The “Invalid literal for int() with base 10” error is a common issue in Python when trying to convert a string to an integer. It occurs when the string doesn’t represent a valid number.
In this blog, we’ll explore what causes this error and how to fix it in Python, SQLAlchemy, and Pandas. If you’re wondering, “What does invalid literal for int() with base 10 mean in Python?”, keep reading to find the answers!
What Does “Invalid Literal for int() with Base 10” Mean?
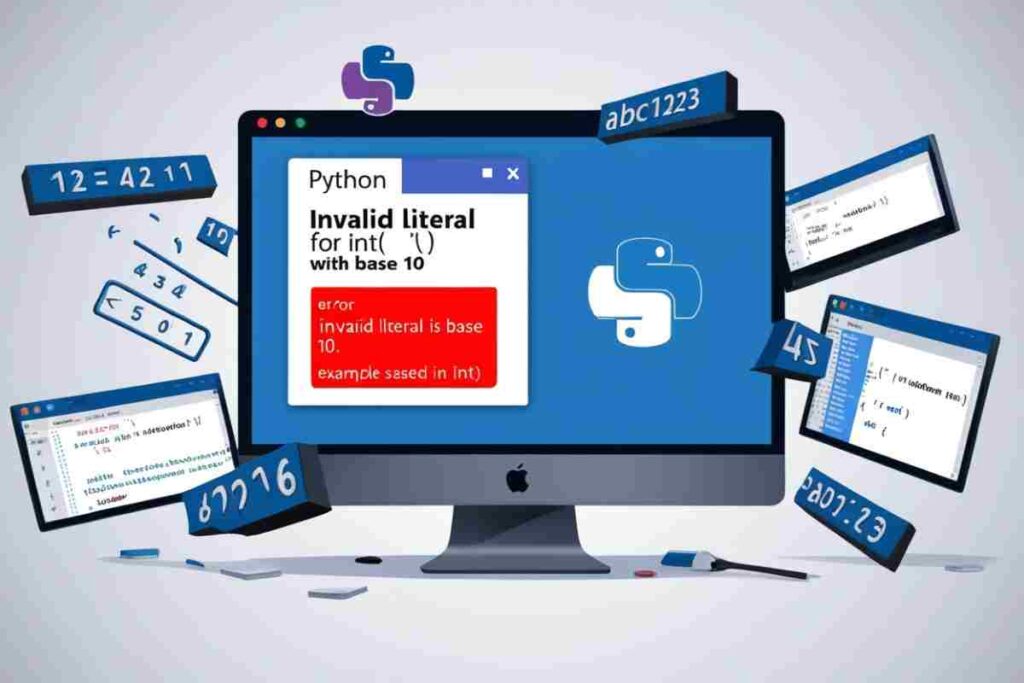
The error message “Invalid literal for int() with base 10” is a ValueError
that arises when attempting to convert a string to an integer using the int()
function in Python.
The int()
function is designed to convert a given string (if the string represents a valid integer) to its corresponding integer value. However, when the string cannot be parsed as an integer, Python raises a ValueError
, accompanied by the message “Invalid literal for int() with base 10.”
The term “base 10” refers to the decimal number system, which is the standard numerical system that humans use in everyday life. This means that Python expects the string to consist of only valid digits (0-9) in base 10.
Common Causes of the “Invalid Literal for int() with Base 10” Error
- Non-numeric Strings: When the string you are trying to convert contains characters other than digits (such as letters or special symbols), Python will throw the
Invalid literal for int() with base 10
error. For example:pythonCopy code

- Empty Strings: An empty string cannot be converted to an integer either.pythonCopy code

- Whitespace: If the string contains whitespace characters, Python may not be able to interpret it as a valid integer.pythonCopy code

- Incorrect Formatting: If the string has commas or other formatting that isn’t compatible with integers, Python will also raise the error.pythonCopy code

Solving the “Invalid Literal for int() with Base 10” Error
Now that we understand the causes of this error, let’s look at how to fix it in various situations.
Using try-except
for Safe Conversion
One way to handle this error gracefully is by using a try-except
block. This allows you to catch the ValueError
and handle it without crashing your program.

This approach helps prevent your program from breaking when it encounters an invalid string.
Cleaning Up the Input String
Before attempting to convert a string to an integer, you can clean up the string by removing any unwanted characters, such as commas or spaces. For example:

The strip()
method removes leading and trailing whitespace, while the replace()
method removes commas.
Validating the Input
If you expect the input to always be a valid integer, you can validate it before attempting conversion. One way to do this is by using the isdigit()
method:
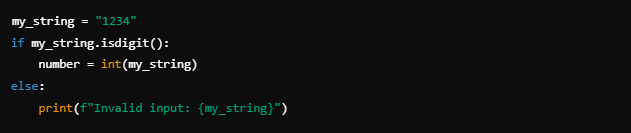
However, keep in mind that isdigit()
only works for positive integers and does not account for decimal points or negative signs.
The “Invalid Literal for int() with Base 10” Error in SQLAlchemy
When working with databases and SQLAlchemy, you may come across the Invalid literal for int() with base 10
error when you attempt to insert data or convert a value from your database to an integer.
For example, this error can arise when you’re working with SQLAlchemy and trying to insert data that isn’t a valid integer. This might happen if you’re working with user inputs or malformed data.
To solve this, ensure that any data you insert or retrieve from the database is properly sanitized and formatted before attempting conversion to an integer.
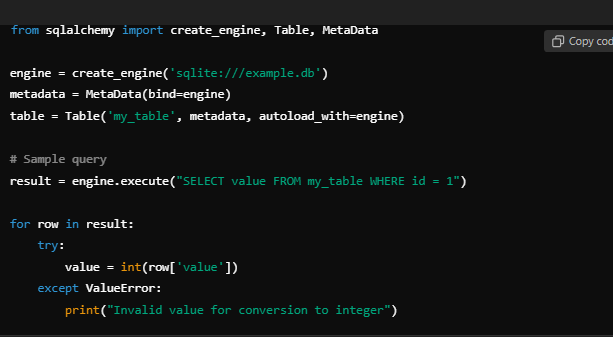
In this example, the program will catch the ValueError
and allow you to handle the issue without crashing.
Handling “Invalid Literal for int() with Base 10” in Pandas
If you’re using Pandas to read data from Excel or other file formats, you might encounter the Invalid literal for int() with base 10
error. This error often occurs when trying to convert columns that contain non-numeric data into integers.
For example, when you attempt to read an Excel file using pd.read_excel()
and convert a column to integers, you may get the error if the column contains non-numeric characters.
Example of Error in Pandas
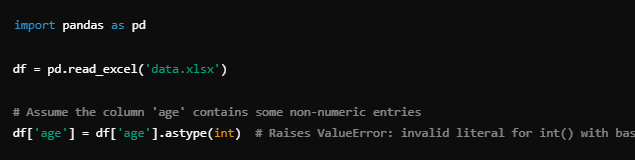
Solution: Clean and Convert Data
To handle this, you can clean your data first by removing or replacing non-numeric values before conversion:

This will replace any non-numeric values with NaN
. You can then use fillna()
to handle these NaN
values or drop them if necessary:

Conclusion
The “Invalid literal for int() with base 10” error is a common issue in Python, especially when working with strings that should be converted to integers.
By understanding its causes and implementing appropriate error handling and data sanitization techniques, you can avoid this problem in your code.
Whether you’re using plain Python, working with SQLAlchemy, or reading data with Pandas, it’s important to ensure that the input is clean and in the expected format.
FAQs
What is the “Invalid literal for int() with base 10” error in Python?
This error occurs when Python tries to convert a string to an integer, but the string contains non-numeric characters or is improperly formatted.
How can I fix the “Invalid literal for int() with base 10” error?
Ensure the string contains only numeric characters. You can clean or validate the string before converting it to an integer.
Does this error happen with non-numeric characters in the string?
Yes, any non-numeric characters like letters, symbols, or spaces in the string will cause this error.
Can I use int()
to convert decimals or float strings?
No, int()
cannot convert strings with decimals or float values. You need to use float()
first if you want to work with floating-point numbers.
How can I handle this error gracefully in Python?
You can use a try-except
block to catch the ValueError
and handle the error without stopping your program.
Can I fix this error in SQLAlchemy?
Yes, ensure any data being inserted into the database is properly formatted as integers. Validate inputs before attempting the conversion.
How does this error occur in Pandas?
It happens when you try to convert a column to integers, but the column contains non-numeric values like text or symbols.
How can I avoid the error in Pandas?
Use pd.to_numeric()
with the errors='coerce'
option to convert non-numeric values to NaN, then handle them as needed.