In JavaScript, the location.reload(true)
method is commonly used to reload a webpage. This function can be a vital tool for developers, especially when you need to refresh the content dynamically.
In this post, we’ll dive deep into the mechanics of location.reload(true)
, its syntax, usage, potential issues, best practices, and offer practical examples.
What is JavaScript:location.reload(true)
?
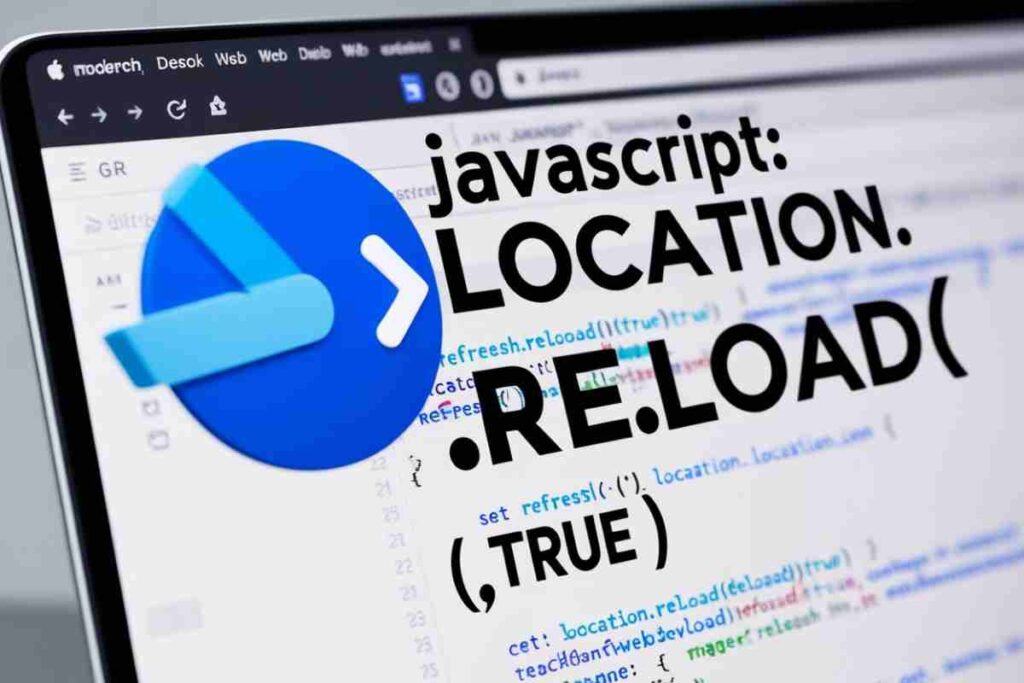
The location.reload(true)
function in JavaScript allows you to reload the current page. It’s often used to refresh the page, especially in situations where the content needs to be updated or re-fetched, such as after a user performs some action or an event occurs on the page.
While location.reload()
can be called without arguments, passing true
as an argument instructs the browser to fetch the page from the server, bypassing the cache.
This is helpful when you want to ensure that the page reloads with the latest version from the server, as opposed to using the browser’s cached version.
Syntax of location.reload(true)
:

The true
argument in this context tells the browser to force a reload from the server. Without it, the browser reloads the page from its cache, which can be faster but may not always show the latest changes.
How location.reload(true)
Works
In JavaScript, when we invoke window.location.reload(true)
, it triggers a page reload by requesting a fresh copy from the server.
This is essential when you need to bypass the browser cache and ensure that the page is updated with the most recent changes from the server.
It is important to note that the true
argument is no longer widely supported in modern browsers, and the method may ignore it or cause unintended behavior.
As a result, developers need to be aware of these changes and adapt their code accordingly.
Example of JavaScript location.reload(true)
:
Here’s a simple example:

In this example, when the reloadPage()
function is triggered, the current page reloads, bypassing the cache.
Why Use location.reload(true)
?
While reloading a page using JavaScript might seem like a simple action, there are several reasons why developers opt to use location.reload(true)
:
- Fetching Fresh Content: When you need to reload a page to retrieve fresh data or to reflect changes made in real-time.
- Bypassing Cache: Sometimes, a page’s content might not be up to date due to caching, and you want to ensure the content is loaded from the server instead of relying on the cached version.
- Dynamic Web Applications: For web applications that frequently update, such as dashboards or real-time apps, reloading the page can ensure that the user is always viewing the most current version.
Common Issues with location.reload(true)
location.reload(true)
Not Working
While location.reload(true)
might seem like the perfect solution to force a fresh reload from the server, there are some caveats:
- Deprecation: In recent years, the
true
argument passed tolocation.reload()
has been deprecated by modern browsers. As a result, callinglocation.reload(true)
might not work as expected, and the page may just reload from the cache. - Caching Problems: Even without the
true
argument, some browsers may still reload the page from the cache if certain caching settings are not configured correctly on the server. - No Refresh: Another common problem is that the browser simply doesn’t refresh the page. This could happen due to script errors, JavaScript restrictions in browsers, or security settings that prevent the reload from happening.
window.location.reload(true)
Deprecated
As mentioned, the true
argument in window.location.reload(true)
has been deprecated. Most modern browsers no longer respect this argument, meaning the page will reload from cache, even if you pass true
.
Instead, developers are advised to use location.reload()
without the true
argument and instead control cache behavior via server-side HTTP headers like Cache-Control
or Pragma
.
To prevent this issue, you can also implement a more robust caching strategy on your web server.
Troubleshooting location.reload(true)
:
- Check Cache-Control Settings: Ensure your web server is sending the correct cache control headers to prevent unwanted caching of resources.
- Use Fallback Solutions: If
location.reload(true)
doesn’t work, consider using a combination oflocation.href
and URL parameters to force a full reload with fresh content. - Browser Compatibility: Test your code across different browsers to ensure compatibility, especially if you’re working with legacy systems or older browsers.
Alternative Methods to Reload a Page in JavaScript
Although location.reload(true)
has its use cases, it’s no longer the go-to solution in modern JavaScript development due to the deprecation of the true
argument.
Let’s explore alternative ways to refresh or reload a page.
Using location.reload()
:
Instead of passing true
to the reload()
method, simply use:

This will reload the page, but it might still load from cache unless the server explicitly tells the browser to ignore the cache.
Using window.location.href
:
Another way to reload a page is by manipulating the href
property of the window.location
object:

This approach will reload the page and can bypass the cache depending on the cache-control headers set on the server.
Using location.replace()
:
You can also use location.replace()
to replace the current page with a fresh version:

This method navigates to the same URL, effectively refreshing the page.
Reloading Specific Elements on the Page
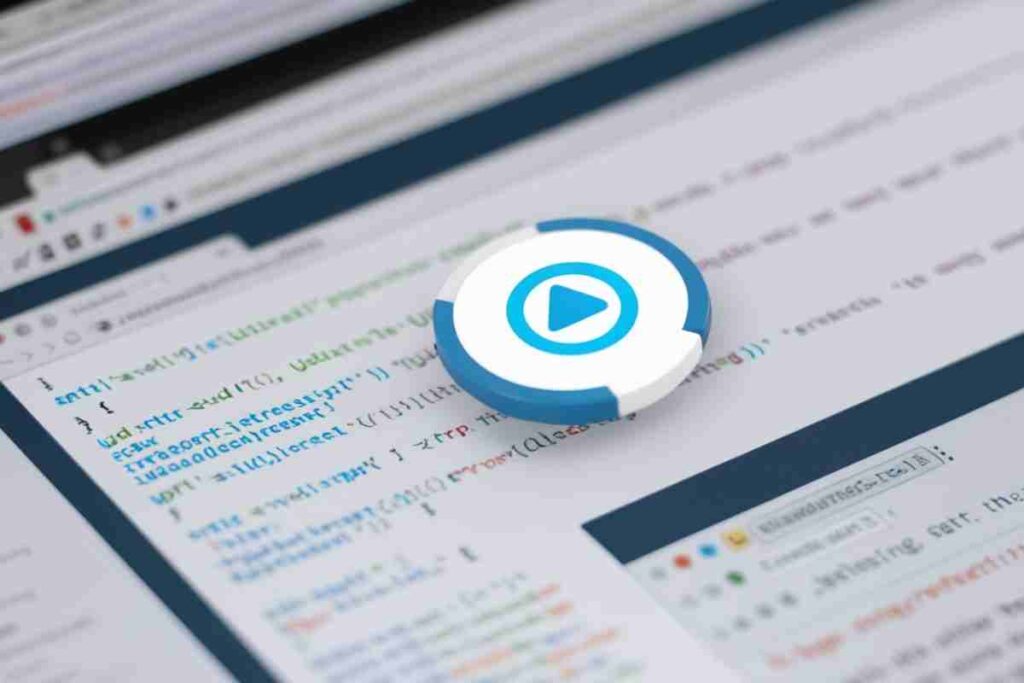
Sometimes, you may not want to reload the entire page but only a specific element. JavaScript provides various ways to refresh an individual element without refreshing the entire page.
JavaScript Refresh Element Example:
Here’s an example where we refresh a specific section of the page

In this case, only the element with the ID elementToRefresh
is updated with new content, avoiding a full page reload.
Using AJAX for Dynamic Content:
Another common solution is to use AJAX to load new content into a specific element, ensuring a smooth user experience without reloading the entire page.
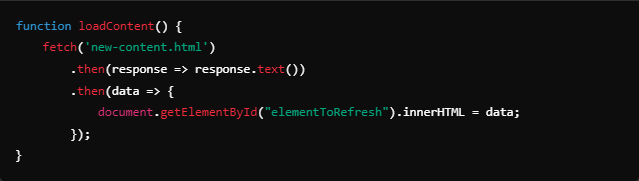
This method is highly effective for dynamic web applications where content changes frequently.
Conclusion
In conclusion, location.reload(true)
is a useful tool for reloading a webpage while bypassing the cache, though modern browsers have deprecated the true
argument.
Developers should be aware of these changes and explore alternative methods like location.reload()
or AJAX for more dynamic content updates.
By understanding caching behavior and using the right techniques, you can effectively manage page refreshes in your web applications.
FAQs
What is location.reload(true)
used for?
It reloads the page from the server, bypassing the browser’s cache, to ensure fresh content is loaded.
Is location.reload(true)
still supported?
No, modern browsers no longer support the true
argument, making it less effective.
How do I reload a page without using true
?
Simply use location.reload()
to reload the page, though it may still load from the cache.
Why isn’t location.reload(true)
working?
The true
argument has been deprecated in most browsers, so it may no longer function as expected.
Can I refresh specific elements on the page?
Yes, you can refresh specific elements using JavaScript, such as by modifying their innerHTML or using AJAX.
What are alternatives to location.reload(true)
?
You can use location.href = location.href
or location.replace(location.href)
for a full page reload.
How do I handle cache issues with page reloads?
Set proper cache-control headers on your server or use AJAX to avoid full page reloads.
What’s the difference between reload()
and location.href
?
reload()
reloads the page, while location.href
can be used to navigate or refresh by reassigning the current URL.