Bash scripting is a powerful tool for automating tasks, and handling arrays is a crucial part of it. Whether you’re processing files, managing lists of strings, or executing commands dynamically, mastering “Bash Iterate Over Array” techniques is essential.
This guide explores different looping methods, optimization techniques, and best practices to handle arrays efficiently.
Understanding Bash Arrays
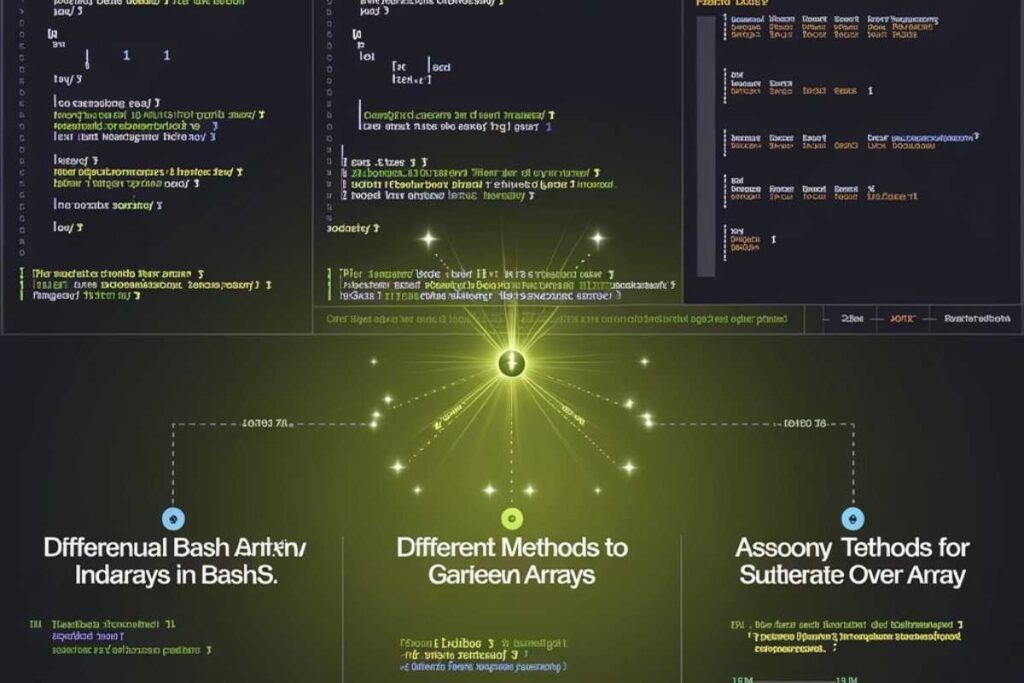
Bash supports two primary types of arrays:
- Indexed Arrays – Use numerical indices starting from 0.
- Associative Arrays – Use named keys instead of numeric indices.
Declaring an Indexed Array
my_array=("apple" "banana" "cherry")
Declaring an Associative Array
declare -A my_assoc_array
my_assoc_array=( [name]="John" [age]=25 )
Different Methods to Iterate Over an Array in Bash
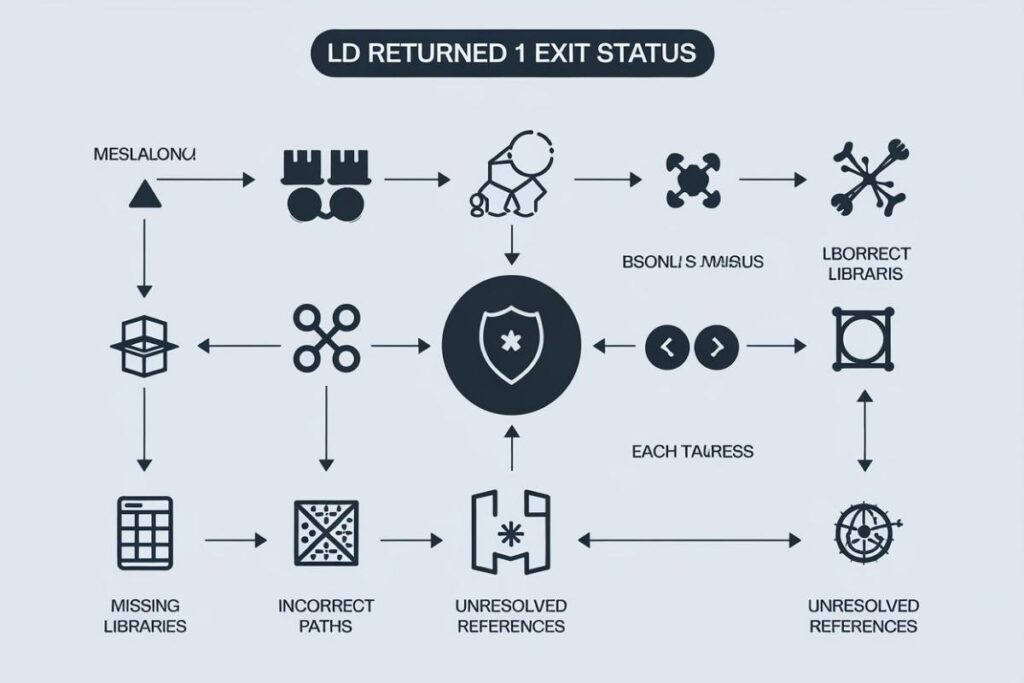
One of the most important aspects of Bash Iterate Over Array is choosing the right looping method to optimize performance.
1. Using a For Loop (Index-Based)
for i in "${!my_array[@]}"; do
echo "Index: $i, Value: ${my_array[$i]}"
done
Best Use Case: When both index and value are needed.
2. Iterating Over Values Directly
for value in "${my_array[@]}"; do
echo "Value: $value"
done
Best Use Case: When index is not required.
3. Using a While Loop with a Counter
count=0
while [ $count -lt ${#my_array[@]} ]; do
echo "Value: ${my_array[$count]}"
((count++))
done
Best Use Case: When more control over loop execution is needed.
4. Using an Until Loop
count=0
until [ $count -ge ${#my_array[@]} ]; do
echo "Value: ${my_array[$count]}"
((count++))
done
Best Use Case: When using conditions opposite to a while loop.
5. Iterating Over an Associative Array
for key in "${!my_assoc_array[@]}"; do
echo "$key: ${my_assoc_array[$key]}"
done
Best Use Case: When working with key-value pairs.
6. Using C-Style For Loop
for ((i=0; i<${#my_array[@]}; i++)); do
echo "${my_array[$i]}"
done
Best Use Case: When iterating over an array using a numerical range.
Advanced Techniques for Bash Iterate Over Array
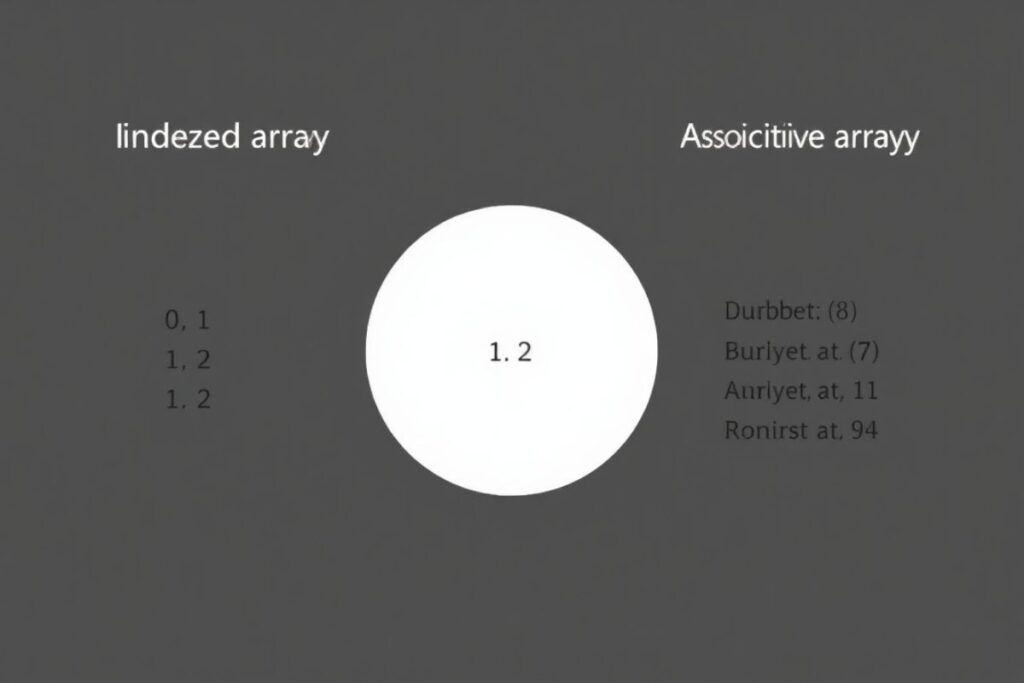
1. Modifying an Array During Iteration
for i in "${!my_array[@]}"; do
my_array[$i]="modified_${my_array[$i]}"
done
2. Filtering Array Elements
for value in "${my_array[@]}"; do
[[ "$value" == "apple" ]] && continue
echo "Filtered Value: $value"
done
3. Iterating Over an Array and Executing a Command
for file in /path/to/directory/*; do
echo "Processing: $file"
cat "$file"
done
4. Using Parallel Processing for Large Arrays
echo "${my_array[@]}" | xargs -n1 -P4 echo
Best Use Case: When handling large arrays to improve performance.
Best Practices for Efficient Bash Iterate Over Array
- Avoid using
eval
unless absolutely necessary. - Use
@
instead of*
to preserve whitespace in values. - Prefer indexed arrays over associative arrays for large datasets.
- Optimize loop execution using
while
oruntil
for frequent condition checks. - Use parallel processing for handling large datasets efficiently.
Performance Optimization Tips
- Use built-in Bash functions for handling large arrays.
- Minimize subshell usage to reduce performance overhead.
- Avoid unnecessary iterations by breaking out of loops when needed.
- Utilize
xargs
orparallel
for concurrent execution of commands.
Common Errors and Troubleshooting in Bash Iterate Over Array
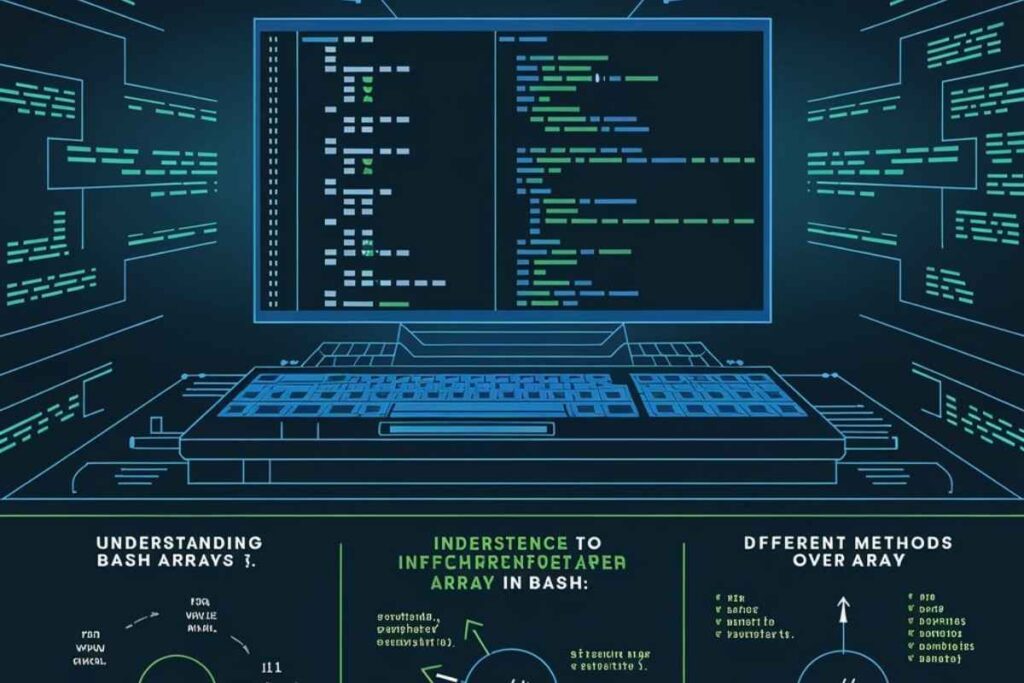
1. Whitespace Issues
my_array=("first item" "second item")
echo ${my_array[0]} # Output: first (only first word is printed)
Fix: Use double quotes to preserve spaces:
echo "${my_array[0]}" # Output: first item
2. Iterating Over Empty Arrays
empty_array=()
for value in "${empty_array[@]}"; do
echo "$value"
done
Fix: Check if the array is empty before iterating:
if [ ${#empty_array[@]} -gt 0 ]; then
for value in "${empty_array[@]}"; do
echo "$value"
done
fi
Additional Techniques for Large Arrays
Using Mapfile to Read Input into an Array
mapfile -t my_array < input.txt
Best Use Case: When reading large files into an array.
Using readarray
to Populate an Array
readarray -t my_array < input.txt
Best Use Case: Similar to mapfile
, useful for handling file-based input efficiently.
Iterating Over Multi-Line Output
while IFS= read -r line; do
echo "$line"
done < input.txt
Best Use Case: When processing a file line by line.
Conclusion
Mastering “Bash Iterate Over Array” techniques can significantly enhance your scripting capabilities. By implementing performance optimizations, troubleshooting errors, and using efficient looping techniques, you can write robust and scalable Bash scripts. Check out our in-depth guide on Bash scripting best practices for more advanced techniques.”
Keep refining your skills and exploring advanced scripting methods to maximize efficiency.
By mastering these Bash Iterate Over Array techniques, you can write cleaner, more efficient Bash scripts and automate tasks effectively.
FAQs
What is the best way to iterate over a Bash array?
Use for value in “${array[@]}” for simple iteration and for i in “${!array[@]}” if indexes are needed. Read our complete Bash scripting tutorial for more details.”
How do I optimize performance when iterating over large arrays?
Use built-in functions, avoid subshells, and leverage parallel processing.
How do I handle whitespace issues in Bash arrays?
Always wrap array elements with double quotes when referencing them.
What is the best way to handle large datasets efficiently?
Use xargs
or parallel
for concurrent execution of commands.