“Python Null” refers to the use of the keyword None
, which represents the absence of a value or “nothingness” in Python. Unlike other languages with a null
keyword, Python uses None
as a unique object and data type.
In this guide, we’ll delve into the concept of Python Null, exploring how to assign, check, and use None
effectively. With practical examples and best practices, you’ll gain the knowledge needed to write more robust and error-free Python applications.
What Is Python Null?
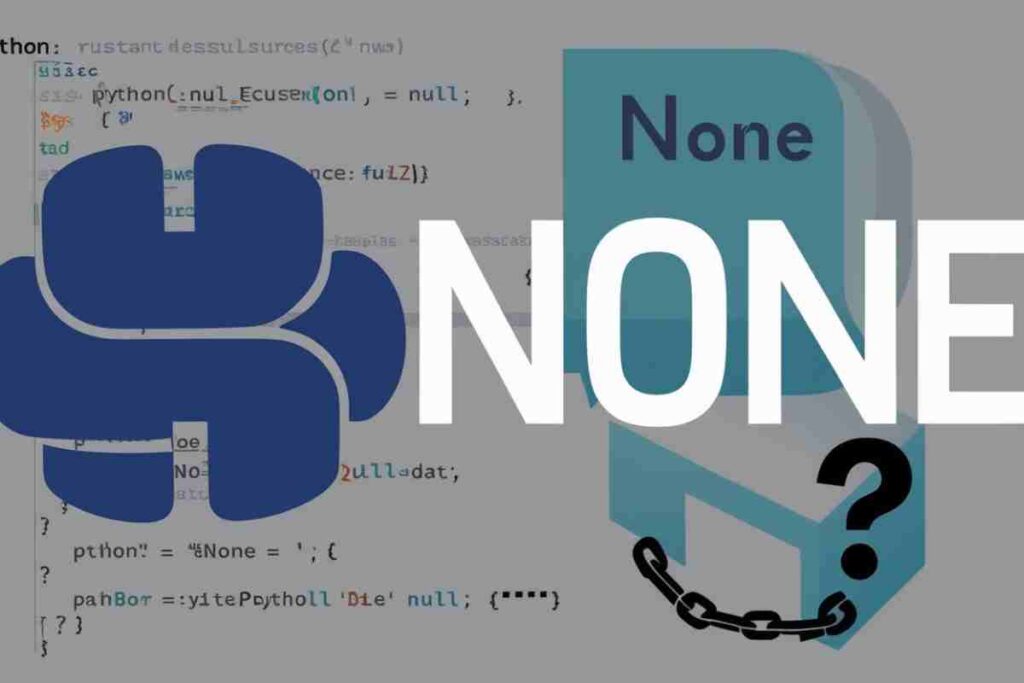
In Python, there isn’t a direct null
keyword like in other programming languages. Instead, Python uses None
to represent the absence of a value or a null state.
It is an object and a data type of its own, and it signals “nothingness.”
For example

The keyword None
is used in scenarios where no specific value has been assigned or where an object is meant to be empty. Understanding Python’s null equivalent (None
) is crucial for ensuring smooth coding practices.
How to Assign Null Value in Python
To assign a null value in Python, you simply use the None
keyword. Here’s how it works:

This sets variable
to a state where it holds no meaningful value. You can think of None
as Python’s built-in way to express “null value” or “no value.”
Why Use Python Null (None)?
The None
keyword in Python is often used:
To Initialize Variables
In situations where you want to declare a variable but don’t have a value to assign yet.pythonCopy code

Default Arguments in Functions
Functions can use None
as a default value when an argument isn’t provided.
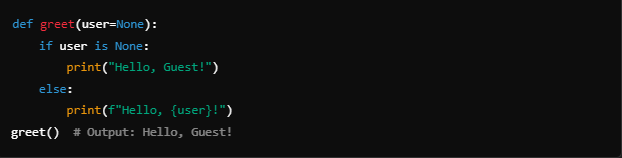
For Optional Return Values
When a function might not return a value under certain conditions.

Python Null Example
Here’s a practical Python null example:
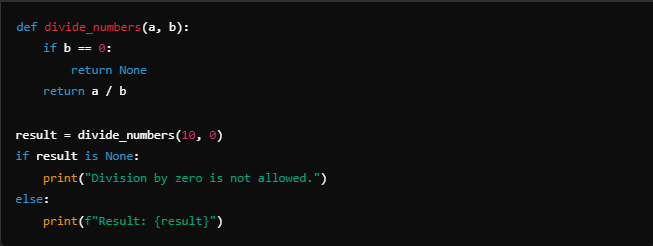
This code demonstrates how None
can be used to signify an invalid operation.
Python Null String
In Python, strings can’t hold a None
value directly as they are specific objects. However, a variable meant to hold a string can be assigned None
to indicate it has no value yet.
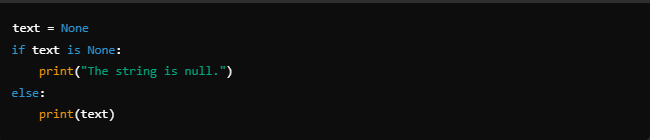
This is a practical use case where a Python null string can indicate the absence of user input or data.
Checking for Null in Python
To check if a variable is null, you use the is
operator. This ensures type-safe comparisons.
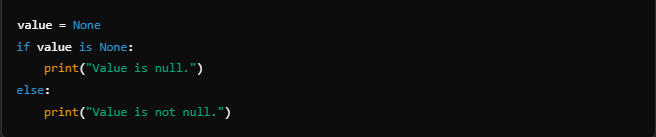
The is
operator is preferred over ==
for checking null values, as it compares the identity of objects rather than their values.
Handling Python Null in Real Applications
Using Python null (None
) effectively requires adopting best practices that not only ensure code clarity but also help prevent common errors. Below are some of the best practices for handling null values in real applications.
Avoid Unexpected Nulls
One of the most important best practices is to validate inputs properly to avoid accidental None assignments, ensuring smoother execution and preventing unexpected errors.
Unexpected None
values can lead to bugs, particularly in larger applications or systems that rely on user input or external data sources (such as APIs, databases, etc.).
To avoid unexpected nulls:
- Always validate inputs to ensure that variables are not accidentally assigned
None
when they should contain meaningful values. - Check for
None
before performing operations that assume the presence of data.
For example, consider a scenario where you’re processing user input:
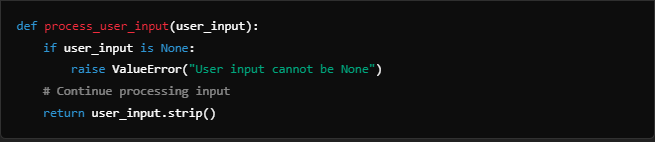
In this example, if the user_input
is None
, a ValueError
is raised with a meaningful message. This validation helps prevent unexpected behavior later in the application when operations are performed on a None
value.
Tip: It’s also a good idea to set sensible default values or make it explicit when a value is missing, rather than leaving it as None
.
Default Values
Using default values is a great way to handle cases where a value might not be provided. Python allows you to set default values for function arguments, which makes it easier to handle missing or optional inputs.
This technique reduces the chances of passing None
by accident and allows the function to handle defaults gracefully.
For example, consider a function that sends a welcome message to a user:

In this case, if user_name
is not provided, the function assigns "Guest"
as the default name. This approach ensures that the program doesn’t break, even if the caller didn’t pass any value.
Another Example: When working with configurations, often some configuration settings might not be passed by the user. By using default values, you can ensure that missing configurations don’t cause the program to fail.

In this case, the function will fall back to default configuration values if None
is provided or if no configuration is passed.
Null-aware Code
Writing null-aware code is crucial when you need to handle None
values without breaking the program. Instead of assuming a value is always present, you should write your code to handle None
explicitly.
This approach ensures that your functions can handle cases where None
is passed, and the code will run smoothly without errors.
Common techniques include using conditional checks, the or
operator, or Python’s built-in functions like get()
for dictionaries.
Example: Handling Missing Dictionary Keys
Consider a scenario where you’re working with a dictionary and trying to access a key that might be missing. If you attempt to access a missing key directly, it will raise a KeyError
.

Instead of directly accessing the key, you can use the get()
method, which returns None
(or a default value) if the key is missing

Using None
with Optional Function Arguments
Sometimes, functions need to handle optional arguments where the absence of a value is meaningful. Instead of using a sentinel value like -1
or "unknown"
, it’s better to use None
to clearly indicate that the argument was not provided.
For example, consider a function that logs user activity, where the user_id
argument might be optional
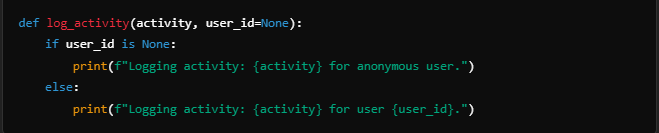
In this case, the absence of a user_id
is explicitly represented by None
, and the function can handle the logging accordingly, knowing that the user is anonymous.
Combining None
with Other Data Types
When working with more complex data structures, None
can be a useful placeholder to indicate the absence of a value.
This can be particularly useful when combining None
with other types such as lists, dictionaries, and custom objects.
Example: None in Lists

In this example, a list of users is filtered to only include those who have a non-None
status. The get()
method is used to safely access the status key, avoiding potential KeyError
exceptions.
Example: None in Custom Objects
In object-oriented programming, you might define a class where attributes may or may not be set by the user. For instance, consider a Book
class where the author
attribute is optional:

Here, if no author
is passed, the class sets the default value to "Unknown Author"
. This prevents the author
attribute from being None
and ensures that the class remains robust.
Differences Between Python Null and Null in Other Languages
Feature | Python (None ) | Java (null ) | C# (null ) |
---|---|---|---|
Keyword | None | null | null |
Data Type | Object | Primitive | Object |
Comparisons | is operator | == | == or ReferenceEquals |
Usability | Singleton | Primitive concept | Works with objects |
Conclusion
Python null (None
) is a versatile and essential concept in the language. Whether you’re initializing variables, managing function defaults, or handling the absence of a value, None
is the go-to construct.
By mastering how to assign, check, and work with Python null values effectively, you’ll ensure that your programs are robust, efficient, and error-free.
FAQs
What is Python null?
Python null is represented by the keyword None
, indicating the absence of a value.
How do you assign a null value in Python?
Use the None
keyword to assign a null value to a variable, like x = None
.
How do you check if a value is null in Python?
Use the is
operator, e.g., if x is None:
to check for null.
Can strings be null in Python?
Strings themselves cannot be null, but variables meant to hold strings can be set to None
.
What does None
mean in Python functions?
It’s often used as a default value or returned when a function doesn’t explicitly return anything.
Is None
the same as 0 or an empty string?
No, None
is a distinct object representing no value, unlike 0 or ""
.
How is None
used in optional arguments?
You can use None
as a default for optional arguments in functions.
Can you compare None
using ==
?
While possible, it’s better to use is
for checking None
to avoid type-related issues.