In JavaScript, there’s no built-in sleep function like in other programming languages, which makes adding delays or pauses in code a bit tricky.
However, developers often need to implement a “sleep” effect to pause execution at certain points in their programs, ensuring smooth user interactions and optimized performance.
This blog will explore how to use JavaScript sleep and introduce common methods to simulate a sleep-like effect in JavaScript, improving control over asynchronous behavior.
What is JavaScript Sleep?
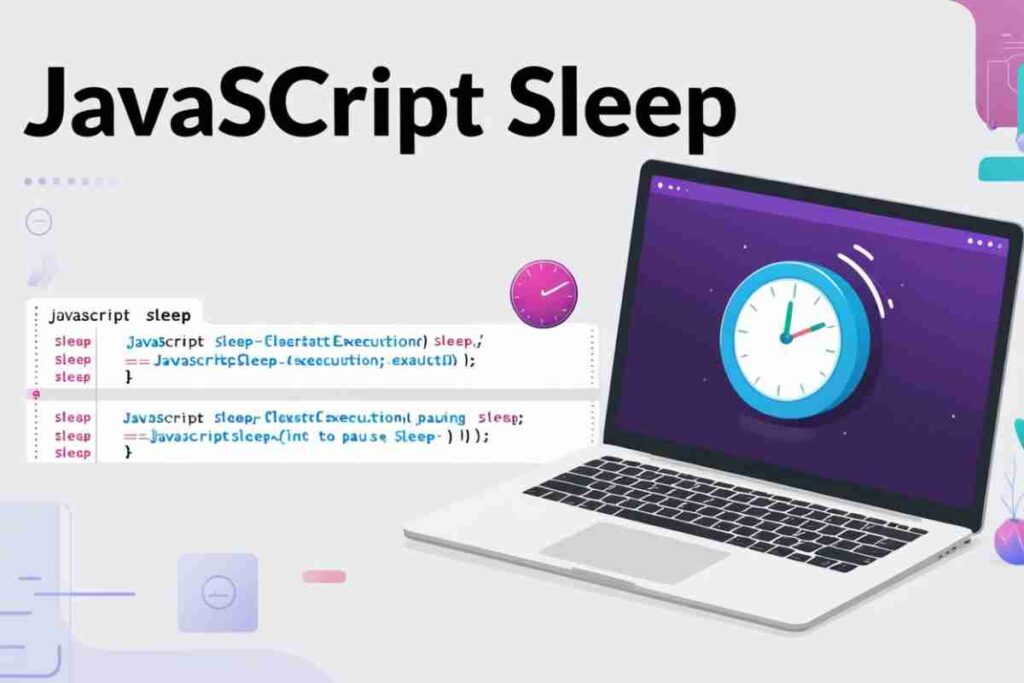
When we talk about JavaScript sleep, we are referring to a method of pausing the execution of JavaScript code for a specific amount of time.
In some programming languages, such as Python, you can call a sleep()
function to pause execution. Unfortunately, JavaScript doesn’t have a native sleep function, which leaves developers with a few alternative approaches to implement pauses in code.
Though JavaScript doesn’t provide an exact equivalent to the sleep()
function, you can simulate delays or pauses using other built-in methods such as setTimeout
, setInterval
, or even async/await
in modern JavaScript.
Why Do You Need a Sleep Function in JavaScript?
In JavaScript, it’s common to use asynchronous operations that might require some delay, such as fetching data from an API or waiting for user input.
Sometimes you may need to pause execution to allow other operations to complete or simulate a time delay (e.g., in games or animations).
Here are a few scenarios where JavaScript sleep might be useful:
- Delaying the execution of a function: This can be helpful in situations like waiting for a UI animation to complete before running other functions.
- Simulating time delays: In cases like testing or building interactive applications, introducing delays can create a more natural experience for the user.
- Pausing loops: Often, developers may need to pause loops to allow for events to complete, especially in complex interactions or simulations.
Implementing Sleep in JavaScript
Although JavaScript doesn’t have a direct sleep
function, there are several ways to mimic this behavior. Let’s explore some popular methods to introduce sleep in JavaScript.
Using setTimeout()
to Simulate Sleep
One of the easiest ways to simulate sleep in JavaScript is by using the setTimeout()
function. This method allows you to schedule a callback function to run after a specified number of milliseconds.
Example: Sleep Using setTimeout()
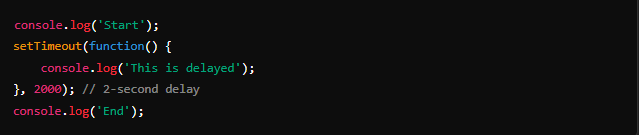
In the above example, the output would be:

Here, setTimeout()
doesn’t halt the execution of code but schedules the delayed function to run after the specified time.
While setTimeout()
allows you to introduce delays in your code, it doesn’t block the execution of subsequent code, making it a non-blocking solution.
Using async/await
with Promise
For more control over asynchronous code, you can use async/await
in combination with a Promise
to create a “sleep” effect. This method is particularly useful for pausing the execution within an async
function.
Example: Sleep Using async/await
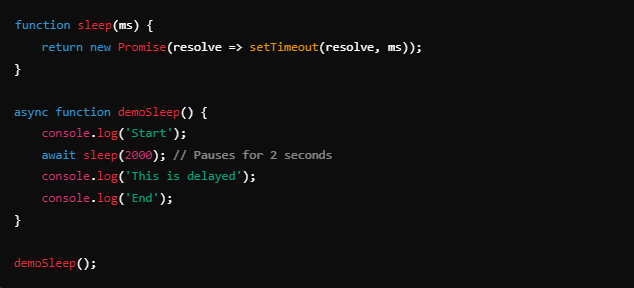
In this example, the sleep()
function returns a promise that resolves after the specified number of milliseconds. The await
keyword pauses the execution of the demoSleep
function until the promise is resolved, effectively creating a sleep effect.
The output will be:

This is an elegant solution as it allows asynchronous code to be written in a synchronous manner.
Using setInterval()
setInterval()
is another way to implement time delays in JavaScript, though it’s often used for repeatedly executing a function at specific intervals.
While it’s not exactly the same as a sleep function, you can combine it with a clearInterval()
method to simulate pauses in execution.
Example: Sleep Using setInterval()
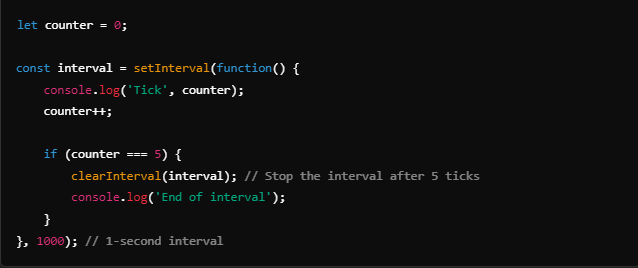
This will output:
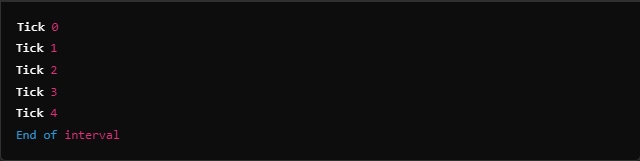
JavaScript Sleep in a Loop
In some cases, developers may need to introduce sleep within a loop, such as for simulations or animation frames. One common approach is to combine async/await
with a loop to simulate delays between iterations.
Example: JavaScript Sleep in Loop Using async/await
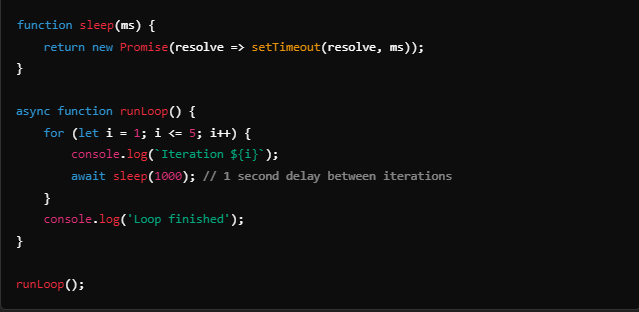
In this example, the loop will print each iteration, pause for 1 second between each, and then finish.
Sleep in JavaScript Without async/await
Although async/await
is the modern approach, you can implement sleep-like behavior without it by using setTimeout
within a loop.
However, it’s less elegant and can lead to callback hell or messy code, so it’s usually not recommended for larger projects.
Example: JavaScript Sleep Without Async
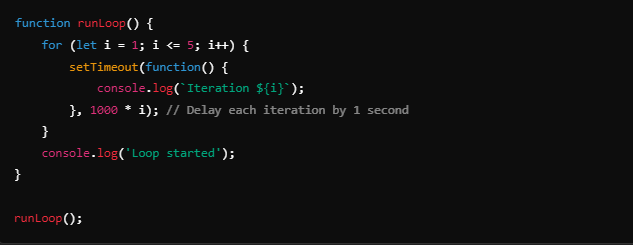
This will produce similar behavior, but it’s harder to control and manage in complex scenarios.
JavaScript Sleep with setTimeout
and Promise
An often-used approach for adding delays is combining setTimeout()
with a promise. This way, you get the non-blocking behavior of setTimeout
but can also handle it with async/await
for a cleaner and more manageable structure.
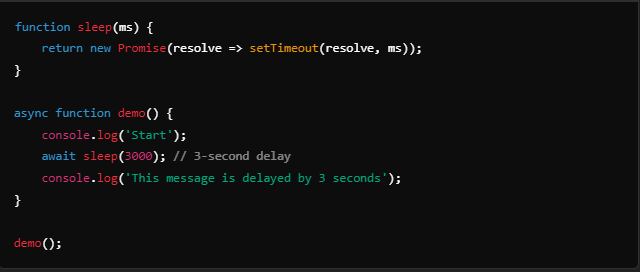
This combination makes the code asynchronous while still allowing you to wait before proceeding with the next step.
Conclusion
While JavaScript doesn’t have a built-in sleep function, using setTimeout
, async/await
, or setInterval
can provide an effective solution to simulate delays in your code.
Each method has its use case, and understanding when and how to use them effectively is essential for writing clean, efficient, and maintainable code.
Whether you’re working with loops, animations, or API requests, adding sleep functionality can enhance your JavaScript applications.
FAQs
What is JavaScript sleep?
JavaScript sleep refers to simulating a delay in code execution, often achieved using methods like setTimeout
or async/await
.
How do I pause execution in JavaScript?
You can pause execution in JavaScript using setTimeout
, setInterval
, or by creating a delay with async/await
.
Can I use sleep()
directly in JavaScript?
No, JavaScript doesn’t have a built-in sleep()
function, but you can simulate it using setTimeout
or async/await
.
What is async/await
in JavaScript?
async/await
allows you to handle asynchronous code in a more readable, synchronous-like manner, often used for delays.
How does setTimeout
work in JavaScript?
setTimeout
schedules a function to run after a specified delay, but it doesn’t block the execution of subsequent code.
Can I use sleep in a loop?
Yes, you can use async/await
or setTimeout
inside a loop to introduce delays between iterations.
Is setInterval
useful for sleep in JavaScript?
setInterval
runs a function repeatedly at a specified interval, and while it’s not for sleep, it can simulate periodic delays.
Can I sleep without using async/await
?
Yes, you can use setTimeout
or setInterval
to simulate sleep without using async/await
. However, it can be harder to manage.