Python provides a straightforward way to work with lists and strings, making it easy to manipulate and transform data effectively for various programming tasks.
One common task is to convert list to string Python, which is essential when preparing data for output, storage, or further processing.
In this guide, we’ll explore different methods and examples to achieve this, covering both simple techniques and more advanced approaches for handling complex scenarios.
What Does Converting a List to a String Mean?
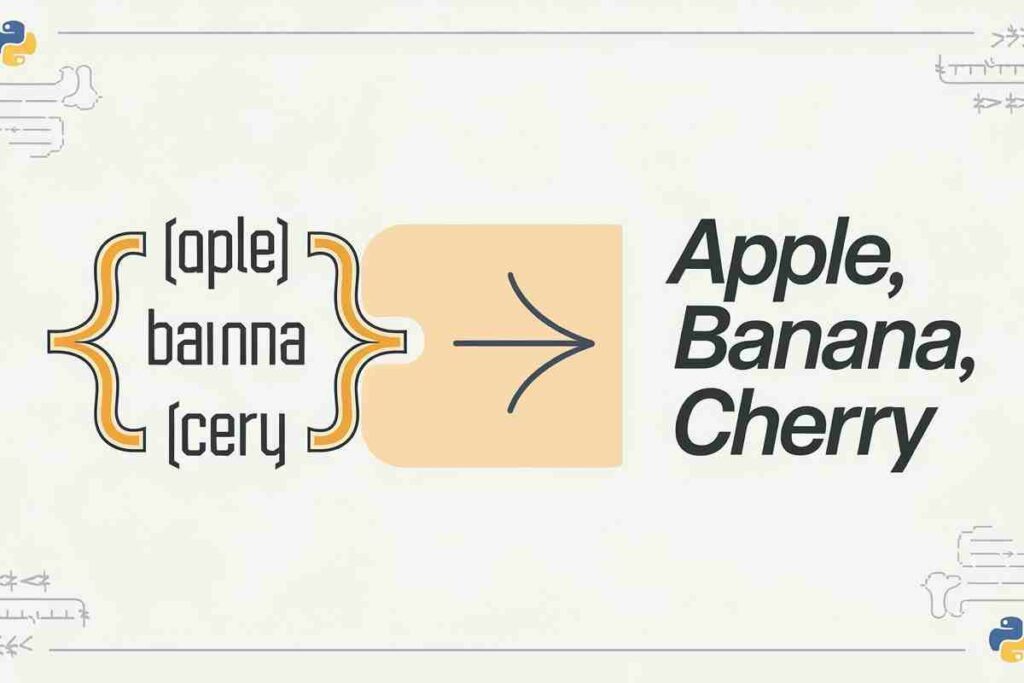
Converting a list to a string involves transforming elements of the list into a single string. This can be useful when:
- Printing a readable representation of a list.
- Storing data in text files or databases.
- Preparing data for APIs or web requests.
Python offers multiple techniques to accomplish this task. Let’s dive into the methods.
How to Convert a List to a String in Python
Using the join()
Method
The join()
method is the most efficient way to convert list to string Python. It works seamlessly with lists of strings and allows you to specify a delimiter.
Example:

Explanation:
- The space (‘ ‘) acts as a delimiter.
- Each element in the list is joined by the specified delimiter.
LSI Keywords Used:
- List to string Python with comma
- List of strings to string Python
Handling Non-String Elements in Lists
If your list contains non-string elements (e.g., integers or floats), the join()
method will raise a TypeError
. You’ll need to convert all elements to strings first.
Example:
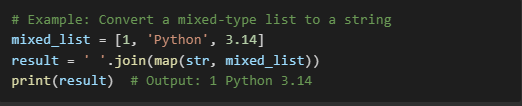
Explanation:
- The
map()
function applies thestr()
function to each element in the list. - The
join()
method then combines the elements.
Using List Comprehension with join()
List comprehensions offer a concise way to process elements before joining them, which is helpful if you need to filter or format the list. This technique is often used when you want to convert list to string Python.
Example:
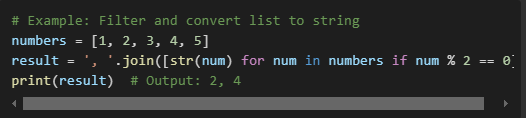
Converting a List of Lists to a String
To convert a list of lists to a string in Python, you need to flatten the list or handle nested lists explicitly.
Example:
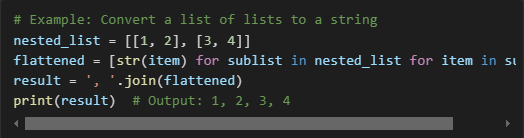
LSI Keywords Used:
- Convert list of list to string Python
Alternatives to join()
Using String Concatenation
Although less efficient, you can manually concatenate list elements using a loop.
Example:
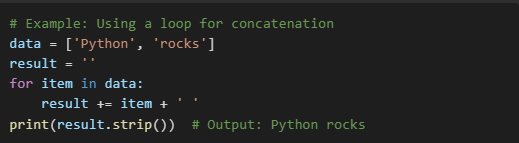
Using the str()
Function
The str()
function can directly convert a list into a string representation, including brackets and commas.
Example:

Note: This approach includes the brackets and is not suitable for clean output.
Real-World Applications
Formatting Data for CSV Files
When preparing data for CSV files, you often need to convert a list to a string with commas.
Example:

Preparing JSON Strings
When creating JSON objects, you may need to convert lists into JSON-compatible strings.
Example:
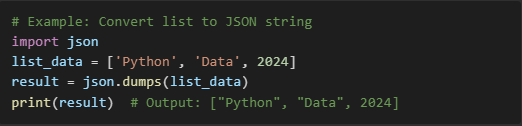
Additional Methods for Advanced Scenarios
Using reduce()
Function from functools
The reduce()
function allows you to process a list and combine elements step by step, making it more flexible for custom concatenation logic when you want to convert list to string Python.
Example:
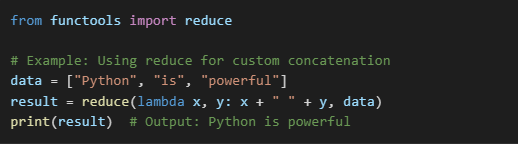
Using Generators for Large Lists
If you’re working with a very large list and need to convert list to string Python, consider using a generator to save memory. Generators create items on the fly rather than storing the entire processed list in memory.
Example:

Tips and Best Practices
- Choose the Right Delimiter: Use delimiters like commas, spaces, or newlines based on the context.
- Handle Non-String Elements Carefully: Always convert non-string elements to strings before joining.
- Optimize for Large Data: For large lists, avoid manual loops; use built-in methods like
join()
for better performance. - Test with Edge Cases: Consider edge cases such as empty lists, nested lists, or lists containing special characters.
Conclusion
In this guide, we’ve covered various methods to convert list to string Python, including join(), handling non-string elements, and working with nested lists.
Advanced methods such as reduce() and generators were also discussed for handling large or complex data structures, making your code efficient and easier to maintain in real-world applications.
Whether you’re formatting data for output or preparing it for storage, Python’s flexibility ensures a solution for every scenario. Practice these techniques to make your Python scripts more efficient and maintainable.
FAQs
How do I convert list to string Python?
To convert list to string Python, you can use the join()
method. For example:

How do I convert a list of numbers to a string in Python?
Use the join()
method with map()
to convert numbers into strings.
Can I convert a list to a string in Java like Python?
Yes, Java offers similar functionality using String.join()
or loops.
What happens if the list contains None
or NaN
?
Convert such elements to strings using str()
or filter them out beforehand.
How do I handle nested lists while converting to strings?
Flatten the list using list comprehensions and then apply the join()
method.
Can I use a custom delimiter for joining list elements?
Yes, specify the delimiter in the join()
method, like ', '.join(list_data)
.
What if my list is empty?
The join()
method will return an empty string for an empty list.
How do I save the converted string to a file?
Open a file in write mode and use file.write()
to save the string.
Can I convert a list with boolean values to a string?
Yes, use map(str, list_data)
to convert boolean values to strings.