When working with Python, determining whether a string contains a particular substring, character, multiple substrings, or patterns using various methods is a common task.
In this post, we’ll explore the versatile ways Python handles such queries. Whether you’re a beginner or seasoned coder, mastering Python string contains operations is a must.
What is Python String Contains?
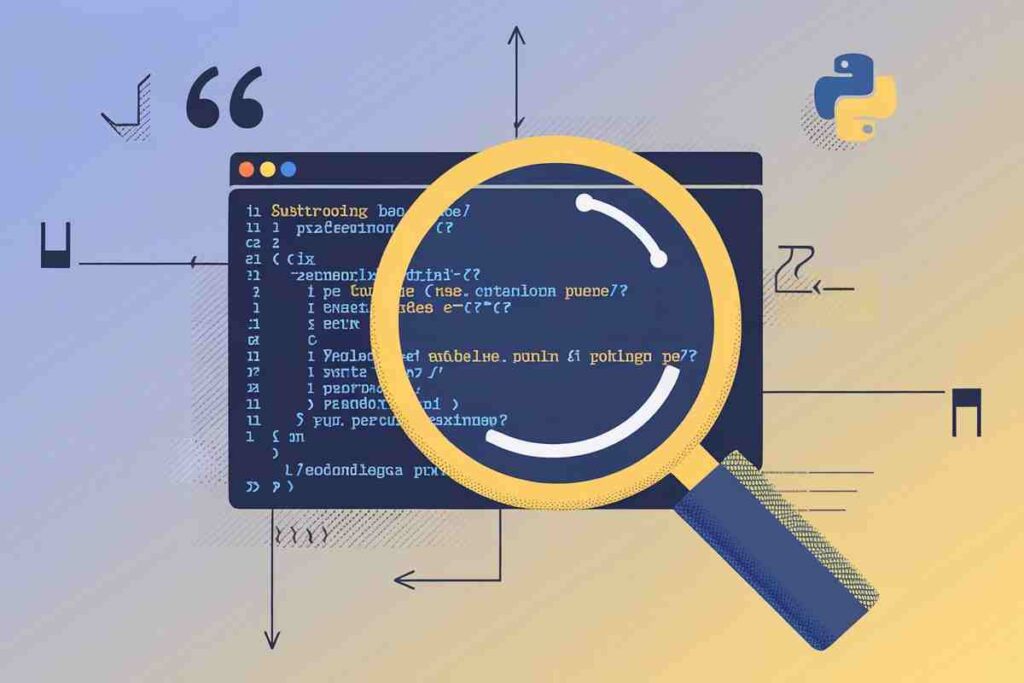
The Python string contains operation refers to verifying if a particular substring, character, or multiple substrings exist within a larger string.
This operation is crucial in scenarios like input validation, filtering data, implementing search functionalities, and processing large-scale textual data efficiently.
Python offers several methods and techniques to perform this task, ranging from the simple in keyword to advanced regex patterns. Let’s dive into the details.
Using the in Keyword to Check Python String Contains
The simplest way to determine if a Python string contains a substring or character is by using the in keyword. This method is concise and highly readable.
Example: Using in for Substring Check
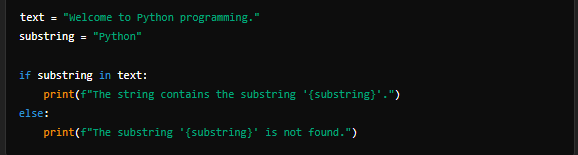
Output
The string contains the substring ‘Python’.
Why Use in?
- Efficient: No additional imports required.
- Readable: Ideal for simple checks.
Python String Contains Multiple Substrings
If you need to check whether a string contains multiple substrings, combining the in keyword with loops or comprehensions is effective.
Example: Check Multiple Substrings
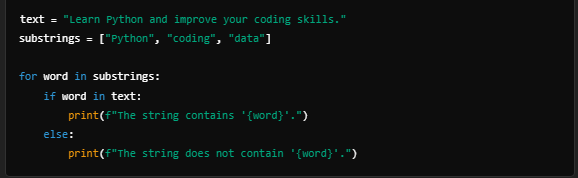
Output

Advanced Techniques: Python Check if String Contains Substring from List
A more efficient way to determine if any substring from a list exists in a string is by using list comprehensions or functions like any().
Example: Using any()
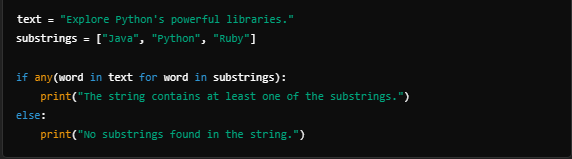
This approach minimizes iteration and is cleaner than traditional loops.
Python String Contains Character
In many cases, checking for a single character is just as important as checking for substrings. The in keyword again proves useful here.
Example: Single Character Check
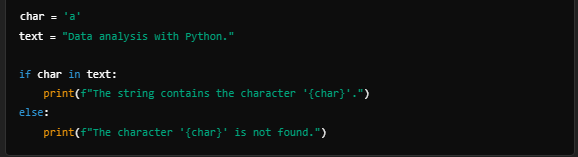
Regex for Character Check
To handle more complex patterns, Python’s re module is your go-to option.
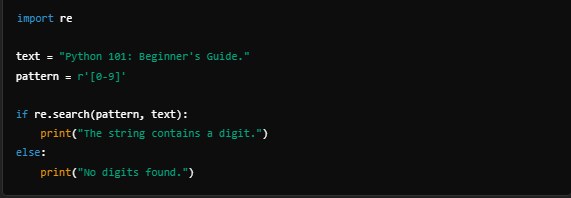
Case Sensitivity in Python String Contains
By default, Python’s in keyword and other methods are case-sensitive. However, you can overcome this by converting the string and substring to lowercase using .lower().
Example: Case-Insensitive Check
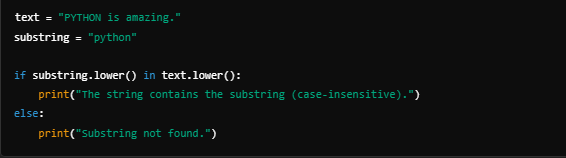
Python String Contains Multiple Substrings Using Regex
Python’s re module provides unparalleled flexibility in searching for multiple patterns simultaneously.
Example: Regex for Multiple Substrings
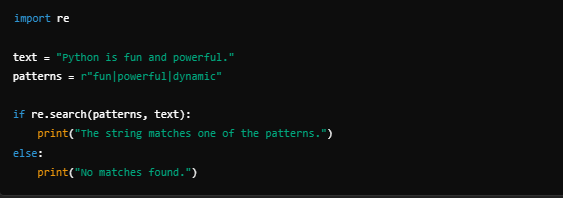
This method is ideal for handling more complex string queries, such as validating formats or parsing data.
External Libraries for String Contains
While Python’s built-in methods are powerful, external libraries like pandas or NumPy are often used for large-scale operations involving strings.
Example: Using Pandas
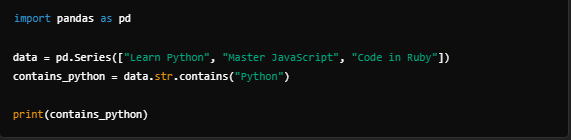
This is particularly useful when working with datasets or text-heavy data in DataFrames.
Real-World Applications
Data Validation
Checking if user inputs contain specific substrings.
Text Analysis
Searching for keywords or patterns in large documents.
Web Scraping
Extracting relevant data by identifying specific substrings in HTML content.
Common Mistakes in String Contains
- Ignoring Case Sensitivity: Always normalize strings before comparison when case isn’t relevant.
- Assuming Exact Matches: Use regex or advanced libraries for approximate matching.
- Overlooking Performance: Avoid nested loops for large datasets; opt for vectorized operations.
Further Reading
- Python Official Documentation: Strings
- Regular Expressions in Python
- Pandas String Methods
Conclusion
Mastering the Python string contains operation equips developers with tools to efficiently handle text data. From simple substring checks to advanced regex matching,
Python offers a variety of approaches tailored to different needs. Explore these methods, and transform your string-handling skills today!
FAQs
How do I check if a string contains a substring in Python?
Use the in keyword: if “substring” in text:.
Can I check for multiple substrings in a string?
Yes, use a loop or any(): any(word in text for word in substrings).
Is the string search in Python case-sensitive?
Yes, but you can normalize with .lower() for case-insensitivity.
What if I want to find multiple patterns?
Use the re module with regex patterns: re.search(“pattern1|pattern2”, text).
How can I check for a single character?
Use char in text to check for any character.
Can I search for substrings in a list of strings?
Yes, iterate through the list or use pandas.Series.str.contains().
What is the fastest method for a simple check?
The in keyword is the fastest and simplest.
Do I need external libraries for string searches?
Not for basic checks, but libraries like pandas help with complex datasets.