Python Get Parent Directory when working with file paths in Python, you may need to navigate up a directory level to access parent directories.
Whether you are managing file structures, handling configuration files, or organizing scripts, retrieving the parent directory can be essential.
In this guide, we will explore multiple methods to Python get parent directory using pathlib
and os
modules.
Why Get the Parent Directory?
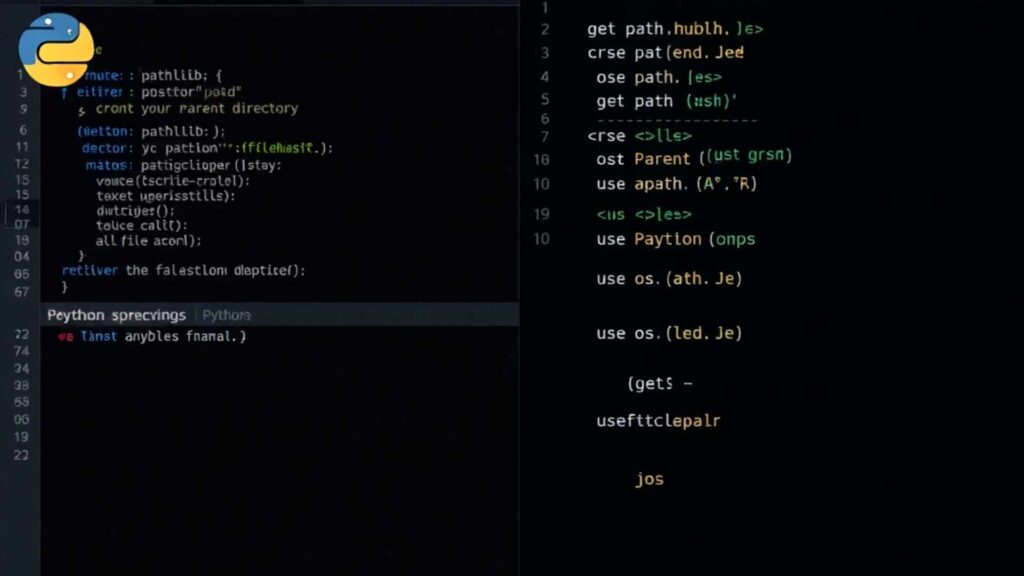
Understanding how to retrieve the parent directory is crucial for:
- Organizing file structures in applications.
- Loading configurations or assets from a common location.
- Working with relative paths in Python scripts.
- Managing project directories efficiently.
- Handling automation scripts that need dynamic file paths.
- Ensuring compatibility across different operating systems.
- Enabling portability in software development.
- Managing logs and temporary files dynamically.
Methods to Get the Parent Directory
Retrieving the parent directory is essential in many programming scenarios. Python provides multiple ways to achieve this, each with its advantages depending on the specific use case.
Below, we will explore various methods, including using pathlib
, os.path
, and os.pardir
, to retrieve parent directories efficiently.
Using pathlib
(Recommended for Python 3.4+)
The pathlib
module provides an object-oriented approach to handling file paths.
from pathlib import Path
# Get the current file's path
current_path = Path(__file__)
# Get the parent directory
parent_directory = current_path.parent
print(parent_directory)
Explanation:
Path(__file__)
creates aPath
object for the current script..parent
retrieves the immediate parent directory.
Example Output:
/home/user/project
Using pathlib
makes it easy to Python get parent directory efficiently.
Using os.path
The os
module provides functions for handling file paths in older versions of Python.
import os
# Get the current file's absolute path
current_path = os.path.abspath(__file__)
# Get the parent directory
parent_directory = os.path.dirname(current_path)
print(parent_directory)
Explanation:
os.path.abspath(__file__)
gets the absolute path of the script.os.path.dirname()
extracts the parent directory.
This is another efficient way to Python get parent directory using os.path
.
Using os.path
with os.pardir
Another approach is using os.pardir
, which represents the parent directory (..
).
import os
# Get parent directory using os.pardir
parent_directory = os.path.abspath(os.path.join(os.getcwd(), os.pardir))
print(parent_directory)
Explanation:
os.getcwd()
gets the current working directory.os.pardir
moves up one directory level.os.path.join()
combines them, andos.path.abspath()
ensures a full path.
Using os.pardir
is another way to Python get parent directory dynamically.
Getting the Parent Directory of the Working Directory
If you need the parent directory of the script’s working directory, use:
from pathlib import Path
# Get the parent directory of the current working directory
parent_directory = Path.cwd().parent
print(parent_directory)
This method ensures a simple way to Python get parent directory of the current working directory.
Use Cases
Understanding how to retrieve parent directories is useful in various practical applications. Below are some common scenarios where getting the parent directory is essential, including managing configurations, automating backups, and handling log files.
Handling Configuration Files
Many applications need access to a central configuration file stored in a parent directory:
from pathlib import Path
config_path = Path(__file__).parent / 'config.json'
print(config_path)
Automating File Backups
If you need to store backups in a parent directory:
import shutil
from pathlib import Path
destination = Path(__file__).parent / 'backup'
shutil.copy(__file__, destination)
Managing Log Files
Storing log files in a separate logs folder:
from pathlib import Path
log_directory = Path(__file__).parent / 'logs'
log_directory.mkdir(exist_ok=True)
log_file = log_directory / 'app.log'
print(log_file)
Each of these use cases involves an efficient way to Python get parent directory and work with files effectively.
Debugging Tips
- Always check if the path exists before using it:
from pathlib import Path
parent_directory = Path(__file__).parent
if parent_directory.exists():
print("Valid path")
else:
print("Path does not exist")
- Use
print(parent_directory)
to debug dynamically changing paths. - Ensure scripts using
__file__
are run as standalone programs.
Debugging can help ensure that your method to Python get parent directory works correctly.
Comparison: pathlib
vs os.path
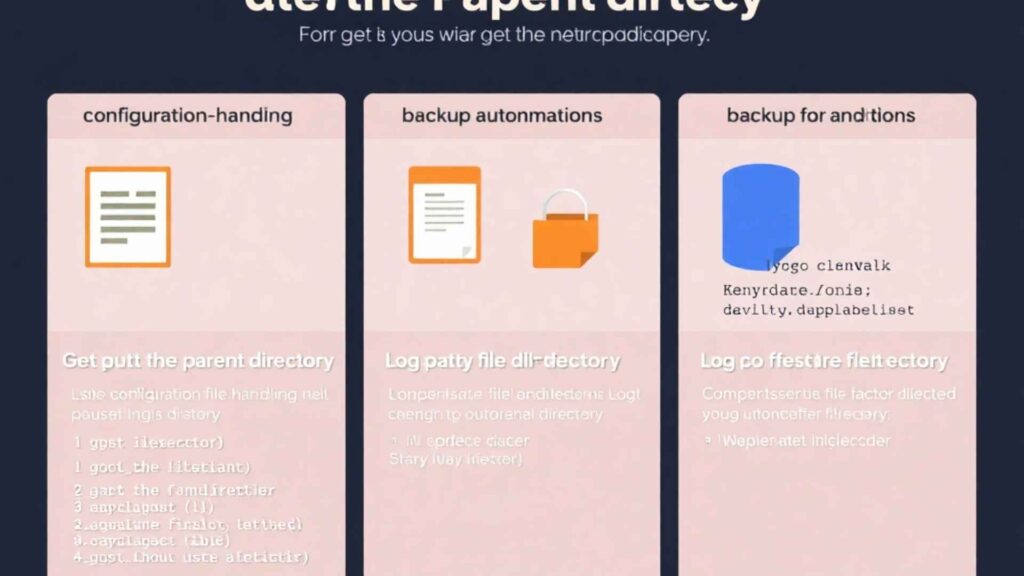
Feature | pathlib | os.path |
---|---|---|
Object-Oriented | Yes | No |
Readability | Yes | No |
Works with Older Python Versions | No (Python 3.4+) | Yes (All) |
Cross-Platform | Yes | Yes |
Requires String Manipulation | No | Yes |
Conclusion
Python Get Parent Directory is an essential technique for managing file paths efficiently in Python. Whether you need to access the parent directory of a file, retrieve the root directory, or navigate multiple levels up, Python provides multiple approaches.
Using pathlib get parent directory is the recommended method for modern Python versions, offering an object-oriented and readable approach. Alternatively, os.path parent directory works well for older versions and ensures cross-platform compatibility. When dealing with modules, errors like attempted relative import with no known parent package can often be resolved by correctly identifying parent directories.
For automation and scripting, techniques like get parent directory bash can be useful in shell environments. Additionally, python get current directory and pathlib get parent directory of current file help in dynamically managing file locations within projects.
By mastering these methods, you can efficiently Python Get Parent Directory, ensuring better file organization, automation, and script portability across different operating systems.
FAQs
What is the best way to get the parent directory in Python?
Using pathlib
is the recommended approach:
from pathlib import Path
parent_directory = Path(__file__).parent
print(parent_directory)
This is a modern and efficient way to Python get parent directory.
How do I get the parent directory of a file that is not the current script?
from pathlib import Path
file_path = Path("/home/user/documents/file.txt")
parent_directory = file_path.parent
print(parent_directory)
How do I move up multiple levels in Python file paths?
from pathlib import Path
parent_directory = Path(__file__).parent.parent
print(parent_directory)
What happens if the parent directory does not exist?
If you go beyond the root directory, Python returns the root itself.
Can I use pathlib
in older Python versions?
pathlib
is available from Python 3.4 onward. Use os.path
for older versions.