Python is a versatile programming language, and one of its key constructs is the condition if __name__ == '__main__':
. Whether you’re a beginner or an experienced developer, understanding this phrase is vital.
In this blog, we’ll break down the meaning, purpose, and practical applications of if name == main in Python.
What is If Name == Main?
At its core, the phrase if __name__ == '__main__':
is Python’s way of defining the entry point of a program. But what does this mean? Let’s unpack it.
Python scripts can either be executed directly or imported as modules. When you run a script directly, Python assigns the special variable __name__
the value '__main__'
.
This allows developers to write code that behaves differently depending on how it’s executed.
For instance:
- Code inside
if __name__ == '__main__':
runs only when the script is executed directly. - When the script is imported into another module, this block of code is skipped.
This feature makes Python scripts modular and reusable, offering flexibility in building scalable applications.
Why is If Name == Main Important?
Understanding if __name__ == '__main__'
is essential for writing Python code that is both clean and maintainable. Here are some benefits:
- Prevents Unintended Execution:
Without this condition, code in a module might execute during import, leading to errors or unexpected behavior. - Improves Code Reusability:
Functions and classes in a script can be imported elsewhere without running unnecessary code. - Clear Program Entry Point:
Similar to main functions in languages like C++ or Java, this construct signals where program execution begins.
For more on its significance, check out Python’s official documentation.
How If Nambe == Main Works in Python
When a Python file is executed, the interpreter assigns '__main__'
to the variable __name__
. Conversely, when the file is imported as a module, __name__
gets the module’s name instead.
Here’s a simple example:
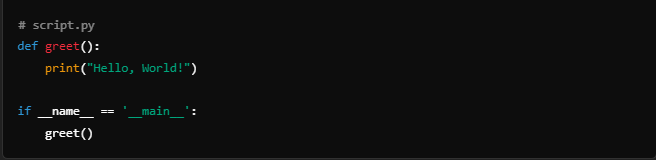
If executed directly:

If imported:

Common Use Cases for If Name == Main
Testing Functions or Modules
Developers often use this construct to test functions within the same script without affecting its behavior when imported.
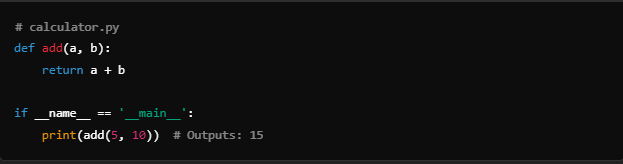
Command-Line Tools
Scripts intended for command-line use rely on this condition to parse input arguments or perform tasks.
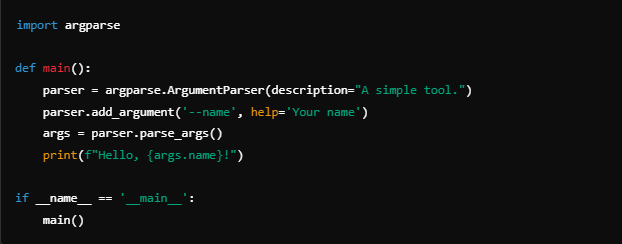
Unit Testing Frameworks
Test scripts often leverage if __name__ == '__main__'
to execute test cases selectively.
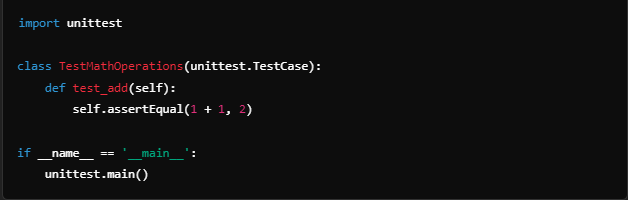
Troubleshooting If Name == Main
If name == ‘main’ Not Working
If your code isn’t running as expected, check the following:
- File Structure: Ensure the script is not unintentionally imported elsewhere.
- Syntax Errors: Double-check for typos in the condition or elsewhere in the code.
- Execution Method: Confirm you’re running the script directly and not through an IDE that alters behavior.
Debugging Tips
Add print statements to verify the value of __name__
:

If Name == Main Example in Practice
To solidify the concept, let’s explore a more complex scenario. Consider a web scraping script:
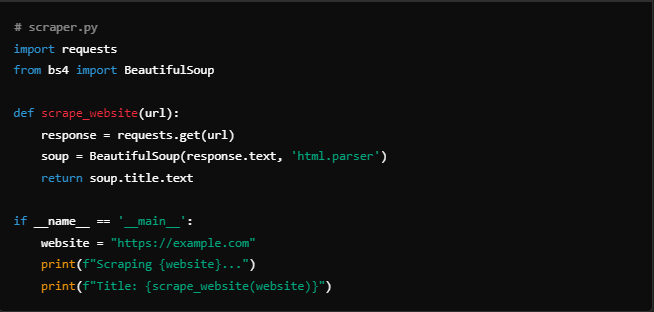
Here:
- The scraping logic is reusable when imported.
- Execution logic is contained within
if __name__ == '__main__':
, ensuring it doesn’t interfere with other scripts.
Extended Guide: If Name == Main in Python
Python’s if __name__ == '__main__'
is more than a programming convention—it’s a critical construct for creating reusable and modular scripts. Let’s expand on the topic with deeper insights, advanced examples, and broader context.
Understanding the __name__
Variable
In Python, every script or module has a built-in variable called __name__
. It serves as an identifier for the execution context. Here’s how it works:
- When a script is run directly,
__name__
is set to'__main__'
. - When a script is imported,
__name__
is set to the script’s filename (without the.py
extension).
This dual behavior allows Python to differentiate between the two scenarios and gives developers control over which parts of the code execute.
Example:

- Run directly:
__name__
is'__main__'
. - Imported:
__name__
reflects the module’s name.
Advanced Use Cases of If Name == Main
Building Modular Libraries
Developers often use if __name__ == '__main__'
to provide standalone functionality while keeping the script importable as a library.
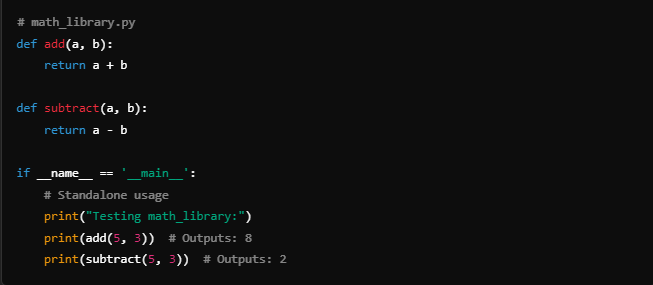
When imported, only the add
and subtract
functions are available without the test code running.
Managing Script Configurations
Command-line scripts often use this construct to handle configurations and arguments.
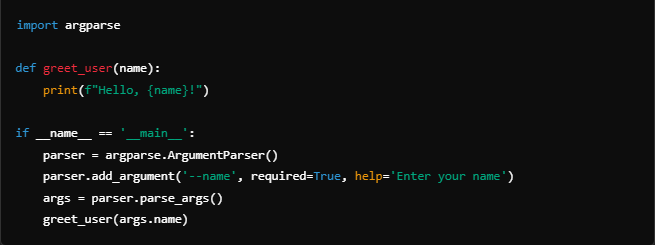
This ensures the script runs only with valid arguments, improving its robustness.
Comparisons to Other Programming Languages
Many programming languages have similar constructs to define the program’s entry point:
- C++/Java: The
main()
function serves as the entry point. - JavaScript: In Node.js, the
require.main === module
check works similarly.
Python’s approach is unique because it offers flexibility without enforcing a specific function name like main()
.
Tips for Using If Name == Main Effectively
- Group Related Code
Useif __name__ == '__main__'
to organize scripts better by grouping test cases, execution logic, or setup code. - Write Modular Code
Functions, classes, and reusable code should always be outside theif __name__ == '__main__'
block. - Use Logging for Debugging:
Replace print statements with Python’slogging
module to gain better control over output.
Conclusion
Understanding if __name__ == '__main__'
in Python is crucial for writing clean, modular, and reusable code. This construct allows scripts to define a clear entry point while preventing unintended execution when imported as a module.
Whether you’re debugging, structuring command-line tools, or building scalable applications, mastering __main__
in Python enhances your coding efficiency.
By implementing best practices—such as grouping related code, writing modular functions, and using logging—you can create well-structured Python scripts.
If you encounter issues with if __name__ == '__main__' not working
, check for syntax errors, execution methods, and file structures. For further learning, resources like W3Schools provide additional explanations and examples of if __name__ == '__main__'
meaning in Python
FAQs
What is if __name__ == '__main__'
in Python?
It is a conditional statement that ensures a script runs only when executed directly, not when imported as a module.
Why use if __name__ == '__main__'
?
It prevents unintended execution of code when a script is imported, making Python programs more modular and reusable.
How does if __name__ == '__main__'
work?
When a script runs, Python sets __name__
to '__main__'
, executing the code inside the block only if the script is run directly.
What happens if if __name__ == '__main__'
is removed?
Without it, all top-level code runs even when the script is imported, which can lead to unexpected behavior or errors.
Why is if __name__ == '__main__'
not working?
Check for syntax errors, ensure the script is executed directly, and verify that an IDE or environment isn’t altering execution.
Can if __name__ == '__main__'
be used in all Python scripts?
Yes, but it is mainly beneficial for scripts that may be imported as modules or contain executable code.
How is if __name__ == '__main__'
used in real-world applications?
It is commonly used in command-line tools, testing scripts, web applications, and modular libraries.
Is if __name__ == '__main__'
similar to main()
in other languages?
Yes, it serves a similar role to main()
in C++ and Java, defining where program execution starts in a script.