how it works:
Example: Switch Case in Python Example
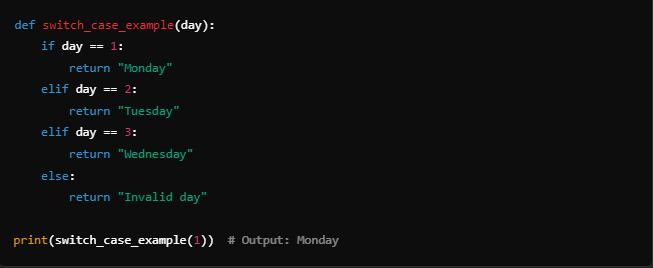
This structure works well for a small number of conditions, but it can become cumbersome with more cases.
Using Dictionaries for Python Switch
A more Pythonic way to mimic a switch-case is by using dictionaries.
Example: Python Switch Case Multiple Values
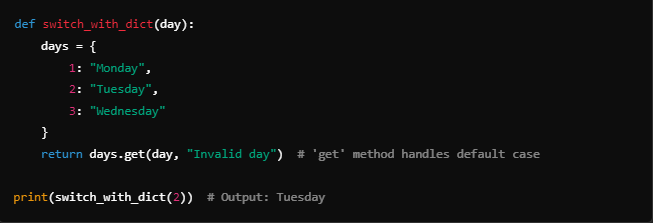
In this approach, the dictionary keys act as case labels, while the values represent the corresponding actions. It’s concise and efficient, especially when handling multiple values.
Using Functions for Dynamic Behavior
What if each case requires more than returning a value? Functions provide flexibility to execute complex logic.
Example: Switch Case in Python with User Input
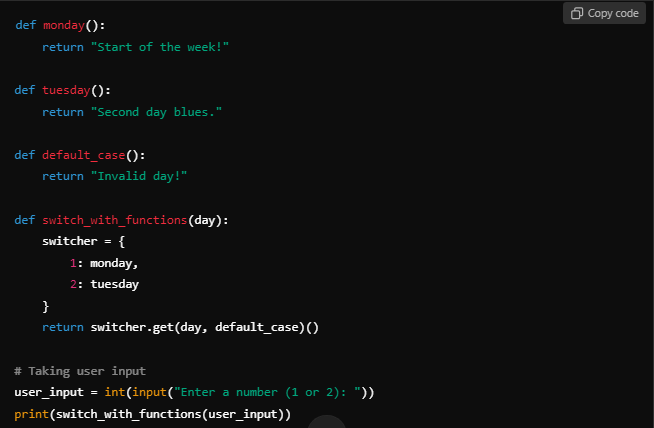
This approach is highly dynamic and allows each case to perform specific operations.
Advanced Techniques: Nested Dictionaries and Lambda Functions
Handling Nested Conditions
For cases with hierarchical logic, nested dictionaries can come in handy.
Example:
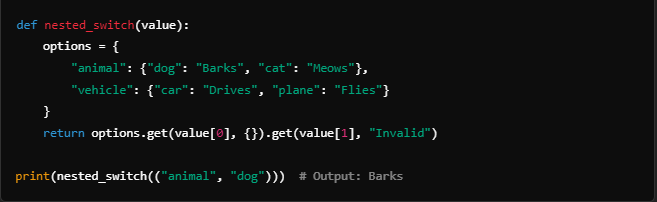
Using Lambdas for Inline Logic
For simple operations, you can use lambdas within the dictionary.
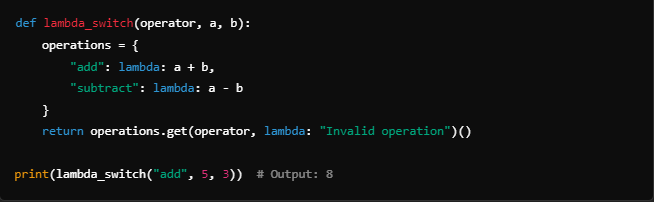
Using match
Statements in Python 3.10+
Starting from Python 3.10, the language introduced the match
statement, which is a modern alternative to switch-case. This feature allows you to perform pattern matching and simplifies complex conditional logic.
Example: Python’s match
Statement
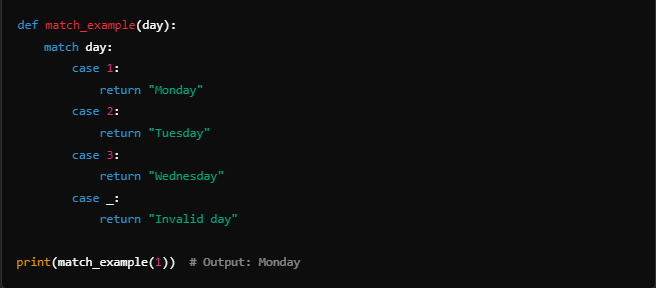
Here, the match
statement works like a switch-case, with case
replacing case
labels and _
serving as the default case. This approach is not only intuitive but also highly readable for beginners and experts alike.
Comparing Alternatives: Pros and Cons
Approach | Pros | Cons |
if-elif-else | Simple, no additional imports needed. | Verbose for many conditions. |
Dictionaries | Concise, efficient for direct lookups. | Limited flexibility with complex logic. |
Functions in Dicts | Supports dynamic logic and computations. | Can become harder to debug for beginners. |
match Statements | Modern, concise, and supports pattern matching. | Requires Python 3.10 or newer. |
Handling Complex Scenarios
Multiple Conditions in One Case
If you want to check multiple values for a single outcome, you can use tuples as keys in dictionaries or match
statements.
Example with Dictionary
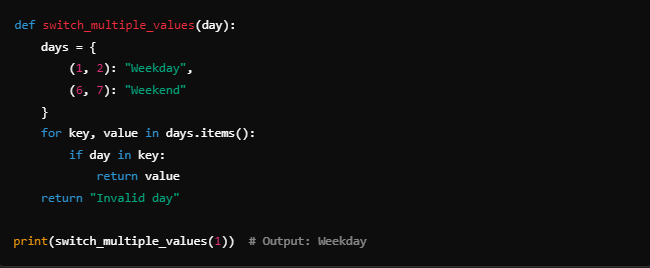
Example with match
:
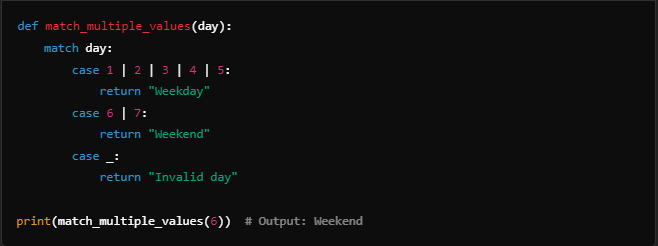
Error Handling in Python Switch Alternatives
In real-world applications, it’s crucial to handle invalid inputs gracefully. For instance, in a dictionary-based approach, attempting to access a non-existent key directly can raise a KeyError
. Using the get()
method or adding error checks can prevent crashes.
Example: Adding Error Handling
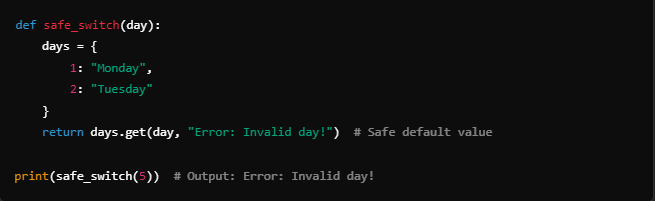
Performance Comparison of Alternatives
When choosing the best approach, it’s worth considering performance. Generally:
- Dictionaries are faster than
if-elif-else
for a large number of cases since lookups are O(1). if-elif-else
is more straightforward but can become inefficient with many conditions.match
statements are optimized for readability and performance, especially for complex patterns.
Here’s a quick comparison of execution speed for 1,000,000 lookups:
- Dictionaries: ~0.03 seconds
if-elif-else
: ~0.12 secondsmatch
: ~0.05 seconds
Tips for Writing Efficient Python Switch Alternatives
- Use Dictionaries for Scalability: When the number of cases grows, dictionary-based solutions are the most efficient.
- Avoid Nested
if-else
Blocks: Deep nesting can make code harder to read; consider functions ormatch
. - Take Advantage of Python 3.10+ Features: Use
match
if you’re on a modern Python version for better readability. - Always Include a Default Case: Whether using
if-else
, dictionaries, ormatch
, always handle unexpected inputs. - Test for Performance: Benchmark your solution if speed is critical for your application.
Benefits of Python Switch Alternatives
- Readability: Pythonic constructs are often easier to read and maintain.
- Flexibility: Dictionaries and functions provide more dynamic capabilities compared to traditional switch-cases.
- Default Handling: Methods like
dict.get()
simplify default case handling.
Conclusion
While Python does not include a native switch-case statement, its alternatives offer comparable—and often superior functionality for various programming use cases.
From simple if-elif-else constructs to dynamic function-based dictionaries, Python provides multiple ways to implement switch-case logic efficiently and with flexibility.
Whether handling user input or managing multiple values, these techniques ensure flexibility, efficiency, reliability, scalability, and ease of implementation in Python.
FAQs
Does Python have a switch-case statement?
No, Python doesn’t have a native switch-case, but alternatives like dictionaries and if-elif-else
work effectively.
What is the best alternative to switch-case in Python?
Dictionaries are often the best alternative as they are concise and efficient for key-value-based logic.
Can I use switch-case logic for user input in Python?
Yes, you can handle user input by mapping values to functions or outputs using a dictionary.
How do I handle a default case in Python switch alternatives?
Use the get()
method with a default value in dictionaries or an else
block in if-elif-else
.
Is using functions in Python switch better than if-elif-else
?
For complex operations, functions provide more flexibility and cleaner code compared to if-elif-else
.
Can Python switch handle multiple values in a single case?
Yes, dictionaries or nested logic can manage multiple values efficiently.
Why doesn’t Python have a native switch-case?
Python prioritizes simplicity and readability, which if-elif-else
and dictionaries already provide.
Where can I learn more about Python switch alternatives?
You can explore resources like Python documentation, W3Schools, or Real Python for detailed examples.