The “SyntaxError: cannot use import statement outside a module” is a common issue in JavaScript development, especially when trying to use ES6 module imports in various environments like Node.js or the browser.
If you’ve encountered this error, you are not alone. This error typically occurs in Node.js, browser environments, or when working with JavaScript files that are not configured properly to use ES6 modules.
In this blog post, we will explore what causes this error, how you can fix it, and provide best practices for working with JavaScript modules.
What Is the “SyntaxError: Cannot Use Import Statement Outside a Module” Error?
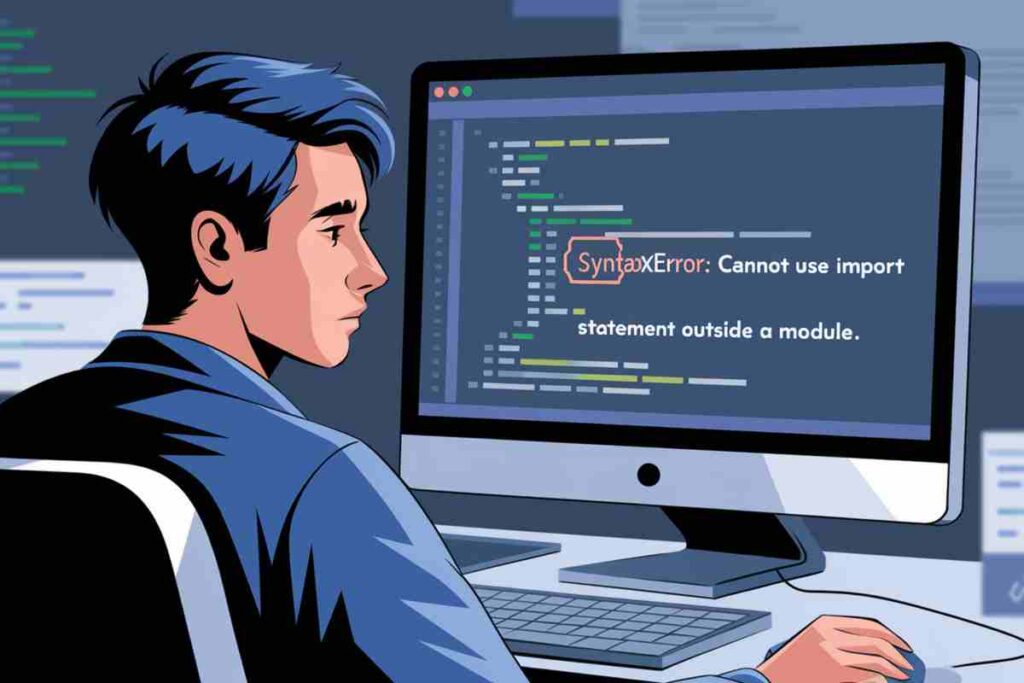
When working with JavaScript in modern applications, ES6 modules (also known as ECMAScript modules) offer a clean way to import and export functionality between different files. This allows for better code organization, reusability, and easier maintenance.
However, when you try to use an ES6 import statement like:

You might encounter the error:

This error typically happens when the JavaScript runtime (like Node.js or the browser) doesn’t know that your script is supposed to be treated as an ES6 module. In simpler terms, the environment you’re working in isn’t set up to support the import
syntax correctly.
Why Does “SyntaxError: Cannot Use Import Statement Outside a Module” Occur?
There are several reasons why this error might occur. Let’s break them down:
Incorrect File Type in Node.js
Node.js, by default, treats JavaScript files as CommonJS modules, not ES6 modules. CommonJS uses require()
to import dependencies, and this can cause issues if you try to use import
instead.
Missing type="module"
in HTML
If you’re working in a browser environment and using ES6 modules, the HTML script tag needs to specify the type as a module. Without this, the browser will assume you’re using a regular script file, which will lead to this error.

Legacy JavaScript Files
If you are working with older JavaScript files or third-party libraries that are not compatible with ES6 modules, they might not support the import
syntax. This can result in unexpected errors if you try to import them in a project set up for ES6 modules.
How to Fix the “SyntaxError: Cannot Use Import Statement Outside a Module” Error?
Now that we understand the possible causes of this issue, let’s explore the solutions. Here are some common fixes:
Solution 1: Configure Node.js to Support ES6 Modules
If you’re using Node.js and trying to use ES6 modules, you need to tell Node to treat your .js
files as modules. You can do this in one of two ways:
- Use
.mjs
Extension: Rename your JavaScript file from.js
to.mjs
. This tells Node.js that the file is an ES6 module. - Update
package.json
: If you want to keep using the.js
extension, you can add"type": "module"
to yourpackage.json
file.
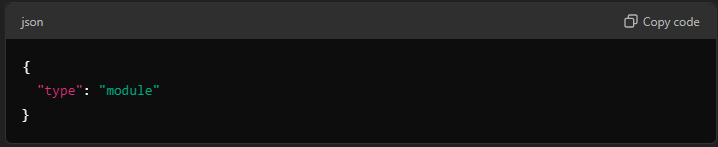
This will allow Node.js to interpret your JavaScript files as ES6 modules, and you can use import
without encountering the "SyntaxError: Cannot use import statement outside a module" error.
Solution 2: Ensure the <script>
Tag Uses the type="module"
If you’re working with JavaScript in the browser, ensure that your script tag is defined as a module in your HTML file:

This tells the browser to treat app.js
as an ES6 module and allows the use of import
statements.
Solution 3: Ensure Compatibility of Imported Libraries
If you’re importing third-party libraries, ensure that they support ES6 modules. Some older libraries may not support import
, and you might need to use require()
instead.
If you are using a bundler like Webpack or Babel, you can often configure it to convert older libraries into a module format.
Solution 4: Use Babel to Transpile ES6 Syntax
If you need compatibility across multiple JavaScript versions, using Babel can help. Babel is a JavaScript compiler that allows you to write modern ES6 code, including imports, and transpile it into older versions of JavaScript for broader compatibility. To use Babel, follow these steps:
Install Babel and its presets:

Create a .babelrc
file in the root directory of your project with the following content:

Use Babel to transpile your code to a compatible version for older environments.
Solution 5: Use Dynamic import()
in Node.js
If you’re working in Node.js and don’t want to change your file structure or configuration, you can use the dynamic import()
syntax, which is available in CommonJS environments.
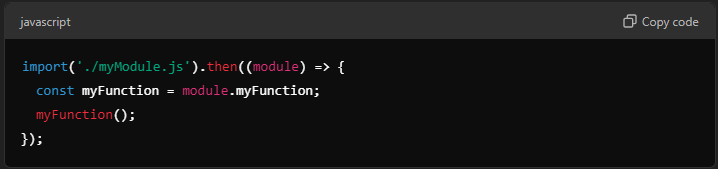
This will allow you to load modules dynamically and use them in a way that’s compatible with older setups.
Best Practices for Working with JavaScript Modules
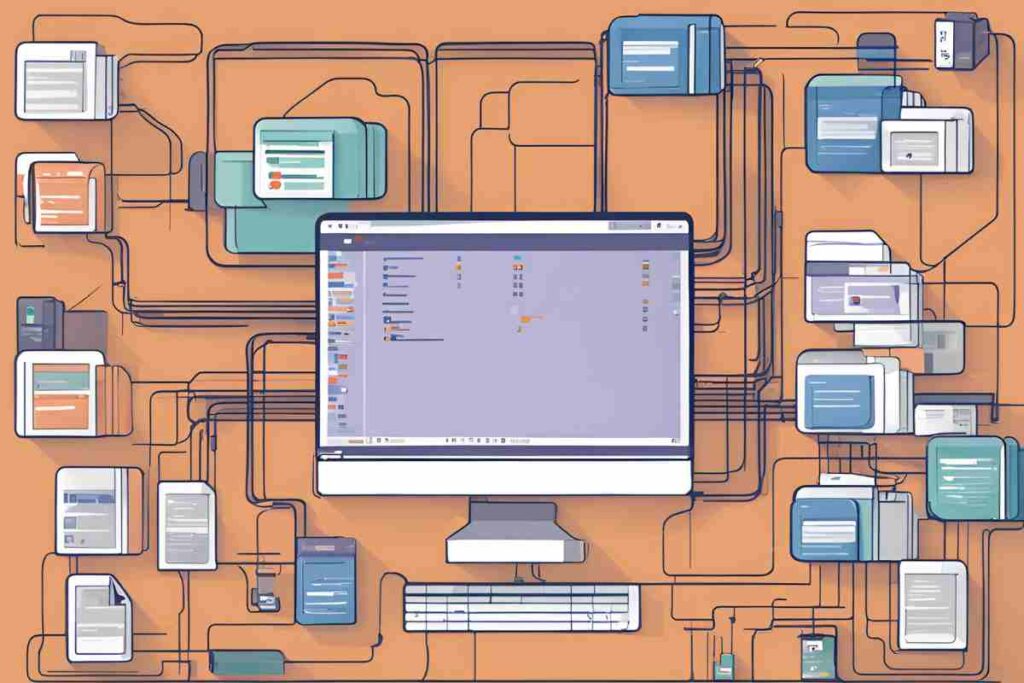
To avoid running into errors like “SyntaxError: Cannot use import statement outside a module,” here are some best practices you can follow when working with JavaScript modules:
Use import
Only in ES6 Modules
Ensure that your files are treated as ES6 modules, either by using the .mjs
extension or by setting "type": "module"
in your package.json
. This avoids confusion and errors down the line.
Use a Bundler Like Webpack or Parcel
If you’re working on a large project, using a bundler like Webpack or Parcel can simplify module management.
These tools automatically handle module resolution and allow you to write modular code that is compatible with various environments.
Stay Updated with JavaScript Specifications
JavaScript is continuously evolving, and new features such as ES6 modules are gaining more widespread support. Keep your environment up to date to ensure compatibility with the latest standards.
Testing and Debugging
Whenever you encounter module-related issues, make sure to test in both Node.js and the browser (if applicable). Use browser developer tools or Node.js’s built-in debugging capabilities to pinpoint the source of the error.
Conclusion
The “SyntaxError: cannot use import statement outside a module” error can be frustrating, but it’s usually easy to fix with the right configuration.
By understanding the causes of this error and following the solutions outlined above, you can successfully use ES6 modules in both Node.js and browser environments.
Remember to keep your environment updated, follow best practices, and use tools like Babel or bundlers to ensure smooth interoperability across different platforms.
FAQs
What does “SyntaxError: Cannot use import statement outside a module” mean?
This error occurs when the environment doesn’t recognize your JavaScript file as an ES6 module.
How can I fix the “cannot use import statement outside a module” error in Node.js?
Add "type": "module"
to your package.json
file or rename your file to use the .mjs
extension.
Why does this error appear in the browser?
In HTML, you need to use <script type="module">
for JavaScript files with import
statements.
Can I use ES6 imports in older JavaScript environments?
Yes, but you’ll need to use Babel or a similar tool to transpile your code.
What’s the difference between CommonJS and ES6 modules?
CommonJS uses require()
, while ES6 modules use import
and export
syntax.
Can I mix import
and require()
in the same file?
Generally, it’s best to use one module system consistently to avoid conflicts.
Do I need a bundler like Webpack to use ES6 modules?
Not necessarily, but bundlers can help manage dependencies and ensure compatibility.
Is there an alternative to import
in CommonJS?
Yes, use require()
in CommonJS environments, like older versions of Node.js.