When working with Git, you may need to Git pull overwrite local changes with the latest updates from the remote repository.
However, git pull by default tries to merge changes, which can lead to conflicts if your local files differ.
In this comprehensive guide, we’ll explore the safest ways to Git pull overwrite local changes, how to avoid conflicts, and expert Git management strategies.
Understanding Git Pull and Overwriting Local Changes
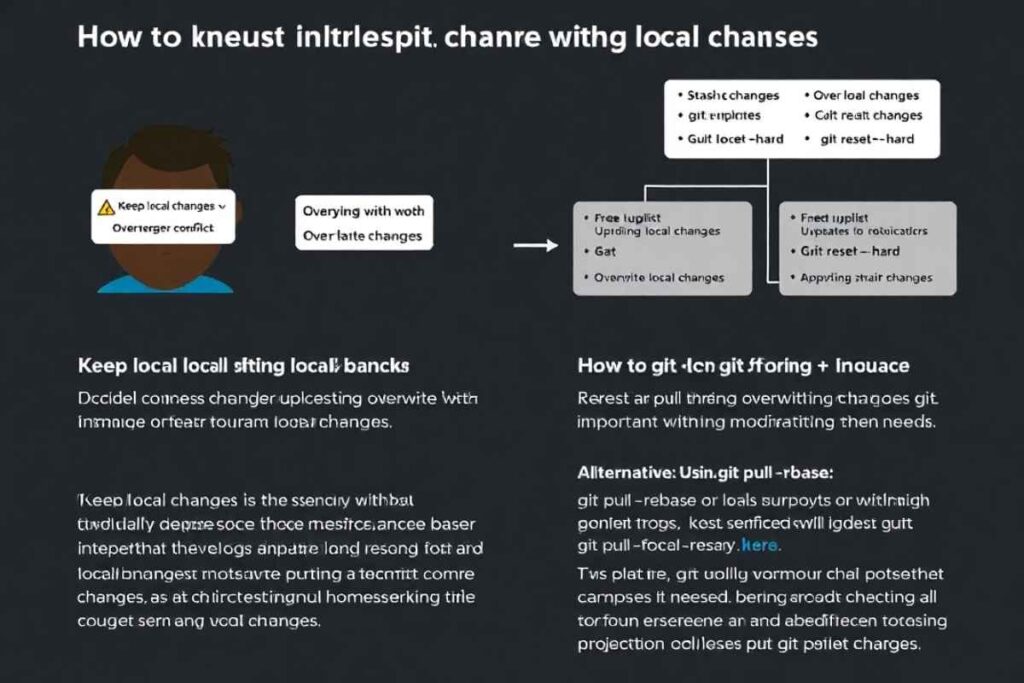
By default, git pull does not overwrite local changes. Instead, it merges the fetched changes from the remote branch into your local branch.
If there are conflicting changes, Git will pause and require you to resolve conflicts manually. This behavior ensures that local work is preserved, but in cases where you need to Git pull overwrite local changes, additional commands are required.
Why Would You Need to Overwrite Local Changes?
There are several scenarios where you might need to Git pull overwrite local files:
- Discarding unwanted local changes: If your local modifications are no longer needed.
- Ensuring consistency with the remote branch: When working in teams, you may need to synchronize your environment.
- Recovering from a merge conflict: If an automatic merge creates issues, resetting to the remote state can resolve them.
- Keeping a production environment clean: Ensuring that a deployed branch always matches the latest remote updates.
How to Git Pull and Overwrite Local Changes
Overwriting local changes with a Git pull requires careful execution to prevent unintentional loss of important data.
The following methods ensure a forceful update while keeping necessary modifications intact when needed.
Stash Local Changes (Recommended)
To prevent accidental data loss, stash your local changes before pulling:
git stash –include-untracked
This command temporarily saves all your uncommitted changes, including untracked files, allowing you to reapply them later if needed.
Forcefully Overwrite Local Changes
To Git pull overwrite local changes, run:
git fetch –all
git reset –hard origin/<branch-name>
Replace <branch-name> with the appropriate branch (e.g., main or develop). This command:
- Fetches the latest changes from the remote repository.
- Resets your local branch to match the remote branch, overwriting all local modifications.
Apply Stashed Changes (If Needed)
If you stashed your changes earlier and need to retrieve them, use:
git stash pop
This restores your previously saved modifications, allowing you to reapply them selectively.
How to Git Pull Without Overwriting Local Changes
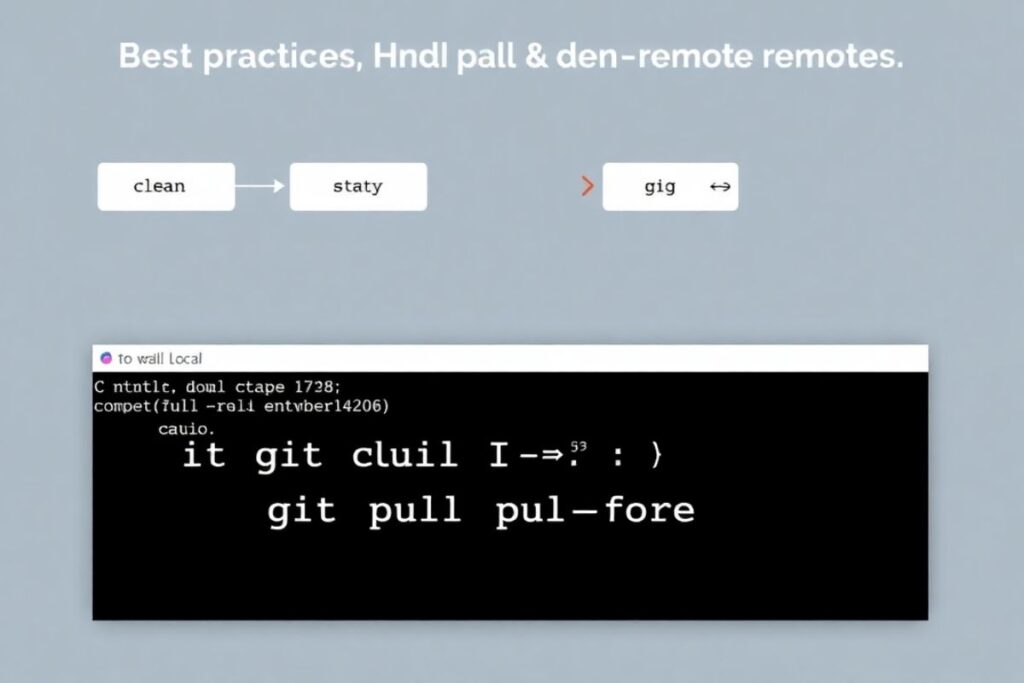
If you want to update your local branch without overwriting local changes, use:
git pull –rebase
This command applies the remote changes on top of your local commits instead of merging, preventing unnecessary conflicts.
Alternative: Using git pull –force
Although git pull –force is not a standard command, you can achieve the same effect with:
git fetch –all
git reset –hard HEAD
git pull origin <branch-name>
This method fetches remote changes and applies them forcefully to your local branch.
Advanced Techniques for Managing Local and Remote Changes
Mastering advanced Git techniques ensures smooth collaboration and prevents data loss. Below are essential strategies to manage local and remote changes effectively. These techniques help maintain a clean codebase while reducing the chances of merge conflicts.
Using git clean to Remove Untracked Files
If you want to remove untracked files before pulling, run:
git clean -fd
This command forcefully deletes untracked files and directories.
Checking for Differences Before Overwriting
To compare your local changes with the remote branch before forcing an update, use:
git diff origin/<branch-name>
This allows you to review changes before deciding to reset your branch.
Using git reflog to Recover Lost Changes
If you accidentally Git pull overwrite local changes, you can recover them using:
git reflog
Identify the commit hash you want to return to, then run:
git reset –hard <commit-hash>
Additional Best Practices for Git Management
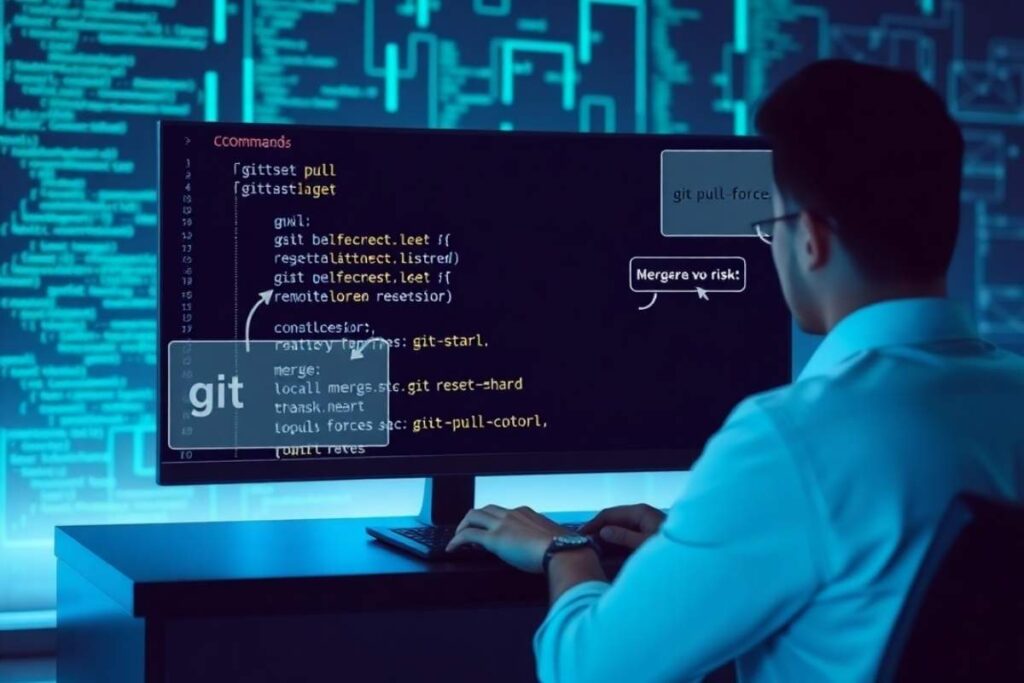
- Use Branching Properly: Always create feature branches instead of working on main.
- Enable Git Hooks: Automate checks to prevent accidental force pulls.
- Regularly Backup Work: Push your work frequently to remote repositories to avoid data loss.
Conclusion
Using Git pull overwrite local requires caution to avoid losing important work. The safest approach is to stash your changes before resetting your branch.
If you want to update your local branch without overwriting local changes, use git pull –rebase. Always double-check before running commands like git reset –hard to prevent accidental data loss.
By following these best practices, you can efficiently sync your local repository while ensuring data safety and avoiding merge conflicts.
Keep these methods in mind for a smooth Git workflow!With the right approach, you can confidently Git pull overwrite local changes when needed while keeping your workflow streamlined and
FAQs
How to Git Pull Overwrite Local Commits?
Use git reset --hard origin/<branch-name>
to discard local commits and sync with the remote branch.
How to Git Pull Force?
Git does not support git pull --force
, but you can achieve the same result with git fetch --all && git reset --hard origin/<branch-name>
.
How to Git Pull Force Overwrite?
Run git fetch --all && git reset --hard origin/<branch-name>
to forcefully overwrite local changes with remote updates.
How to Git Delete a Local Branch?
Use git branch -d <branch-name>
to delete a merged branch, or git branch -D <branch-name>
to force delete an unmerged branch.
How to Git Overwrite Local Branch with Remote?
Execute git fetch --all && git reset --hard origin/<branch-name>
to replace your local branch with the remote version.
What Causes “Your Local Changes Would Be Overwritten by Merge” Error?
This happens when local changes conflict with remote updates; resolve it by committing, stashing (git stash
), or discarding changes.
How to Git Pull Remote Branch?
Use git checkout <branch-name>
to switch to the branch, then run git pull origin <branch-name>
to fetch the latest changes.
How to Use Git Reset?
Run git reset --hard <commit-hash>
to revert your branch to a previous state, permanently discarding all local changes.