Git is a powerful version control system that allows developers to manage changes in their codebase efficiently, track project history, collaborate with others, and ensure seamless versioning.
One of the core tasks when working with Git is managing branches, whether it’s for adding new features, fixing bugs, testing new code, or experimenting with different solutions.
However, once branches have served their purpose, they need to be deleted to maintain a clean and organized repository, improving workflow efficiency.
In this post, we’ll explain how to Git delete branch both locally and remotely, as well as how to handle common errors such as “cannot delete branch checked out at” or “Git delete branch not on remote.”
Why Git Delete Branch?
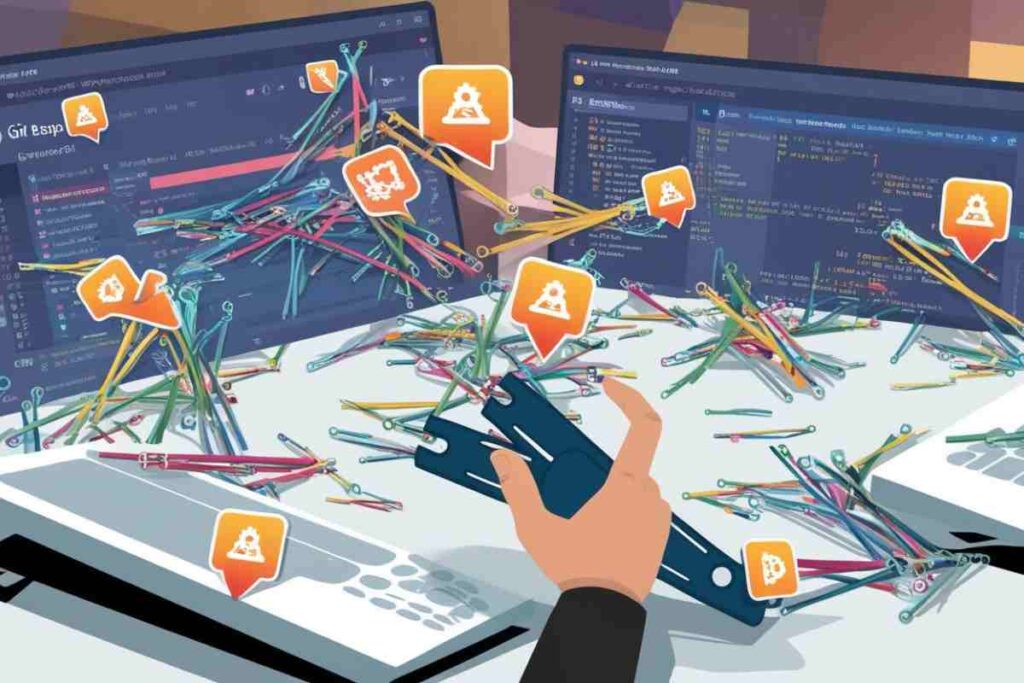
Before diving into the technical details, it’s important to understand why deleting branches is necessary. Over time, as developers work on various features and fixes, multiple branches are created.
These branches can pile up and make the repository cluttered. Deleting unnecessary branches helps keep the repository clean, easier to navigate, and reduces the chances of confusion or accidental commits to the wrong branch.
Git provides several commands for deleting branches, both locally and remotely. In this guide, we’ll walk through the steps for deleting branches, resolving potential issues, and exploring error messages you might encounter along the way.
How to Git Delete Branch Locally
Deleting a local Git branch is a straightforward process. Follow these steps to remove a branch from your local repository.
Step 1: Checkout the Branch You Want to Keep
Before deleting any branch, ensure that you’re not currently on the branch you wish to delete. Git won’t allow you to delete the branch you’re currently working on.
Use the following command to switch to a different branch:

In this case, we are switching to the main
branch. You can also use any other branch name as long as you’re not on the one you want to delete.
Step 2: Delete the Local Branch
Once you’re on a different branch, it’s safe to delete the local branch. Use the following command to delete the local branch:

Here, replace branch-name
with the name of the branch you want to delete. The -d
flag stands for “delete.” It’s a safe operation, as it prevents deletion if the branch has unmerged changes.
If you are sure you want to delete the branch, even if it has unmerged changes, use the -D
flag instead:

Deleting a Remote Git Branch
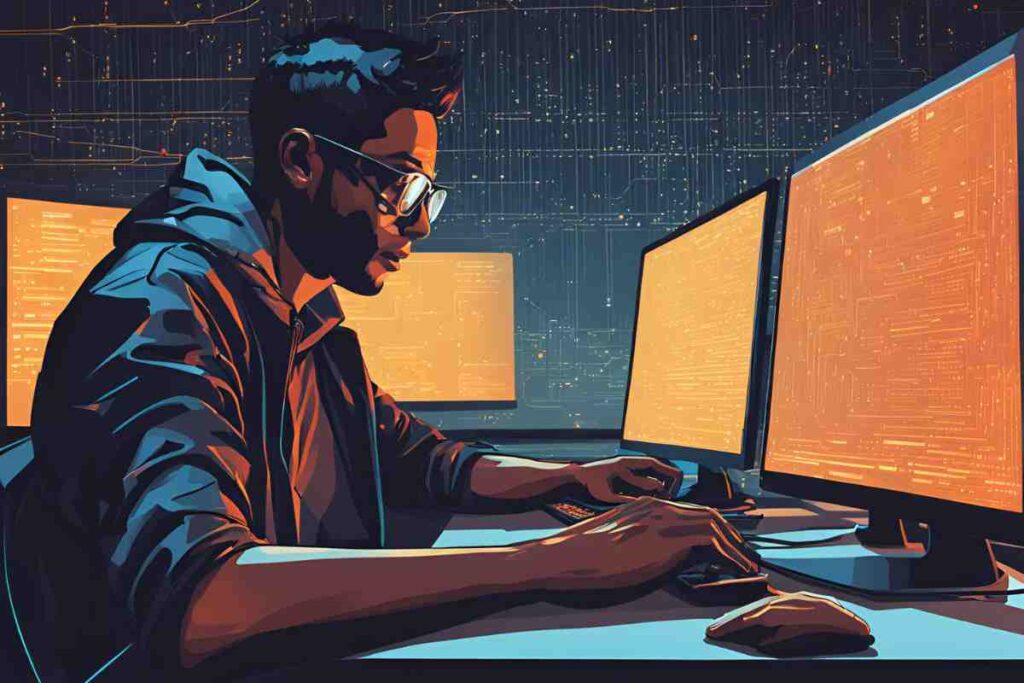
In addition to deleting a local branch, it’s also important to clean up remote branches that are no longer needed.
This is especially important when working in a team environment to ensure that others don’t accidentally push changes to outdated branches, which is why knowing how to Git Delete Branch is essential.
Step 1: Delete the Remote Branch
To delete a branch from a remote repository, use the following command:

This command deletes the specified branch from the remote repository (usually GitHub, GitLab, Bitbucket, etc.).
Step 2: Verify Deletion
To verify that the branch has been deleted from the remote repository, use the following command:

This will list all remote branches. If the branch has been deleted, it should no longer appear in the list.
Error Handling When Deleting Branches
While working with Git Delete Branch, you may encounter a few errors when attempting to delete branches. Let’s take a look at some of the most common errors and how to fix them..
Error: Cannot Delete Branch Checked Out At
One of the most common errors when trying to delete a branch is the “cannot delete branch checked out at” error. This happens when you try to delete the branch you’re currently on.
To resolve this, simply switch to a different branch before attempting to Git Delete Branch that you no longer need, ensuring that no changes are lost.
For example, if you’re on feature-branch
, you need to switch to main
or any other branch before deleting feature-branch
.

Error: Git Delete Local Branch Not on Remote
Another common issue occurs when you try to Git Delete Branch that has already been deleted on the remote but still exists in your local repository.
If you encounter this error, it’s usually because the branch has been removed from the remote but the local reference hasn’t been updated.
To fix this, first run:

This will clean up any stale remote-tracking branches in your local repository. Then, try deleting the local branch again.

Best Practices for Git Branch Management
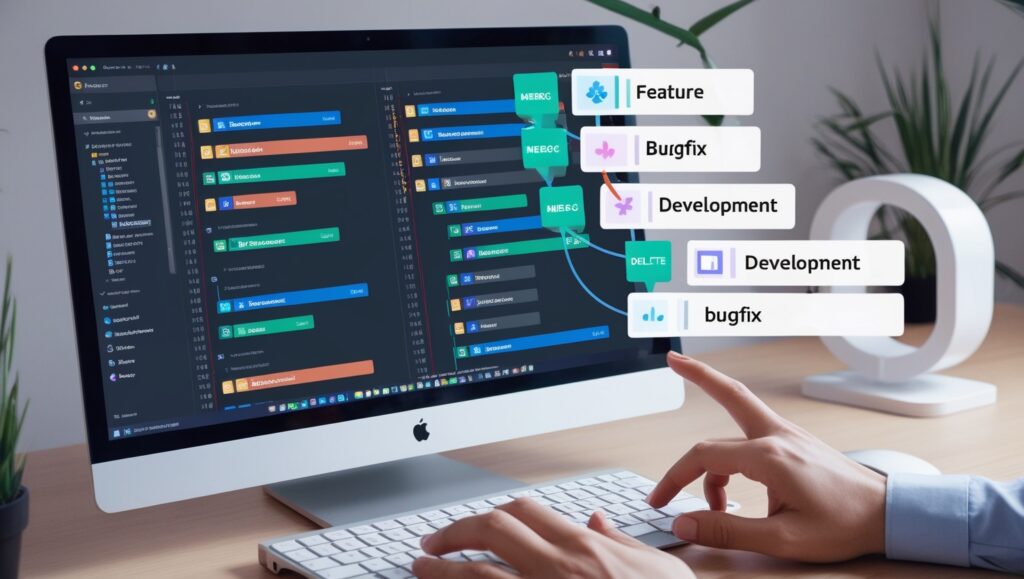
Now that we’ve covered how to Git Delete Branch locally and remotely, here are a few best practices to keep your branches organized and your repository clean.
- Use Descriptive Branch Names:
Always give your branches clear and descriptive names. This will help you and your teammates easily identify the purpose of each branch. - Delete Branches After Merging:
After successfully merging a branch into the main codebase (usuallymain
ordevelop
), delete the branch to avoid cluttering the repository. Both local and remote branches should be cleaned up. - Regularly Prune Stale Branches:
Periodically run thegit fetch --prune
command to remove outdated remote-tracking references. This helps avoid errors when deleting branches that no longer exist on the remote. - Communicate with Your Team:
In a team environment, always communicate with your teammates when deleting branches, especially remote ones. It’s important to ensure no one is working on the branch before it’s deleted.
Conclusion
Deleting branches in Git helps keep your repository clean and organized. Use git branch -d
for local deletions and git push origin --delete
for remote ones.
Always ensure you’re not on the branch you wish to delete and communicate with your team. By following these simple steps, you can avoid common errors and keep your Git workflow smooth. Happy coding!
FAQs
How do I delete a local Git branch?
Use git branch -d branch-name
to safely delete a local branch.
Can I delete a branch that’s not fully merged?
Yes, use git branch -D branch-name
to forcefully delete an unmerged branch.
How do I delete a remote Git branch?
Use git push origin --delete branch-name
to delete a branch from the remote repository.
What happens if I try to delete the branch I’m currently on?
Git won’t allow you to delete the branch you’re checked out on; switch to another branch first.
How can I fix “cannot delete branch checked out at” error?
Switch to a different branch with git checkout main
and then try deleting the branch.
How do I clean up branches no longer on the remote?
Run git fetch --prune
to remove references to deleted remote branches from your local repo.
How can I list all remote branches?
Use git branch -r
to view all remote branches.
What’s the difference between git branch -d
and git branch -D
?
-d
deletes a branch safely, while -D
forces deletion, even with unmerged changes.