Regular expressions (regex) are a cornerstone for advanced text processing and pattern matching across various programming languages and tools.
One of the most crucial components in this domain is the “Regex or” operator. Represented by the pipe symbol (|
), the “Regex “ operator allows developers to match multiple patterns within a single expression,
In this guide, we explore the concept of “Regex “, its applications in multiple programming environments
Regular Expressions and “Regex or”
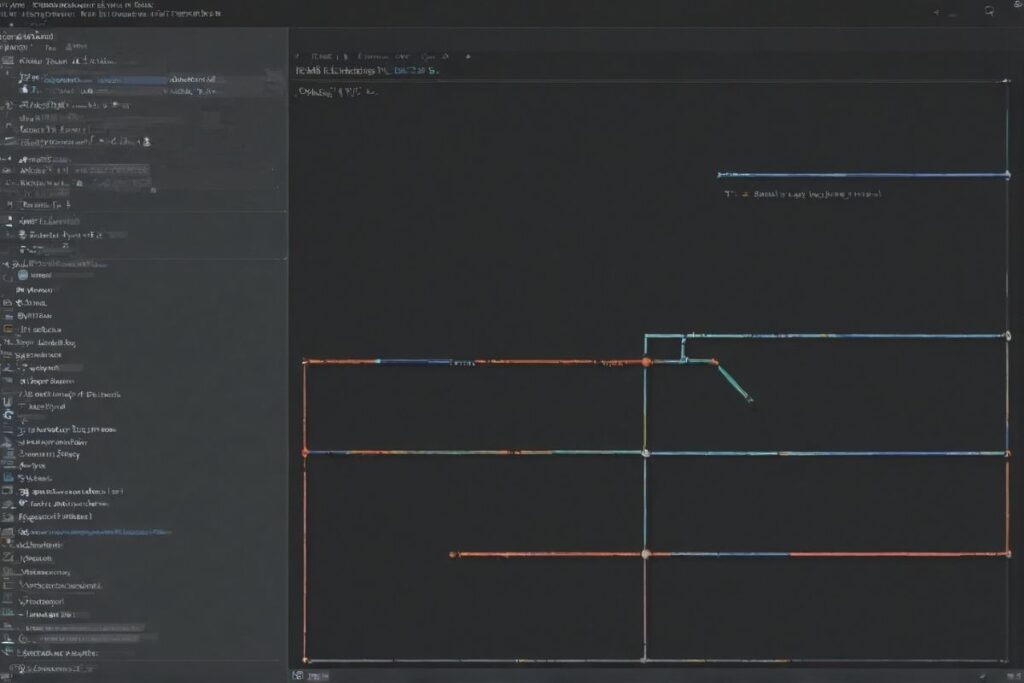
Regular expressions are specialized strings that define search patterns used for matching sequences of characters in text. They are indispensable for tasks such as:
- Data validation: Ensuring user input meets specific criteria.
- Search and replace: Modifying text in documents or code.
- Syntax highlighting: Identifying keywords and patterns in code editors.
- Log file analysis: Extracting useful information from system logs.
At the heart of many regex patterns lies the “Regex or” operator, which uses the pipe symbol (|
) to denote alternation. This operator enables matching one pattern or another, making it extremely useful when there are multiple valid possibilities.
The “Regex or” Operator Explained
The “Regex or” operator is used to separate multiple alternatives in a regex pattern. For instance, the regex pattern:
regex
cat|dog
matches either the word “cat” or “dog”. By leveraging the “or Regex“ operator, you can combine different patterns into a single, compact expression.
Key Characteristics of the “Regex or” Operator:
- Alternation: The operator allows for the alternation between multiple patterns. For example,
(cat|dog)
defines a group where either “cat” or “dog” can be matched. - Grouping: Parentheses are frequently used with the “Regex or” operator to limit the scope of the alternation. For example,
(cat|dog)s?
will match “cat”, “cats”, “dog”, or “dogs”. - Precedence: It is important to note that the “or Regex“ operator has a lower precedence than concatenation. This means that without proper grouping, concatenated parts of the regex might be evaluated before the alternation.
“Regex or” in Various Programming Languages
The concept of “Regex or” is ubiquitous in programming. Here, we examine its implementation in Python, C++, and JavaScript, highlighting practical examples for each.
Using “or Regex” in Python
Python’s built-in re
module offers robust support for regular expressions, making it simple to use the “Regex or” operator.
Example:
python
import re
# Define a regex pattern using the "or Regex" operator
pattern = r'cat|dog'
text = "I have a cat and a dog in my house."
# Find all matches
matches = re.findall(pattern, text)
print(matches) # Output: ['cat', 'dog']
Explanation:
- The pattern
r'cat|dog'
utilizes the or Regex“ operator to search for either “cat” or “dog”. - The
re.findall
function returns a list of all matched occurrences.
Implementing “Regex or” in C++
C++ supports regular expressions through the <regex>
library, which was introduced in C++11. The “or Regex“ operator works seamlessly in this environment.
Example:
cpp
#include <iostream>
#include <regex>
#include <string>
#include <iterator>
int main() {
std::string text = "I have a cat and a dog in my house.";
std::regex pattern("cat|dog");
auto words_begin = std::sregex_iterator(text.begin(), text.end(), pattern);
auto words_end = std::sregex_iterator();
std::cout << "Matches using Regex or:" << std::endl;
for (std::sregex_iterator i = words_begin; i != words_end; ++i) {
std::smatch match = *i;
std::cout << match.str() << std::endl;
}
return 0;
}
Explanation:
- The
<regex>
library is used for pattern matching. - The “Regex or” operator in the pattern
"cat|dog"
successfully captures both alternatives.
Using “Regex or” in JavaScript
JavaScript’s built-in regex engine makes it straightforward to use the “or Regex“ operator.
Example:
javascriptconst text = "I have a cat and a dog in my house.";
const pattern = /cat|dog/g;
const matches = text.match(pattern);
console.log("Matches using Regex or:", matches); // Output: ["cat", "dog"]
Explanation:
- The regex pattern
/cat|dog/g
employs the “Regex or” operator. - The global flag (
g
) ensures that all occurrences of the pattern are returned.
Practical Applications and Advanced Examples of “or Regex”
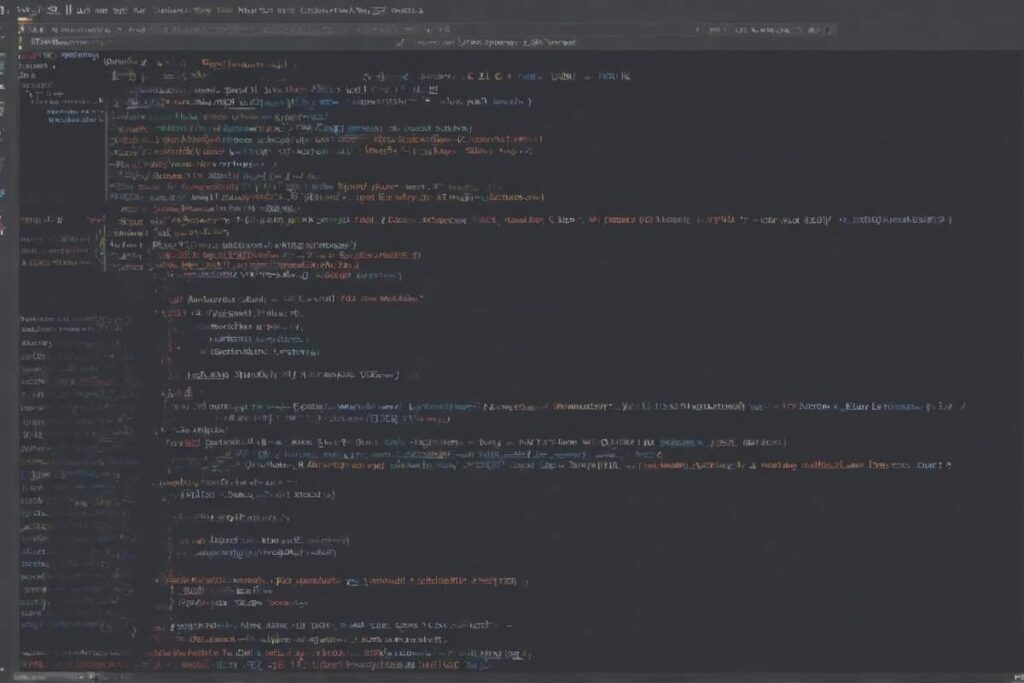
The “Regex or” operator can be applied in a variety of practical scenarios. Here are some advanced examples to illustrate its versatility.
Matching Multiple File Extensions
Imagine you need to match file names with extensions such as .jpg
, .png
, or .gif
. The “Regex or” operator can simplify this task:
regex\.(jpg|png|gif)$
Explanation:
- The expression
\.
matches a literal dot. - The group
(jpg|png|gif)
uses the “or Regex“ operator to match any of the file extensions. - The
$
asserts the position at the end of the string.
Matching Alternative Date Formats
To match dates in either YYYY-MM-DD
or DD/MM/YYYY
formats, the “Regex or” operator provides a concise solution:
regex
(\d{4}-\d{2}-\d{2})|(\d{2}\/\d{2}\/\d{4})
Explanation:
- The pattern contains two alternative groups separated by the “or Regex“ operator.
- Each group corresponds to a different date format.
- The use of
\d
for digits and appropriate delimiters (hyphen or slash) makes the expression flexible for matching dates.
Searching for Multiple Keywords
When you need to search for any of two or more keywords within a text, the “or Regex“ operator is invaluable. For example, to match the keywords “error” or “failure”:
regex
(error|failure)
Explanation:
- This pattern directly matches either “error” or “failure”.
- It can be integrated into larger expressions for more complex matching rules.
Tools and Resources for Testing “Regex or” Patterns
Before integrating regex patterns into your projects, testing them thoroughly is crucial. There are several online tools designed to help you experiment with and perfect your “or Regex“ patterns.
Recommended Online Tools:
- Regex101: Offers detailed explanations and real-time testing.
- Regexr: Provides an intuitive interface and community-shared regex patterns.
- RegEx Tester by GeeksforGeeks: Features interactive testing with examples.
- Regex Pal: A straightforward tool for quick regex testing.
Using these tools, you can adjust your patterns and maintain the correct ” or Regex” operator usage to meet your specific needs.
“Regex or” in Unix with Grep
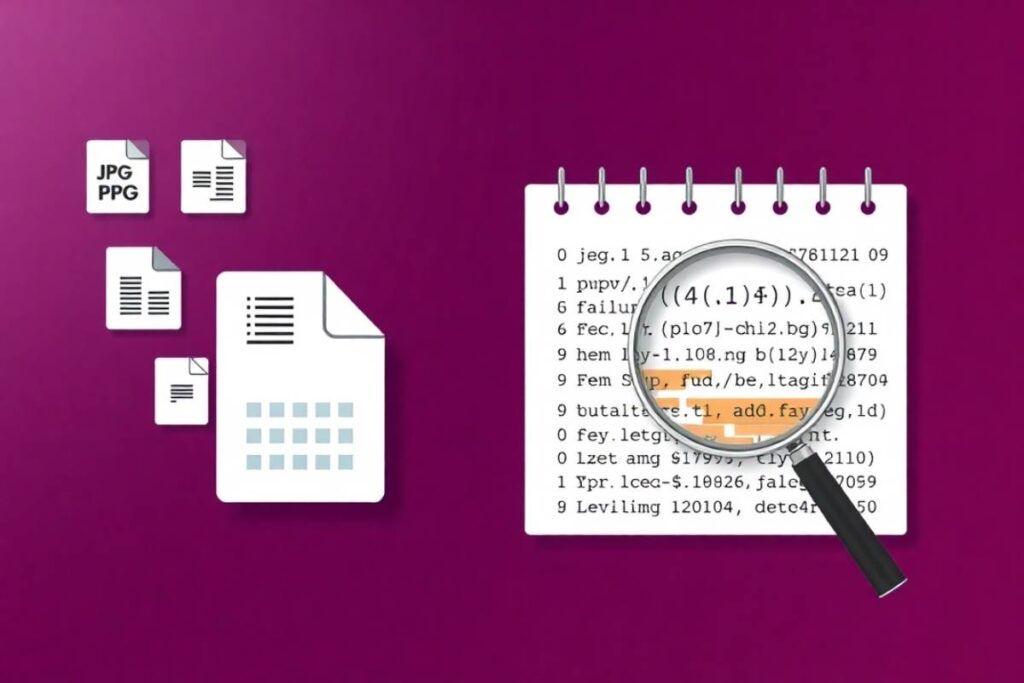
In Unix-like systems, the grep
command is a powerful utility for searching text using regular expressions. By employing the ” or Regex” operator, you can search for multiple patterns simultaneously.
Example using Grep:
bash
grep -E 'cat|dog' filename.txt
Explanation:
- The
-E
flag enables extended regex, which supports the ” or Regex “ operator. - The command searches for lines containing either “cat” or “dog” in the file
filename.txt
.
Conclusion
The alternation operator is an essential element in the toolbox of anyone working with regular expressions.
Its ability to match multiple patterns simultaneously makes it a powerful tool for developers and data professionals alike.
By understanding and applying the or operator in various environments—be it Python, C++, JavaScript, or Unix grep—you can create more efficient, readable, and effective regex patterns.
Embrace the power of alternation and elevate your regex proficiency to new heights. Happy coding and regex testing!
FAQs
What is the alternation operator?
It is represented by the pipe symbol (|) in regex and allows you to specify multiple alternative patterns in a single expression.
How do I use the operator with grouping?
Parentheses are used to group alternatives. For example, (cat|dog) matches either “cat” or “dog”. Grouping helps control the scope of the operator.
Can I combine this operator with other regex elements?
Yes, it is highly versatile and can be combined with quantifiers, character classes, and other regex constructs. For example, (cat|dog)s? matches both singular and plural forms of “cat” or “dog”.
Why is the operator important?
It simplifies regex patterns by allowing multiple alternatives in one expression, reducing redundancy and improving readability, especially when matching complex patterns.
What precautions should I take?
Always use parentheses to properly group alternatives and avoid unintended matches. Be mindful of operator precedence in regex to ensure correct application within the pattern.
How do I test my patterns?
Use online regex testers such as Regex101, Regexr, or RegEx Tester by GeeksforGeeks for real-time feedback and detailed explanations of your expressions.
Can I use this operator with grep?
Yes, when using grep with the -E flag (extended regex), you can apply the operator. For example, grep -E ‘cat| dog’ filename.txt will search for lines containing either “cat” or “do