Are you encountering the dreaded “Expected string or bytes-like object” error in Python? You’re not alone! This common error often confuses beginners and experienced developers alike. But don’t worry it’s fixable!
In this guide, we’ll break down what causes this error, explore different scenarios where it occurs, and provide step-by-step solutions to resolve Expected String or Bytes-Like Object.
What Causes the “Expected String or Bytes-Like Object” Error?
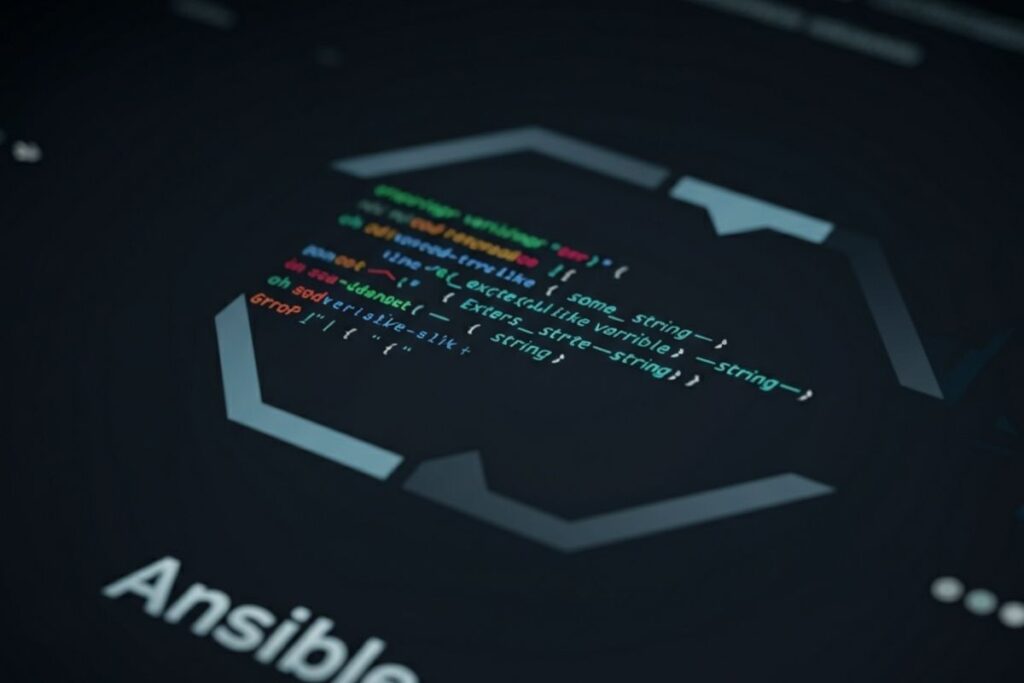
This error occurs when Python functions that expected string or Bytes-Like Object receive a different data type, such as an integer, list, dictionary, or NoneType.
Common Scenarios:
- Using
re.match()
orre.search()
with a non-string input. - Incorrect data types being passed to functions in libraries like Pandas, Django, or Boto3.
- Working with file handling and S3 storage in Ansible or AWS SDK (Boto3).
- Processing user inputs that are not validated.
- Working with API responses that return unexpected data types.
Fixing the “Expected String or Bytes-Like Object” Error
This error occurs when Python functions that expected string or bytes-like Object receive a different data type, such as an integer, list, dictionary, or NoneType.
Fixing the Error in Python’s re.match()
Regular expressions in Python require a string input. Passing an integer or None will result in this error
Problem:
import re
pattern = r"\d+"
number = 12345 # Integer instead of a string
match = re.match(pattern, number)
Solution: Convert the integer to a string before using re.match()
match = re.match(pattern, str(number))
Handling the Error in Boto3 (AWS SDK)
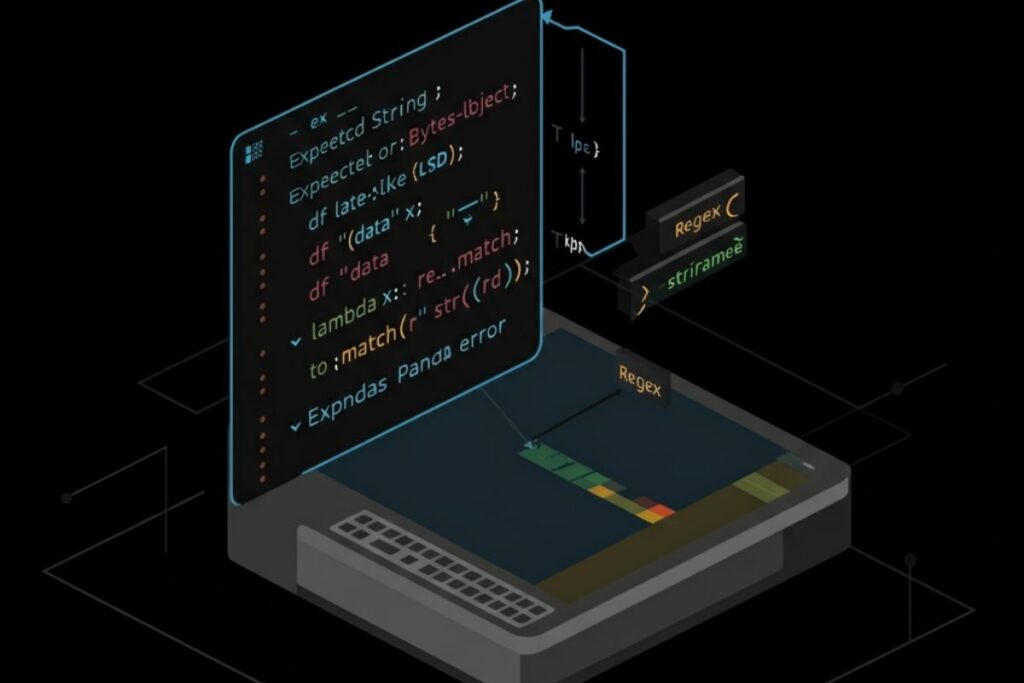
AWS services require string inputs for parameters like Bucket names and File Keys. Passing incorrect types can lead to errors.
Problem: Passing None
or a non-string value to AWS functions.
import boto3
s3 = boto3.client('s3')
response = s3.get_object(Bucket='my-bucket', Key=None) # Key must be a string
Solution: Ensure the Key is a valid string.
key = 'my-file.txt'
response = s3.get_object(Bucket='my-bucket', Key=key)
Fixing the Error in Django Models
Django expects string inputs for CharFields and similar fields in models. Assigning None
can cause errors.
Problem: Assigning None
to a CharField that expects a string.
from django.db import models
class MyModel(models.Model):
name = models.CharField(max_length=100)
data = MyModel(name=None) # Expected a string, got None
Solution: Provide a default empty string instead of None
.
data = MyModel(name="")
Resolving the Issue in Pandas
Pandas operations that involve string methods require the column values to be of type str
.
Problem: Applying a regex operation on a non-string column.
import pandas as pd
import re
df = pd.DataFrame({'data': [123, 'hello', None]})
df['data'].apply(lambda x: re.match(r'\d+', x)) # Error
Solution: Convert non-string values to strings before applying regex.
df['data'].apply(lambda x: re.match(r'\d+', str(x)))
Preventing Errors in Ansible
Ansible tasks that involve text operations expect string inputs.
Problem: Passing a non-string value to a text-processing module.
- name: Check file content
lineinfile:
path: /etc/config
line: {{ some_variable }} # Error if some_variable is not a string
Solution: Ensure some_variable
is a string.
line: "{{ some_variable | string }}"
Handling Errors in S3 Storage (Python & AWS)
Incorrect data types in S3 file operations can lead to unexpected errors.
Problem: Providing a non-string Key
parameter.
s3.download_file('my-bucket', 123, 'localfile.txt')
Solution: Convert it to a string.
s3.download_file('my-bucket', str(123), 'localfile.txt')
Fixing the Error in Stack Overflow Discussions
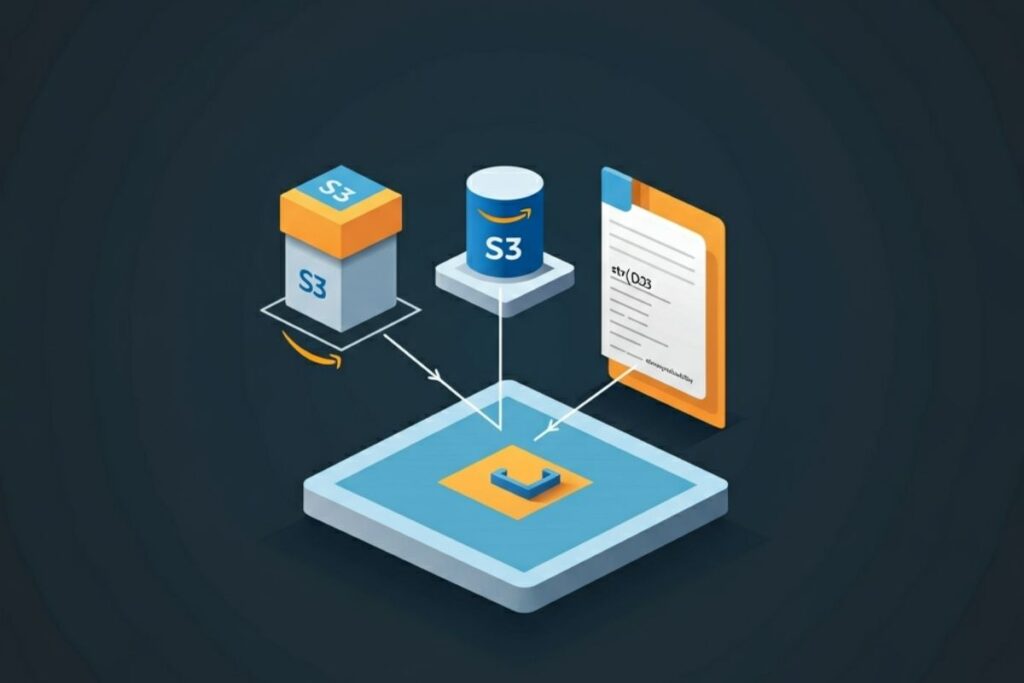
Many developers on Stack Overflow report Expected String or Bytes-Like Object error when working with web scraping libraries like BeautifulSoup or Scrapy. The issue often arises when extracting text from elements that return instead of a string.
Solution: Always check for None
before processing text.
if element.text is not None:
text = element.text.strip()
else:
text = ""
Conclusion
The “Expected String or Bytes-Like Object” error is common, but easily avoidable if you ensure that inputs to functions expecting strings are correctly formatted. Whether you’re working with Django, Boto3, or Pandas, the key takeaway is to always check and convert data types where necessary.
By following these solutions, you’ll be able to debug and fix this error effectively in your Python projects. Have questions? Drop them in the comments below!
(FAQs)
How do I fix “Expected String or Bytes-Like Object”?
Ensure that the input to functions expecting a string is indeed a string by using str(variable)
.
What is a bytes-like object?
A bytes-like object is any object that behaves like bytes
in Python, such as bytearray
or memoryview
.
How do you convert a bytes-like object to a string in Python?
Use .decode()
method, e.g., b'hello'.decode('utf-8')
.
How do you create a bytes-like object in Python?
Use bytes()
or bytearray()
, e.g., bytearray('hello', 'utf-8')
.
How do you fix an “object object” error in HTML?
Ensure you are not displaying an object directly; convert it to a string using .toString()
in JavaScript.
What is the expected string error message?
It usually indicates that a function expected a string but received another data type, such as an integer or None
.
How to fix “a bytes-like object is required, not str”?
Convert the string to bytes using .encode()
, e.g., b = 'hello'.encode('utf-8')
.
How to convert a string to bytes?
Use encode()
, e.g., b'hello'.encode('utf-8')
.
How many bytes are in a string?
It depends on the encoding; UTF-8 uses 1-4 bytes per character, while ASCII uses 1 byte per character.
How do I avoid type-related errors in Python?
Always validate and convert data types before passing them to functions, using isinstance()
checks.