When it comes to data transformation and object mapping, the C# map concept plays a crucial role in modern development.
While C# does not provide a built-in map()
function like some other languages, it offers versatile techniques to achieve similar results.
In this comprehensive guide, we’ll explore everything from the basics of mapping data in C# to advanced strategies using LINQ and dictionaries.
You’ll learn how to map arrays, lists, and even transform dictionaries, and understand the differences between mapping concepts and collections such as arrays and dictionaries
What is C# Map and How Does It Work?
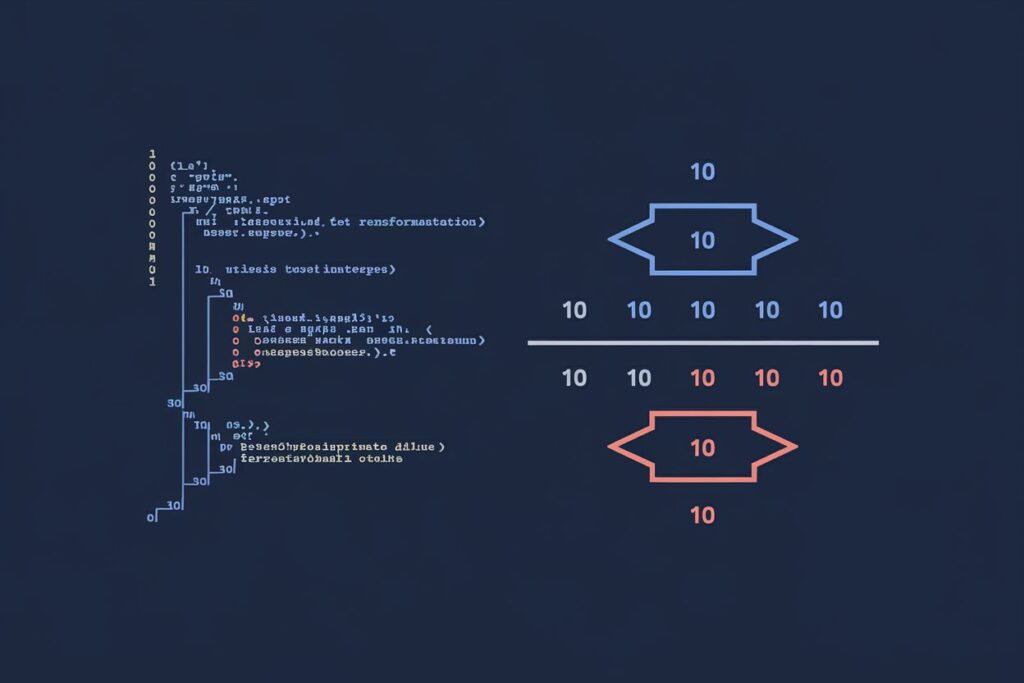
In C#, C# map typically refers to the process of converting data from one structure into another. Whether you’re transforming a list of values, mapping object properties, or creating a new representation of data for further processing, mapping is an essential operation.
C# Map Functionality with LINQ
While C# does not have a native map()
function, you can easily achieve mapping functionality using the LINQ Select()
method. This method transforms each element in a collection based on the provided lambda expression.
Example: Mapping an Array Using LINQ
csharpCopyEditusing System;
using System.Linq;
public class Program
{
public static void Main()
{
int[] numbers = { 1, 2, 3, 4, 5 };
var doubledNumbers = numbers.Select(n => n * 2).ToArray();
foreach (var num in doubledNumbers)
{
Console.WriteLine(num);
}
}
}
In this example, every element of the array is doubled, illustrating a straightforward approach to a C# map function using LINQ.
Understanding C# Dictionary: The Core of Mapping in C#
A C# Dictionary is a collection type that stores data as key-value pairs. It is extremely efficient for data retrieval when you have a unique key to access its associated value. The dictionary data structure is often used in mapping scenarios where quick lookups are required.
What is a Dictionary in C#?
A dictionary is part of the System.Collections.Generic
namespace. It provides methods to add, remove, and access elements based on keys, making it a fundamental tool for implementing C# map dictionary operations.
Example: Using a Dictionary in C#
csharpCopyEditusing System;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
Dictionary<string, string> capitals = new Dictionary<string, string>
{
{ "USA", "Washington D.C." },
{ "Canada", "Ottawa" },
{ "UK", "London" }
};
Console.WriteLine($"The capital of USA is {capitals["USA"]}");
}
}
This example clearly shows how a C# Dictionary can be used for key-value pair mapping and fast data lookup.
C# Map and Array: Key Differences
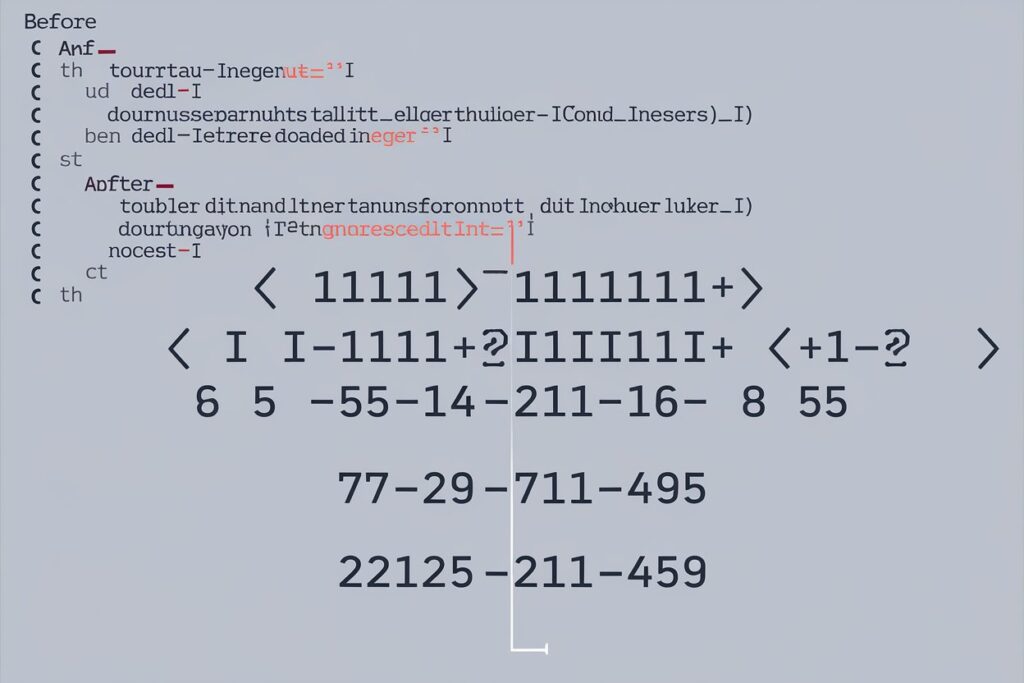
It is important to distinguish between C# map operations and using arrays for data storage. While both have their place in programming, they serve different purposes.
Arrays in C#
An array is a fixed-size, ordered collection of elements accessible by index. Arrays are ideal for sequential data storage but lack the flexibility of key-based access.
Map: A Transformation Concept
The C# map concept, on the other hand, often refers to transforming each element in a collection (such as an array or list) to a new form. This process is more dynamic and powerful when combined with LINQ, enabling complex data manipulation.
Example: Mapping a List with LINQ
csharpCopyEditusing System;
using System.Collections.Generic;
using System.Linq;
public class Program
{
public static void Main()
{
List<int> numbers = new List<int> { 1, 2, 3, 4, 5 };
var tripledNumbers = numbers.Select(n => n * 3).ToList();
tripledNumbers.ForEach(n => Console.WriteLine(n));
}
}
This example demonstrates the use of the C# map array and C# map list functionality to transform data within a list.
Advanced Mapping Techniques: C# Map LINQ and More
Transforming Dictionaries with LINQ
One powerful use case is mapping one dictionary to another with modified values. This is sometimes referred to as a C# map dictionary operation.
Example: Adjusting Employee Salaries
csharpCopyEditusing System;
using System.Linq;
using System.Collections.Generic;
public class Program
{
public static void Main()
{
var employeeSalaries = new Dictionary<string, double>
{
{ "Alice", 50000 },
{ "Bob", 60000 },
{ "Charlie", 70000 }
};
// Adjust salaries by 10%
var adjustedSalaries = employeeSalaries.ToDictionary(
e => e.Key,
e => e.Value * 1.1
);
foreach (var salary in adjustedSalaries)
{
Console.WriteLine($"{salary.Key}: ${salary.Value}");
}
}
}
This example shows how the C# map function can be applied to a dictionary to create a new mapping with updated values.
Mapping in Object-Oriented Programming (OOP
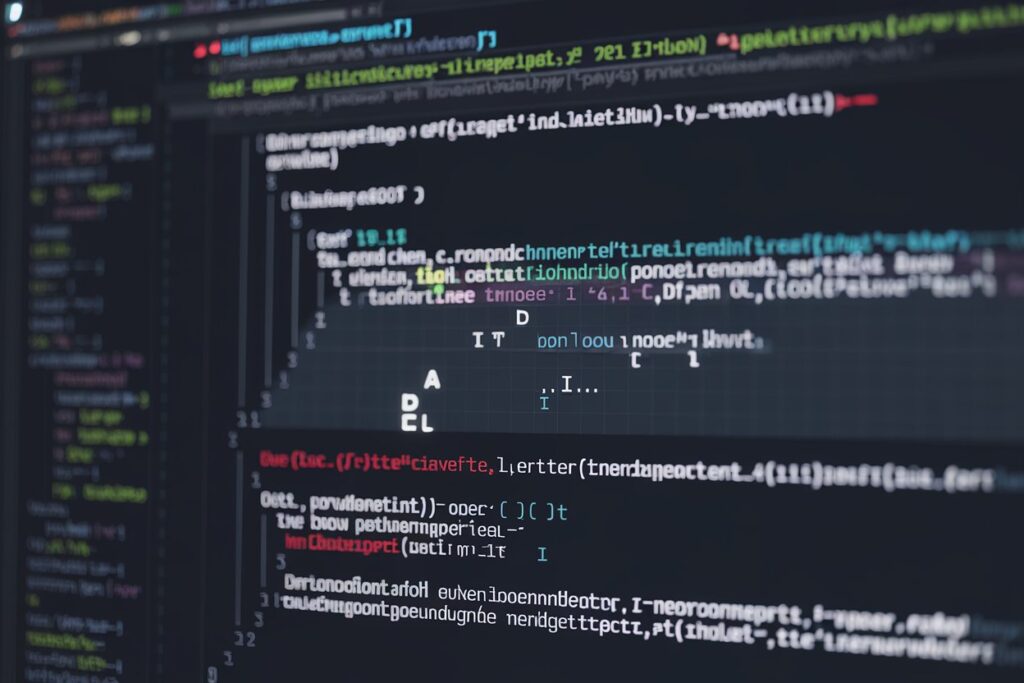
In OOP, a map often refers to data structures that store relationships between keys and values. This concept is widely used when designing classes and objects to maintain associations and perform transformations.
What is a Map in OOP?
A map in OOP can be any data structure that supports key-value associations, such as dictionaries or custom mapping classes. It is a fundamental concept when implementing design patterns like the Repository or Service Locator patterns.
Example: Mapping Object Properties
Consider two classes, where one is used to map data from another:
csharpCopyEditpublic class Source
{
public string Name { get; set; }
public int Age { get; set; }
}
public class Destination
{
public string Name { get; set; }
public int Age { get; set; }
// Constructor for mapping
public Destination(Source source)
{
this.Name = source.Name;
this.Age = source.Age;
}
}
Here, the C# map operation is used to transfer data between objects in a clear and maintainable way.
C# Map vs Dictionary: A Detailed Comparison
C# Map
- Conceptual Use: Refers to the process of transforming data.
- Implementation: Often realized using LINQ methods like
Select()
. - Flexibility: Can be applied to arrays, lists, and dictionaries.
- Usage Examples: Transforming arrays/lists, mapping object properties.
C# Dictionary
- Data Structure: A concrete implementation for storing key-value pairs.
- Access Speed: Provides O(1) lookup times.
- Usage: Ideal for scenarios requiring fast and efficient data retrieval.
- Usage Examples: Storing configuration settings, caching, or lookup tables.
Both concepts are integral to C# map vs Dictionary discussions, but they serve distinct purposes in data handling and transformation.
Internal and External Resources
For more in-depth tutorials on C# data structures and LINQ, check out our C# Tutorials section. Additionally, you can explore the official Microsoft documentation on C# programming for authoritative guidance.
Optimizing Data Transformation: Real-World Examples
Mapping Complex Data Structures
In real-world applications, you might need to map complex nested objects. For instance, consider a scenario where you have a list of orders, and each order contains a list of products. Using LINQ, you can flatten or transform these collections as needed.
csharpCopyEditusing System;
using System.Collections.Generic;
using System.Linq;
public class Order
{
public int OrderId { get; set; }
public List<Product> Products { get; set; }
}
public class Product
{
public string Name { get; set; }
public double Price { get; set; }
}
public class Program
{
public static void Main()
{
List<Order> orders = new List<Order>
{
new Order
{
OrderId = 1,
Products = new List<Product>
{
new Product { Name = "Laptop", Price = 999.99 },
new Product { Name = "Mouse", Price = 25.50 }
}
},
new Order
{
OrderId = 2,
Products = new List<Product>
{
new Product { Name = "Keyboard", Price = 45.00 },
new Product { Name = "Monitor", Price = 150.75 }
}
}
};
// Flatten all products from all orders
var allProducts = orders.SelectMany(o => o.Products)
.Select(p => new { p.Name, p.Price })
.ToList();
allProducts.ForEach(p => Console.WriteLine($"{p.Name}: ${p.Price}"));
}
}
This C# map list example uses LINQ’s SelectMany()
to map a list of orders to a flat list of products, demonstrating the flexibility of mapping complex data structures.
Best Practices for Effective C# Map Operations
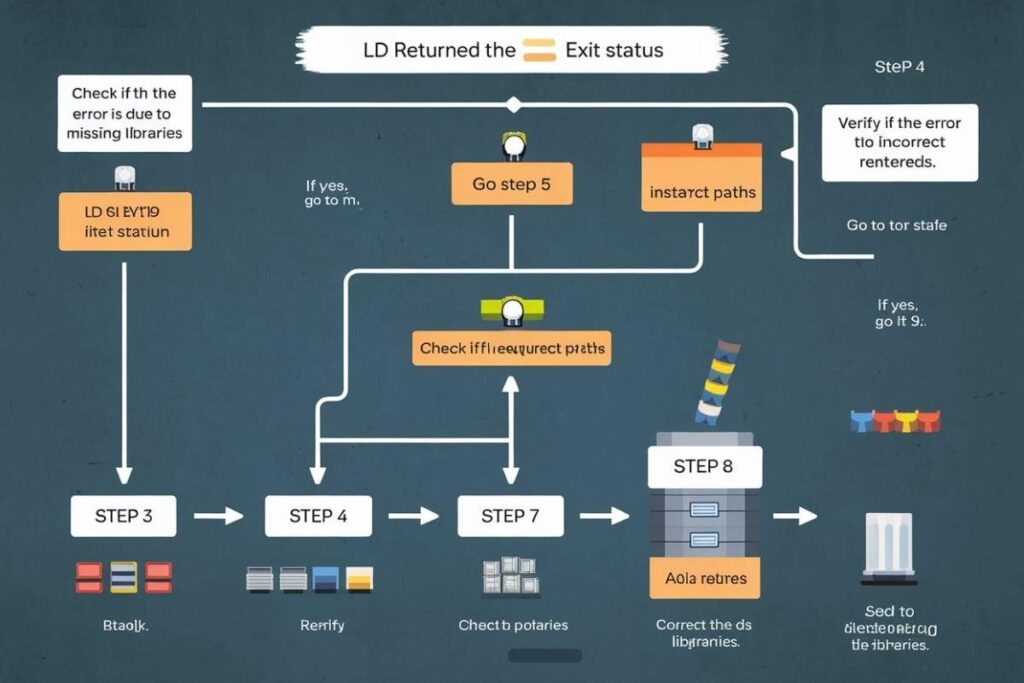
- Use LINQ for Readability:
LINQ’sSelect()
andSelectMany()
methods make your mapping operations concise and easy to understand. - Maintain Consistent Naming:
Whether mapping arrays, lists, or dictionaries, use clear variable names to represent your data transformations. - Optimize for Performance:
When dealing with large collections, consider performance implications. Dictionaries, for example, offer quick key-based lookups that can be leveraged during mapping. - Incorporate Error Handling:
Validate your data during the mapping process to avoid runtime exceptions, especially when dealing with nullable types or external data sources. - Document Your Code:
Clear documentation and code comments can help others understand your mapping logic, particularly in complex transformation scenarios.
For more tips on C# performance optimization, see our Performance Tips section.
Conclusion
The C# Map concept is essential for transforming and organizing data efficiently. While C# does not have a built-in map()
function like some other languages, LINQ’s Select()
method and dictionaries provide powerful mapping capabilities.
C# Map plays a crucial role in transforming and organizing data efficiently. While C# lacks a built-in map() function like C++, it leverages LINQ’s Select() method and dictionaries for powerful mapping capabilities.
Comparing C# Maps with C++ Maps highlights key differences in data structures, with C# using hash-based dictionaries for fast lookups.
Additionally, integrating C# with Google Maps API (maps.google.c) and using object-to-object mapping tools like AutoMapper enhances development efficiency.
Understanding these mapping techniques, including c-map, c maps, and c++ map, helps developers build optimized, scalable, and maintainable applications.
FAQs
What is C# Map and How Does It Work?
C# Map refers to transforming data structures (arrays, lists, dictionaries) using LINQ (Select method) or dictionaries. Unlike C++ Maps, C# lacks a built-in map() function but achieves similar results.
How Does C# Map Compare to C++ Map?
C# Dictionary uses hash-based lookups (O(1)), while C++ Map (std::map) is tree-based (O(log n)). C++ Map maintains sorted order, whereas C# Dictionary is unordered.
What is the Difference Between C# Dictionary and C++ Map?
C# Dictionary is a hash table-based unordered collection, while C++ Map is a tree-based ordered container. C++ also has std::unordered_map, similar to C# Dictionary.
How Can I Use C# Map with Google Maps API?
C# can interact with Google Maps API using HttpClient for geolocation and mapping. Google Maps SDK for .NET also provides integration for mapping functionalities.
What is the Best Way to Implement Mapping in C++?
Use std::map for ordered key-value storage and std::unordered_map for faster lookups. Choose based on whether sorted access or performance is needed.
How to Perform Object-to-Object Mapping in C#?
Use AutoMapper or manually assign properties to transform objects in C#. AutoMapper simplifies property mapping between different object types.
What Are the Key Differences Between C Maps and C# Maps?
C lacks built-in maps, relying on arrays and structs, while C# offers Dictionary<TKey, TValue>. C# also provides LINQ for efficient data transformations.
How to Convert a C++ Map to a C# Dictionary?
Serialize the C++ Map to JSON and deserialize it in C# using Newtonsoft.Json. This ensures compatibility when transferring data between languages.