When writing Python code, you often encounter situations where you need to add explanatory notes, temporarily disable parts of your code, or document your logic.
Python offers a range of ways to comment code, and one common query developers have is: how to create Python multiline comments effectively and efficiently?
In this article, we’ll explore how to comment multiple lines in Python using various methods. We’ll also highlight shortcuts for popular Integrated Development Environments (IDEs) like VSCode and PyCharm and provide examples to help you understand how to use them effectively.
What is a Python Multiline Comment?
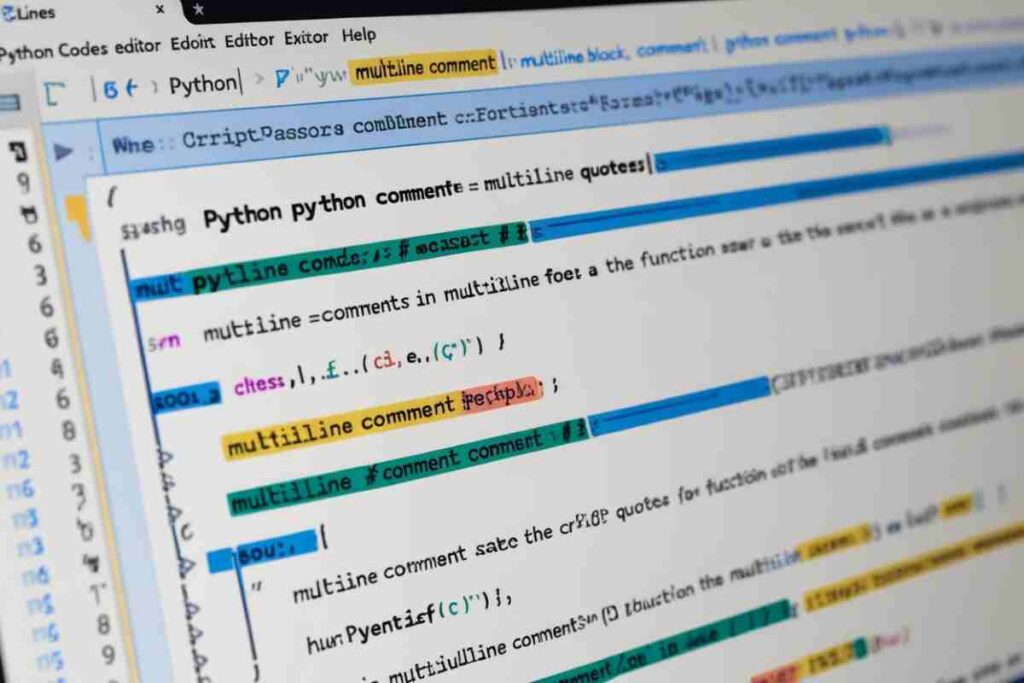
In Python, comments are vital for making your code more understandable to others (and yourself) by explaining the purpose of the code.
There are single-line comments (those that span one line) and multiline comments, which span multiple lines, providing more detailed explanations and context.
A Python multiline comment is simply a block of text that is ignored by the Python interpreter. It does not affect how your code runs and is used for adding detailed explanations, temporarily disabling code, or documenting functions, classes, or larger sections of code.
While Python doesn’t have a built-in syntax for multiline comments like some languages (e.g., /* */
in C), there are several ways to create multiline comments using triple quotes or multiple single-line comments.
How to Comment Multiple Lines in Python
Using Triple Quotation Marks for Multiline Comments
One of the simplest ways to write multiline comments in Python is to use triple quotes ('''
or """
). Triple quotes can span multiple lines, and everything enclosed within them is treated as a comment when not assigned to a variable.
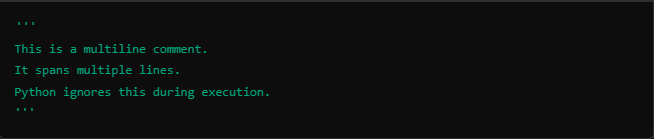
This method is commonly used to document code, but it can also be applied as a multiline comment if not assigned to any variable. Triple quotes can also serve as docstrings in Python, which are used to document functions or classes.
Example:
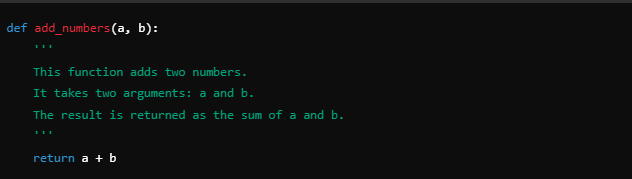
In this example, the comment explains what the function does, and Python ignores it during execution.
Using Hash (#
) for Multiple Single-Line Comments
Another way to comment out multiple lines in Python is to use a #
symbol at the beginning of each line. Although this isn’t as elegant for long explanations, it’s perfect for commenting out code or adding simple remarks.

This method is simple and straightforward. However, when you have long comments, using #
can be cumbersome, especially when commenting out large sections of code.
Python Comment Multiple Lines Shortcut
In popular Integrated Development Environments (IDEs) like VSCode and PyCharm, there are shortcuts available to help developers comment out multiple lines of code quickly.
These shortcuts can save time and improve productivity, especially when working with large codebases.
Python Comment Multiple Lines Shortcut in VSCode
In VSCode, you can easily comment out multiple lines using the following shortcut:
- Windows/Linux:
Ctrl + /
- Mac:
Cmd + /
This shortcut works for both single-line comments (#
) and multiline comments (using triple quotes). Here’s how to use it:
- Highlight the lines of code you want to comment.
- Press
Ctrl + /
(orCmd + /
on Mac).
VSCode will toggle comments on and off for the selected lines.
How to Comment Multiple Lines in PyCharm
In PyCharm, commenting out multiple lines is just as easy. The shortcut is similar to VSCode:
- Windows/Linux:
Ctrl + /
- Mac:
Cmd + /
This command adds #
to the beginning of each selected line, commenting them out. To comment a block with triple quotes in PyCharm, you will need to manually insert '''
or """
at the start and end of the block of code.
Best Practices for Python Multiline Comments
To make your Python code more readable and maintainable, it’s important to follow best practices when using multiline comments.
Use Triple Quotes for Documentation
When documenting a function, class, or module, it’s recommended to use triple quotes for your comments, as they provide clear and structured documentation.
This makes the code more readable and is also the standard for docstrings, which can be accessed using Python’s built-in help()
function.
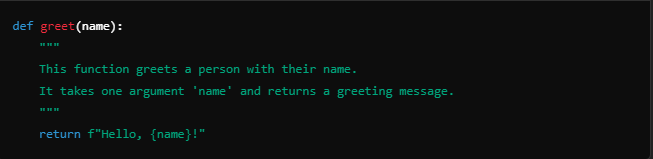
Use #
for Short Explanations
For simpler, inline comments, use the #
symbol. It’s clear and easy to use for short, temporary remarks.

Be Concise and Clear
When writing multiline comments, be concise. Avoid writing lengthy or redundant comments. Your aim should be to provide meaningful explanations that are easy to understand.

Common Issues with Python Multiline Comments
Commenting Out Large Blocks of Code
When you need to comment out a large block of code, Python allows you to use multiline comments effectively, making your code cleaner and more organized.
However, if your code includes single or double quotes within the block, this may result in syntax errors. In such cases, using the #
symbol on each line is the safer approach.
Unused Multiline Comments
Sometimes, developers use multiline comments to disable sections of code for testing or debugging. It’s important to remove these comments once you’re done, as leaving unused comments in your code can lead to confusion.
Conclusion
Understanding how to use Python multiline comments effectively is crucial for writing clean, readable, and well-documented code that is easy to maintain and understand.
Whether you’re using triple quotes, single-line comments, or the shortcuts available in popular IDEs like VSCode and PyCharm, it’s essential to document your code in a way that makes it easy for others (and your future self) to understand.
By following best practices for commenting and using shortcuts for efficiency, you can keep your Python code well-documented and maintainable.
FAQs
What is a Python multiline comment?
A Python multiline comment is a comment that spans more than one line, used for documentation or explaining code in detail.
How do you create a multiline comment in Python?
You can create multiline comments using triple quotes (”’ or “””) or by placing a hash (#) at the beginning of each line.
Can I use multiline comments in Python for documentation?
Yes, multiline comments are often used for documentation purposes, especially in functions or classes, to explain their purpose and usage.
What is the shortcut for commenting multiple lines in VSCode?
In VSCode, use Ctrl + / (Windows/Linux) or Cmd + / (Mac) to comment or uncomment multiple lines quickly.
How do you comment multiple lines in PyCharm?
In PyCharm, use Ctrl + / (Windows/Linux) or Cmd + / (Mac) to comment multiple lines with a hash symbol.
Can you nest comments in Python?
No, Python doesn’t support nested comments. Once a comment starts, the entire line is treated as a comment until the end.
Is using triple quotes for comments a good practice?
Yes, using triple quotes for multiline comments is a common practice, though it’s more often used for docstrings.
What’s the difference between comments and docstrings in Python?
Comments are used for explanations and are ignored by Python, while docstrings provide structured documentation and can be accessed during runtime.