Understanding data types is a crucial aspect of programming in Python. Knowing how to check data type Python helps you write efficient, bug-free code.
This guide provides detailed insights on using Python’s built-in tools like the type() function to identify and manage data types efficiently and accurately.
What Does “Check Data Type Python” Mean?
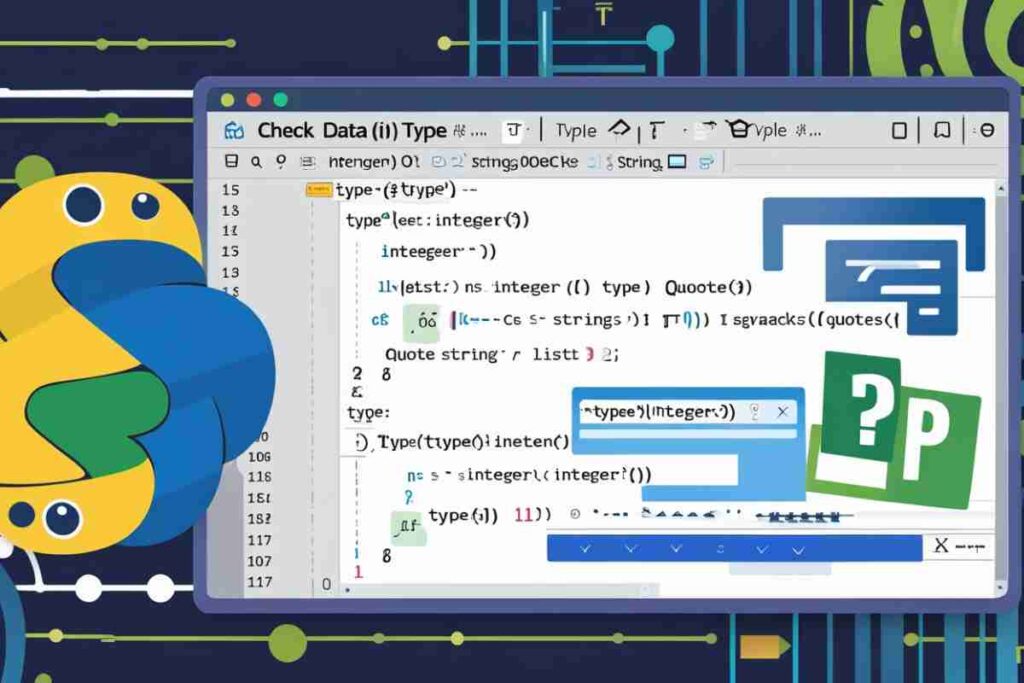
Data types define the nature of a value, such as whether it is an integer, string, or list. In Python, checking the type of a variable is straightforward with the help of Check data type Python methods, thanks to the language’s dynamic typing. This means variables can store different types of data without explicit declaration.
Why is Checking Data Types Important?
- Error Prevention: Ensures operations are performed on compatible types.
- Debugging: Helps identify issues caused by unexpected types.
- Code Maintenance: Makes code easier to read and maintain.
How to Check Data Type Python?
Using Python’s type()
Function
The most common method to check the data type of a variable is by using the type()
function.
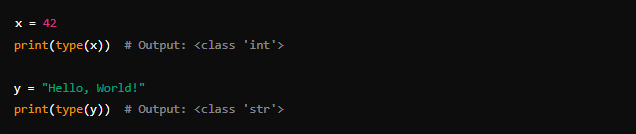
The type()
function returns the class type of the variable passed as an argument.
Checking Data Type in an If Condition
You can use type()
in if-else statements to make decisions based on data types.
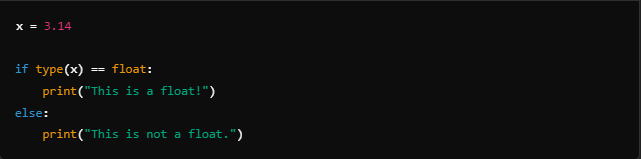
This method is helpful in scenarios where behavior changes based on the variable’s type.
Using isinstance()
for Type Checking
The isinstance()
function checks whether a variable belongs to a specific data type.

This method is preferred over type()
because it supports inheritance checks.
Working with Complex Data Types in Python
Strings and Lists
Checking whether a variable is a string or a list is common in Python.

Data Frames with Python Pandas
When working with Pandas, you may need to check the data type of DataFrame columns.

This method is crucial for cleaning and analyzing data in Python.
Checking Types in Numpy Arrays
Numpy arrays have their unique data type methods.

How to Check Data Type in Python w3schools Style?
W3Schools emphasizes simple and effective coding practices. A direct way to check the type of variable is:

Practical Scenarios for Checking Data Types
Input Validation
When taking user input, validating its type ensures smooth execution.
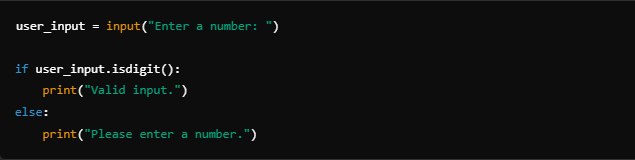
Type Conversion
Sometimes, you might want to convert types explicitly.

Common Errors When Checking Data Types
Direct Comparison of type()
Output:
Avoid comparing types with strings. Use type(variable)
or isinstance()
.
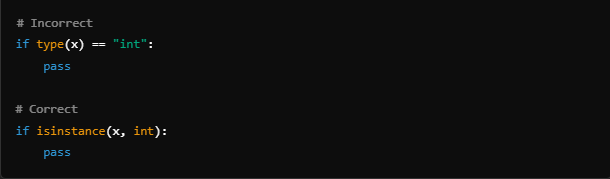
Overlooking Inheritance:
If your application involves custom classes, prefer isinstance()
over type()
.
Advanced Type Checking Techniques
Type Hints with Python
Python 3.5 introduced type hints, which help specify expected types in function arguments and return values.

Dynamic Type Checking
For advanced scenarios, the collections.abc
module provides more robust type-checking options.
Additional Details about Check Data Type Python
Check Data Type Python Built-in Functions
In addition to the type()
and isinstance()
functions, Python offers a few other useful methods for type checking.
isinstance()
vstype()
: Whiletype()
is used to check the exact class type of a variable,isinstance()
also checks for inheritance. This is useful when you want to confirm if an object is an instance of a class or any subclass.For example:
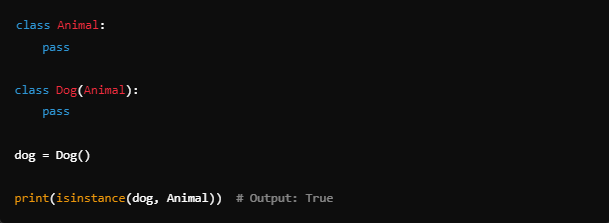
issubclass()
Function: Theissubclass()
function is used to check if a class is a subclass of another class, which can be useful when working with object-oriented code.

Practical Use Cases for Data Type Checking
- Error Handling with Type Checking: Check Data Type Python helps prevent runtime errors by ensuring you are working with the correct type. This is especially helpful when working with external data or user input. For example, ensuring a variable is a string before performing string operations:
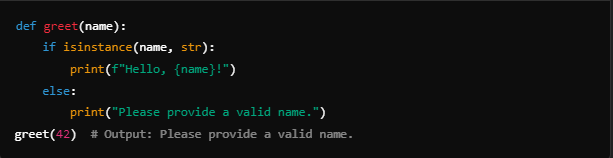
- Type Checking in Type Hinting (Python 3.5+):
- Python allows you to define expected data types in function signatures using type hints. This isn’t enforced during execution but helps in static analysis and with IDE tools.

- Dynamic Typing and Data Types in Python:
- Python is dynamically typed, meaning the type of a variable is determined at runtime. This allows you to use the same variable to store different types at different points in the code. For example:

While dynamic typing offers flexibility, it requires developers to be cautious and check types when necessary.
- Checking Data Types in Complex Data Structures (Lists, Dictionaries, etc.): When working with more complex data structures like lists and dictionaries, you might want to check the types of the individual elements or values.

This approach allows you to inspect mixed data types within a collection, which is particularly useful when processing data from user input or external sources.
Conclusion
Check Data Type Python is a fundamental skill every developer should master. From basic methods like the type()
function to advanced techniques involving Pandas and type hints, Python offers versatile tools for managing and identifying data types.
Use these techniques in your projects to ensure your code remains efficient, readable, and error-free. Explore additional resources like W3Schools and Python documentation to deepen your understanding.
FAQs
How do I check a variable’s data type in Python?
Use the type()
function: type(variable)
to check its data type.
What is the difference between type()
and isinstance()
?
type()
checks the exact type, while isinstance()
checks for inheritance.
Can type()
be used in an if statement?
Yes, you can check the type in an if
statement like if type(x) == int:
.
How do I check if a variable is a string?
Use isinstance(variable, str)
to check if it’s a string.
How do I check data types in Pandas DataFrames?
Use df.dtypes
to check the types of DataFrame columns.
Is it possible to Check Data Type Python of Numpy arrays?
Yes, use array.dtype
to check the type of elements in a Numpy array.
Can I check the data type of user input?
Yes, use type()
after converting input to a type, such as int(input())
.
How do I convert a string to an integer in Python?
Use int()
to convert a string to an integer: int("123")
.