When working with file operations in Python, it’s crucial to check if a file exists before performing any actions like reading, writing, or updating it.
This ensures that your program doesn’t encounter errors like trying to open a non-existent file or overwriting important data unintentionally.
In this blog post, we’ll explore various methods to check if a file exists in Python. Whether you’re working with local files, ZIP files, or directories, you’ll find a solution here.
Why Should You Check If a File Exists in Python?
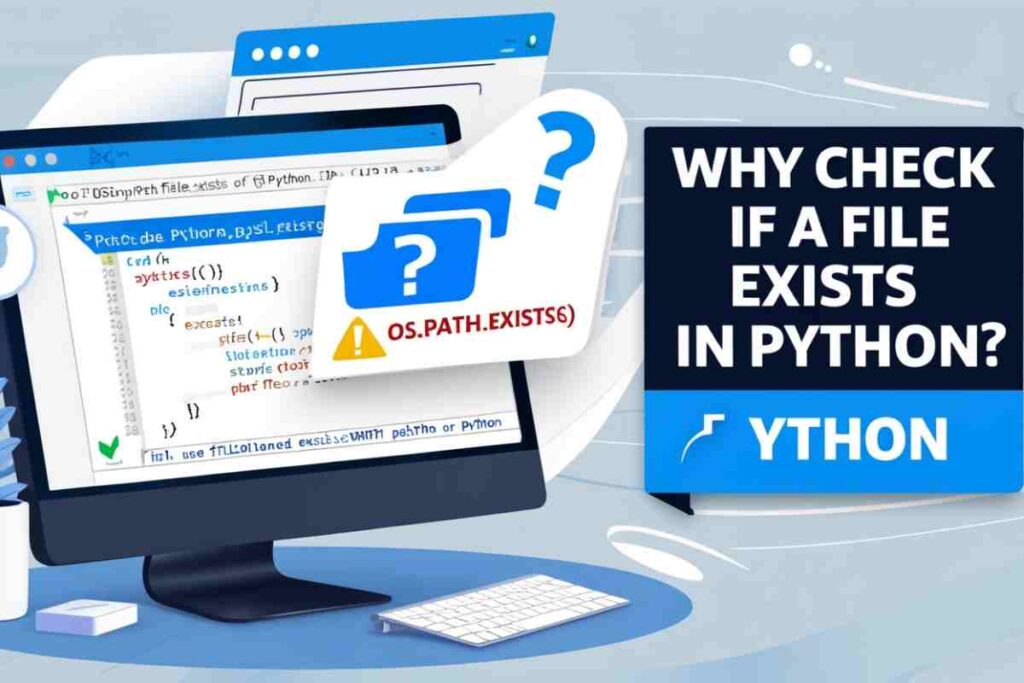
Handling files in Python can sometimes be error-prone, especially when you’re uncertain whether the file you’re trying to interact with already exists, leading to potential bugs and crashes in your program.
Without checking first, you might end up with errors like FileNotFoundError
or PermissionError
. By checking if the file exists before performing operations like reading or writing, you can avoid unexpected program crashes and handle the situation smoothly.
Python Check If File Exists: Methods You Can Use
Python provides a few ways to check if a file exists. Let’s explore the most commonly used methods:
Using os.path.exists()
to Check If a File Exists
The os.path.exists()
method is one of the simplest ways to check if a file exists in Python. This method returns True
if the file or directory exists and False
otherwise.
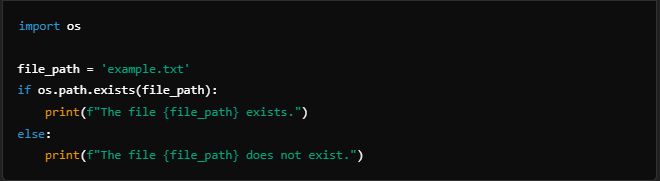
Using os.path.isfile()
to Check if a File Exists
While os.path.exists()
checks if a path exists (it could be a file or a directory), the os.path.isfile()
method specifically checks if a file exists. This can be useful when you need to make sure the path is not a directory.
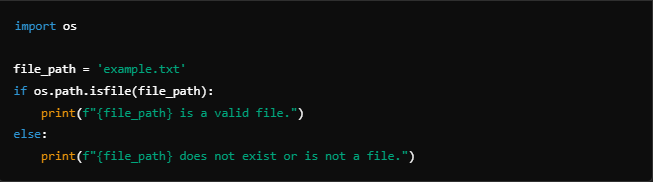
Using os.access()
to Check File Access Permissions
Sometimes, even if a file exists, you may want to check if you have read or write permissions for it. You can use the os.access()
function to check if the file exists and if it’s accessible.
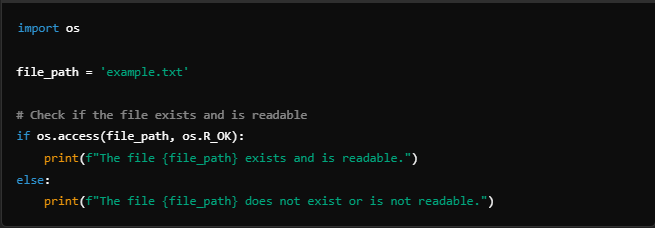
Using try-except
Block to Check If a File Exists
Another way to check if a file exists is by using a try-except
block. This method tries to open the file and catches any exception that may arise if the file doesn’t exist. It’s often used when you need to handle errors gracefully.
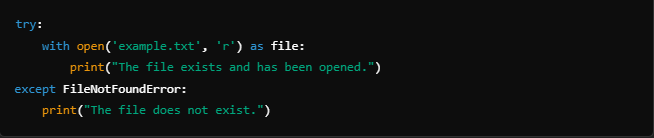
This approach is handy for situations where you might want to handle the error differently, for instance, by creating the file if it doesn’t exist.
Python Check If File Exists and Create If Not
If you want to check if a file exists and create it if it doesn’t, you can use a combination of the methods mentioned above and open()
in write mode. Here’s an example of how to do this:
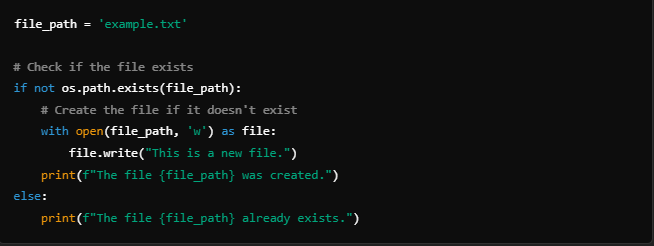
This method ensures that if the file doesn’t exist, it will be created, and if it does, you won’t accidentally overwrite the existing data.
Python Check If File Exists in ZIP File
Sometimes, you may need to check if a file exists within a ZIP archive. You can use the zipfile
module to interact with ZIP files in Python. Here’s how you can check if a file exists inside a ZIP archive:
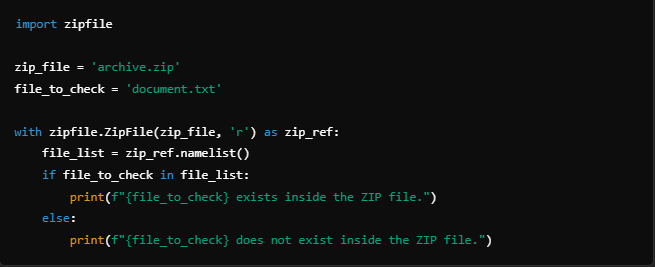
This code will check if the file document.txt
exists in the ZIP archive archive.zip
and print the appropriate message.
Python Check If Directory Exists
In addition to checking for files, you might need to check if a directory exists. Python’s os.path.isdir()
method allows you to check whether a directory exists at a specific path.
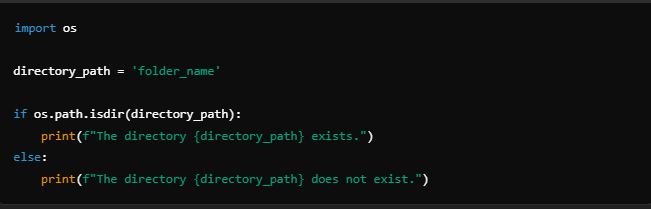
Checking directories before performing operations like file creation or deletion is an essential step to prevent errors.
Best Practices for File Existence Checks
- Always handle errors: While checking for file existence is a good practice, you should still handle potential errors in your code, especially if you plan to open or modify files.
- Use absolute paths: When checking if a file exists, it’s often better to use absolute file paths to avoid ambiguity.
- Log your checks: In a production environment, it’s useful to log file existence checks and any file manipulation activities. This helps with debugging and maintaining your code.
Conclusion
Checking if a file exists is crucial for file operations in Python. Whether it’s a file, directory, or file within a ZIP archive, Python provides simple methods like os.path.exists()
, os.path.isfile()
, and os.access()
, or you can use a try-except
block for error handling.
Python also lets you easily check if a file exists and create it if needed. With support for ZIP files and directories, Python offers flexibility in managing files efficiently.
FAQs
How do I check if a file exists in Python?
You can use os.path.exists()
or os.path.isfile()
to check if a file exists in Python.
Can I check if a directory exists in Python?
Yes, you can use os.path.isdir()
to check if a directory exists.
How can I check if a file exists and create it if not?
Use os.path.exists()
to check, and if the file doesn’t exist, create it with open()
in write mode.
How do I handle errors when checking if a file exists?
Use a try-except
block to catch errors like FileNotFoundError
when opening files.
Can I check if a file exists inside a ZIP archive?
Yes, use the zipfile
module and namelist()
to check for files inside a ZIP archive.
How do I check file access permissions in Python?
Use os.access()
with flags like os.R_OK
for read and os.W_OK
for write permission checks.
Is there a method to check if a file exists and is a valid file?
Yes, use os.path.isfile()
to check if a path exists and is a file.
Can I check if a file exists before reading it?
Yes, you can use os.path.exists()
or os.path.isfile()
before attempting to read a file.