If you’ve encountered the “ld returned 1 exit status” error while compiling your C, C++, or Arduino code, you’re not alone.
This frustrating issue typically occurs during the linking stage of the compilation process, where the linker (ld) fails to combine your compiled code into an executable file.
In this comprehensive guide, we’ll explain the causes of this error and show you how to fix ld returned 1 exit status across different platforms such as Dev C++, Arduino IDE, and Ubuntu.
What Does “ld Returned 1 Exit Status” Mean?
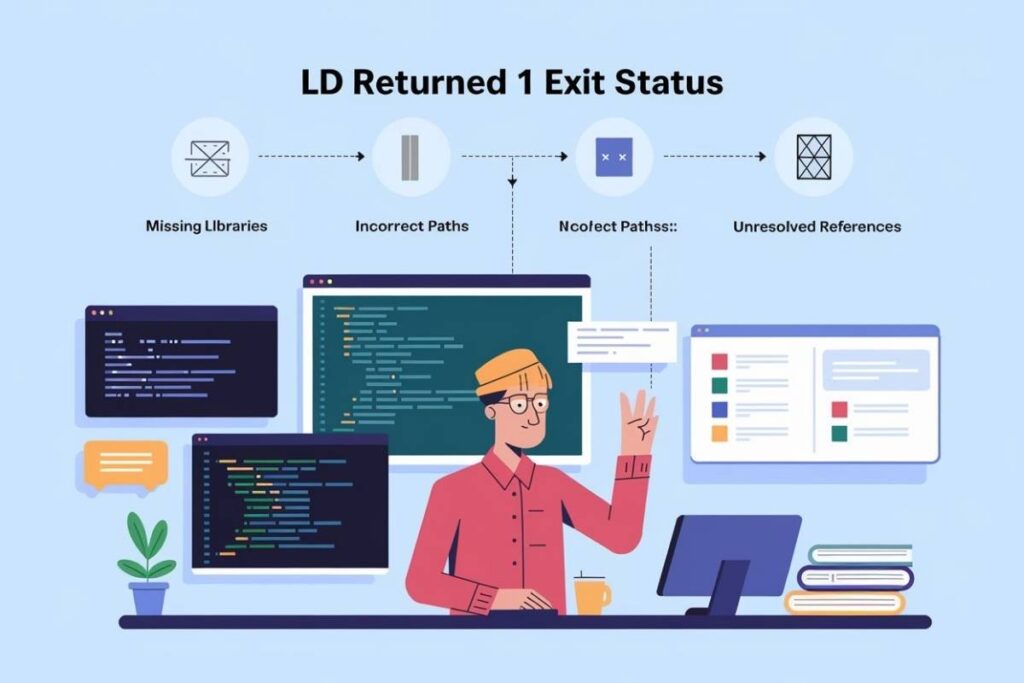
The “ld returned 1 exit status” error means that the linker (ld) was unable to successfully combine your object files and libraries into an executable program.
The exit status 1 is a general error code indicating that something went wrong during the linking phase of the build process.
Common Causes of “ld Returned 1 Exit Status”
Before fixing the error, it’s important to understand what causes it. Below are some of the most common reasons:
Missing Libraries or Header Files
One of the most common causes of the “ld returned 1 exit status” error is missing libraries or header files. If the linker can’t find a necessary library or header, it fails to complete the compilation process.
Incorrect File Paths or References
If your source files or libraries are not correctly referenced in your project or build settings, the linker won’t be able to find them, leading to this error.
Undefined or Incorrect Function Calls
The linker may also fail if your code refers to functions or variables that are either undefined or incorrectly declared. Check all your function definitions and declarations for consistency.
Symbol Conflicts
Conflicting symbols across your files can confuse the linker. For example, two functions with the same name in different files can cause this error. Renaming the conflicting symbols or using namespaces can help resolve these conflicts.
How to Fix “ld Returned 1 Exit Status” in Different Environments
Fixing the Error in Dev C++
If you’re working in Dev C++, the “ld returned 1 exit status” error is often due to missing libraries or incorrect compiler settings. Here’s how to fix it:
- Check for Missing Libraries: Open your project and go to the “Project” menu. Ensure that all required libraries are included.
- Verify Compiler and Linker Settings: In the “Compiler Options” section of your project settings, ensure you have selected the correct compiler and linker options.
- Rebuild the Project: Sometimes, old object files can cause the error. Rebuild the entire project to clear out any corrupt files.
Fixing the Error in Arduino IDE
In the Arduino IDE, the “ld returned 1 exit status” error typically results from missing libraries or incorrect board settings. Here’s how you can fix it:
- Install Missing Libraries: Open the “Library Manager” and install the libraries required for your project.
- Select the Correct Board: Ensure that you’ve selected the correct Arduino board under the “Tools” menu.
- Check for Syntax Errors: Make sure there are no syntax errors or issues in your code that could be affecting the linking process.
Fixing the Error in Ubuntu or Linux (GCC)
On Ubuntu or Linux, you may encounter this error due to missing libraries or incorrect library paths. Follow these steps to resolve it:
- Install Missing Libraries: Use the
apt-get
command to install any missing libraries:bashCopyEditsudo apt-get install libname-dev
- Specify Library Paths: If you’re using custom library paths, make sure to include them during the compilation process:bashCopyEdit
gcc -o myprogram myprogram.c -L/path/to/libs -lmylibrary
- Clean and Rebuild: Sometimes, old or corrupt object files cause issues. Clean the project using
make clean
and then rebuild.
Fixing the Error in Visual Studio Code (VS Code)
For VS Code, the “ld returned 1 exit status” error can be caused by incorrect configurations or missing dependencies. Here’s how to resolve it:
- Install Necessary Extensions: Ensure you have the C/C++ extensions installed for proper compilation support.
- Check Build Configurations: Verify that your
tasks.json
andlaunch.json
files are properly configured with the correct paths to source files and libraries. - Link the Correct Libraries: Make sure you’re linking all necessary libraries. Use verbose output to identify missing references.
General Solutions to Fix “ld Returned 1 Exit Status”
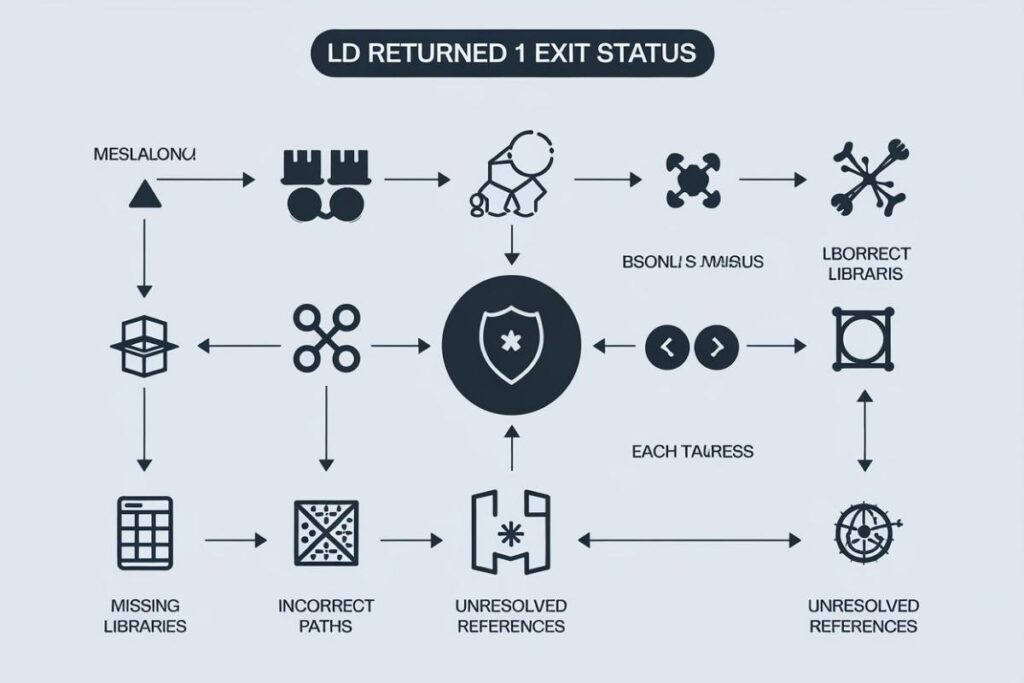
- Check for Undefined References: The linker can’t resolve references to functions or variables that are not properly declared. Ensure all references match their declarations.
- Verify File and Library Paths: Double-check the paths to your libraries and source files to ensure the linker can find them.
- Rebuild the Project: Clean up old object files and rebuild the project from scratch to avoid conflicts and outdated files.
- Use Verbose Output: Enable verbose mode in your compiler (e.g.,
gcc -v
), which will give you more detailed error messages, helping you identify missing libraries or object files.
Conclusion
The “ld returned 1 exit status” error is a common issue for developers working with C, C++, or Arduino. By understanding the causes such as missing libraries, incorrect paths, and unresolved references you can efficiently troubleshoot and resolve the issue.
Follow the fixes provided for different environments like Dev C++, Arduino IDE, and Ubuntu to get your program back on track. Happy coding!
(FAQs)
What Does “ld Returned 1 Exit Status” Mean?
The “ld returned 1 exit status” error indicates that there was a problem during the linking phase of compilation. It generally means that the linker was unable to combine your object files and libraries into an executable.
How Do I Fix “ld Returned 1 Exit Status” in Dev C++?
In Dev C++, make sure all necessary libraries are included, check your compiler settings, and try rebuilding the project to clear out any old object files.
How Do I Fix “ld Returned 1 Exit Status” in Arduino?
In Arduino IDE, ensure that all required libraries are installed and that the correct Arduino board is selected under the “Tools” menu.
How Do I Fix “ld Returned 1 Exit Status” in Ubuntu?
On Ubuntu, install any missing libraries and ensure that all file paths are correctly specified during the compilation process.
What Is Exit Status 1 in Compilation?
Exit status 1 indicates that the program failed to compile due to an issue in the code or build configuration, such as missing files, undefined references, or incorrect paths.